Javascript the winning style
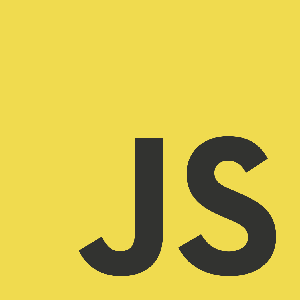
Code written in one style has many advantages: fewer minor errors, many errors are easy to detect almost immediately, while others can be easily debugged at the design stage. New programmers will not have to spend extra time studying your code (this does not mean that they will not have to understand the code itself, which means that it will be easier to read) and it will be easier for them to join the project.
The quality of the code will only get better if the whole team adheres to a predetermined style. It is definitely worth it to spend some time developing common rules.
Unlike Python, which has a single set of rules
for the Style Guide for Python Code , JavaScript does not have this. However, there are as many as 6 popular guides to choose from:
')
In addition to the guides, you should not forget about automatic code analyzers, such as JSLint and JSHint. And they already have their own settings.
The question is, what is the most correct way to write JavaScript code that would be relevant and would most closely match most recommendations? Let's try to combine most of the recommendations in this article and think about how to optimize the code quality verification process.
Registration
Indent
- 2 spaces, no more, and no tabs: Google, npm, Node.js, Idiomatic
- Tab: jQuery
- 4 spaces: Crockford
Space between arguments and expression
Many guides also remind that there are no spaces at the end of lines (trailing spaces)
Line length
- Maximum 80 characters: Google, npm, Node.js, Crockford
When transferring lines, the indentation does not have to be equal to 2, if you need, for example, to transfer function arguments to the next line, you can align them to the place where the first argument stands. Alternatively, you can also use 4 spaces instead of 2 when you move long lines.
- No special opinion: jQuery, Idiomatic
Semicolon
- Always use a semicolon: Google, Node.js, Crockford
- Not use in some situations: npm
- No special opinion: jQuery, Idiomatic
Comments:
- JSDoc : Google, Idiomatic
- Idiomatic Style Guide also allows simpler comments, but JSDoc is preferable
- No special opinion: npm, Node.js, jQuery, Crockford
Quotes
- Single quotes are preferred. Better
'value'
than "value":
Google, Node.js - Double quotes ": jQuery
- No special opinion: npm, Idiomatic, Crockford
Variable declaration
- One variable on one line: Node.js
var foo = ''; var bar = '';
- Several variables, separated by commas at the end of the line, as in the example: Idiomatic, jQuery
var foo = "", bar = "", quux;
- Comma at the beginning of the line: npm
var foo = "" , bar = "" , quux;
- No special opinion: Google, Crockford
Parentheses
Global variables
- Do not use global variables: Google, Crockford
Google:
Global problems of conflicts, try to integrate. In order to prevent common javascript, we've adopted conventions to prevent collisions.
Transfer:
Global variables are harder to debug, and they can cause nontrivial problems when two projects need to be integrated. We accepted the coding style agreement to be able to distribute compatible code.
- Crockford believes that global variables should not be used at all.
- No special opinion: Idiomatic, jQuery, npm, Node
Names
Variables
Constants
Functions
Arrays
- Use the plural form of the word: Idiomatic
var documents = [];
- No special opinion: Google, jQuery, npm, Node, Crockford
Objects and Classes
Other
Use all-lower-hyphen-css-case for multi-part file names and settings: npm
Use JSHint and .jshintrc file
JSHint is a code analyzer that will point you to errors in the code. It is compatible with many widely used text editors. It is also a good way to maintain the stylistic unity and integrity of the code. Various uses can be found in the
documentation . Below is our sample .jshintrc file, which follows all of the above recommendations. Place this file in the root folder of your project, and if you have the JSHint plugin installed, then your editor will now check the code in accordance with the rules you have defined.
{ "camelcase" : true, "indent": 2, "undef": true, "quotmark": "single", "maxlen": 80, "trailing": true, "curly": true }
In all files that are processed by the browser, add:
Add to Node.js files:
In all types of JS files, it is also better to add:
'use strict';
This will affect both JSHint and the JavaScript handler as a whole, which will become less error-tolerant, but will run faster.
Read more about 'use strict' (external link)
Automatic JSHint code checking before git commit
If you want to be sure that all your JS files are validated and follow the general style that you defined in .jshintrc. Then add these lines to your
.git / hooks / pre-commit file. Now, before committing the changes, the script will check only the changed files for violations of the code style. And if any, the operation will be interrupted.
ps.
At the request of those who read (the original article), the
Git repository was created. Anyone can make their own changes to the .jshintrc or documentation.
From the translator:
I personally know the author of the original article and got the go-ahead to make changes when translating. I ask you to inform me of especially serious translation inaccuracies or errors in any way that you like. Perhaps not the most skillful translation, but I tried, thank you who read it all to the end.
Original article: (http://seravo.fi/2013/javascript-the-winning-style)