Good day to all!
Well, I did not write for a long time articles on Habr. Well, I will fix this thing.
In general, ActionBar in Android is a rather complicated thing. In the
first and
second parts I considered only its main functions - the menu and navigation. But there are after all many additional ones: Split ActionBar, custom View for the menu item and ActionProvider. About them and will be discussed. In addition, the bonus: many people know that the Up button is used to switch to the previous Activity. But if the screen can only be accessed from one other Activity, then you can not code the transition up. Intrigued? I ask under the cat.
Split actionbar
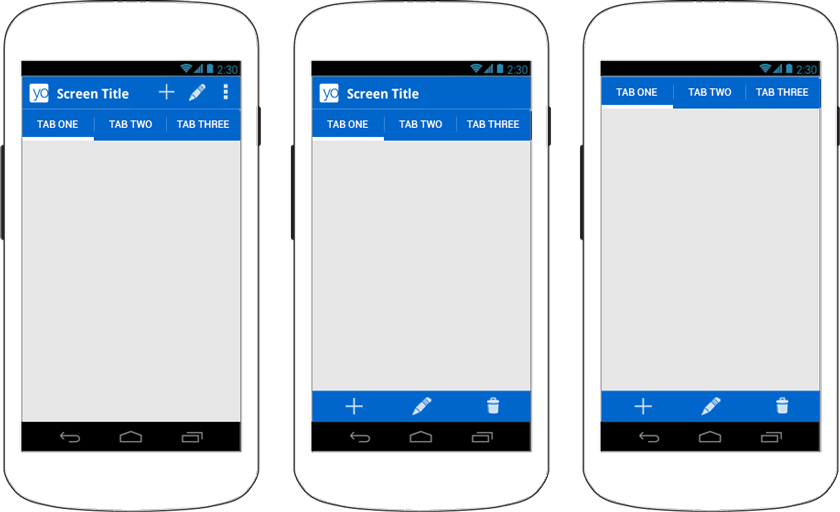
')
Split ActionBar is a bar at the bottom of the screen with menu items. It can be used if, in general, the ActionBar does not have enough space (in the middle), or it is missing (to the right). It is easy to add it: it’s enough to add the following lines for the Activity or for the entire application in the manifest file:
<manifest ...> <activity … uiOptions="splitActionBarWhenNarrow" ... > // API 14+ <meta-data android:name="android.support.UI_OPTIONS" // Support Library API 7+ android:value="splitActionBarWhenNarrow" /> </activity> </manifest>
To remove the top ActionBar (as in the figure on the right), call the ActionBar methods
setDisplayShowHomeEnabled (false) and
setDisplayShowTitleEnabled (false) .
Actionview
By default, the menu item has the form of a picture (icon). There are two ways to change it:
-Add attribute actionLayout for tag. It accepts a link to the layout file as input.
-Add the actionViewClass attribute for the tag, where to write the name of the class, the heir to View, for use instead of the standard view
<menu xmlns:android="http://schemas.android.com/apk/res/android" xmlns:yourapp="http://schemas.android.com/apk/res-auto" > <item android:id="@+id/custom_action " android:title="@string/custom_action " android:icon="@drawable/ic_custom_action " yourapp:actionViewClass="com.you.app.CustomView" /> </menu>
In the android namespace in old versions there are no these attributes, so you need to use the Support Library namespace - for this we add the line
xmlns: yourapp = " schemas.android.com/apk/res-auto " and use the
yourapp attribute
: actionViewClass . You can programmatically change the MenuItem markup using the MenuItemCompat
setActionView (MenuItem item, int resId) and
setActionView (MenuItem item, View view) methods to get the current View -
getActionView (MenuItem item) .
One example of ActionView is SearchView, which is written more about it
here .
Action Provider
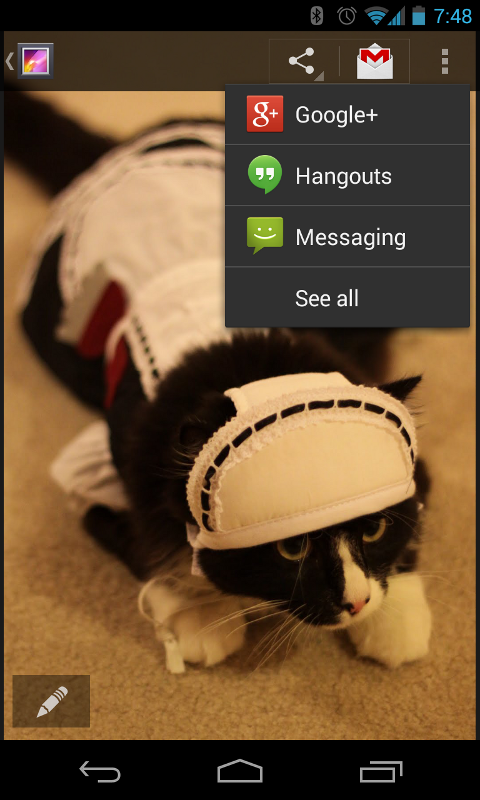
ActionProvider has a lot in common with ActionView. It also replaces the button with its own layout, but can show a drop-down list and control the behavior of the menu item. To set it for a menu item, it’s enough for the tag to add the
actionProviderClass attribute, where to write the address of your class. It must inherit from
ActionProvider and override the following methods:
-
ActionProvider (Context context) - constructor, takes as input a context that needs to be stored in the class field for use in other methods;
-
onCreateActionView (MenuItem) - here we create a view for a menu item using a LayoutInflater, obtained from the context, and return it;
-
onPerformDefaultAction () - called when the user clicks on an item in the ActionBar.
If you use ActionProvider, then you should NOT handle clicks on it in
onOptionsItemSelected () , well, or return false there, because otherwise
onPerformDefaultAction () will not be called.
If your ActionProvider shows a submenu, then you need to override its
hasSubMenu () method and return true. Then instead of
onPerformDefaultAction () will be called
onPrepareSubMenu (SubMenu subMenu) . There you create or change submenus.
ShareActionProvider
Android has its own ActionProvider for sending content -
ShareActionProvider . When we create a menu with it, it is necessary in the onCreateOptionsMenu method to get its instance using
MenuItemCompat.getActionProvider (MenuItem) and call
setShareIntent () , where to transfer Intent with ACTION_SEND and attached content:
private ShareActionProvider mShareActionProvider; @Override public boolean onCreateOptionsMenu(Menu menu) { getMenuInflater().inflate(R.menu.main_activity_actions, menu); Intent share_intent = new Intent(Intent.ACTION_SEND); intent.setType("image/*"); mShareActionProvider = (ShareActionProvider) getActionProvider(menu.findItem(R.id.action_share)); mShareActionProvider.setShareIntent(share_intent); return super.onCreateOptionsMenu(menu); }
Since the content on the screen can change (for example, flipping pictures), then you need to call
setShareIntent () every time the content changes.
Parent Activity
If you can get to the screen in the application only with ONE other Activity, then it makes sense to configure automatic switching to it by pressing the Up button in the ActionBar. It is very simple to do this: add the lines to the manifest file for the desired Activity:
<application ... > <activity android:name="com.you. app.MainActivity" ...> // Activity ... </activity> ... <activity android:name="com.you. app.DetailsActivity" android:label="@string/title_activity_display_message" android:parentActivityName="com.example.myfirstapp.MainActivity" > // API 16+ <meta-data android:name="android.support.PARENT_ACTIVITY" // Support Library API 7+ android:value="com.you. app.MainActivity" /> </activity> </application>
Remember that the Home button works as Up, call the SupportActionBar methods
setHomeButtonEnabled (true) and
setDisplayHomeAsUpEnabled (true) .
For API 11+If your application only supports Android 3 or higher, then instead of using the MenuItemCompat method, use the same for MenuItem, instead of SupportActionBar - ActionBar. In addition, all attributes in the menu will begin with android:, and the line xmlns: yourapp = http: //schemas.android.com/apk/res-auto is not needed.
All information is taken
from here (official guide in English).
Part 1 - Adding Support Library to a project, simple example, search
Part 2 - Navigation with tabs and drop-down list