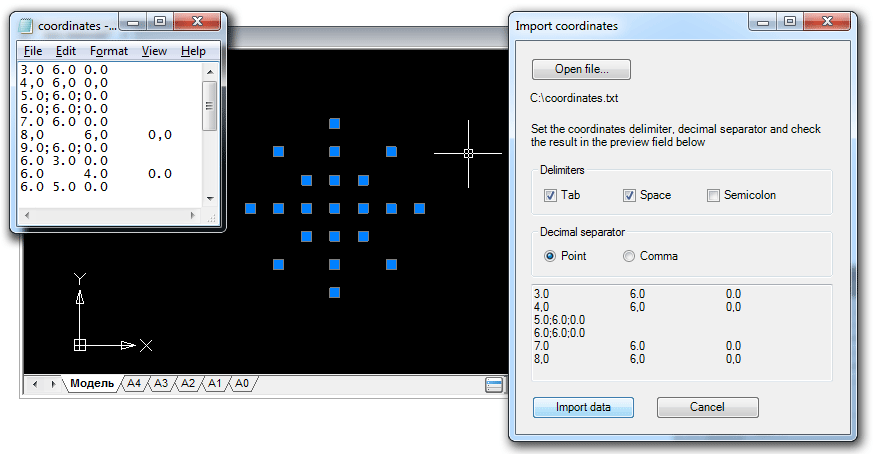
One of the most popular questions about programming under nanoCAD is “How do I import points from a text file?”. The task is simple, but a professional designer does not have to be a professional programmer, so we wrote this article in the “for beginners” style.
You can import coordinates into a drawing on any of the existing API types in nanoCAD. We decided to choose .NET and compare two close APIs: the classic .NET API and the cross-CAD system platform MultiCAD.NET API. Under the cut - the first part - import points on the classic .NET API.
Given: a text file with the coordinates X, Y, Z points, one point on the line. The coordinates are separated by a space, the fraction separator is a period.
')
Required: write an application that, using the IMPORTCOORDS command, queries the file name and imports the coordinates found into the current drawing space in the form of
DatabaseServices.DBPoint
objects. Object coordinates should be imported in the current user coordinate system (UCS) of the drawing.
Creating and setting up a working draft
To create the application, we need the following tools:
- nanoCAD (version not lower than 3.5)
- Microsoft Visual Studio 2008 (nanoCAD 3.5 - nanoCAD 5.0 supports the download of .NET applications built on the .NET Framework 3.5).
And, of course, it means that you can program in C # at least a little. If not, welcome to
the MSDN library .
Create a new project in Visual Studio with the following settings:
- Project type: Visual C #
- Template: Class Library
Thus, our application is a regular .NET assembly (DLL), which will later be loaded into nanoCAD.
In the References tab, we include the following libraries that are part of the nanoCAD package:
Now you can safely proceed to writing the program itself.
Program structure
The implementation can be broken down into the following steps:
- Register the IMPORTCOORDS command.
- Get the current drawing database and command line editor.
- Request the name of the file with the coordinates.
- Open the file, read the lines with coordinates.
- Create DBPoint objects with individual coordinates. Convert their coordinates to the current user coordinate system.
- Add created objects to the current drawing space (Model Space or Paper Space).
In order to register the command that will call our application in nanoCAD, you need to define the
[CommandMethod]
attribute and specify the name of the command before defining the method to be called by this command. Note that the method must have a public modifier:
[CommandMethod("IMPORTCOORDS")] public void importCoords() { ... }
Before proceeding, I would like to stop and briefly tell you what a “drawing database” is. The .dwg file is a database with a strict structure, the main elements of which are the Symbol Tables, which contain all the drawing objects. These are not only graphic objects that we see in the drawing (straight lines, arcs, points, etc.), but also many other objects that define the contents and settings of the drawing. For example, the Layer Table contains all the layers that are in the drawing, the Linetype Table stores all the line styles defined in the drawing, the User Coordinate Systems Table (UCS Table) - all coordinate systems created by the user. for this drawing, etc. Thus, to create a new drawing object is to create the corresponding database object.
So, we continue. First of all, we need to select the current one from all open documents and open its database. To do this, we get the object manager of all open documents, and then with its help and the database with which we will continue to work.
DocumentCollection dm = Application.DocumentManager; Database db = dm.MdiActiveDocument.Database;
In order for our application to request the file name, you need to get the Editor object and call a method that requests user input of a certain type (in our case, the file name):
Retrieving coordinates from a file is quite simple using C # functionality for reading text files and working with string data types:
We proceed to the creation of graphic primitives (Entity). As noted above, in order to create any object (not only a graphic one) that will be stored in a drawing, it must be added to the drawing database, namely, to the corresponding container object. For example, all layers are stored as records in a layer table (Layer Table), which in this case is a container object for them. The overall database structure is as follows:

Graphic primitives are stored in the database not directly, but in the structure of individual blocks, which in turn are entries in the Block Table. This is very convenient, since such a mechanism makes it easy to group objects into named blocks and manage them as a single entity. By the way, the model space and the leaf space in the database are also represented by separate blocks. Thus, for the graphic primitive, the container will be a separate block, which, in turn, will belong to the parent object - the block table.
Since we work with the database, it is necessary to ensure its integrity and protection in case some error occurred during the execution of the program. The transaction mechanism is used for this purpose. Transactions combine a whole range of operations that are performed as a single unit: if something goes wrong, the transaction is canceled and objects created as part of this transaction will not be added to the document. If all operations have completed successfully, the transaction is confirmed, and the objects are added to the database.
Armed with this knowledge, we can safely add “point” primitives to the current drawing space along the coordinates we read from the file.
using (Transaction tr = db.TransactionManager.StartTransaction()) {
The task is almost solved. It remains to fulfill one condition: primitive points must be created in the coordinates of the user coordinate system (UCS). It should be noted that primitives are stored in the drawing database in the world coordinate system (WCS). Therefore, when creating primitives it is necessary to perform the transformation: UCS-> WCS. This is done using the matrix of the user coordinate system:
Matrix3d ucsMatrix = ed.CurrentUserCoordinateSystem;
Add the transform:
{ ... point.TransformBy(ucsMatrix.Inverse()); ... }
So, the program is completely written. What next?
Application loading in nanoCAD
The most pleasant part is to download the program to nanoCAD and enjoy the results of your work. As you remember, we created a working project as a class library, so after successful compilation an assembly with the name of your project will be built. We open nanoCAD, in the command line we write the NETLOAD command, select the built library from the list and load it. To run the program, simply type the name of the IMPORTCOORDS command in the command line.
Import coordinates. Version 2.0
Let's improve the first version of the application, adding some useful functions and elements of the user interface.
If the first version of the application "understood" a text file in which coordinates are separated only by spaces and a dot was used as a decimal separator, then the application will be able to "recognize" coordinates separated by a tabulation symbol, space or semicolon. As for the decimal separator, now both a dot and a comma can act as a decimal separator; imports will be made without taking into account regional settings. The IMPORTCOORDS command will now open a modal coordinate import dialog in which the user can select a file and specify the desired coordinate import settings.
The general mechanism for importing coordinates and creating primitives remains almost unchanged, but now it will occur within the form class, and the task of the IMPORTCOORDS command handler method now comes down to creating a form object and displaying the form on the screen as a modal dialog:
[CommandMethod("IMPORTCOORDS")] public void importCoords() { Form form = new ImportForm(); HostMgd.ApplicationServices.Application.ShowModalDialog(form); }
After that the control will be transferred to the window of the form of import of coordinates.
Application form
The application form includes the following elements:
- Button to open the file
- File Open Dialog
- Group of checkboxes for selecting coordinate separator characters: tab, space, semicolon
- Text field for previewing parse of lines with coordinates
- Button to import coordinates
- Cancel button
Using these controls, the user can now specify the desired delimiter characters, check the result in the preview field (approximately as done in MS Excel when importing a text file) and initiate the coordinate import:
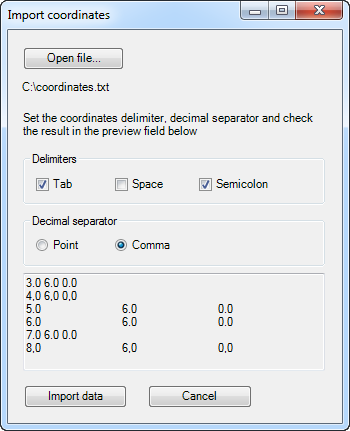
AutoCAD Compatibility
In conclusion, I would like to note that the application written for nanoCAD can be easily recompiled to work in AutoCAD. To do this, do the following:
Both versions of the project are available
here .
Discussion of the article is also available on our forum:
forum.nanocad.ru/index.php?showtopic=6508 .
Translation of the article into English:
Importing coordinates using the classic .NET API .