Introduction
Firebug adds a global variable called “console” to each webpage loaded in Firefox. This object contains many methods that allow you to write to the Firebug console and display information passing through the scripts.
firebug.ruFortunately, not only firebug has this functionality:
- Chrome Javascript console - almost exact analog
- Opera Dragonfly console - partially implemented functionality
- Firefox Web console - partially implemented functionality
This article is the Console API manual.
Examples are given from

Chrome 28.0.1500.72 m,

Firebug 1.11.3,

Firefox 22.0,

Opera 12.15 (version before leaving presto)
Content
Manual
console.log (object [, object, ...])

+

+

±

±
Displays the arguments passed by the message to the console. If more than one argument is given, separates them with a space.
This is the most commonly used method of the console object.
Examplevar a = ' '; console.log(a); console.log(' ', 12, true, [1,2,3], {a:1,b:2}); console.log('Node', document.getElementsByTagName('body')); console.log('DOM', document); console.log('', alert); console.log('', NaN, null, undefined);
Screenshots
Chrome ->

Firebug ->

Firefox ->

Opera
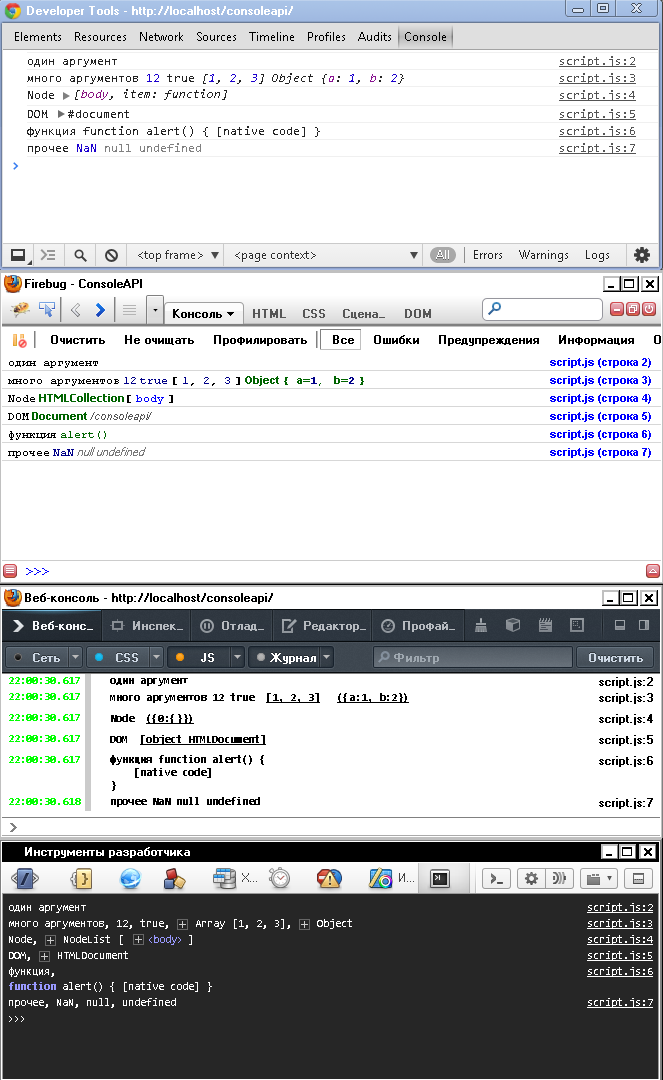
In all the above console implementations, it is possible to click on a click to view the structure of complex elements, such as arrays, objects, DOM elements, etc.
')
There is a special case of using
console.log with several arguments.
The first argument may contain substitution patterns as in
printf () , but they are much smaller than in the implementation of this function in C, PHP etc.

±

±

±

-
Pattern | Description |
---|
% s | Line. |
% d or % i | Number. |
% f | Floating point number Firebug's official documentation is blatantly lying "(numeric formatting is not yet supported)" - don’t believe it
|
% o | DOM element Firebug displays as a link to the item. Chrome, in addition to the link, also knows how to display this element in the console itself. In the current version 28.0.1500.72 m there is a bug (?) - each time updating the page, the DOM console is displayed alternately as DOM, then as a Javascript object. Firefox on click, opens the item in a modal window. (all attributes and methods of this object are available in it).
|
% O | JavaScript object Not supported in Firefox. Chrome in the case when not just an object gets into% O, but DOM converts it into a js object (all methods and attributes are available). Firebug does not distinguish% o from% O.
|
% c | css style (color, background, font). Not supported in Firefox.
|
Example console.log(' %d %s',10,''); console.log(' %f',Math.PI); console.log('%c %c %c%c ','color:red;','font-size:16px;color:orange;','background:black;color:yellow;','font:normal;color:normal;background:normal;'); console.log('body as DOM: %o',document.getElementsByTagName('body')[0]); console.log('object: %O',{a:1,b:2}); console.log('body as Object: %O',document.getElementsByTagName('body')[0]);
comment from streetstriderThe bottom line is that the console object can be implemented differently in different environments. In some cases, the object is implemented with full, albeit native functions, that is, if you try to display console.log.toString (); we get instead of the source code of the [native code] function. In some environments, as I understand it, this object simply provides things that can be called with parentheses, but they are not full functions: they cannot be called with call / apply / bind. From examples: Chrome debugger and IE debugger.
The main disadvantage:
Let there is an EventEmitter and we want to activate its activation. To do this, it is enough to sign some logger on it, for example console.log. object.on('event', console.log);
That is, console.log will be called with all parameters with which any callback on this emitter is called. You can replace it with console.dir, then in some environments (Node.js) there will be a more detailed output of the first parameter. In any case, if console.log, console.dir are not functions, they cannot be called, you have to write a binding. Moreover, in the case of console.log, the binding will be incomplete: we will not be able to pass all the parameters, because we cannot make apply.
function log (a1, a2, a3) { console.log(a1, a2, a3);
And there is only one solution - without grace.
In general, functions must be functions.
console.assert (expression [, object, ...])

+

+

-

±
If
expression is wrong, outputs
console.error ; otherwise, it does not output anything.
About differences throw new Error () from console.error () in more detail in console.errorIf there are more arguments after the expression, then:
- Chrome - displays them separated by a space.
- Firebug - additionally displays an array of all remaining elements.
In Opera, if
expression == false , it works like
console.log , otherwise it doesn't output anything.
Example console.assert(2>1); console.assert(1>2); console.assert(2>1,' '); console.assert(1>2,' '); console.assert(1>2,'',''); console.assert(1>2,' : [%s]',''); console.assert(1>2,'','',1,2,3,{a:1});
console.clear ()

+

+

+

+
Clears the console. Examples will not be.
console.count ([object])

±

+

-

±
Displays the number of times this code has been executed. If the optional
object argument is passed, then the value of the counter is the output of
object.toString () **.
All implementations have their own characteristics:
- Chrome combines multiple console.count with the same object.toString () result.
- Firebug - does not display several console.count separately, if they are in a loop.
- Opera ignores complex objects instead of casting them to a string value.
Does not display a link to the string, with the current call to the console.
I do not think the implementation of Chrome is inaccurate, even if I put it ±. Just the look of google programmers on this feature is different from firebug programmers.Example console.count(); console.count();
console.debug (object [, object, ...])

±

±

±

±
Alias on
console.log .
It exists for compatibility with the ancient version of the Console API, in which
console.debug differed from
console.log in that it added an additional link to the line in which the console call occurred. In the current API implementation,
console.log does the same.
In Chrome - displays text in blue (text is displayed in dark gray in
console.log ).
Example var a = ' '; console.debug(a); console.debug(' ', 12, true, [1,2,3], {a:1,b:2}); console.debug('Node', document.getElementsByTagName('body')); console.debug('DOM', document); console.debug('', alert); console.debug('', NaN, null, undefined); console.debug(' %d %s',10,'');
console.dir (object)

±

±

±

+
Displays the passed value as a Javascript object (for DOM elements, all their attributes and methods are output).
Similarly,
% O works in
console.log in Chrome.
As in the case of
console.count , everyone has their own approach to the implementation of this API method:
- Chrome - simple arguments, which do not have any methods or attributes, displays all the same as objects.
- Firebug - ignores all simple argument types (String, Number, Boolean).
The output is the same as in the DOM tab.
Does not display a link to the string, with the current call to the console.
- Firefox - works like Chrome.
The output is the same as in modal windows.
- Opera - can take more than 1 argument.
Simple arguments are rendered in the same way as console.log .
In the derivation, it separates the methods and attributes into the classes parents and grandparents (noticeable in the derivation of [1,2] and {a: 1}).
Example console.dir(' '); console.dir([1,2]); console.dir({a:1}); console.dir([1,2],{a:1}); console.dir(document);
console.dirxml (object)

±

±

-

±
Displays the XML for the element.
- Chrome - works identical to console.log with the % o pattern and is buggy as well (:
- Firebug - on all elements except DOM displays <> \ n </>.
- Opera - if the arguments are objects, but not DOM - displays their type and a reference to the current line in the code.
In version 12.15, upon receipt of 2 or more complex objects (for example, two arrays, or an object and an array), the dragonfly engine hangs, due to which it has to be restarted.
Example console.dirxml(' '); console.dirxml([1,2]); console.dirxml({a:1}); console.dirxml(document); console.dirxml([1,2],{a:1});
console.error (object)

+

±

±

±
Displays the error and the result of
console.trace for the place from which it was called.
! Patterns as in
console.log are supported.
Implementation Differences:
- Chrome - displays nothing if no argument has been passed.
Ignores message from error (if you pass an object of type Error).
- Firebug - the result of console.trace and the code that caused the error will output only if exactly 1 argument is passed.
- Firefox - the output differs from console.log only by a small cross on the left. Does not display console.trace .
- Opera - outputting everything that is not an object of type Error and its subclasses differs from console.log only in the red color of the text. Does not display console.trace .
Objects of type Error and its subclasses in turn are displayed in great detail.
In version 12.15, when I try to output console.error with no arguments, it is not displayed, but a link to the line on the right is displayed, which knocks the link of the next line to the side.
! It is important to know:
- throw new Error ('mesasge') - stops the execution of JavaScript code.
- console.error ('message') - just displays an error message without interrupting the rest of the code.
console.exception (error-object [, object, ...])

-

±

-

±
Displays the error and
trace () result for the place from which it was called.
One of the most ambiguous methods:
- Firebug - unlike console.error , is functionally far from console.log . Does not support patterns , all arguments except the first one are output to the next line in one array.
In the case when no arguments are passed, instead of message it displays “Error in approval”.
- Opera - because of attempts to implement a trace - takes everything apart. The rest works as console.error .
console.group (object [, object, ...])

+

+

±

+
Opens a new
expanded group of entries in the console.
! Patterns are supported as in
console.logIn Firefox, groups cannot be collapsed or expanded.
console.groupCollapsed (object [, object, ...])

+

+

-

+
Opens a new group immediately
collapsed .
in Firefox - creates an expanded group.
console.groupEnd ()

+

+

+

+
Closes an open group.
Example console.log(' '); console.group();
console.info (object [, object, ...])

±

+

+

+
Displays log with minor visual changes.
! Patterns are supported as in
console.log- Chrome - no change. Why in debug , and not here the lines are displayed in blue? Because fuck you, that's why!
- Firebug - puts an icon with a blue exclamation mark to the left of the output, highlights the line with blue.
- Firefox - puts an icon with an exclamation mark in the circle to the left of the output.
- Opera - the message is displayed in blue text.
Example var a = ' '; console.info(a); console.info(' ', 12, true, [1,2,3], {a:1,b:2}); console.info('Node', document.getElementsByTagName('body')); console.info('DOM', document); console.info('', alert); console.info('', NaN, null, undefined); console.info(' %d %s',10,'');
console.profile ([title])

+

+

-

-
Includes a Javascript profiler.
Code profiling helps you track which part of it takes the longest. Useful tool for debugging.
console.profileEnd ()

+

+

-

-
Turns off the Javascript profiler
- Chrome - displays 2 messages, in the second - a link to the profile.
- Firebug - the profile is displayed directly in the console.
- Firefox - profiling engine is, but this console method is not supported.
- Opera - displays a message stating that support has not yet been implemented.
Example console.profile(); (function someFunction() { for (i=0;i<10000000;i++) {var s='a'; s+='a'; delete s;} })() console.profileEnd(); console.profile(" "); (function someFunction() { for (i=0;i<10000000;i++) {var s='a'; s+='a'; delete s;} })() console.profileEnd();
console.table (data [, columns])

±

+

-

-
Displays a table.
In
data , an array or an array-like object is expected.
The optional
columns argument is used to set the names of the columns of the table, as well as for flexible control of the output of an array-like object.
(This functionality deserves a separate article. For details, go where the official documentation sends you.)
Chrome does not know how to display complex values, substituting for them such text as “Array [3]”, Object, etc.
Example var table = []; table[0] = [1,'2',[1,2,3]]; table[1] = [document,document.getElementsByTagName('body'),window]; table[2] = [{a:1},null,alert]; console.table(table); function Person(firstName, lastName, age) { this.firstName = firstName; this.lastName = lastName; this.age = age; } var family = {}; family.mother = new Person("", "", 32); family.father = new Person("", "", 33); family.daughter = new Person("", "", 5); family.son = new Person("", "", 8); console.table(family);
console.time (name)

±

±

±

±
Creates a new timer and binds it to
name . In this case, the
name applies
toString () **.
- Chrome throws an error if no arguments have been passed.
- Firebug - throws an error if no arguments were passed.
- Firefox - ignores calls without arguments.
- Opera - ignores calls without arguments.
In version 12.15, the output to the console is stopped, without throwing an error, in cases where several elements were passed, or as an argument DOM-object.
console.timeEnd (name)

±

±

±

±
Stops the timer
associated with
name.toString () and displays the elapsed time in the console
thanks to mithgol for the correction .
console.timeStamp (name)

-

±

-

-
Displays the current timestamp with the text that was passed to the
name .
Opera throws an error if it hits a string with
console.timeStamp () ; Chrome and Firefox - they are simply ignored.
Example console.timeStamp(); console.time(' '); (function someFunction() {for (i=0;i<1000000;i++) {var s='a'; s+='a'; delete s;}})() console.time(' '); (function someFunction() {for (i=0;i<1000000;i++) {var s='a'; s+='a'; delete s;}})() console.timeEnd(' '); (function someFunction() {for (i=0;i<1000000;i++) {var s='a'; s+='a'; delete s;}})() console.timeEnd(' '); console.timeStamp(' '); console.time(12); (function someFunction() {for (i=0;i<1000000;i++) {var s='a'; s+='a'; delete s;}})() console.timeEnd(12); console.time(document.getElementsByTagName('script')); (function someFunction() {for (i=0;i<1000000;i++) {var s='a'; s+='a'; delete s;}})() console.timeEnd(document.getElementsByTagName('body')); console.time(1,2,3);
For Opera tests, the lines with timeStamp had to be commented out.
console.trace ()

+

+

±

±
Displays the call stack leading to the execution of this code (it is also displayed when error messages are reported).
Takes no arguments.
- Firefox - instead of the stack displays a link to the line where the code was called + the name of the function that called it.
- Opera - displays a very strange way.
Example console.trace(); (function firstlevel () { (function secondlevel () { (function lastlevel () { console.trace(' '); })(); })(); })();
console.warn (object [, object, ...])

+

+

+

+
Like
console.info , it displays
console.log with minor visual changes.
! Patterns are supported as in
console.log- Chrome - puts an icon with an exclamation mark to the left of the output.
- Firebug - puts an icon with a yellow exclamation mark to the left of the output, highlights the line with yellow.
- Firefox - puts an icon with an exclamation point in the triangle to the left of the output.
- Opera - the message is displayed in orange text.
Example var a = ' '; console.info(a); console.info(' ', 12, true, [1,2,3], {a:1,b:2}); console.info('Node', document.getElementsByTagName('body')); console.info('DOM', document); console.info('', alert); console.info('', NaN, null, undefined); console.info(' %d %s',10,'');
Conclusion
Console is a very powerful and useful tool for web developers. I advise you to remember at least the main points:
useful links
* Rather, "Common Console API" because some methods support Firebug worse than Chrome.
** However, due to the
toString method, it does not distinguish calls with
document.getElementsByTagName ('script') argument from calls with
document.getElementsByTagName ('body') , etc.