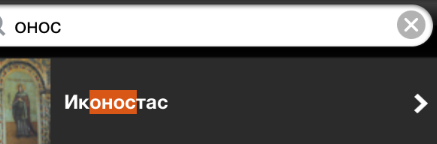
Recently, a task has appeared - to build in the application a dynamic information search, that is, the output should be generated in parallel with the user entering characters. All information is stored in a SQLite database. Everything would be fine, but in each word of the output the required characters should be highlighted.
The decision was made not to use external libraries, but to try to do it on their own.
Let's start with the external form.
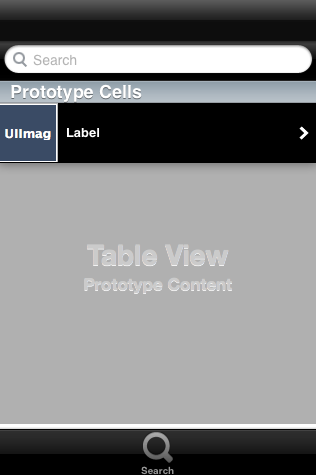
')
UISearchBar is used as a search string. The results are displayed in the usual
UITableView (in this case, with cells, in addition to the text that includes the image).
The handler for a text change event in a string is:
- (void)searchBar:(UISearchBar *)searchBar textDidChange:(NSString *)searchText { _data = [SBHotspotData findHotspot:searchText]; [self.searchResultsTableView reloadData]; }
where _data is NSArray storing the output results.At this stage, a problem arose - in all the names of the objects being searched, there are both uppercase and lowercase letters that SQLite perceives differently, and the
lower () and
upper () functions, unlike SQL, are not supported. By the least resistance, we decided to add a separate column to the database, which stores all the same headings, but in lower case, especially since in this case the increase in the base volume was almost imperceptible. Search method (FMDatabase is used for working with the database):
+ (NSArray *) findHotspot: (NSString *)partOfTitle { SBHotspotData *item; NSMutableArray *result = [NSMutableArray array]; NSString *path = [self getDatabasePath]; FMDatabase *database; database = [FMDatabase databaseWithPath:path]; [database open]; NSString *query = [NSString stringWithFormat:@"select * from hotspots where TitleLow like '%%%@%%'", [partOfTitle lowercaseString]]; FMResultSet *results = [database executeQuery:query]; while([results next]) { item = [[SBHotspotData alloc ]init]; item.hotspotIdentity = [results stringForColumn:@"Identity"]; item.hotspotTitle = [results stringForColumn:@"Title"]; item.hotspotDescription = [results stringForColumn:@"Description"]; [result addObject:item]; } [database close]; return [result sortedArrayUsingSelector:@selector(compare:)]; } - (NSComparisonResult)compare:(SBHotspotData *)otherObject { return [self.hotspotTitle compare:otherObject.hotspotTitle]; }
When generating a query,
%%% @ %% is used , where percent signs indicate that any number of characters can appear before and after the line. The method returns an array of objects from the database, sorted alphabetically.
The most interesting part of the task is highlighting the entered characters. It is implemented as follows:
NSMutableAttributedString *attributedString = [[NSMutableAttributedString alloc] initWithString:item.hotspotTitle]; NSRange range = [item.hotspotTitle rangeOfString:_searchHotspotBar.text]; [attributedString addAttribute:NSBackgroundColorAttributeName value:[UIColor colorWithRed:216/255.0f green:87/255.0f blue:23/255.0f alpha:1.0f] range:range]; cell.cellLabel.attributedText = attributedString;
NSMutableAttributedString and its method
addAttribute: value: range: are used to highlight certain characters.
Well, the result of the program:
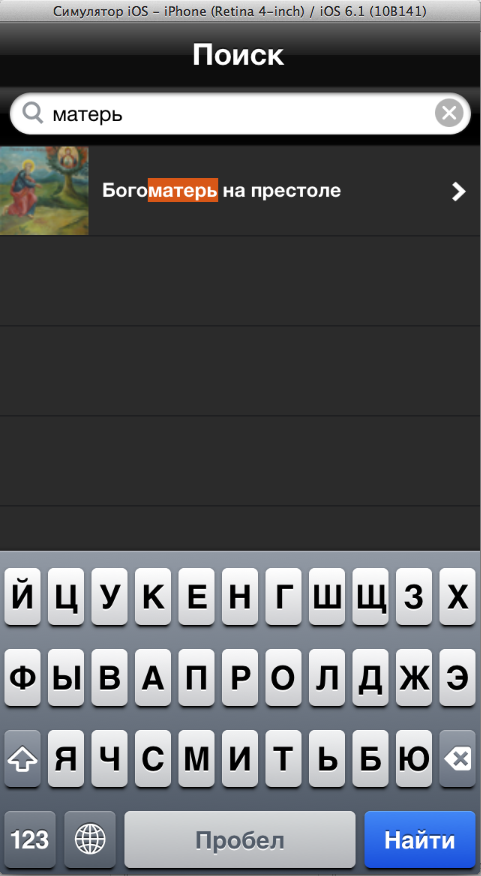
The task turned out to be quite trivial, but, I hope, the experience gained will be useful to someone from the Habrovo community.