While developing a mobile application, I discovered a couple of things, knowledge of which would be useful to me when I started. Therefore, I decided to write an article in the format of small tips. These tips are more focused on developing mobile apps for PhoneGap, Windows 8 and Firefox OS. But mobile web developers can come in handy too. At the end of the article I will give links to what eventually happened.
Do not trust media selectors.
Especially on Android. Media selectors work when using the keyboard and you have to handle it. It starts to be a real hell if our application has a different look for Portrait and Landscape. This is well demonstrated by the following example:
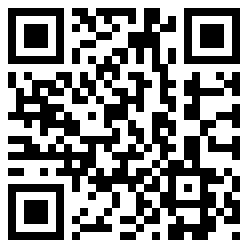
If we open our example on the phone with a resolution of 800px and more, we will not see a color block, since A media selector is applied to it, but when we click on input to enter data, the keyboard opens and a block appears (the window height changes), which is a bit of a logical flow of business.
Solution: Using Javascript to change the orientation and orientation change based on the properties of screen.availWidth and screen.availHeight. Please note that these properties do not work in FirefoxOS, as the orientationchange event. Such code can help us save some time and effort.
var displayModeLandscape = false; var width = 0; var height = 0; var setPortrait = function() { $('html').addClass('portrait').removeClass('landscape'); displayModeLandscape = false; }; var setLandscape = function() { $('html').addClass('landscape').removeClass('portrait'); displayModeLandscape = true; }; var currentOrientation = function() { width = screen.availWidth || $(window).width(); height = screen.availHeight || $(window).height(); if(height > width) { setPortrait(); } else { setLandscape(); } }; $(window).on('orientationchange', currentOrientation); currentOrientation();
Extend reset.css
To the standard reset.css it will be useful to add a style that overrides the selection of elements at tapes. It does not look awkward and not naive.
*:not(html) { -webkit-user-select: none; -moz-user-select: none; user-select: none; -webkit-tap-highlight-color: rgba(255, 255, 255, 0); }
')
Use graphic acceleration.
To optimize the animations in your application, you will need properties that support graphic acceleration. These include: transform, opacity. Pay attention to the transform css property and try to use it as an alternative to left, top, right in case you are going to make a transition of these properties. Transform works much faster.
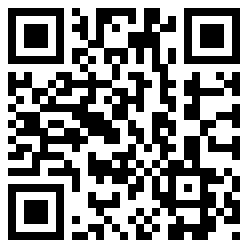
Use tapas, not clicks.
I already wrote about the delay of 300ms when processing clicks in mobile browsers. Therefore, use the event tache and tapes to handle clicks on the elements. About gestures more
in the relevant article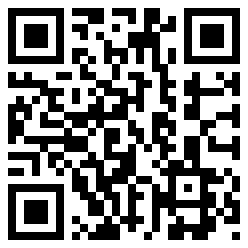
As you can see from the example, the difference between the processing of touch-events and click-effects on the desktop is ~ 100 ms and ~ 400 on mobile browsers.
LocalStorage and Caching
Use LocalStorage to cache user information, as well as to store information on the device. LocalStorage is a persistant storage and remains when the application is updated. But do not forget about restrictions, for example, the maximum size on iOs is 5MB or that the version of webkit on Android <3.2 throws an exception for JSON.parse (null), all other browsers return null.
Use suitable tools.
Use the appropriate browser for testing applications. If you are developing an application for Android or iOS, use Chrome, under Firefox OS — Firefox, under Windows 8 — IE10. To develop games and applications with a large number of animations,
https://github.com/mrdoob/stats.js/ will also be useful. Or you can use the built-in Chrome FPS counter. All you need to do is log in to
chrome: // flags / and turn it on. Also, Chrome emulates tapes - this can be very useful in the debugging of gestures.
Prohibit scaling
By inserting the following tag into the head tag, you disable scaling and you will be able to track gestures.
<meta name="viewport" content="user-scalable=no, width=device-width, initial-scale=1, maximum-scale=1">
Optimization of gestures.
Run preventDefault () and stopPropagination () when you no longer need gesture processing to increase application performance.
Optimize your code.
All of the above will not make sense if your code is run insanely long. Use Profiler to find thin spots, try to update the DOM as little as possible and always remove or hide (display: none) nodes that you don’t use. The more DOM you have, the slower the operations are with it.
Instead of an afterword
Well, in the end you can look at the application live on
Google Play