<AbsoluteLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent"> <Button android:id="@+id/backbutton" android:text="Back" android:layout_x="10px" android:layout_y="5px" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TextView android:layout_x="10px" android:layout_y="110px" android:text="First Name" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <EditText android:layout_x="150px" android:layout_y="100px" android:width="100px" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TextView android:layout_x="10px" android:layout_y="160px" android:text="Last Name" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <EditText android:layout_x="150px" android:layout_y="150px" android:width="100px" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </AbsoluteLayout>
<FrameLayout android:layout_width="fill_parent" android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android"> <ImageView android:src="@drawable/icon" android:scaleType="fitCenter" android:layout_height="fill_parent" android:layout_width="fill_parent"/> <TextView android:text="Learn-Android.com" android:textSize="24sp" android:textColor="#000000" android:layout_height="fill_parent" android:layout_width="fill_parent" android:gravity="center"/> </FrameLayout>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button" /> <Button android:id="@+id/button3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button" /> </LinearLayout>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="horizontal" android:layout_width="fill_parent" android:layout_height="fill_parent"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button" /> <Button android:id="@+id/button3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button" /> </LinearLayout>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="horizontal" android:layout_width="fill_parent" android:layout_height="fill_parent"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button" /> <Button android:id="@+id/button3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button" /> <LinearLayout android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button" /> <Button android:id="@+id/button3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button" /> </LinearLayout> </LinearLayout>
<RelativeLayout android:layout_width="fill_parent" android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android"> <Button android:id="@+id/backbutton" android:text="Back" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TextView android:id="@+id/firstName" android:text="First Name" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@id/backbutton" /> <EditText android:id="@+id/editFirstName" android:width="100px" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_toRightOf="@id/firstName" android:layout_below="@id/backbutton"/> <EditText android:id="@+id/editLastName" android:width="100px" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@id/editFirstName" android:layout_alignLeft="@id/editFirstName"/> <TextView android:id="@+id/lastName" android:text="Last Name" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignBaseline = "@id/editLastName" android:layout_below="@id/editFirstName" /> </RelativeLayout>
, .
android:layout_span.
, , android:layout_column
<TableLayout android:layout_width="fill_parent" android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android"> <TableRow> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column1" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column2" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column 3" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> <TableRow> <TextView android:id="@+id/textViewSpan" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text=" span three column" android:layout_span = "3" android:textSize="23sp" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> </TableLayout>
Alternate Layouts
Alternate Layouts - . .
XML :
res/layout-land โ landscape UI
res/layout-port โ portrait UI
res/lauout-square โ square UI
res/lauout .
.
.
:
<TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:textColor="#00FF00" android:typeface="monospace" android:text="@string/hello" />
xml res/values/
:
<?xml version="1.0" encoding="utf-8"?> <resources> <style name="CodeFont" parent="@android:style/TextAppearance.Medium"> <item name="android:layout_width">fill_parent</item> <item name="android:layout_height">wrap_content</item> <item name="android:textColor">#00FF00</item> <item name="android:typeface">monospace</item> </style> </resources>
, style.
<TextView style="@style/CodeFont" android:text="@string/hello" />
, .
android:layout_span.
, , android:layout_column
<TableLayout android:layout_width="fill_parent" android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android"> <TableRow> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column1" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column2" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column 3" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> <TableRow> <TextView android:id="@+id/textViewSpan" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text=" span three column" android:layout_span = "3" android:textSize="23sp" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> </TableLayout>
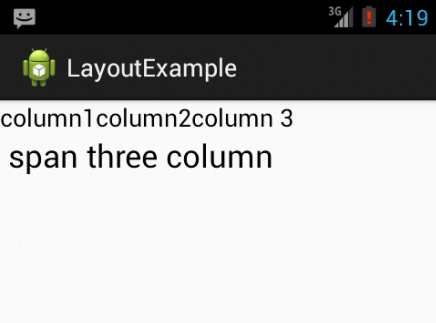
Alternate Layouts
Alternate Layouts - . .
XML :
res/layout-land โ landscape UI
res/layout-port โ portrait UI
res/lauout-square โ square UI
res/lauout .
.
.
:
<TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:textColor="#00FF00" android:typeface="monospace" android:text="@string/hello" />
xml res/values/
:
<?xml version="1.0" encoding="utf-8"?> <resources> <style name="CodeFont" parent="@android:style/TextAppearance.Medium"> <item name="android:layout_width">fill_parent</item> <item name="android:layout_height">wrap_content</item> <item name="android:textColor">#00FF00</item> <item name="android:typeface">monospace</item> </style> </resources>
, style.
<TextView style="@style/CodeFont" android:text="@string/hello" />
, .
android:layout_span.
, , android:layout_column
<TableLayout android:layout_width="fill_parent" android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android"> <TableRow> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column1" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column2" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column 3" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> <TableRow> <TextView android:id="@+id/textViewSpan" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text=" span three column" android:layout_span = "3" android:textSize="23sp" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> </TableLayout>
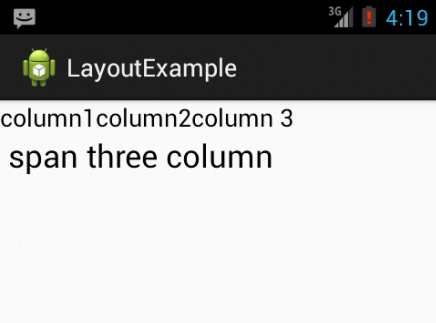
Alternate Layouts
Alternate Layouts - . .
XML :
res/layout-land โ landscape UI
res/layout-port โ portrait UI
res/lauout-square โ square UI
res/lauout .
.
.
:
<TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:textColor="#00FF00" android:typeface="monospace" android:text="@string/hello" />
xml res/values/
:
<?xml version="1.0" encoding="utf-8"?> <resources> <style name="CodeFont" parent="@android:style/TextAppearance.Medium"> <item name="android:layout_width">fill_parent</item> <item name="android:layout_height">wrap_content</item> <item name="android:textColor">#00FF00</item> <item name="android:typeface">monospace</item> </style> </resources>
, style.
<TextView style="@style/CodeFont" android:text="@string/hello" />
, .
android:layout_span.
, , android:layout_column
<TableLayout android:layout_width="fill_parent" android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android"> <TableRow> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column1" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column2" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column 3" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> <TableRow> <TextView android:id="@+id/textViewSpan" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text=" span three column" android:layout_span = "3" android:textSize="23sp" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> </TableLayout>
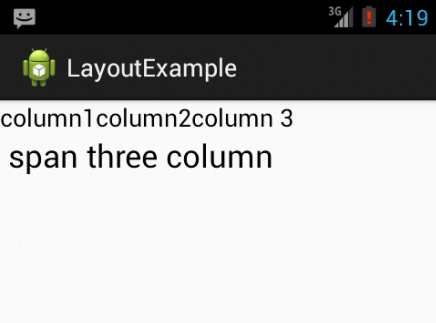
Alternate Layouts
Alternate Layouts - . .
XML :
res/layout-land โ landscape UI
res/layout-port โ portrait UI
res/lauout-square โ square UI
res/lauout .
.
.
:
<TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:textColor="#00FF00" android:typeface="monospace" android:text="@string/hello" />
xml res/values/
:
<?xml version="1.0" encoding="utf-8"?> <resources> <style name="CodeFont" parent="@android:style/TextAppearance.Medium"> <item name="android:layout_width">fill_parent</item> <item name="android:layout_height">wrap_content</item> <item name="android:textColor">#00FF00</item> <item name="android:typeface">monospace</item> </style> </resources>
, style.
<TextView style="@style/CodeFont" android:text="@string/hello" />
, .
android:layout_span.
, , android:layout_column
<TableLayout android:layout_width="fill_parent" android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android"> <TableRow> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column1" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column2" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column 3" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> <TableRow> <TextView android:id="@+id/textViewSpan" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text=" span three column" android:layout_span = "3" android:textSize="23sp" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> </TableLayout>
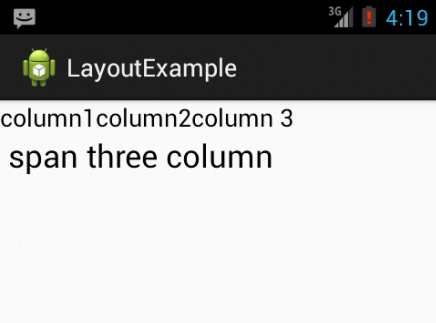
Alternate Layouts
Alternate Layouts - . .
XML :
res/layout-land โ landscape UI
res/layout-port โ portrait UI
res/lauout-square โ square UI
res/lauout .
.
.
:
<TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:textColor="#00FF00" android:typeface="monospace" android:text="@string/hello" />
xml res/values/
:
<?xml version="1.0" encoding="utf-8"?> <resources> <style name="CodeFont" parent="@android:style/TextAppearance.Medium"> <item name="android:layout_width">fill_parent</item> <item name="android:layout_height">wrap_content</item> <item name="android:textColor">#00FF00</item> <item name="android:typeface">monospace</item> </style> </resources>
, style.
<TextView style="@style/CodeFont" android:text="@string/hello" />
, .
android:layout_span.
, , android:layout_column
<TableLayout android:layout_width="fill_parent" android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android"> <TableRow> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column1" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column2" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column 3" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> <TableRow> <TextView android:id="@+id/textViewSpan" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text=" span three column" android:layout_span = "3" android:textSize="23sp" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> </TableLayout>
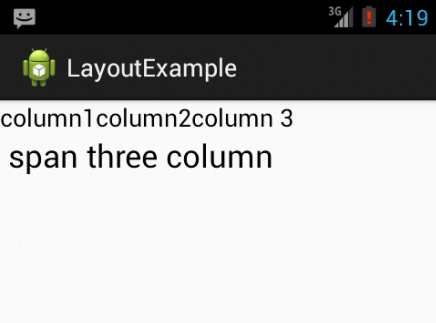
Alternate Layouts
Alternate Layouts - . .
XML :
res/layout-land โ landscape UI
res/layout-port โ portrait UI
res/lauout-square โ square UI
res/lauout .
.
.
:
<TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:textColor="#00FF00" android:typeface="monospace" android:text="@string/hello" />
xml res/values/
:
<?xml version="1.0" encoding="utf-8"?> <resources> <style name="CodeFont" parent="@android:style/TextAppearance.Medium"> <item name="android:layout_width">fill_parent</item> <item name="android:layout_height">wrap_content</item> <item name="android:textColor">#00FF00</item> <item name="android:typeface">monospace</item> </style> </resources>
, style.
<TextView style="@style/CodeFont" android:text="@string/hello" />
, .
android:layout_span.
, , android:layout_column
<TableLayout android:layout_width="fill_parent" android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android"> <TableRow> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column1" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column2" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column 3" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> <TableRow> <TextView android:id="@+id/textViewSpan" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text=" span three column" android:layout_span = "3" android:textSize="23sp" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> </TableLayout>
Alternate Layouts
Alternate Layouts - . .
XML :
res/layout-land โ landscape UI
res/layout-port โ portrait UI
res/lauout-square โ square UI
res/lauout .
.
.
:
<TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:textColor="#00FF00" android:typeface="monospace" android:text="@string/hello" />
xml res/values/
:
<?xml version="1.0" encoding="utf-8"?> <resources> <style name="CodeFont" parent="@android:style/TextAppearance.Medium"> <item name="android:layout_width">fill_parent</item> <item name="android:layout_height">wrap_content</item> <item name="android:textColor">#00FF00</item> <item name="android:typeface">monospace</item> </style> </resources>
, style.
<TextView style="@style/CodeFont" android:text="@string/hello" />
, .
android:layout_span.
, , android:layout_column
<TableLayout android:layout_width="fill_parent" android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android"> <TableRow> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column1" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column2" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column 3" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> <TableRow> <TextView android:id="@+id/textViewSpan" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text=" span three column" android:layout_span = "3" android:textSize="23sp" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> </TableLayout>
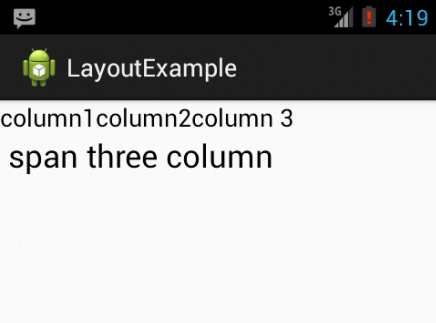
Alternate Layouts
Alternate Layouts - . .
XML :
res/layout-land โ landscape UI
res/layout-port โ portrait UI
res/lauout-square โ square UI
res/lauout .
.
.
:
<TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:textColor="#00FF00" android:typeface="monospace" android:text="@string/hello" />
xml res/values/
:
<?xml version="1.0" encoding="utf-8"?> <resources> <style name="CodeFont" parent="@android:style/TextAppearance.Medium"> <item name="android:layout_width">fill_parent</item> <item name="android:layout_height">wrap_content</item> <item name="android:textColor">#00FF00</item> <item name="android:typeface">monospace</item> </style> </resources>
, style.
<TextView style="@style/CodeFont" android:text="@string/hello" />
, .
android:layout_span.
, , android:layout_column
<TableLayout android:layout_width="fill_parent" android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android"> <TableRow> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column1" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column2" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column 3" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> <TableRow> <TextView android:id="@+id/textViewSpan" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text=" span three column" android:layout_span = "3" android:textSize="23sp" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> </TableLayout>
Alternate Layouts
Alternate Layouts - . .
XML :
res/layout-land โ landscape UI
res/layout-port โ portrait UI
res/lauout-square โ square UI
res/lauout .
.
.
:
<TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:textColor="#00FF00" android:typeface="monospace" android:text="@string/hello" />
xml res/values/
:
<?xml version="1.0" encoding="utf-8"?> <resources> <style name="CodeFont" parent="@android:style/TextAppearance.Medium"> <item name="android:layout_width">fill_parent</item> <item name="android:layout_height">wrap_content</item> <item name="android:textColor">#00FF00</item> <item name="android:typeface">monospace</item> </style> </resources>
, style.
<TextView style="@style/CodeFont" android:text="@string/hello" />
, .
android:layout_span.
, , android:layout_column
<TableLayout android:layout_width="fill_parent" android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android"> <TableRow> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column1" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column2" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column 3" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> <TableRow> <TextView android:id="@+id/textViewSpan" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text=" span three column" android:layout_span = "3" android:textSize="23sp" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> </TableLayout>
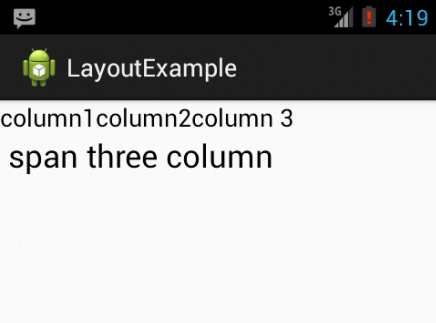
Alternate Layouts
Alternate Layouts - . .
XML :
res/layout-land โ landscape UI
res/layout-port โ portrait UI
res/lauout-square โ square UI
res/lauout .
.
.
:
<TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:textColor="#00FF00" android:typeface="monospace" android:text="@string/hello" />
xml res/values/
:
<?xml version="1.0" encoding="utf-8"?> <resources> <style name="CodeFont" parent="@android:style/TextAppearance.Medium"> <item name="android:layout_width">fill_parent</item> <item name="android:layout_height">wrap_content</item> <item name="android:textColor">#00FF00</item> <item name="android:typeface">monospace</item> </style> </resources>
, style.
<TextView style="@style/CodeFont" android:text="@string/hello" />
, .
android:layout_span.
, , android:layout_column
<TableLayout android:layout_width="fill_parent" android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android"> <TableRow> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column1" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column2" android:textAppearance="?android:attr/textAppearanceMedium" /> <TextView android:id="@+id/textView3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="column 3" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> <TableRow> <TextView android:id="@+id/textViewSpan" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text=" span three column" android:layout_span = "3" android:textSize="23sp" android:textAppearance="?android:attr/textAppearanceMedium"/> </TableRow> </TableLayout>
Alternate Layouts
Alternate Layouts - . .
XML :
res/layout-land โ landscape UI
res/layout-port โ portrait UI
res/lauout-square โ square UI
res/lauout .
.
.
:
<TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:textColor="#00FF00" android:typeface="monospace" android:text="@string/hello" />
xml res/values/
:
<?xml version="1.0" encoding="utf-8"?> <resources> <style name="CodeFont" parent="@android:style/TextAppearance.Medium"> <item name="android:layout_width">fill_parent</item> <item name="android:layout_height">wrap_content</item> <item name="android:textColor">#00FF00</item> <item name="android:typeface">monospace</item> </style> </resources>
, style.
<TextView style="@style/CodeFont" android:text="@string/hello" />
Source: https://habr.com/ru/post/181820/
All Articles