Starting Windows Phone 8 Development: Lesson 1. Application Layout and Event HandlerThe beginning of Windows Phone 8 Development: lesson 2. Access to the local storage applicationThe beginning of Windows Phone 8 Development: lesson 3. Navigating through pages and passing parametersThe beginning of Windows Phone 8 Development: lesson 4. Communication with services and data bindingAs promised, by the end of this lesson you will already feel almost like a real developer under Windows Phone 8). Now we can build GUI using XAML, create event handlers, navigate through pages, save data in local storage. All that is left to master is to create real applications - this is data binding and interaction between services.
What is data binding?
Remember the description of the news application from lesson 3? We have a lot of news that needs to be shown to the user. The first thing you need to figure out is what the news will look like and how to show it to the user. As for me, the news should consist of a headline, a brief description, maybe a picture, and something like the Details button.
')
The process of binding news to the UI (user interface) - this is the process of data binding.
However, due to well-known reasons, we cannot show all the news at once with all the details on one page, since the user will have to use a long enough scroll to read everything. This is solved by using two pages - on just one news list, on the other - the details of the selected news.
How to make data binding?
First we define the list box. Note that you can specify exactly how the list will look inside the ListBox.ItemTemplate tag. The DataTemplate will contain a data binding plan. To bind the control, we first find the attribute that needs to be bound, for example, a picture (image) to the Image control, and we will bind the text to the TextBlock control.
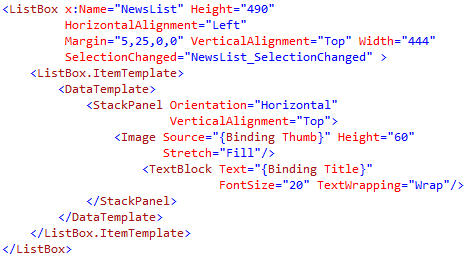
Code<ListBox x:Name="NewsList" Height="490" HorizontalAlignment="Left" Margin="5,25,0,0" VerticalAlignment="Top" Width="444" SelectionChanged="NewsList_SelectionChange" > <ListBox.ItemTemplate> <DataTemplate> <StackPanel Orientation="Horizontal" VerticalAlignment="Top" > <Image Sourse="{Binding Thumb}" Height="60" Stretch="Fill" > <TextBlock Text="{Binding Title}" FontSize="20" TextWrapping="Wrap" > </StackPanel> </DataTemplate> </ListBox.ItemTemplate> </ListBox>
Here I use StackPanel for the content items template: the picture, and next to it is the TextBlock. It looks like this:
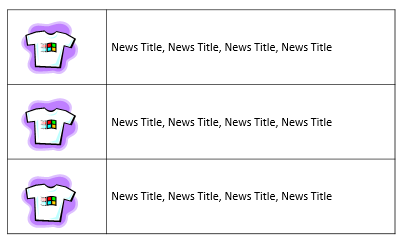
Using WCF service
I won't tell you what WCF is, but I'll tell you what this service does. Lazy loading code and checking it. I have a class called WindowsPhoneLessonNews, which contains the properties Title, Content, Thumb. There are also 2 operations: one to download all the news, and the second - to download the news on its index.
Add a link to our service so that you can use it. Right click on References and select Add Service Reference.

My services are located on the local service with port 8080. Go to the service and select it. I have the service name Lesson4PhoneNewsService.

Now that we’ve added a link to the service, we can start using it. For a start, let's see what happens when the page loads. Most likely, you have already noticed that I use an asynchronous call to receive all the news. Due to the use of an asynchronous call, you must make sure that we do not block the GUI until we receive a response from the service.

Code private void PhoneApplicationPage_Loaded_1(object sender, RoutedEventArgs e) { NewsServiceClient newsClient = new NewsServiceClient; newsClient.GetAllNewsCompleted += newsClient_GetAllNewsCompleted; newsClient.GetAllNewsAsync(); ProgressLoader.Visibility = Visibility.Visible; ProgressLoader.IsIndeterminate = true; }
That the download would not be too boring for the user - we’ll add a download bar: it will animate while data is being received, if there is no data retrieval, then the bar becomes invisible.

Code </ListBox.ItemTemplate> </listBox> <ProgressBar x:namespace="ProgressLoader" Height="10" Visibility="Collapsed"/>
Another feature of asynchronous programming is that you need to tell the application what to do after receiving a response from the service. I will simply assign the ItemsSource of our ListBox response result that we get from the service and hide the download bar.

Code void newsClient_GetAllNewsCompleted(object sender, GetAllNewsCompletedEventArgs e) { NewsList.ItemSourse = e.Result; NewsList.SelectedIndex = -1; ProgressLoader.Visibility = Visibility.Collapsed; ProgressLoader.IsIndeterminate = false; }
I also know that the service returns a list (list), so I immediately attach the answer to the ListBox.
And what happens if you click on the news?
Let's create an event handler when you click on the news. We check the validity of the index and redirect the user to the page, while simultaneously passing the index as a parameter, where full information is displayed. Thus, the NewsDetails page will be able to determine which particular news to show in full.
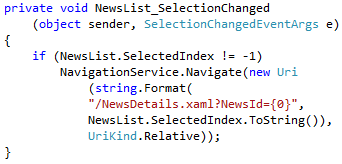
Code private void News_List_SelectionChanged(object sender, SelectionChangedEventArgs e) { if(NewsList.SelectedIndex != 1) NavigationService.Navigate(new Uri(string.Format("/NewsDetails.xaml?NewsId={0}", NewsList.SelectedIndex.ToString()), UriKind.Relative)); }

Code private void PhoneApplicationPage_Loaded_1(object sender, RoutedEventArgs e) { NewsServiceClient client = new NewsServiceClient(); client.GetNewsByIDCompleted += client_GetNewsByIDCompleted; client.GetNewsByIDAsunc(int.Parse(NavigationContext.QueryString["NewsID"].ToString())); }
And almost the same when it comes to an asynchronous call:

Code void client_GetNewsByIDCompleted(object sender, GetNewsByIDCompletedEventArgs e) { List<WindowsPhoneLessonNews> selectedNews = new List<WindowsPhoneLessonNews>(); selectedNews.Add(e.Result); NewsList.ItemSource = selectedNews; }
If you want to return to the previous page, press the Back button on the phone.
Work on the appearance
The following is the layout of the main page, consisting of TextBlock, which says that the content is obtained from the web service and so on ....

Code <StackPanel x:Name="ContentPanel" Grid.Row="1" Margin="12,0,12,0"> <TextBlock Text="List is being populated from the web service, tap" TextWrapping="Wrap" Padding="0,0,10,0"> </Textlock> <ListBox x:Name="WewsList" Height="490" HorizontalAlignment="Left" Margin="5,25,0,0" VerticalAlignment="Top" Width="444" SelectionChanged="WewsList_SelectionChanged" > <ListBox.ItemTemplate> <DataTemplate> <StackPanel Orientation="Horizontal" VerticalAlignment="Top"> <Image Source="{Binding Thumb}" Height="60" Stretch="Fill"/> <TextBlock Text="{Binding Title}" FontSize="20" TextWrapping="Wrap"/> </StackPanel> </DataTemplate> </ListBox.ItemTemplate> </ListBox> <ProgressBar x:Name="ProgressLoader" Height="10" Visibility="Collapsed"/> </StackPanel>
NewsDetails page. Almost identical to the XAML of the main page. The orientation of the StackPanel is changed to vertical, the images are cut out and the news content is added. And the font size is changed to 40 for the title.
We are testing!

If you click on the desired news, a page with detailed information will open:

Let's sum up all 4 lessons.
And so, now you have enough knowledge to write your application for Windows Phone 8 using XAML, a visual page editor, layouts, event handlers, navigation, data binding.
I hope you have helped my lessons and you can build on them to self-develop and self-improve.
Good luck.