Table of contents
- Introduction
- Initializing Prism Applications
- Manage dependencies between components
- Modular Application Development
- Implementation of the MVVM pattern
- Advanced MVVM scripts
- Creating user interface
- User Interface Design Recommendations
- Navigation
- View-Based Navigation (View-Based Navigation)
- The interaction between loosely coupled components
The
Model-View-ViewModel pattern (MVVM, model-view-view model) helps you to separate business logic and view logic from the user interface. Supporting the division of responsibility between application logic and UI can make your application easier to test, support, and develop. It can also significantly improve the reusability of the code and allow developers and designers to collaborate more easily when developing relevant parts of the application.
Using the MVVM pattern, the application user interface, presentation logic, and business logic are divided into three separate classes. This is a view that encapsulates the UI and its logic, a view model that encapsulates view logic and its states, and a model that encapsulates the business logic of the application and data.
Prism includes examples and sample implementations that show how to implement the MVVM pattern in Silverlight or in WPF applications. The Prism library also provides functions that can help implement this pattern. These functions embody the most common methods for implementing the MVVM pattern and are designed to provide testability and compatibility with Expression Blend and Visual Studio.
')
This chapter gives a brief overview of the MVVM pattern and describes how to implement it. Chapter 6 describes how to implement more complex MVVM scripts using the Prism library.
Responsibilities and Characteristics of Classes
The MVVM pattern is a close variation of the
Presentation Model pattern, optimized to better align with some of the basic WPF and Silverlight features, such as data binding, data patterns, commands, and behavior.
In the MVVM pattern, the view encapsulates the UI and any UI logic, the view model encapsulates view logic and its states, and the model encapsulates business logic and data. The view interacts with the view model through data binding, command, and notification events. The view model requests data from the model, or subscribe to notifications about their changes, and coordinates updates of the state of the model, and also converts, validates and aggregates, if necessary, the data to display them in the view.
The following illustration shows the three parts of the MVVM pattern and their interaction.
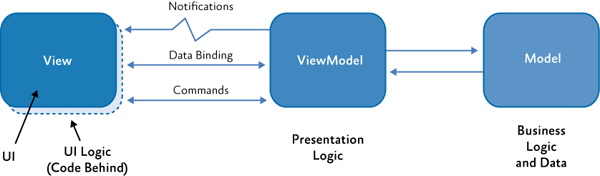
As with all patterns of shared views, the key to effectively using the MVVM pattern lies in understanding how to divide your application code into the correct classes, and in understanding the ways in which these classes interact in different scenarios. The following sections describe the responsibilities and characteristics of each of the classes in the MVVM pattern.
View Class
The presentation is responsible for determining what the user sees on the screen. Ideally, the code-behind view contains only the constructor, which calls the
InitializeComponent
method. In some cases, the code-behind may contain UI logic code that implements visual behavior that is difficult or ineffective to express in XAML, such as complex animations, or when the code must directly control the visual elements that are part of the presentation. It is not allowed to put logic code that needs testing into a representation. Typically, the code in the code-behind view can be tested through UI automation.
In Silverlight and WPF, data binding expressions in a view are evaluated against its data context. In MVVM, the view model is set as the view data context. The view model implements properties and commands to which the view can be associated, and notifies the view of any state changes through change notification events. Usually there is a direct relationship between the view and its view model.
Views are generally inherited from the
Control
or
UserControl
classes. However, in some cases, a view may be represented by a data template that defines the elements of the UI that will be used to visually represent the object. Using data templates, the developer can easily define how the presentation model will be rendered, or can change its default visual presentation without changing the underlying object or its behavior directly.
Data templates can be thought of as representations that lack code-behind. They are designed to bind to certain types of the view model when it should be mapped to the UI. At run time, the view, as defined by the template, is automatically created and the corresponding view model is set by its data context.
In WPF, you can associate a data template with an application-level view model. Then WPF will automatically apply this data template to any objects of the representation model of the specified type whenever they appear in the UI. This is known as implicit data patterns. In Silverlight, you must explicitly define the data template for the view model object within the control that is supposed to display it. The data template can be defined as embedded in the control that uses it, or defined in the resource dictionary outside the parent view and declaratively combined with the view resource dictionary.
Summing up, the presentation has the following key characteristics:
- A view is a visual element, such as a window, page, user control, or data template. The view defines the controls, their layout and style.
- A view references a view model through its
DataContext
property. The controls in the view are bound to the properties and commands of the view model. - The view can customize the data binding behavior between the view and the view model. For example, a view can use value converters to format the data that will be shown in the UI, or use validation rules to provide additional validation of user input.
- The view defines and processes the visual behavior of the UI, such as animations or transitions, which can be triggered by changing the state of the view model, or through user interaction with the UI.
- Code-behind views can define UI logic to implement some visual behavior that is difficult to express in XAML, or which requires direct references to certain UI controls defined in the view.
View Model Class
The view model in the MVVM pattern encapsulates view logic and data for display. She has no direct reference to the representation or knowledge of the implementation or type of representation. The view model implements properties and commands to which the view can bind data and notifies the view of any state changes through notification events. The properties and commands that the view model provides determine the functionality required by the UI, but the view determines how this functionality will be presented to the user.
The view model is responsible for coordinating the interaction of the view with the required classes of the model. Often, there is a one-to-many relationship between the view model and the model classes. A view model can substitute model classes directly into views so that controls in the view can bind data directly to them. In this case, model classes must be designed to support data binding and the corresponding change notification events. For more information about this scenario, see the “Data Binding” section later in this topic.
A view model can transform or manipulate model data so that the view can easily use it. The view model may have additional properties for the specific needs of the view. These properties usually cannot be part of the model. For example, the view model can combine the value of two fields to make it easier to display them on the screen, or it can calculate the number of remaining characters as you type for fields with a limited string length. The view model can also implement data validation logic to ensure their consistency.
The view model can also define logical states that the view can use for visual changes in the UI. A view can define markup or style changes that reflect the state of the view model. For example, the view model may have a state that indicates that data is asynchronously transmitted to the web service. The view can show animation during this state to provide visual feedback to the user.
Typically, the view model defines commands or actions that can be represented in the UI and invoked by the user. A typical example is when the view model provides the
Submit
command, which allows the user to submit data to a web service or repository. A view can present this command as a button so that the user can press it to transfer data. As a rule, when a command becomes unavailable, its associated view in the UI becomes disabled. Commands allow you to encapsulate user actions and separate them from their visual presentation.
In summary, the presentation model has the following key characteristics:
- It is not a visual class and is not inherited from any WPF or Silverlight base class. It encapsulates the presentation logic needed to support user actions in the application. The view model is tested independently of the view and model.
- Usually does not directly refer to the presentation. It implements the properties and commands to which the view can bind data. It notifies the view of any state changes through the notification events of the
INotifyPropertyChanged
and INotifyCollectionChanged
. - Coordinates the interaction of the view with the model. It can transform or manipulate data so that it can be easily used by the view, and it can implement additional properties that may not be present in the model. It can also implement data validation via the
IDataErrorInfo
or INotifyDataErrorInfo
. - It can determine the logical states that the view can visually present to the user.
The note. View or view model?
Often the definition of where a particular functionality should be implemented is not obvious. The general rule is: Anything related to a particular visual display and that can be upgraded later (even if you are not currently planning to upgrade it) should be included in the presentation; anything important to the logic of the application should go into the view model. In addition, since the view model should not have any explicitly defined knowledge of certain visual elements in the view, code to programmatically control visual elements within the view must be in the view code-behind or encapsulated in behaviors ( Behaviors ). Similarly, the code for getting or manipulating the data items that should be displayed in a view through data binding must be in the view model. For example, the highlight color of the selected item in the list box must be defined in the view, but the list of items to display and a link to the selected item must be defined in the view model.
Model Class
The model in the MVVM pattern encapsulates business logic and data. Business logic is defined as any application logic that deals with extracting and managing application data and in order to make sure that business rules are satisfied that guarantee the consistency and validity of the data. To maximize reusability, models should not contain presentation-specific information, logic, or behavior.
Typically, the model represents the essence of the subject area of ​​the client part of the application. It can contain data structures based on the application's data model and any business and validation logic. A model may also have access to data and a cache, although usually a separate data repository or the corresponding service is used for this. Often, the model and data access layer are automatically generated as part of the ADO.NET Entity Framework infrastructure, WCF Data Services, or WCF RIA Services.
Typically, the model implements the means to facilitate binding to the view. This usually means that notifications of changes to properties or collections are supported via the
INotifyPropertyChanged
and
INotifyCollectionChanged
. Classes of models that provide collections of objects are typically inherited from the
ObservableCollection, INotifyCollectionChanged . IDataErrorInfo
( INotifyDataErrorInfo
). WPF Silverlight UI, . UI.
, ?
, INotifyPropertyChanged
, INotifyCollectionChanged
, IDataErrorInfo
, INotifyDataErrorInfo
. , , . . , , .
:
, -. , - . , . INotifyPropertyChanged
INotifyCollectionChanged
. . , , ObservableCollection.
IDataErrorInfo
, INotifyDataErrorInfo
.
, .
class
ObservableCollection, INotifyCollectionChanged
. IDataErrorInfo
( INotifyDataErrorInfo
). WPF Silverlight UI, . UI.
, ?
, INotifyPropertyChanged
, INotifyCollectionChanged
, IDataErrorInfo
, INotifyDataErrorInfo
. , , . . , , .
:
, -. , - . , . INotifyPropertyChanged
INotifyCollectionChanged
. . , , ObservableCollection.
IDataErrorInfo
, INotifyDataErrorInfo
.
, .
ObservableCollection, INotifyCollectionChanged
. IDataErrorInfo
( INotifyDataErrorInfo
). WPF Silverlight UI, . UI.
, ?
, INotifyPropertyChanged
, INotifyCollectionChanged
, IDataErrorInfo
, INotifyDataErrorInfo
. , , . . , , .
:
, -. , - . , . INotifyPropertyChanged
INotifyCollectionChanged
. . , , ObservableCollection.
IDataErrorInfo
, INotifyDataErrorInfo
.
, .
ObservableCollection, INotifyCollectionChanged
. IDataErrorInfo
( INotifyDataErrorInfo
). WPF Silverlight UI, . UI.
, ?
, INotifyPropertyChanged
, INotifyCollectionChanged
, IDataErrorInfo
, INotifyDataErrorInfo
. , , . . , , .
:
, -. , - . , . INotifyPropertyChanged
INotifyCollectionChanged
. . , , ObservableCollection.
IDataErrorInfo
, INotifyDataErrorInfo
.
, .
ObservableCollection, INotifyCollectionChanged
. IDataErrorInfo
( INotifyDataErrorInfo
). WPF Silverlight UI, . UI.
, ?
, INotifyPropertyChanged
, INotifyCollectionChanged
, IDataErrorInfo
, INotifyDataErrorInfo
. , , . . , , .
:
, -. , - . , . INotifyPropertyChanged
INotifyCollectionChanged
. . , , ObservableCollection.
IDataErrorInfo
, INotifyDataErrorInfo
.
, .
ObservableCollection, INotifyCollectionChanged
. IDataErrorInfo
( INotifyDataErrorInfo
). WPF Silverlight UI, . UI.
, ?
, INotifyPropertyChanged
, INotifyCollectionChanged
, IDataErrorInfo
, INotifyDataErrorInfo
. , , . . , , .
:
, -. , - . , . INotifyPropertyChanged
INotifyCollectionChanged
. . , , ObservableCollection.
IDataErrorInfo
, INotifyDataErrorInfo
.
, .
ObservableCollection, INotifyCollectionChanged
. IDataErrorInfo
( INotifyDataErrorInfo
). WPF Silverlight UI, . UI.
, ?
, INotifyPropertyChanged
, INotifyCollectionChanged
, IDataErrorInfo
, INotifyDataErrorInfo
. , , . . , , .
:
, -. , - . , . INotifyPropertyChanged
INotifyCollectionChanged
. . , , ObservableCollection.
IDataErrorInfo
, INotifyDataErrorInfo
.
, .
ObservableCollection, INotifyCollectionChanged
. IDataErrorInfo
( INotifyDataErrorInfo
). WPF Silverlight UI, . UI.
, ?
, INotifyPropertyChanged
, INotifyCollectionChanged
, IDataErrorInfo
, INotifyDataErrorInfo
. , , . . , , .
:
, -. , - . , . INotifyPropertyChanged
INotifyCollectionChanged
. . , , ObservableCollection.
IDataErrorInfo
, INotifyDataErrorInfo
.
, .