AngularJS is a great framework for building web applications. He has excellent documentation, with examples. In the training "trial" applications (like the
TodoMVC Project ), it shows itself very worthily among other other frameworks. There are great presentations and screencasts on it.
However, if a developer has never encountered Angular-like frameworks and used mostly jQuery libraries in his work, then it may be difficult for him to change his way of thinking. At least that was the case with me, and I would like to share some notes on this topic. Maybe someone will be useful.
Not a library
The first thing to understand about Angular: it is a completely different tool. jQuery is a library. AngularJS is a framework. When your code works with the library, it decides when to call this or that function. In the case of a framework, you implement event handlers, and the framework already decides when to call them.
This difference is easier to understand if you think about what happens during the performance. What does jQuery do in runtime? Almost nothing. JQuery code is called only in response to something that happened in your code — when a trigger of any function triggered by a DOM event worked.
')
Angular at the boot stage turns your DOM tree and code into an angular application. HTML page layouts with angular directives and filters in it are compiled into a template tree, the corresponding scope and controllers are attached to them in the right places, the internal loop of the application ensures proper data binding between the view and the model. This is a real working scheme in full compliance with the principles of MVC, which provides a very clean separation between the view, the controller and the model. If we talk about the general cycle of events, page rendering and data binding, then you can assume that it runs continuously all the time, while calling the code from your controllers only when it is required.
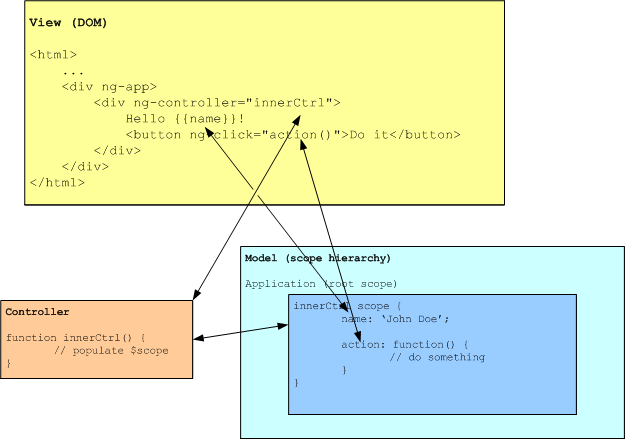
Every time the model is updated (whether through an asynchronous AJAX request or directly by changing data from the controller), Angular restarts the $ digest special procedure loop, which updates the data bindings and keeps the entire system up to date.
Declarative approach instead of imperative
Unlike some other libraries and frameworks, Angular does not regard HTML as a problem that needs to be solved (I won’t point the finger). Instead, he expands them in such a natural way that he unwittingly thinks why he himself hadn’t thought of it before. This is easier to show than to explain.
Suppose we want to show and hide some element based on the state of the checkbox. In jQuery, we would do it something like this:
<input id="toggleShowHide" type="checkbox"> <div id=”specialParagraph”> , </div>
$(function() { function toggle() { var isChecked = $('#toggleShowHide).is(':checked'); var specialParagraph = $('#specialParagraph'); if (isChecked) { specialParagraph.show(); } else { specialParagraph.hide(); } } $('#toggleShowHide).change(function() { toggle(); }); toggle(); });
Please note that the javascript code here perceives the DOM from the point of view of the imperative approach: take this element and its attribute, look at its value, do this and that.
Now let's look at the same in terms of Angular:
<input ng-model="showSpecial" type="checkbox"> <div ng-show=”showSpecial”> , </div>
And that's it! There is no code at all, just a pure declarative style of defining bindings and rules. Here is a demonstration of this code in jsFiddle:
jsfiddle.net/Y2M3rDirect manipulations with the DOM do not just cease to be mandatory; even more can be said, their use in the Angular approach is extremely discouraged. The DOM tree must be fully defined in the templates, the data in the models and scopes, the functionality in the controllers, any nonstandard transformations in its own filters and directives.
At first glance, this clear separation of tasks looks difficult to digest, but as the project grows, it will pay off handsomely: the code is easy to maintain, it is easy to divide it into modules, it is convenient to test and explore.
Two-way data binding
The process of binding values ​​from the DOM to the model data through the controller and its scope creates the most “binding” in the full sense of the word. Most likely, you already know this from the documentation and examples, but I just can not say it. This is one of the most powerful first impressions of using Angular.
<input type="text" ng-model="yourName" placeholder=" " /> <h1>Hello {{yourName}}!</h1>
Demonstration:
jsfiddle.net/6UnVA/1Dependency injection
I will say a little confidently, but Angular implements the most elegant way of tracking dependencies in the world.
Suppose you have some source of JSON data wrapped in a special $ resource service on the Angular side.
DataSource = $resource(url, default_params, method_details)
(refer to the documentation for details)
Any controller function that requires this data can include the DataSource in its list of parameters. This is all that is required of her. This is such a little street magic that never ceases to amaze me every day when working with AngularJS. Do you need to perform asynchronous HTTP requests from the controller? Add $ http to options. Need to write to console log? Add $ log as an argument to your function.
Inside, at this moment, the following happens: Angular analyzes the source code of the function, finds the list of arguments, and concludes which services are required by your code.
Data access
Besides the fact that Angular gives you complete freedom of choice in how to organize data in a model (you can use simple variables, objects and arrays in any combination), it also provides a convenient way to communicate with the REST API on the server. For example, we want to receive and save records about users. Here's how access to them can be organized:
var User = $resource('/user/:userId', {userId:'@id'}); var user = User.get({userId:123}, function() { user.abc = true; user.$save(); });
Angular has quite good presets for normal operations of sampling, deleting, receiving and changing data. And special parameters in the URL make it possible to customize access according to your needs.
Other important points that have been ignored here are, for example, the validation of forms, unit testing (
I personally think that unit testing is even more important than much of this article - the translation ) and the angular-ui library. Perhaps in further posts.