In Ext.NET, understanding the difference between different types of events and their handlers is very important. The choice depends on the specific scenario that you want to implement your application.
In this series of four articles, we will look at various events on the client and on the server, how they are triggered, processed, and which ones have advantages. This article will look at
Listeners - allowing you to “listen” to events on the client and process it in the same place on the client, avoiding a request to the server.
All components in Ext.NET, such as
Panel, Window, Button , have the following four types of event handlers:
Listeners
| Listen to events on the client and process them on the client side as well.
|
DirectEvents
| Listening to events on the client, but processing them on the server side, the call is made via an AJAX request.
|
Directmethods
| They allow you to call functions on the server, for example, written in C # or VB, the call is made via an AJAX request.
|
MessageBus
| MessageBus allows you to send various events between components that may not know about each other. Message processing occurs through subscription to certain messages and sending event messages via MessageBus. Each component on the client has a special MessageBusListeners handler, as well as MessageBusDirectDirectEvents for transferring processing to the server side.
|
Listeners
Listeners are event handlers on the client. They execute your code after the appearance of some event, for example, pressing a button (
Button ).
Using
Intellisense in Visual Studio allows you to find out what specific events each component has. Each component inherits events from its ancestor and usually adds its own.
All classes in Ext.NET inherit events from the
Observable base class. All events are in the
Listeners .
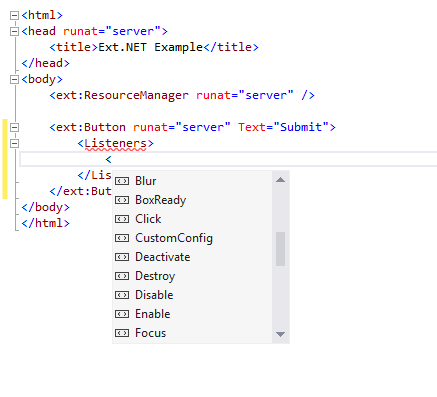
All available events can be viewed using Intellisense.
If a component raises some event on the client, then in order for your code on the client to handle this event, you must subscribe to this event by adding a JavaScript function.
')
Configure Listeners
Customizing
Listeners can be done both in markup and in Code-Behind. For example, to process a “click” event for a button (
Button ), you must use the Click
Handler field, in this field you must enter your JavaScript code that will be executed by the browser when you click on this button.
// C# Button1.Listeners.Click.Handler = "alert('Hello World');"; // ASPX <ext:Button runat="server" Text="Submit"> <Listeners> <Click Handler="alert('Hello World');" /> </Listeners> </ext:Button> // Razor (ASP.NET MVC) @(Html.X().Button() .Listeners(ev => ev.Click.Handler = "alert('Hello World');" ) )

Pressing the button brings up the 'Hello World' window.
Another important property of the Listener is
Fn .
The
Fn property must contain the name of a JavaScript function or an anonymous function (inline function):
// JavaScript , // - var doSomething = function () { alert('Hello World'); }; // #1 // Fn JavaScript Fn="doSomething"; // #2 // JavaScript Fn="function () { alert('Hello World'); }" // #2b // , , Fn="function (item, e) { alert('Hello World'); }"
The JavaScript code that is declared in the
Handler must be the body of the function and, when displayed on the Ext.NET page, will automatically wrap it with a function template that corresponds to this event.
For example:
<ext:Button runat="server" Text="Submit"> <Listeners> <Click Handler="alert('Hello World');" /> </Listeners> </ext:Button>
Ext.NET will automatically wrap this code and reflect on the page as follows:
Ext.create("Ext.button.Button", { text: "Submit", listeners: { click: function (item, e) { alert('Hello World'); } } });
Each event has its own list of arguments, which you can find either on the
Sencha Ext JS API documentation , or by examining the source code of the Listener you are interested in (if you have the Ext.NET source code, you can view all the Listeners classes in the
<Ext .Net> \ Events \ Listeners ).
Also on
the Ext.NET page with examples there is a special example -
Listeners Arguments Reflector , in which you can view all the function signatures of any event for any component.

Description of the Click event signature of the Button component
For example, the
Click event of the
Button component has two arguments:
item and
e : item is a reference to the
Button entity itself,
e is an object that stores information about the event.
The
Reconfigure event handler of the
GridPanel component
is called with the following arguments:
item, store, and
columns .

Arguments for the GridPanel Reconfigure Event
If you use the
Fn property, you must add the arguments to the function yourself. Arguments can have arbitrary names, but the order of the arguments is very important. For example, the following two functions have different argument names, but work exactly the same.
var doSomething = function (item, e) { alert(item.id);
Each Listener has the following properties:
Autopostback
| Specifies whether to automatically send the status of the component to the server. Identical to a regular field in ASP.NET Control.
| false
|
BroadcastOnBus
| Indicates whether to send an event to a MessageBus (to be explained in detail in the MessageBus article)
| false
|
Buffer
| Specifies the number of milliseconds to which the event will be postponed, if during this time the event appears again, the original handler will be replaced with a new one. For example, for some event (let it be 'click'), this field is equal to 1000, and if 10 such events occur in 1000 milliseconds ('click'), the event handler will be called only once, for the most recent event.
| 0
|
Delay
| Specifies the number of milliseconds to which the event handler call will be deferred.
| 0
|
Delegate
| Filters the HTML element for which this event can be triggered. For example, if you assign the following value to this property: Delegate = <a>, then for the Click event, the handler will be called only if you click on the HTML element with the <a> link inside the component's HTML markup.
| ""
|
PreventDefault
| If the value is True, the default browser handler will be prevented. (For example, following the link)
| false
|
Scope
| Specifies the context of the this variable for the event handler function. If no value is specified, then by default this points to the object that triggered the event.
| this
|
Single
| If the value is True, the event handler will be called only once and will automatically be deleted after its first and last call.
| false
|
StopEvent
| If the value is True, then it stops the event, which stops the further sending of the event and prevents the browser from processing it. Should be used only for events that are processed by the browser. (click, mousemove, keypress, etc.)
| false
|
StopPropagation
| If the value is True, then stops further sending of the event.
| false
|
Using server methods .On and .AddListener
These methods are used to add a Listener for some event during
DirectEvent , after the component has already been displayed in the browser (server event handling will be discussed in further articles).
These methods allow you to add client-side handlers to components that are already displayed on the page, and are usually used during the execution of
DirectEvent . Unlike using
<Listeners> , the
On method allows you to add several handlers for one event (one handler for each
On method call).
Button1.On("click", new JFunction("alert('The button is clicked');", "button", "e"));
Arguments can be omitted if you do not use them in the handler.
However, it should be remembered that if the handler returns
false , then other handlers will not be called, just as
DirectEvent event
handlers will not be called either.
Using client-side .on and .addListener methods
If you want to add an event handler using JavaScript, you can call the
on function for some entity entity:
Button1.on( "click", function (button, e) { alert('The button is clicked'); } );
Additional information and usage examples can be found on
this help page .
You can also trigger an event manually using the
fireEvent JavaScript function. You must pass the name of the event as the first argument and then list the arguments. The following example shows a call to the
fireEvent function:
It should be remembered that server event handlers (
DirectEvents ) can also be called using the
fireEvent function (Server handlers (
DirectEvent ) are called after the client (
Listeners ), but this sequence can be changed using the
Delay property).
Calling the server handler (
DirectEvent ) can be canceled if any handler (without the
Delay property set) returns
false . More information can be found on the help page of the
fireEvent method.
Conclusion
In this part of the article, we looked at
Listeners , which are event handlers on the client side. They provide an opportunity to quickly process events on the client side and avoid unnecessary interaction with the server.
The following article will describe the server event handlers -
DirectEvents .