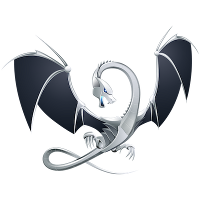
LLVM is a set of building blocks for building compilers, and clang is a
new C ++ compiler based on it. Compared to gcc, it provides a high compilation speed with comparable code quality, more human-readable sources; They do not carry decades of cruel C-only development as in gcc.
In addition, Clang and llvm distributed under the BSD license, in contrast to the GPLv3 from GCC. BSD allows you to not open source when distributing executable files of the compiler.
With LLVM + CLang it will be interesting to play anyone who has ever wanted to write his own compiler, or believes that the compiler assembled with his own hands gives a warmer binary code.
')
Most of the developers llvm / clang use it under Linux / MacOS - and there its build / installation does not cause any problems, the path is thoroughly trodden. But in the case of windows, the situation is somewhat complicated - about bypassing several rakes during the build, about what binds clang and gcc, and what bugs will have to be fixed in the release - under the cat.
Assembly
There are 2 main ways to build llvm + clang under windows:
CMake allows you to generate a solution for Visual Studio. clang + llvm still depends on the gcc components (for example, the standard library, but work on eliminating the dependency), because even collecting clang in Visual Studio this dependency will remain, and you will get the wildest hodgepodge of clang, VS and gcc pieces, where everything components will eventually change versions. In addition, at the moment Visual Studio 2012 crashes when building clang 3.2 with optimization - Microsoft is working on a fix (everything is OK in 2010). In light of this, I decided not to use Visual Studio, especially this should not affect the final result (clang compiled clang).
Mingw32 + MSYS .
Mingw32 - contains gcc and a thin wrapper that implements a linux-like environment for programs, streaming windows <> linux style. Specific functions (like fork) are not fully implemented, because compatibility is worse than that of cygwin, but it works faster and faster. Partial description of the build process -
on the website llvm .
MSYS - gives us the “linux” shell, in which you can run standard build scripts. This is the path we will follow.
Run the MSYS console, download the llvm, clang, compiler-rt sources (low-level system code for the build), unpack llvm to the root of your source folder, clang - to llvm / tools, compiler-rt - to llvm / projects. Checking the version of gcc - for llvm 3.2, gcc will fit no newer than 4.6.2 (from 4.7.2 I couldn’t collect myself from me, but it’s not too old either).
After that, create a directory for binaries, for example llvm / bin, go there, and execute the command:
../configure --disable-docs --enable-optimized --enable-targets=x86 --prefix=/newclang
After the configuration is completed, we write make, and the project will start building. When make is over, we can do a make install, and our compiled binaries will be written to the newclang directory in the root of the MSYS file system.
Next - self-assembly . It is needed to make sure that the compiler is working reliably, we did not miss any problems, and to eliminate one variable: the “version of the xenocompiler”. We switch the system to use the newly assembled clang for the further assembly of itself:
export CXX=/newclang/bin/clang++.exe export CC=/newclang/bin/clang.exe
Create a new directory for compilation, run it there again ../configure ... and then make. But the clang will not assemble itself so simply, otherwise what would be the point of this article?
Fix problems
Work speedThe first thing that catches your eye is that the assembled clang slows down monstrously, and that is with the release build! Even the simplest "clang ++ --version" can run for a few seconds. The solution is simple: you need to collect it with a static link, then everything works quickly, but at the cost of an increased size of binaries (tens of megabytes).
Export LDFLAGS=-static
Error "Only alloca-wide stores can be split and recomposed"This is a
bug in llvm , which is already fixed in the repository and will be included in 3.3. But yes, the release of clang 3.2, because of this, cannot assemble itself.
You need to roll into the file \ lib \ Transforms \ Scalar \ SROA.cpp fix from revision svn 170270:
=================================================================== --- SROA.cpp (revision 170269) +++ SROA.cpp (revision 170270) @@ -2607,7 +2607,7 @@ TD.getTypeStoreSizeInBits(V->getType()) && "Non-byte-multiple bit width"); assert(V->getType()->getIntegerBitWidth() == - TD.getTypeSizeInBits(OldAI.getAllocatedType()) && + TD.getTypeAllocSizeInBits(OldAI.getAllocatedType()) && "Only alloca-wide stores can be split and recomposed"); IntegerType *NarrowTy = Type::getIntNTy(SI.getContext(), Size * 8); V = extractInteger(TD, IRB, V, NarrowTy, BeginOffset,
Multiprocessor build freezesIf you use make -j 8 to speed up the build, then yes, mingw32 can hang tight in the middle of the build, at least on an SSD (no delays in accessing the disk - more chance to meet some race condition). I did not find a solution.
clang cannot find standard librariesAs we remember, it uses standard libraries from gcc. In order for him to find them - they must be the correct version, and lie in the expected place clang.
Do not fall off the chair, the paths for searching for files are “hardcoded” in the \ tools \ clang \ lib \ Frontend \ InitHeaderSearch.cpp file. If your MinGW is not in the default folder, clang will not find it. Then, clang checks only some versions of gcc to look for directories with header files, make sure you have the right version of gcc.
You can install packages of the required version in MSYS as follows:
mingw-get install "gcc=4.6.*" mingw-get install "g++=4.6.*"
Now the clang will be able to assemble itself, and you will get a fresh and fast, warm and tube, self-compiled compiler.