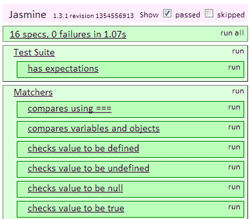
Client-side programming has long become the norm, and the amount of JavaScript code and its complexity is constantly growing. Often, testing is applied only on the server side, but you should not forget about testing client code. Jasmine can be successfully used for testing JavaScript both on the client side and for Node.js.
Jasmine is a BDD framework (Behavior-Driven Development - Behavioral Based Development) for testing JavaScript code that borrowed many features from RSpec.
')
For convenience, we will consider testing in the browser, and for conciseness, examples are given using CoffeeScript (
examples in JavaScript ).
You can install Jasmine by downloading the
Jasmine standalone package. Required files:
- lib / jasmine - * / jasmine.js - the framework itself
- lib / jasmine - * / jasmine-html.js - formatting results as HTML
- lib / jasmine - * / jasmine.css - the appearance of the test result
- SpecRunner.html - the file that should be opened in the browser to run tests
The main keywords when working with Jasmine are:
- describe - test set definition, sets can be nested
- it - test definition inside any test suite
- expect - defines the expectations that are checked in the test
The keywords
describe and
it are normal function calls that are passed two parameters. The first is the name of the group or test, the second is the function containing the code. A simple example for clarity:
describe " ", -> it " ", -> expect(1 + 2).toBe(3)
To disable the execution of a test suite or a specific test, you must use the keywords
xdescribe and
xit, respectively.
describe "", -> xdescribe " ", -> it " , ", -> expect(true).toBe(true) xit " ", -> expect(true).toBe(true)
Jasmine has a standard set of expectations for validating results:
it " ===", -> expect(1 + 2).toBe(3) it " ( )", -> a = {x: 8, y: 9} b = {x: 8, y: 9} expect(a).toEqual(b) expect(a).not.toBe(b)
In order to avoid repetition when creating / deleting objects and loading fixtures necessary for executing tests, the
beforeEach /
afterEach functions are used. They run before / after each test in the kit.
describe "/", -> val = 0
Jasmine supports testing asynchronous calls using the runs and waitsFor functions.
- runs - accepts an asynchronous function for execution.
- waitsFor - takes the first parameter of the function that should return true if the asynchronous call made in the runs was executed, the second parameter is the error message, the third is the wait time in milliseconds.
describe "", -> a = 0 async = -> setTimeout((-> a = 5), 1000) it " ", -> runs(-> async()) waitsFor((-> a == 5), " ", 3000)
Consideration of working with “spies” spies (mock object) and working with time (mock clock) will be left for the next article. If you have questions or comments, I will be glad to answer them.
The second part:
"Jasmine - additional features"References:
github.com/pivotal/jasmine - Jasmine page on GitHub
www.inexfinance.com/en/blog/2013/1/25/jasmine_for_clientside_testing - English version of the article
github.com/inex-finance/blog-examples/tree/master/jasmine - code examples from this article