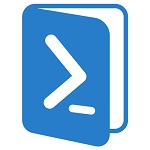
Introduction
Sometimes, using PowerShell, Windows Server 2008 can be managed much faster than with the usual GUI tools. In this article you will find the 9 most common tasks that can be implemented using PowerShell. (10 task was excluded from the translation due to the fact that the script shown in the article was incomplete -
Note of the translator ).
There will be two posts on this topic. There is a lot of material, the material is good, but unfortunately, placement in one post will make it unreadable.
So, what tasks will be considered:
- Changing the local administrator password using PowerShell
- Reboot or shutdown server
- Restart service
- Process stop
- Create a disk usage report
- We get the last 10 errors in the event log
- We reset folder access control
- Get server's uptime
- Get information about Service Pack
The first five tasks we will consider in this post, the remaining 4 subsequent. We invite interested persons under cat.
1. Change the local administrator password
Suppose you are logged in as a domain administrator on a computer running Windows 7 that is part of your domain. Now, suppose you want to change the local administrator password on a remote
CHI-WIN7-22 server in Chicago. After using the password for a certain period of time, it is desirable to change it; the procedure must be repeated periodically.
The first thing the administrator does to change the administrator password is to create an ADSI object for the local administrator on this computer. This is done in PowerShell as follows:
')
[ADSI]$Admin=”WinNT://CHI-WIN7-22/Administrator”
The administrator account on the
CHI-WIN7-22 server will be extracted and assigned to it by ADSI to the $ Admin object. The WinNT name in this string is case sensitive, take this into account. If you want to connect to another computer, simply replace CHI-WIN7-22 with the name of the computer to which you would like to connect.
However, first you need to know how long the password is used to determine whether it is time to change it or not. Information from
$ admin can be obtained as follows:
$Admin.PasswordAge
The time elapsed since the last password change is displayed. The result is shown in seconds, translate it into days (let's divide by 86400 (the number of seconds in a day)):
$Admin.PasswordAge.Value/86400
Notice that the
Value property was used. This was done because
PasswordAge is stored as a collection, and therefore we need to first assign the value of this collection in order to return it to the number to which the division operation can be applied.
As a result, you can change the password by calling the
SetPassword method and then using the new password as an argument.
$Admin.SetPassword(“S3cre+WOrd”)
Attention: By pressing Enter, do not wait for a confirmation letter. That will not be! Changes will be applied immediately. What I demonstrated here is a method, not a cmdlet. Among other things, this means that, unlike cmdlets,
SetPassword does not support
-whatif or -
confirm .
It's all. Let me demonstrate all of these PowerShell images.

2. Reboot or stop the server
Moving on. Our task is to restart or stop the server using PowerShell. As in the first case, suppose that you are logged in as a domain administrator on a Windows 7 machine that is part of your domain.
We will use two WMI cmdlets -
Restart-Computer and
Stop-Computer . And although we will not show them here, it is worth mentioning that these cmdlets accept alternate credentials. Alternative credentials allow you to clarify a user account (other than the one under which you are logged in), so that you can perform actions for which this (alternative) account has rights.
Also among the nice
things about these cmdlets - you can use
-whatif and -
confirm . This means that if you want to restart or shut down the server, you must first make sure that you are doing this on the designated computer. This is convenient when you perform similar operations with multiple computers.
To restart the computer, the syntax is:
Restart-Computer -ComputerName <string[ ]>
where
-ComputerName <string []> is an array of strings, which can consist of the name of one or several computers.
Stop-Computer uses almost the same syntax. For example, if you want to restart two computers, CHI-DC02 and CHI-FP01, use the following command:
Restart-Computer “CHI-DC02”, “CHI-FP01”
The following is actually a screenshot of PowerShell, in which we used the
–whatif argument. Use it if you just want to see what happens if you run the command.

Everything is quite simple. Let's complicate the task now. Suppose you have a list of computers in the
servers.txt file. Use the
Get-Content cmdlet to extract their names from the file.

So, you have a number of computers that you would like to periodically reboot, and you store their names in a text file. Every time you need to reload them, you just use the
Get-Content cmdlet. Below is an example of how
Get-Content and
Restart-Computer work .

First we get the contents of the file using
Get-Content . To start pinging these computers. In this expression, we will run
test-connection , which is actually equivalent to
ping on each computer.
-quiet returns true or false, and
-count 2 means that each computer will be pinged only twice. Those computers that will be successfully pinged will be further put into operation.
Then we use
foreach . The purpose of this is this: for each name that passes the ping test, a message is displayed in green text indicating that the computer is restarted. “$ _” Means the current object in the pipeline. Then we use the
Restart-Computer cmdlet to reboot the computers that are pinging. We also use the
–force parameter to reset everyone logged in to this computer.
The
–whatif parameter
is used to see what happens without actually restarting computers.
3. Restart service
Restart-Service , as the name implies, is a cmdlet that restarts the service. Although it does not have the ability to connect to a remote server,
PowerShell Remoting can be activated, so you can perform it locally on a remote computer. This is useful when you want to restart a service on a group of computers.
To restart the service locally, simply write
Restart-Service “service ”, where “service” is the name of the service you want to restart. On the other hand, if you want to restart services on one or more remote machines, use the
Invoke-Command cmdlet and
PowerShell Remoting .
The screenshot below shows two examples of the work of the
Restart-Service cmdlets to restart the
wuauserv service (Windows Update). In the first example,
Restart-Service runs locally. In the second, it runs on the remote database server CHI-DB01 using the
Invoke-Command cmdlet.

By default,
Restart-Service does not display any objects unless you use the
-passthru parameter. Additional information (Status, Name, etc.) - the result of its use. If the service is running on multiple computers and you want to restart them also, list them separated by commas.
The same can be done using WMI. Create a WMI object:
gwmi is short for
Get-WmiObject .
Let's look at the methods of the object. We introduce
Get-Member (abbreviated
gm ).

As you can see, there is no method for restarting the service. This means that you first have to stop the service through the
StopService method and start it again using the
StartService .
Here's how to stop a service using the object's
StopService method. A parenthesis indicates the presence of a method. If you get
ReturnValue equal to 0, then the service was successfully stopped. Otherwise, refer to the MSDN documentation for the Win32 service class.

Start the service - the
StartService method.

Check: run the
get-service command on this computer.
Get-service allows you to get information about the service on a remote computer. Sample request for remote computer CHI-DB01.

4. Stop the process
Another common task is to stop the process. Use the
Stop-Process cmdlet for this. It can be executed both locally and on a remote machine (see clause 3).
There are two ways to stop a process using the
Stop-Process cmdlet.
The first is simple. Start the
Stop-Process and pass it the name or the corresponding process ID. Please note that we stop “Calc” (Windows Calculator). In this example, the process is running locally.

The second method involves using the
Get-Process cmdlet to get one or more processes or transfer them to the
Stop-Process . For example, the process taken Notepad.
Kill is an abbreviation for
Stop-Process . Notepad running locally.

Moving on. Let us turn to the processes running on the remote machine. To begin with, let's run, for example, notepad on the remote computer chi-fp01.

Then, check if the process is running. For these purposes we use
ps , which is an abbreviation for
Get-Process .

5. Create a report on the use of disks
Administrators must track the free space left on the servers. This can be done by using WMI and the Win32_LogicalDisk class, which give us information such as device ID, disk size, free space and other information.
Through WMI we can access local and remote computers. We can also fulfill these requests both on one and several machines. We can also: export data to a .csv or database, create a text or HTML report, or simply display the results on the screen.
An example of a command running on a local computer.
Get-WmiObject win32_logicaldisk -filter “drivetype=3” | Out-File c:\Reports\Disks.txt
We use the
GetWmiObject cmdlet to return information from the Win32_LogicalDisk class. Then we use
-filter to return only information for which drivetype = 3 is true, which means fixed logical drives, such as C :. This means that information regarding USB and network drives will not be included. The resulting information will be recorded in the file
Disks.txt .
Example in PS.

Although everything seems to be fine, it is better to make a couple of improvements. For example, add a display of free space in gigabytes, not bytes. This is what we will do.
To do this, create a function
Get-DiskUtil . Although in the previous example we did everything interactively, in this case let's write the function to a file, upload it to your profile to other scripts that can be used later.
And here is the function itself:

Let's take it apart.
The function takes the computer name as a parameter and sets it as the default local computer name.

Then we use a fragment of the
Process script, where the property “computer name” is passed to the function. “$ _” Indicates that the computer name is set as a variable. Otherwise, the computer name will be interpreted as a parameter.

The following is the expression
GetWmiObject .

The output of this expression is passed to the
Select-Object cmdlet (abbreviated to
Select ). We use hash tables to create a custom property called
Computername . In fact, the
SystemName of the current object ($ _) will be renamed to
Computername .
DeviceID remains unchanged.

Let's make a couple more hash tables. The first one takes the
Size property and divides it by 1GB, the output will be with two decimal places and renames the property to
SizeGB . The second produces the same with the
Freespace property.

Then we create the
UsedGB property, which is not in WMI. The difference between
Size and
FreeSpace properties is calculated and divided by 1GB.

In the end, we create another
PerFree property - freely in percent. It terminates the function.

Below is the function for the computer CHI-FP01, displayed in the table (
Format-Table (or
ft )) with auto formatting (parameter
–auto ).
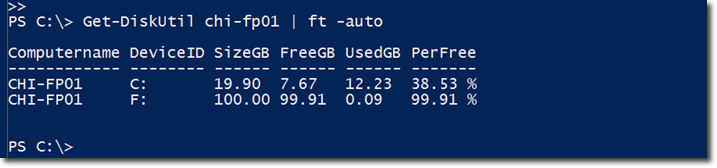
Everything is good, but from this function we can get more. Therefore, suppose you need to receive a weekly report on disk usage on all servers in your company. Let's see how this can be achieved.
To begin with, we will save the results of our expression into the
$ data variable so that we do not type this command each time. Then, we will transfer the results to the
where object, ping the server (twice) and pass the computer name to the
Get-DiskUtil function that we created.

The data will be stored in the
$ data variable. You can pull information from
$ data and sort by
computername by applying
–auto . Information can also be sent to print (
Out-Printer ) or to a file (
Out-File ).

Convert to csv:

Then you can import this csv file to get a snapshot of the disk usage status at the time of the command launch:

Example:

And finally: I will show you how to create an HTML report that can be accessed from anywhere.
Take
$ data and pass it to
Sort Computername . The result is passed to the cmdlet
ConvertTo-HTML . You can assign a CSS header and path. CSS is required because
ConverToHTML does not have formatting capabilities. So if you want the report to look decent, you'll need a CSS file. At the last stage, write the result to a file.

Now the file is ready, it can be viewed using the
start command.

Sample HTML report.

Remember that information needs to keep information up to date.
Upd:
The post is a translation of an article from the portal
petri.co.ilTop 10 Server 2008 Tasks done with PowerShell - Part 1The second part of the translation is
here .
PS Could not fail to note that the same task of obtaining information about free disk space can be solved using our free program NetWrix Disk Space Monitor.
You can see how the program works on
youtube , download it
here , and activate the free license for an unlimited period of validity (and without any restrictions at all) using the following data:
License name: NetWrix Disk Space Monitor Freeware License
License count: 1,000,000
License code: EhEQEhoaEhEQEhYTEhYaExQa