Foreword
Greetings to you habroody. The dream of my life has recently come true and I bought myself a Mac (13 'unibody). Late 2008, but for our village will come down. Since then, I began to slowly delve into the development of applications for iOS (in particular for the iPhone).
Now more to the point. I first decided to write a simple application that allows you to create and view notes. Here is how it looked in the end:
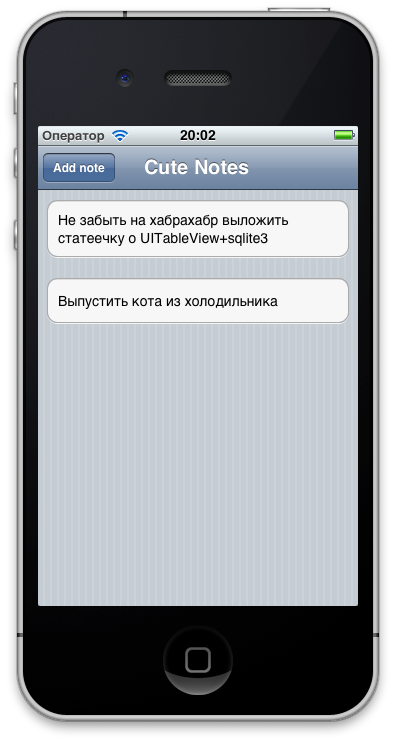


')
But even for writing such a simple application I had to beat my head against the walls, because There are few articles on the Internet for tractor drivers (whom I am essentially). So I decided to fill this gap. If there are admiring reviews, I will continue this topic. And I immediately warn you that I know Russian far from perfect. Well, ok, let's go!
Setting up the environment and the project
The first thing you need to do is buy yourself a poppy. Of course there is another option to set yourself a hackintosh and you should not discard it, this is a worthy alternative if there are no problems with iron compatibility.
Next, download Xcode (the IDE in which we will create the application) from here:
developer.apple.com/xcode (together with the IDE we get a compiler, debugger, profiler, and iPhone simulator). In order to download you need to register, I think it will not be difficult. Install and run.
When you start Xcode, we will offer us to create a new project, select the “Create new Xcode project”:

Then we are offered several frameworks for a new application, we will go against many recommendations of the Internet and create a Single View Application (this is an application in which there is only one type that we will see after launching the application):

Next, we indicate the name of our application (ActiveTable) and other nonsense (note that the Use Storyboards checkbox is checked; what is it you can see here
habrahabr.ru/post/131128 ):

Next, select the folder to save the project (a subfolder will be created for the project), optionally we can have full Git support (which pleases) and see the settings of our project (they are shown if you select the item with the project name in the panel on the left).
Overview of the project created by the master
The most interesting is here:

In the “Main Storyboard” dropbox, we can select the storyboard to be loaded when the application starts (for us, a storyboard with the name “MainStoryboard” was automatically created and assigned), and under the darpbox a list of supported orientations of our device (in the simulator “iOS Simulator” you can turn selecting “Hardware — Rotate Left” or “Hardware — Rotate Right”). On the left we see this:

Here we have the “Run” button to launch the application in “iOS Simulator”, and below the project tree:
- AppDelegate.h / AppDelegate.m - class for handling events of our application;
- MainStoryboard - file with our views and transitions;
- ViewController.h / ViewController.m is a class for managing our default view, which was created for us by the project creation wizard.
The remaining files and folders are not particularly interesting in the context of this article. But I will tell you more about ViewController. If you select MainStoryboard-ViewController in the project tree (left panel), select the “Show the Identity inspector” tab in the panel on the right, you will see that the ViewController class is selected as the controlling controller in the “Custom Class-Class” class, which is described in the files ViewController.h / ViewController.m:

Creating a database and connecting sqlite to the project
Well, it's time to create a sqlite database. To do this, open our favorite terminal (oh yes, it drives me crazy. You know, mac os as ubuntu, just not at all, but much better!), Go to the project folder and create a base there (immediately create a table and fill her data):
$ cd ~/Documents/Projects/ActiveTable/ActiveTable $ sqlite3 Base.db sqlite> create table animals (id integer primary key, name text); sqlite> insert into animals(name) values('Cat'); sqlite> insert into animals(name) values('Dog'); sqlite> insert into animals(name) values('Horse'); sqlite> .quit
The base is ready. Now you need to add a file with the base to the project. To do this, perform:
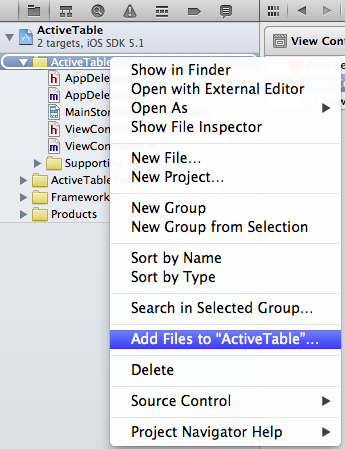
In the dialog, select the Base.db file. Now connect the libsqlite3.0.dylib. To do this, go to the project settings (the root of the project tree on the left) -TARGETS-ActiveTable-Build Phases-Link Binary With Libraries, click on the plus sign and find libsqlite3.0.dylib.

Now attention, one more thing. Working with sqlite3 will be a complete nightmare if you do not write a sensible wrapper. But fortunately there is a ready and very cool FMDB library (https://github.com/ccgus/fmdb). There is also a good tutorial on the use of this miracle
www.icodeblog.com/2011/11/04/simple-sqlite-database-interaction-using-fmdb . So we need to download this library. If Git is not worth it, you need to download it from here
code.google.com/p/git-osx-installer . We clone FMDB (being in the folder with the project):
$ git clone https://github.com/ccgus/fmdb
Next, add the fmdb folder to the project as well as Base.db. After that, you need to find and delete from the project tree fmdb / src / main.m (this is the file for building the FMDB library). Well, finally we can podkododit! :)
Refinement of the controller for the tableview
First we need to create a tableview. To do this, go to the MainStoryboard, we find in the right panel below in the TableView

and drag it to our ViewController:

TableView we have. Now we need to delegate event handling and data retrieval to one of the controllers. To do this, we select our TableView, in the panel on the right, go to the “Show the Connections inspector” tab and drag the marker next to the “delegate” to the icon of our controller (the one that is ViewController):

Also we do for “dataSource”. The connection has been created; now the TableView will know which controller to contact to obtain the data it needs.
In the ViewController class (ViewController.h) we declare a field for storing table data:
@interface ViewController : UIViewController { NSMutableArray *_items; }
In the implementation of ViewController (ViewController.m), there are already two methods for handling input and output from view, add data loading and freeing to them:
- (void)viewDidLoad { [super viewDidLoad]; _items = [[NSMutableArray alloc] init]; [self loadItems]; } - (void)viewDidUnload { [super viewDidUnload]; [_items release]; }
As can be seen from the example above, to create records, we create the loadItems method:
- (void)loadItems { // NSString *path = [[[NSBundle mainBundle] resourcePath] stringByAppendingPathComponent:@"Base.db"]; // FMDatabase *database; database = [FMDatabase databaseWithPath:path]; database.traceExecution = true; // [database open]; // animals FMResultSet *results = [database executeQuery:@"select * from animals"]; while([results next]) { NSString *name = [results stringForColumn:@"name"]; //atIndex - - , [_items insertObject:name atIndex:[_items count]]; } // [database close]; }
The link to the tutorial on using FMDB is given above, I think everything is transparent here, the main thing is to remember to include the FMDB header (#import "fmdb / src / FMDatabase.h").
Here we have the data, which means that we slowly came to a denouement! Then, there are three main methods that are necessary (except numberOfSectionsInTableView, I cite it for completeness) for the data to appear in the TableView. The “numberOfSectionsInTableView” method returns the number of sections of the table. The table with sections looks something like this:

Since we have a table without sections, we simply return the unit:
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView { return 1; }
But the method “numberOfRowsInSection” just returns the number of rows in the section. For a single table, this is just the total number of rows:
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section { return [_items count]; }
Well and actually method which returns a cell for drawing:
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath { // , , // "Cell" NSString *cellIdentifier = @"Cell"; UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:cellIdentifier]; if (cell == nil) { // , cell = [[[UITableViewCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:cellIdentifier] autorelease]; } // indexPath.row NSString *item = [_items objectAtIndex:indexPath.row]; // label cell.textLabel.text = item; return cell; }
Run and enjoy:

Conclusion
IOS development is a very interesting lesson. Built-in components are very powerful and quite customizable. The IDE and its associated tools are impressive. Well, really, you can display the table without any problems.
Finished project (File-Download):
docs.google.com/open?id=0B-5edA19iu-TSXZ6QUpoVWtUN28 .
PS: do not judge strictly, this is my first attempt at writing! But all comments are welcome.