This article is a translation of this article.
I hope my translation will not be too clumsy, and the material is interesting.Today, vector displays are rather old wonders than a means of displaying information; nevertheless, their use in arcade machines and radar systems gives them a certain charm.
Unlike ordinary raster displays, where the beam passes from left to right and top to bottom each time to draw each line, in vector displays the beam moves along the lines defining the image.
Most two-channel oscilloscopes have an XY mode, in which the signal used to scan the image in time is replaced by the input signal, thereby allowing control of the position of the beam in two planes. Using
PWM with a low pass filter can be effective for controlling the brightness of the LED, but in order to draw complex shapes a faster way is needed. The easiest way is to use a
DAC (digital-to-analog converter) according to the R-2R scheme.
')

If you are interested in learning more about creating a vector display or how to draw an oscilloscope on the screen, welcome under cat.
Parallel DAC under the scheme R-2R

Eight-bit DAC is simple to create, it requires only 16 resistors per channel. It is advisable to use resistors with a one percent tolerance, but 5% will also fit. You need 7 resistors with resistance R and 9 with resistance 2 * R.
In this case, 0.5 and 1 kΩ resistors are used.
Schematic diagram of the converter:

Since we control two channels of the oscilloscope, we need two DACs.
If you do not want to solder so many parts, then you can use resistor assemblies in SIP packages or use something like MC1408 / 1508 (multiplying DAC) in a 16 pin DIP package.
This project uses a card based on the ATMEGA32U4 microcontroller, you can use almost any other microcontroller, the main thing is that it has two 8-pin ports free so that you can change the state of the pins simultaneously.
I use ports PORTD and PORTB.
Oscilloscope setup
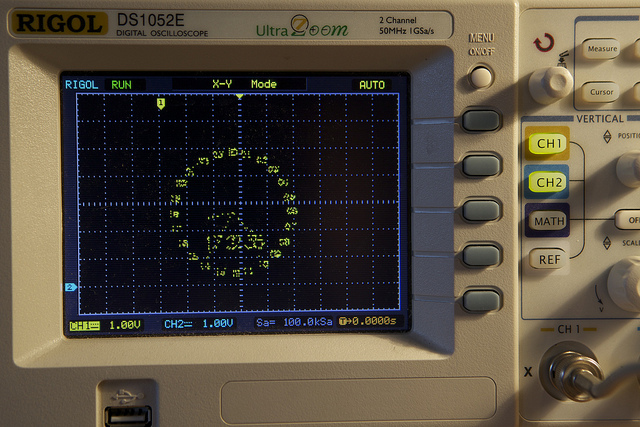
After splicing the circuit, connect it to the oscilloscope and put it in XY mode.
In inexpensive Rigol, for this you need to enter the Horizontal Menu - Time Base menu and select the XY mode.
Unfortunately, inexpensive digital oscilloscopes with LCD screens are inferior to analog ones in the drawing context, although some of them have a Digital Phosphor mode that simulates screen inertia.
Horizontal and vertical lines

By connecting the DACs directly to the ports of the microcontroller, the analog voltage can be changed by writing values to the port. For example, to generate a 2 volt signal, the value should be 256 * 2.0V / 5.0V == 102.
DDRB = 0xFF; PORTB = 102;
In principle, we are not interested in the specific voltage value, it is important that the output voltage varies linearly from 0 volts at 0x00 to 5 volts at 0xFF.
The oscilloscope should be adjusted to the range of 0-5 Volts and aligned on each of the axes.
The first thing to try is to draw horizontal and vertical lines; this can be done with the help of cycles, leaving one variable unchanged. For example, when drawing a horizontal line, the value of Y is constant and vice versa.
void line_vert( uint8_t x0, uint8_t y0, uint8_t w ) { PORTB = x0; PORTD = y0; for (uint8_t i = 0 ; i < w ; i++) PORTD++; } void line_horiz( uint8_t x0, uint8_t y0, uint8_t h ) { PORTB = x0; PORTD = y0; for (uint8_t i = 0 ; i < h ; i++) PORTB++; }
The code for drawing a square, like the one in the previous figure.
line_vert(64, 64, 128); line_vert(196, 64, 128); line_horiz(64, 64, 128); line_horiz(64, 196, 128);
Sloping lines
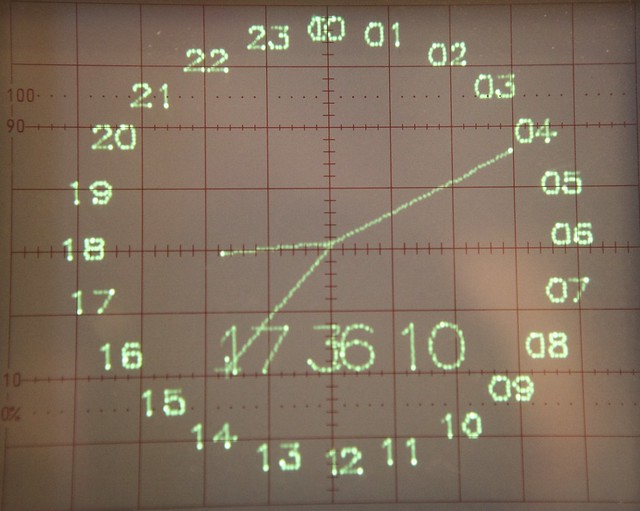
This image shows a common artifact of vector displays: bright dots at the beginning and end of a line. This effect arises because the intensity of the glow point depends on the time spent by the beam at this point, and since the program needs some time to load a new line, the beam is delayed a little longer in one place. To solve this problem, you can use 16-bit arithmetic or add NOPs, so that switching always takes place at the same time.
Here it is necessary to say about the burnout of the screen.
If the values do not change for a long time, then the phosphorus in this place can fade and become a different color. Screens can also be damaged if you constantly display the same image. During the firmware of the microcontroller, the ports usually switch to the Z-mode, which means that zeros are fed to the oscilloscope input, which results in a bright spot in the corner of the screen.
During flashing it is best to reduce the brightness.Drawing slanted lines is much more difficult than drawing vertical or horizontal lines.
An ideal option is to use analog circuits to smoothly change the signal, as, for example, this is done in the
SC200 .
In other matters, instead, we can use the Brezenham algorithm, to split the line into points. The use of this algorithm causes the appearance of steps on the image, but this is offset by the simplicity of the implementation.
void line( uint8_t x0, uint8_t y0, uint8_t x1, uint8_t y1 ) { int dx, dy, sx, sy; if (x0 < x1) { dx = x1 - x0; sx = 1; } else { dx = x0 - x1; sx = -1; } if (y0 < y1) { dy = y1 - y0; sy = 1; } else { dy = y0 - y1; sy = -1; } int err = dx - dy; while (1) { PORTB = x0; PORTD = y0; if (x0 == x1 && y0 == y1) break; int e2 = 2 * err; if (e2 > -dy) { err = err - dy; x0 += sx; } if (e2 < dx) { err = err + dx; y0 += sy; } } }
Fonts

After we learned to draw lines, nothing prevents us from drawing symbols.
It is convenient to use the
Hershey Fonts library as a font.
In it, each character consists of less than 32 points. All coordinates of the points are located relative to the lower left corner of the symbol and are connected until the value (-1, 1) is encountered, which means that you need to jump to the next point. The contour ends at the point with the value (0, 0).
Example for the number 1.
typedef struct { int8_t x, y; } path_t; static const PROGMEM path_t digits[][32] = { [1] = { { 6,17}, { 8,18}, {11,21}, {11, 0}, }, };
Sometimes in the image you can see the lines between two points that do not connect, this is because the voltage on the DAC does not change instantaneously. This effect can be corrected if you use the brightness channel of the oscilloscope, unfortunately my oscilloscope does not have such a function.
Raster images
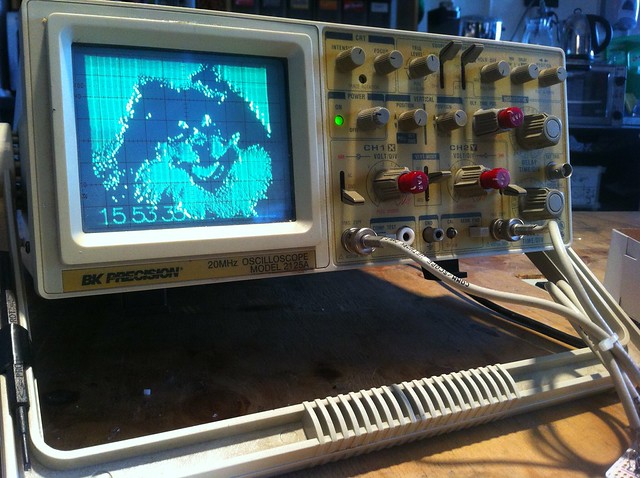
Using the oscilloscope can also display raster images.
Images are obtained by converting the image to the X Bitmap format using ImageMagick. X Bitmap is a text format for representing images, can be used directly in the source code.
convert -resize 256x256 input.jpg image/image.xbm
The result is a monochromatic image that takes up 8 kilobytes of program memory.
Video
If the video is not displayed, it can be found at
www.flickr.com/photos/osr/7925036218/in/set-72157631344809602Future plans
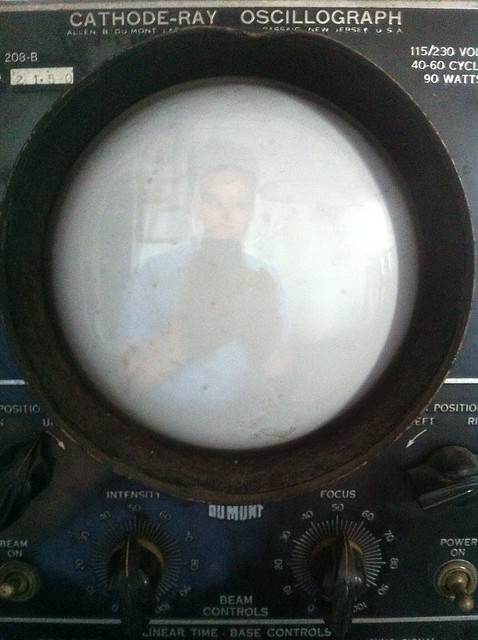
In the future, I plan to restore a wonderful CRT oscilloscope and port Spacewar with a PDP-1.