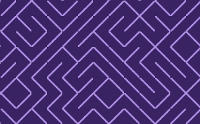
It seemed to me a little strange, the desire of the author of
this post to generate images on the server, while modern browsers allow you to do this on the client. Therefore, I want to suggest another, or rather even two other ways of generating the background.
Immediately I’ll make a reservation that, most likely, they will not work in all modern browsers without revision (tested only in Chrome 24 and FF 16 under Linux).
background-image
As you
know , in CSS3, you can specify several background image files in background-image, and their coordinates in background-position. Consequently, by “shifting” just two small pictures, you can generate a random maze, for example with the following code:
var images = [];
')
I think it is not difficult to guess that such a maze is quite simple to dynamically update, for example, something like this:
for(var i=0;i<images.length*0.05;i++){ images[Math.floor(images.length*Math.random())]=('url('+src[Math.round(Math.random())]+')'); } body.style.backgroundImage = images.join(', '); body.style.backgroundPosition = positions.join(', '); body.style.backgroundRepeat = "no-repeat";
A serious disadvantage of this method is that it inflates the element to indecent sizes, besides it is rather slow (it seems for the same reason).
Canvas + Blob
A little thought, I decided that you can make an implementation on Canvas + Blob. The essence is approximately as follows:
- create a canvas and set its size equal to the size of the element for which we create the background;
- fill the canvas with a picture;
- using JavaScript-Canvas-to-Blob convert content to Blob;
- and using the URL.createObjectURL we get the blob URL.
The resulting URL is assigned to the body.style.backgroundImage property:
var body = document.getElementsByTagName('body')[0]; canvas.toBlob(function(blob){ var url = URL.createObjectURL(blob); body.style.backgroundImage = "url('" + url + "')"; })
The truth is not without drawback. When the backgroundImage value is updated, the background disappears for a moment. Although this is quite easily eliminated, for example, by overlaying pictures for loading time:
var url = URL.createObjectURL(blob); body.style.backgroundImage += ", url('" + url + "')"; body.style.backgroundRepeat = "no-repeat"; setTimeout(function(){ body.style.backgroundImage = "url('" + url + "')"; },200);
Example 1 (background-image)Example 2 (Canvas + Blob)On this, perhaps, everything. I did not set a goal to make a ready, stable, cross-browser (etc.) solution; I just wanted to describe a more rational, in my opinion, approach to solving the problem of generating a background image.
ps I have almost no experience writing articles, so do not swear much if something is wrong.