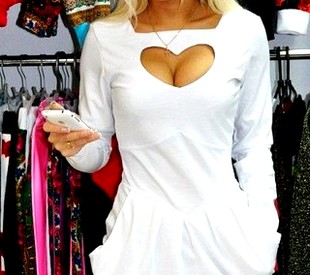
Imagekit is an application that allows you to manipulate images without changing the original image. I think the convenience is understandable, you can have several variants of the picture and always be able to return to the source.
Allows you to resize, create previews, impose watermarks.
Installation
To work with imagekit you will need PIL or PILLOW.
We put from PyPI:
pip install django-imagekit
Add 'imagekit' to INSTALLED_APPS
')
Use in model
import PIL ... from PIL import Image from imagekit.models.fields import ImageSpecField from imagekit.processors import ResizeToFit, Adjust,ResizeToFill class Jobseeker(models.Model): def get_file_path(self, filename): extension = filename.split('.')[-1] filename = "%s.%s" % (uuid.uuid4(), extension) return os.path.join("images", filename) ... photo = models.ImageField(verbose_name=u'Poster',upload_to=get_file_path,max_length=256, blank=True, null=True) photo_small =ImageSpecField([Adjust(contrast=1.2, sharpness=1.1), ResizeToFill(50, 50)], image_field='photo', format='JPEG', options={'quality': 90}) photo_medium =ImageSpecField([Adjust(contrast=1.2, sharpness=1.1), ResizeToFit(300, 200)], image_field='photo', format='JPEG', options={'quality': 90}) photo_big =ImageSpecField([Adjust(contrast=1.2, sharpness=1.1), ResizeToFit(640, 480)], image_field='photo', format='JPEG', options={'quality': 90})
Use in pattern
In view.py we transfer object
def some_def(request): ... photo = Jobseeker.objects.get() ... return (render_to_response('jobseeker/resume_template.html', { ... 'photo': photo, ... }, context_instance=RequestContext(request)) )
and in the template we can refer to the following fields:
photo.photo_small.url # 5050 photo.photo_medium.url # 300200 photo.photo_big.url # 640480 # photo.photo_small.width # 50 photo.photo.width #
In this example, we used 2 image processors, namely: ResizeToFit and ResizeToFill.
In fact, there are several more -
processors Module ResizeToFit(width=None, height=None, upscale=None, mat_color=None, anchor='c')
Purpose: an image with the specified dimensions will be created, on which the original image is superimposed, proportionally compressed, so as not to go beyond the specified frames.
width = None, height = None - width and height, respectively.
upscale - a boolean value that determines whether the image will be enlarged if it is less than the specified parameters
mat_color - the fill color of the blank area of the original image.
ResizeToFill(width, height)
Purpose: to change the size to the specified size, all that is beyond the specified size will be cut off
The attributes respectively indicate the tire and the height.
Work examples
Original photo
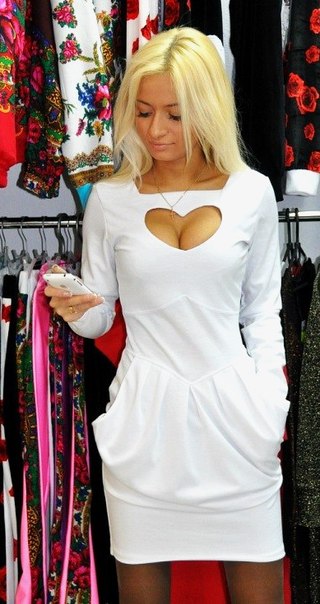
ResizeToFill
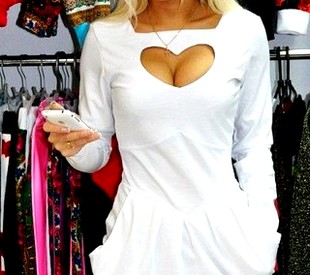
ResizeToFit using the mat_color parameter to show the actual size of the new image

All additional images created using imagekit are saved in the cahe folder, all originals are saved in a separate folder. They are created at the first entry into the page in which they are used. At any time, all the pictures can be deleted without harming the original and recreated with new parameters.
All this greatly facilitates the work with images, and saves the developer’s nerves, if the initial settings were suddenly wrong, and the originals did not think to save.
References:
GithubDocumentation