<nav role=""> <ul> <li><a href="#">Stream</a></li> <li><a href="#">Lab</a></li> <li><a href="#">Projects</a></li> <li><a href="#">About</a></li> <li><a href="#">Contact</a></li> </ul> </nav>
@media screen and (max-width: 44em) { }
<nav role="full-horizontal"> <ul> <li><a href="#">Stream</a></li> <li><a href="#">Lab</a></li> <li><a href="#">Projects</a></li> <li><a href="#">About</a></li> <li><a href="#">Contact</a></li> </ul> </nav>
@media screen and (max-width: 44em) { nav[role="full-horizontal"] { ul > li { width: 100%; } } }
<nav role="select"> <!-- basic menu goes here --> <select onchange="if (this.value) window.location.href = this.value;"> <option value="#">Stream</option> <option value="#">Lab</option> <option value="#">Projects</option> <option value="#">About</option> <option value="#">Contact</option> </select> </nav>
nav[role="select"] { > select { display:none; } }
@media screen and (max-width: 44em) { nav[role="select"] { ul { display: none; } select { display: block; width: 100%; } &:after { position: absolute; content: "Menu"; right: 0; bottom: -1em; } } }
<nav role="custom-dropdown"> <!-- Advanced Checkbox Hack (see description below) --> <!-- basic menu goes here --> </nav>
+
and ~
h1 ~ p { color: black; } h1:hover ~ p { color: red; }
:checked
pseudo-class with a selector ~
. And while the bug was not fixed in WebKit 535.1 (Chrome 13) and is relevant for Android 4.1.2 WebKit 534.30, the hack did not work on any Android device..
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

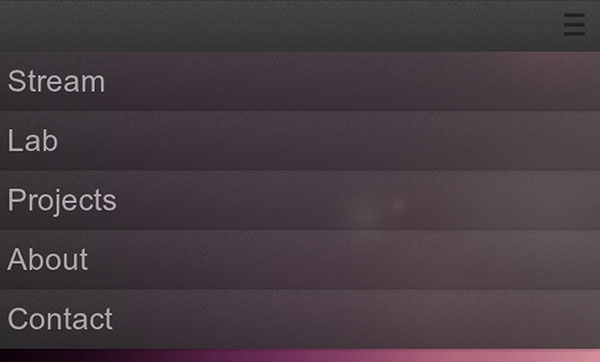
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:
JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

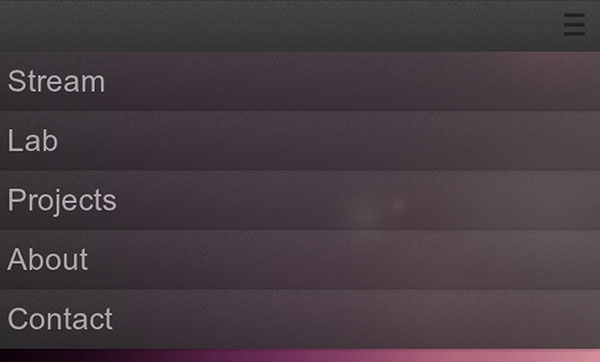
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:
JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

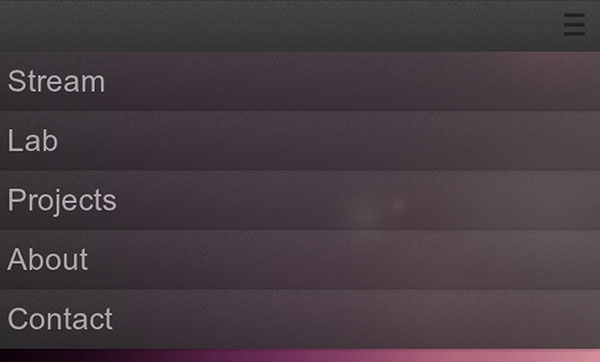
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:
JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

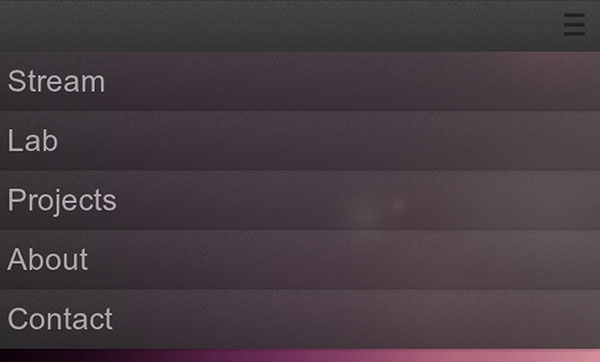
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:
JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

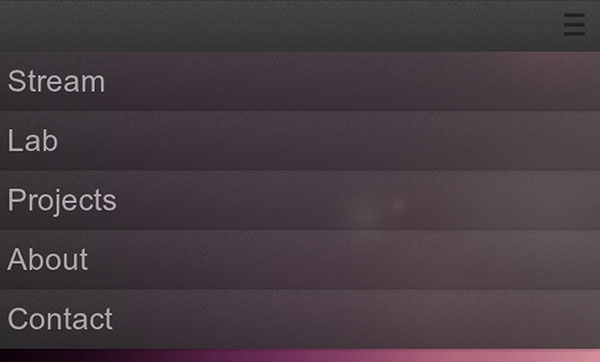
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

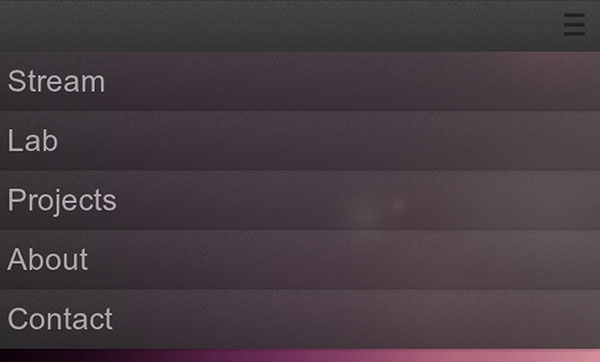
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

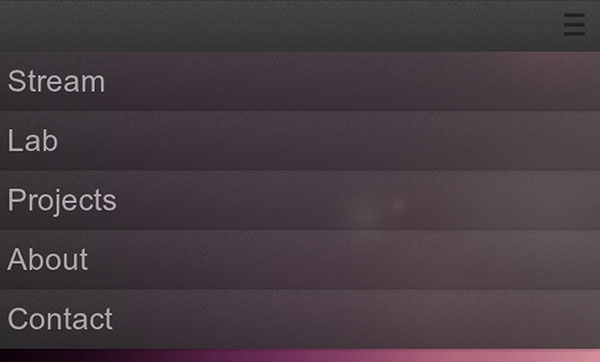
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

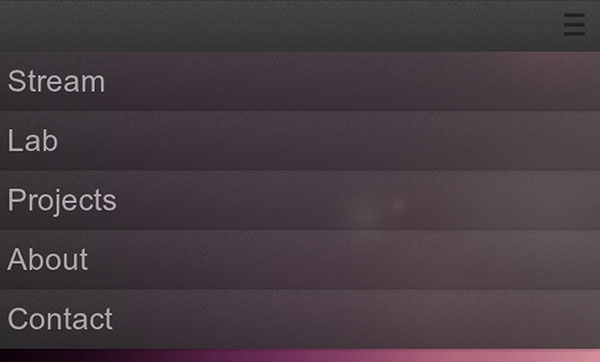
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

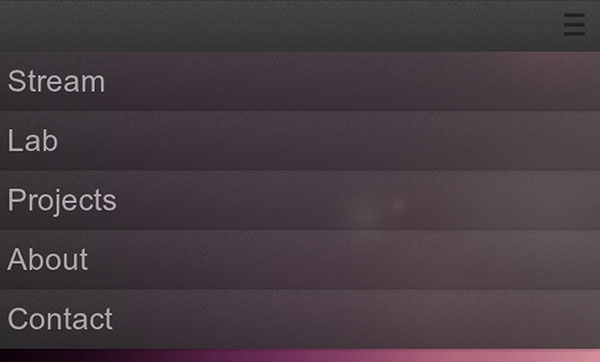
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

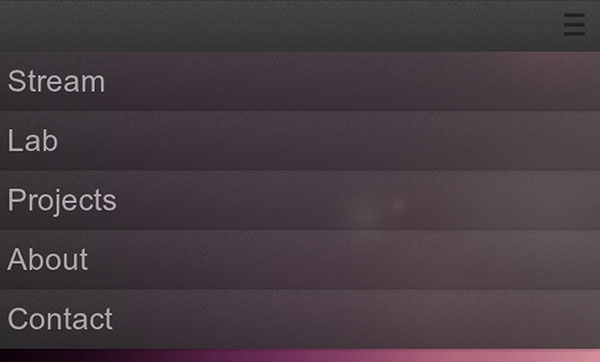
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

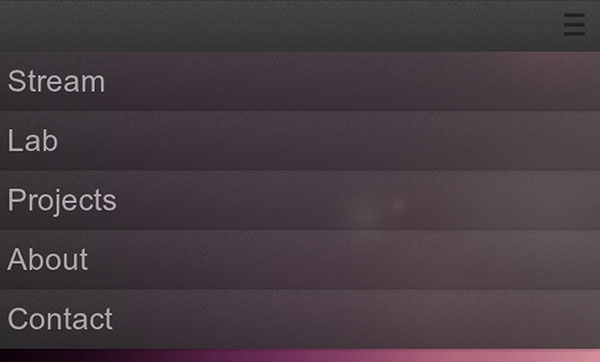
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

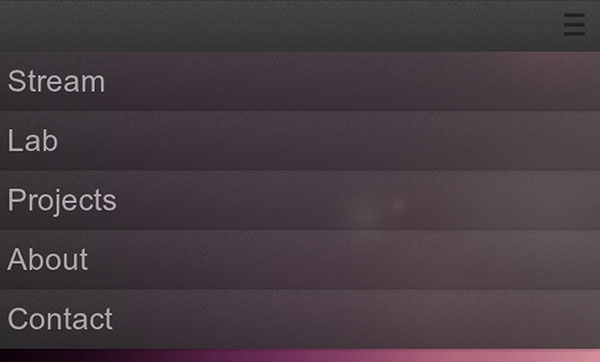
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

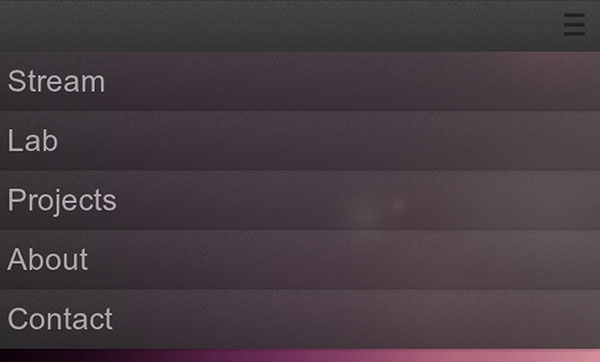
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

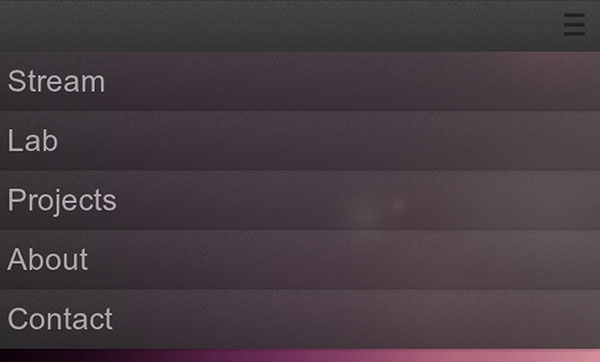
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

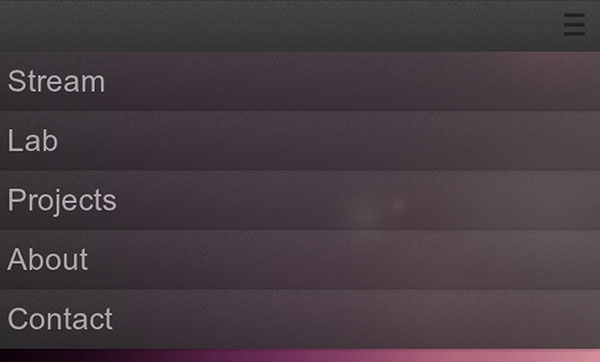
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

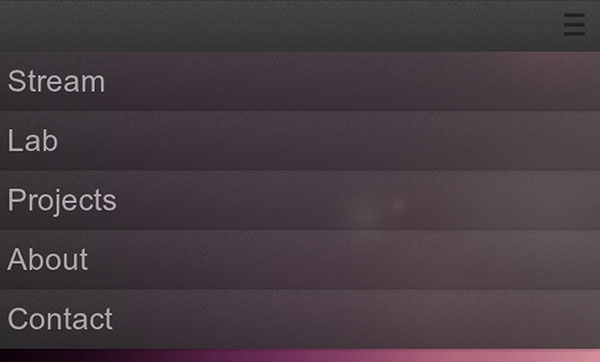
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

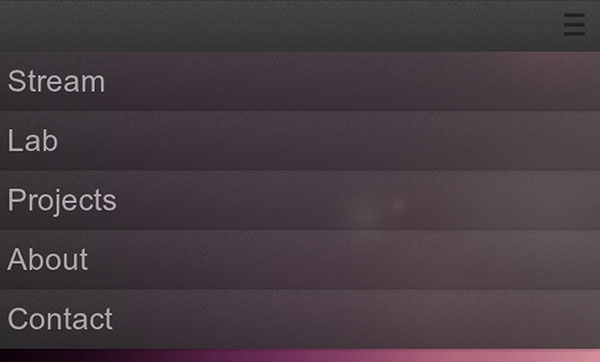
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

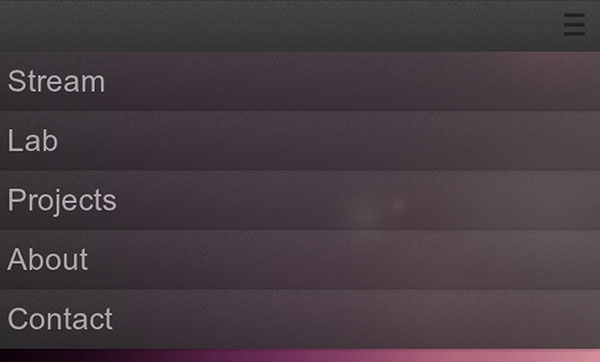
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:
JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

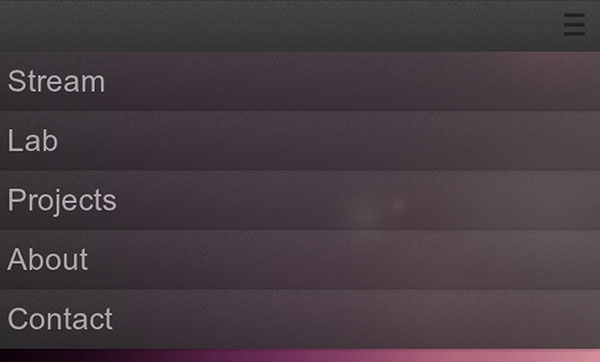
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:
JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

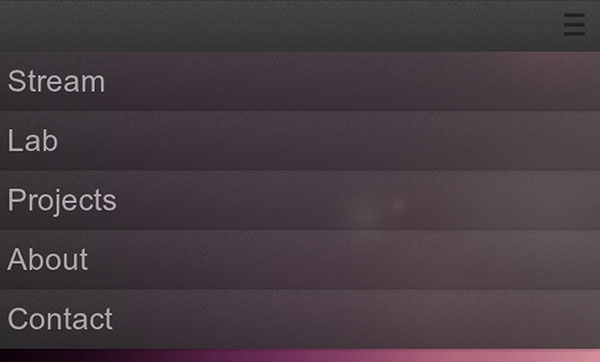
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:
JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

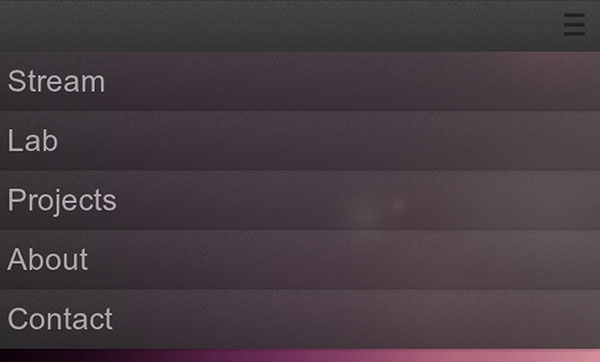
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

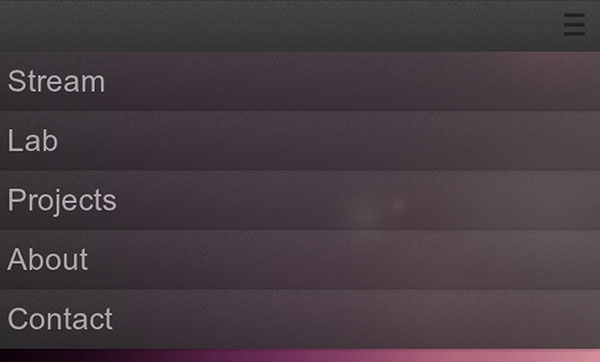
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

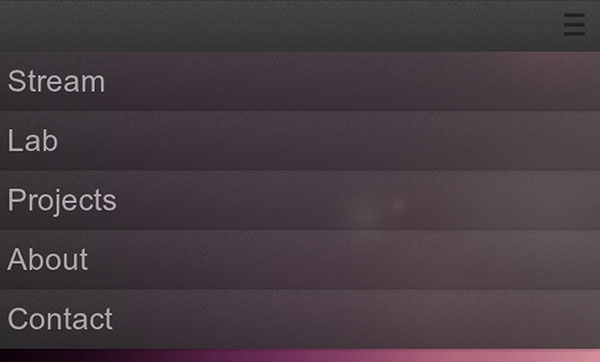
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

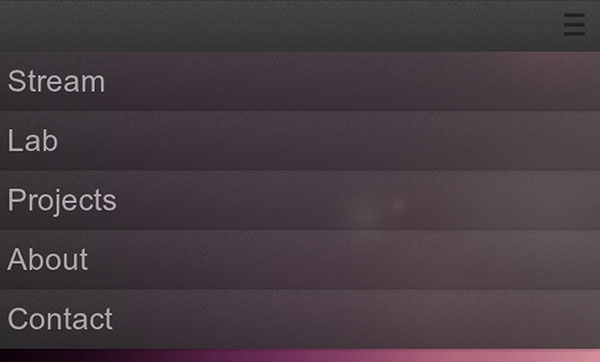
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

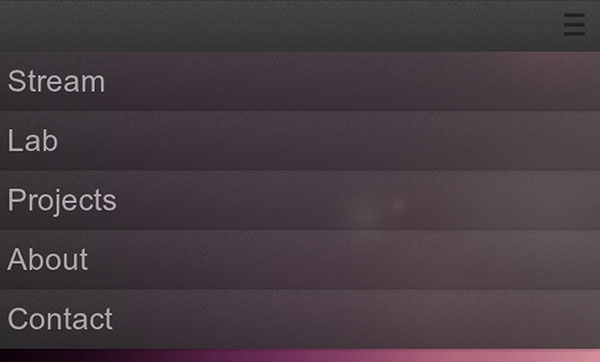
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

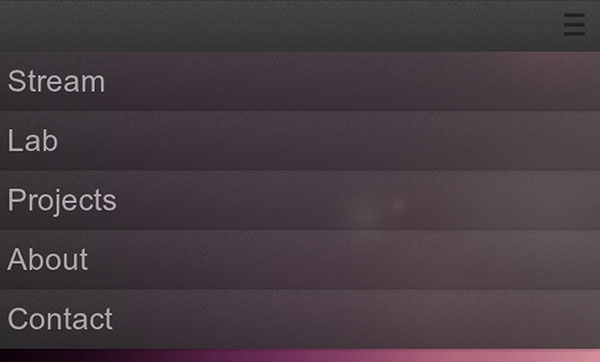
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

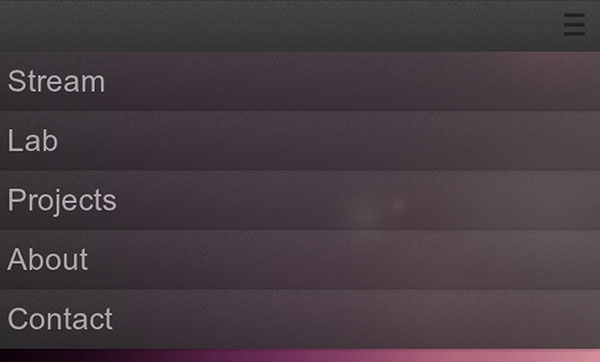
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

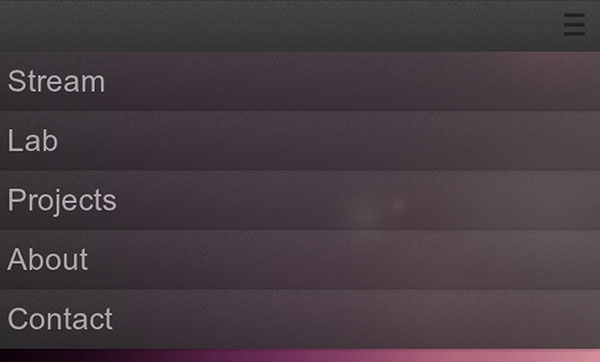
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

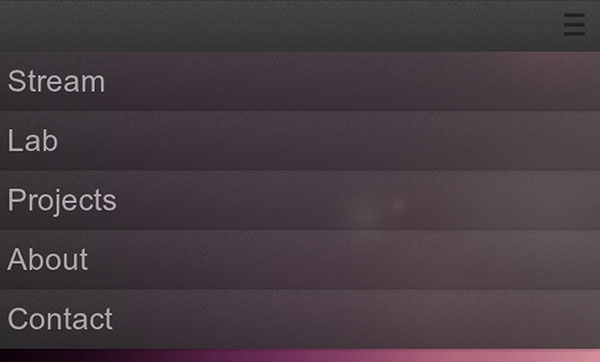
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

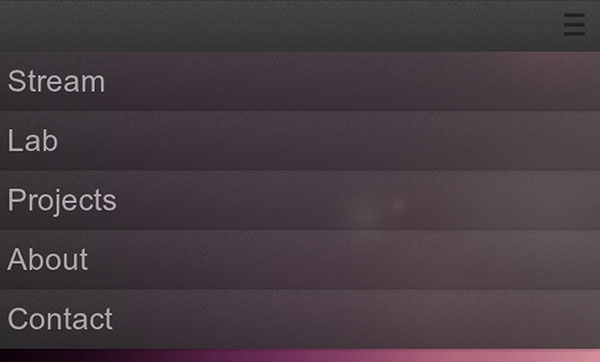
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

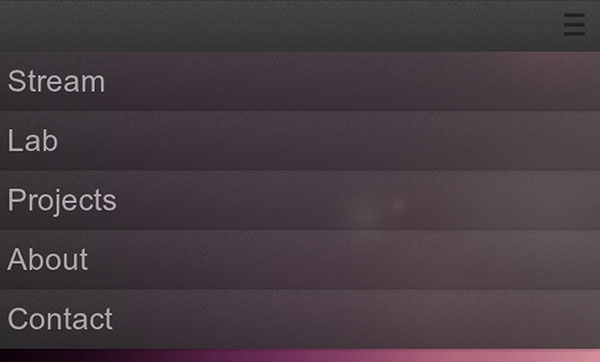
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

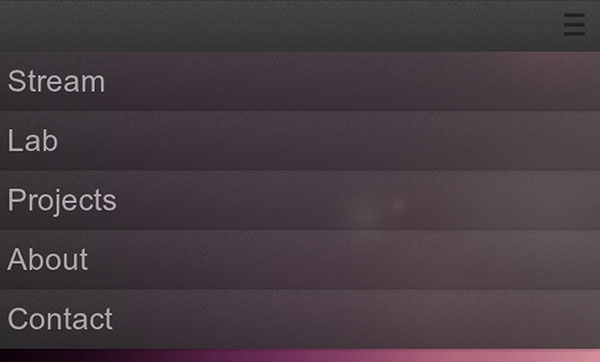
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

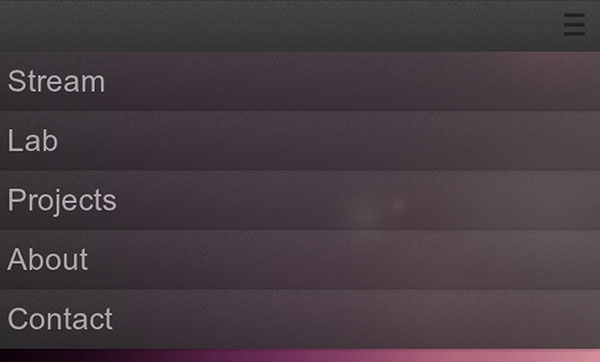
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

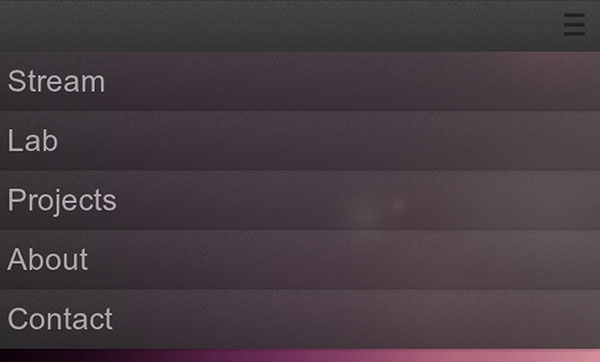
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

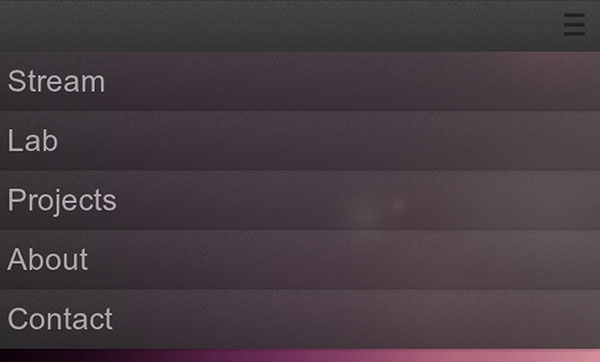
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
.
:
<!-- Fix for iOS --> <input type="checkbox" id="menu"> <label for="menu" onclick></label>
/* Fix for Android */ body { -webkit-animation: bugfix infinite 1s; } @-webkit-keyframes bugfix { from { padding: 0; } to { padding: 0; } } /* default checkbox */ input[type=checkbox] { position: absolute; top: -9999px; left: -9999px; } label { cursor: pointer; user-select: none; }
label:
nav[role="custom-dropdown"] { label { display: none; } }
label. , , "≡" label ( "\2261", ). input, .
@media screen and (max-width: 44em) { nav[role="custom-dropdown"] { ul { display: none; height: 100%; } label { position: relative; display: block; width: 100%; } label:after { position: absolute; content: "\2261"; } input:checked ~ ul { display: block; > li { width: 100%; } } } }
:

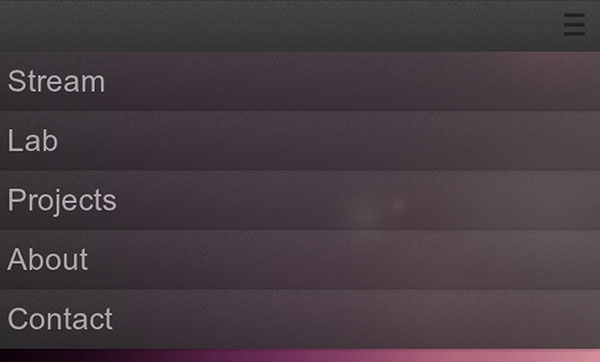
JavaScript
(input / label) HTML
.
4. Canvas
, , input label 3. label, . 80% 20% ( , CSS)
<input type="checkbox" id="menu"> <label for="menu" onclick></label> <!-- basic menu goes here --> <div class="content"> <!-- content goes here --> </div>
label.
label { position: absolute; left: 0; display: none; }
label input. . , , "≡" label ( "\2261", ).
@media screen and (max-width: 44em) { $menu_width: 20em; body { overflow-x: hidden; } nav[role="off-canvas"] { position: absolute; left: -$menu_width; width: $menu_width; ul > li { width: 100%; } } label { display: block; } label:after { position: absolute; content: "\2261"; } input:checked ~ nav[role="off-canvas"] { left: 0; } input:checked ~ .content { margin-left: $menu_width + .5em; margin-right: -($menu_width + .5em); } }
:


JavaScript Facebook / Google+
(input / label) HTML body
.
IE?
: ! , IE8 , , .
Source: https://habr.com/ru/post/159359/
All Articles