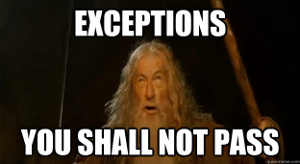
Four months ago,
I wrote about how you can conveniently catch exceptions in node.js, including asynchronous ones, that is, those that are thrown by the code that is called by the event loop. In that article, I used the
control-block module to deal with them, since the standard try-catch unit could not cope.
As it turned out, at about the same time, Adam Crabtree released a stable version of a similar to the control-block module called
trycatch .
The trycatch module provides some additional features that are not in the control-block:
')
1. Eliminates the need to additionally wrap callbacks passed to setTimeout, etc.
To do this, the trycatch module, when loaded, replaces the functions setTimeout, setInterval, the functions of the fs module, so there is no need for constant calls to Block.guard () when passing callbacks. This is done automatically.
2. As a consequence, trycatch provides support for third-party libraries.
Because of the need to wrap callbacks, the control-block could not catch some asynchronous exceptions that occurred in third-party libraries.
Suppose we have such an unreasonable third-party library that is too tough for the control-block module:
function blackBox() { setTimeout(function() { throw new Error('black box error'); }, 10); }
And this is the code that, thanks to trycatch, can now safely work with it:
var trycatch = require('trycatch'); trycatch(function() { setInterval(blackBox, 1000); }, function(err) { console.log('caught!'); console.log(err.stack); });
It will output:
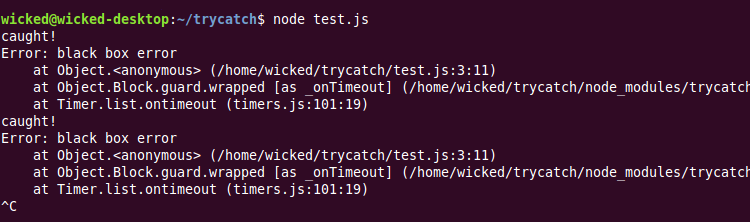
3. Long stack traces
Thanks to integration with the
long-stack-traces module, the trycatch module can help in debugging if you ask it to output long stack traces that correctly track asynchronous exceptions:
var trycatch = require('trycatch'); trycatch.configure({'long-stack-traces': true}); trycatch(function() { setInterval(blackBox, 1000); }, function(err) { console.log('caught!'); console.log(err.stack); });
This code will display the following stack:
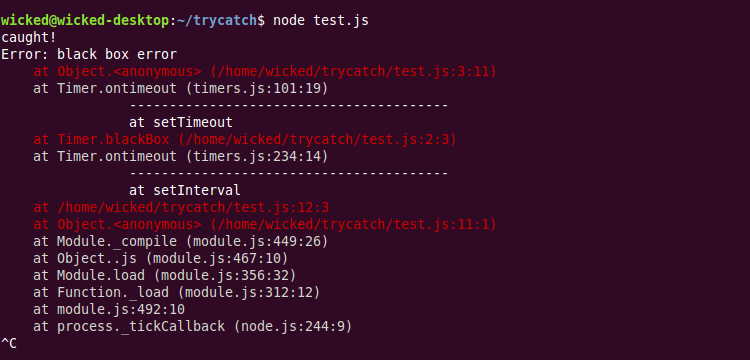
By the way, until recently, in trycatch, the preliminary compilation of a long stack of traces was mandatory, which, compared to the control-block, gave a performance of about 70-100 times lower. I
discussed this problem with the author, suggested a possible solution, and yesterday they
released a new version , which is now based on the control-block module, which gives a high speed of the module. At the same time, it is possible to include long stack traces when necessary, for example, on the server where development is underway, and speed is not critical. It turned out a sort of symbiosis, which took from both projects only the best.
So despite the fact that the trycatch module, even in my opinion, is
not quite perfect yet, I think that this is the best solution to handle asynchronous exceptions.