As is known, many functions of working with threads are absent in the software interface of applications for the Windows Store (Windows Store apps), starting with CreateThread () and ending with working with TLS keys. And this is an excellent reason to move from parallelism based on system-dependent threads to parallelism based on tasks. This post outlines a step-by-step instruction on how to write the simplest multithreaded example that is being evaluated for the Windows App Store (Windows App Certification Kit validation) and, hypothetically, can be scaled to space-scale toys. And since the Intel Threading Building Blocks cross-platform library (Intel TBB, TBB,
threadingbuildingblocks.org ) is used, the computing part can be easily transferred to other platforms, and the task will be only to draw a new beautiful graphical interface.
The recently released release of the Intel TBB library tbb41_20121112oss, which is available for download on our website
threadingbuildingblocks.org , adds experimental support for applications for the Windows store, i.e. using only an authorized software interface for developing such applications.
And so, what needs to be done to build a simple application using Intel TBB.
First you need to unpack and compile the library, since this release is distributed only in source code. It is assumed that there is gnu make, in the command line for studio 2012 we execute the command
gnumake tbb tbbmalloc target_ui=win8ui target_ui_mode=production
From the library side, go to the studio.
We create a new project “Blank App (XAML)” using the standard template “Visual C ++” -> “Windows Store”. For simplicity, let's leave the default project name “App1”.
Now add the <tbb directory> / include directory to the project “Additional Include Directories” and the directory with the built tbb.lib library in the Additional Library Directories.
Then add a couple of buttons to the main page (Class App1.MainPage). After that, the page XAML file will look something like this.
<Page x:Class="App1.MainPage" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:local="using:App1" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" mc:Ignorable="d"> <Grid Background="{StaticResource ApplicationPageBackgroundThemeBrush}"> <Button Name="SR" Margin="167,262,0,406" Height="100" Width="300" Content="Press to run Simple Reduction"></Button> <Button Name="DR" Margin="559,262,0,406" Height="100" Width="300" Content="Press to run Deterministic Reduction"></Button> </Grid> </Page>
')
By the way, before connecting the library it would be good to check that up to this point we did not have time to mess up in the code, and there are all the necessary rights and developer licenses. To do this, you need to build and run the application, and check that the buttons are pressed, and nothing falls. With a positive result, go to the library connection.
We connect the TBB and add button handlers. For example, let's take the reduction (tbb :: parallel_reduce) and
deterministic reduction (tbb :: parallel_deterministic_reduce) algorithms and add the MainPage.xaml.cpp to the source file for the main page:
#include "tbb/tbb.h" void App1::MainPage::SR_Click(Platform::Object^ sender, Windows::UI::Xaml::RoutedEventArgs^ e) { int N=1000000; float fr = 1.0f/(float)N; float sum = tbb::parallel_reduce( tbb::blocked_range<int>(0,N), 0.0f, [=](const tbb::blocked_range<int>& r, float sum)->float { for( int i=r.begin(); i!=r.end(); ++i ) sum += fr; return sum; }, []( float x, float y )->float { return x+y; } ); SR->Content="Press to run Simple Reduction\nThe result is " + sum.ToString(); } void App1::MainPage::DR_Click(Platform::Object^ sender, Windows::UI::Xaml::RoutedEventArgs^ e) { int N=1000000; float fr = 1.0f/(float)N; float sum = tbb::parallel_deterministic_reduce( tbb::blocked_range<int>(0,N), 0.0f, [=](const tbb::blocked_range<int>& r, float sum)->float { for( int i=r.begin(); i!=r.end(); ++i ) sum += fr; return sum; }, []( float x, float y )->float { return x+y; } ); DR->Content="Press to run Deterministic Reduction\nThe result is " + sum.ToString(); }
And the XAML file for the main page will look like this.
<Page x:Class="App1.MainPage" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:local="using:App1" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" mc:Ignorable="d"> <Grid Background="{StaticResource ApplicationPageBackgroundThemeBrush}"> <Button Name="SR" Margin="167,262,0,406" Height="100" Width="300" Content="Press to run Simple Reduction" Click="SR_Click"></Button> <Button Name="DR" Margin="559,262,0,406" Height="100" Width="300" Content="Press to run Deterministic Reduction" Click="DR_Click"></Button> </Grid> </Page>
Add the tbb.dll and tbbmalloc.dll libraries to the application container. To do this, add files to the project (via the project-> add existing item) and set the “Content” property to “Yes”. In this case, the files will be copied to the container (AppX) inside the application and can be loaded both at the start of the application and later.
Is done. You can run the simulator and see how the application works:
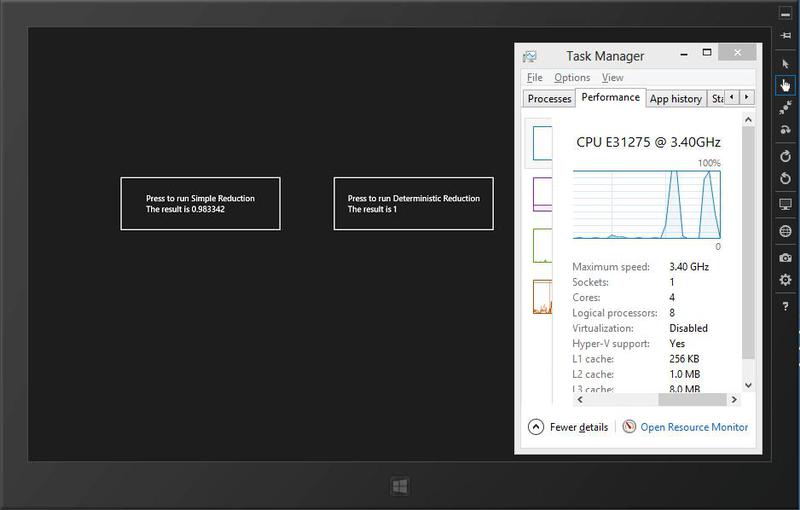
The next step is to run the “Windows App Cert Kit” and check that the application successfully passes certification:
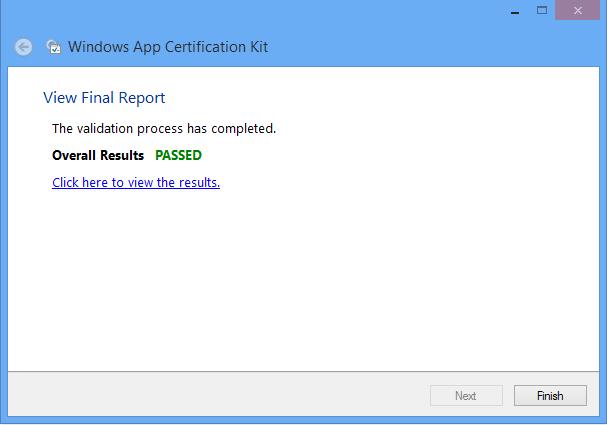
That's all! A simple application is ready, now you can complicate things.
For those interested, try:
Download Intel Threading Building Blocks Library (Open Source Version):
threadingbuildingblocks.orgCommercial version of Intel TBB (not functionally different):
software.intel.com/en-us/intel-tbbEnglish and Russian blogs about Intel TBB
software.intel.com/en-us/tags/17207software.intel.com/en-us/tags/17220And, of course, our forum,
software.intel.com/en-us/forums/intel-threading-building-blocks