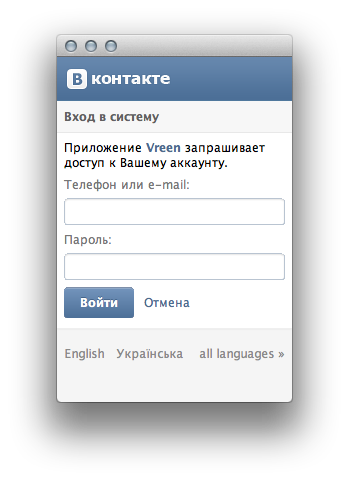
I present to you a new Qt library for working with vk api, which can be useful to you when creating any desktop and mobile applications that interact with vk. The project was born from the vkontakte plugin for qutIM and has grown into a separate independent library, which everyone can now use.
Short review
Vreen is a wrapper over vk.com api, written in C ++ 11 / Qt, designed for quick and easy creation of mobile and desktop applications, as well as embedding interaction with vk.com api into existing applications. The library supports several authorization methods and allows you to add your own if necessary. Also in vreen there is a full binding to qml, which allows you to create applications without writing a single line in C ++. To reduce the number of required dependencies, all authorization methods are moved to separate static libraries.
')
Key features:
- Support any kind of dialogue including group chats.
- Support viewing news feeds, walls.
- Work with comments, including uploads, likes and reposts.
- Support work with attachments.
- Support roster including tracking the status of interlocutors.
- Audio support.
- The ability to directly work with the API from qml in the usual for many XHTTPRequest style.
- Extensibility
- A free LGPL license allowing use of a library with proprietary applications.
Basics:
Base classes for working with API:
- Connection is a class based on QNetworkAccessManager that performs authorization and directly implements API requests.
- Reply - the class tracks the execution of the request.
- Longpoll is a class that polls the server for events.
- Roster is a class that manages the list of friends.
- Client is a base class that stitches everything together.
Performing API requests:
Simple request:
QVariantMap args; args.insert("uids", join(ids)); args.insert("fields", fields.join(",")); auto reply = d->client->request("users.get", args); reply->connect(reply, SIGNAL(resultReady(const QVariant&)), this, SLOT(_q_friends_received(const QVariant&)));
The resultReady signal is thrown if the request is completed and in response contains a response from a server converted to QVariant json or nothing in case of an error, the error code in this case is contained in reply-> error ()
In addition, you can hide the result processing from the recipient by adding a result handler function to the reply; in this case, the result can be obtained by calling the reply-> result () method, the result will be returned in the form of a QVariant, so you will need to add more convert using QVariant :: fromValue.
QVariantMap args;
In order to clearly indicate the type of return value, you can use the following template magic:
typedef ReplyBase<int> SendMessageReply; QVariantMap args;
As a result, in the slot, SendMessageReply :: result () will return an int instead of QVariant.
auto reply = static_cast<Vreen::SendMessageReply*>(sender()); m_unreachedMessagesCount--; int mid = reply->result();
In general, all interaction with the API is simple and transparent.
Receiving and assembling
Vreen uses submodules and therefore the most optimal way to get the source is.
$ git clone git://github.com/gorthauer/vreen.git $ cd vreen $ git submodule update --init
The build requires cmake 2.8, Qt 4.7.4, and any compiler with basic C ++ 11 support (gcc 4.4+, clang 3.0+, msvc2010 +).
The assembly itself is quite trivial:
$ mkdir build $ cd build $ cmake .. -DCMAKE_INSTALL_PREFIX=/usr $ make $ make install (sudo)
If you have questions regarding the build release or debug version, it’s best to simply refer to the cmake documentation. As an experimental option, build with qbs is supported. It is possible to build a library with qmake ', but this method is not officially supported.
Use in C ++ project
Connection
To connect to other projects in Vreen, pkg-config is used, so to connect vreen with the standard authorization method through a browser, it is enough to add a line to the pro file.
CONFIG += link_pkgconfig PKGCONFIG += vreen \ vreenoauth
In the case of systems that do not support pkgconfig, you can either manually add LIBS and INCLUDEPATH or write a find script for cmake or qbs.
Those interested can help me write a prf file for Qt.
Using
Let's write as an example a small console application that will pull out all the phone numbers from the list of friends.
To begin with, we simply initialize the client, indicating to it the connection method, application identifier, and also ask it to independently create a window with permission for access from the application to the api.
auto auth = new Vreen::OAuthConnection(3220807, this);
After the client successfully connects to the api, the onOnlineChanged slot will be called in which we will request a list of friends from the roster. When prompted, you can select the fields you want to receive. There are several macros with the most common VK_COMMON_FIELDS, VK_EXTENDED_FIELDS and VK_ALL_FIELDS options for all known fields.
The description of the fields and the resulting properties can be read
here u.
In this case, we are only interested in three fields and there is no need to strain the server with more difficult requests.
roster()->sync(QStringList() << QLatin1String("first_name") << QLatin1String("last_name") << QLatin1String("contacts"));
After successful completion of the synchronization of the roster, simply display the result:
qDebug() << tr("-- %1 contacts recieved").arg(roster()->buddies().count()); foreach (auto buddy, roster()->buddies()) { QString homeNumber = buddy->property("_q_home_phone").toString(); QString mobileNumber = buddy->property("_q_mobile_phone").toString(); qDebug() << tr("name: %1, home: %2, mobile: %3").arg(buddy->name()) .arg(homeNumber.isEmpty() ? tr("unknown") : homeNumber) .arg(mobileNumber.isEmpty() ? tr("unknown") : mobileNumber); }
All properties that have not yet been implemented properly for contacts will have the _q_ prefix, the rest can be obtained via getters or through the names specified in Q_PROPERTY.
As a second example, try using qml api and get a list of friends.
We import the support of Vreen into qml:
import com.vk.api 1.0
And create a client:
Client { id: client connection: conn
We will simply connect via client.connectToHost, in the confirmation window that appears, you will be able to enter your login and password, so you can not create separate fields for input, but confine ourselves to an extremely concise invitation.

To display a list of dialogs, you can use the finished model from com.vk.api 1.0:
DialogsModel { id: dialogsModel client: client }
To get the list of the last conversations, it is rather easy to request the list after connecting:
Connections { target: client onOnlineChanged: { if (client.online) { client.roster.sync(); dialogsModel.getDialogs(0,
The properties of the model available to the delegate can be seen in the code for its implementation:
roles[SubjectRole] = "subject"; roles[BodyRole] = "body"; roles[FromRole] = "from"; roles[ToRole] = "to"; roles[ReadStateRole] = "unread"; roles[DirectionRole] = "incoming"; roles[DateRole] = "date"; roles[IdRole] = "mid"; roles[AttachmentRole] = "attachments"; roles[ChatIdRole] = "chatId";
Using these fields you can get a quite neat looking list of dialogs:

Similarly, create a photo album:
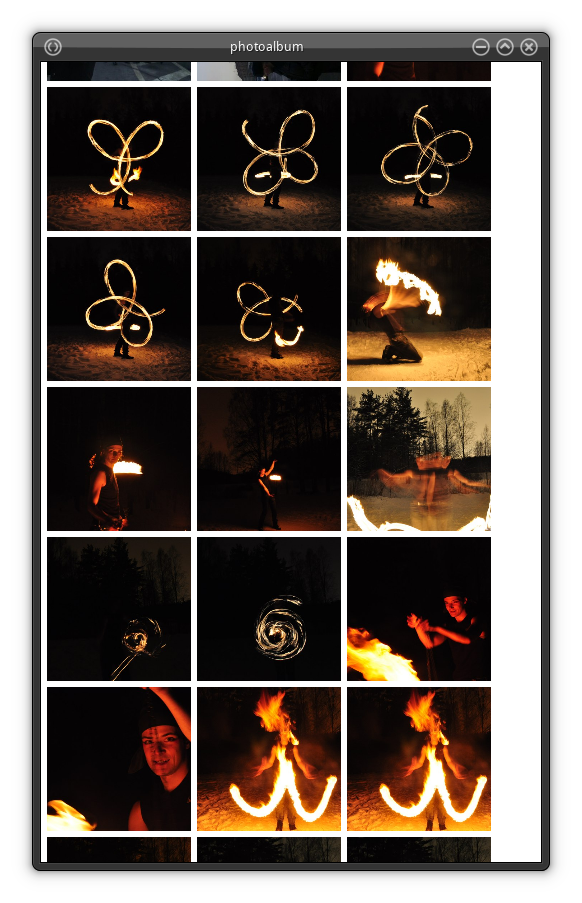
And I would like to note that in the case of creating a photo album, we work through qml directly with vk.api
function getAll(ownerId, count, offset) { if (!offset) offset = 0; if (!count) count = 200; var args = { "owner_id" : ownerId, "offset" : offset, "count" : count, "extended" : 0 } var reply = client.request("photos.getAll", args) reply.resultReady.connect(function(response) { var count = response.shift() for (var index in response) { var photo = response[index] model.append(photo) } }) }
It turns out very briefly and concisely.
Conclusion
The current version of vreen is 0.9.5, that is, in fact, it is almost a release, which means that the API for the most part will no longer change and binary compatibility between versions will be maintained, which means you can already safely join the use and testing in real conditions.
At the moment, API support is still very incomplete, but the writing of wrappers over it is simplified as much as possible, so that I will be happy with all the patches with the implementation of various features.
For other questions, ready to answer in a personal or jabber'e, which can be found in the profile.
Fork me with github .