Probably every Android programmer at least once thought about writing something useful using computer vision or augmented reality. And some even wrote hello, word using opencv, which they also ported to Android. Unfortunately, if we want to write something serious, we will find that the set of libraries with already implemented functions of Computer Vision is not so great, especially this concerns the Android platform. Most often for this purpose they use opencv, written in C ++, or write their bikes, which is generally good, but not as fast as we would like in terms of implementation. However, not everything is so bad. There is such a wonderful project
BoofCV , which is a computer vision library written in pure Java. The last two letters in the name of the library mean exactly what you thought. And in the latest release appeared the long-awaited support for Android. Below we look at the main buns provided by the library on a specific example.
BoofCV - short description
So, BoofCV is an open source computer vision library written in pure Java. The algorithms used inside are well optimized and, as practice shows, in speed in some cases they are not inferior to the implementation in C ++ opencv. The main features of the library:
• Work with video and web cameras;
• 3D Computer Vision;
• Filters (blur, gradient), noise removal (using wavelets);
• Binarization, morphological operations;
• Allocation of boundaries (Kenny, Sobel);
• Search for points of interest;
• Search for lines, segments, rectangles;
• Stereo images.
In addition, the library contains many more functions used in computer vision. The library is connected quite simply - you can download the source code from the official site and compile it yourself, or you can download the jar files already collected and simply connect them to the project.
')
For experiments, take this picture:

First, let's try a Gaussian blur:
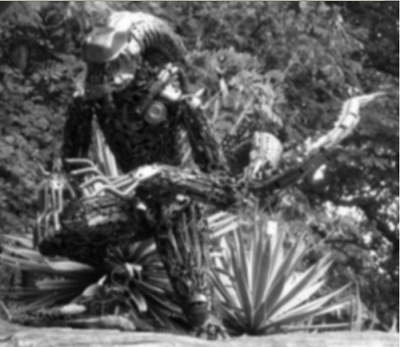
To get this picture, we use the BlurImageOps.gaussian function:
// Here and below, image is a source image of type ImageUInt8
ImageUInt8 blurred = new ImageUInt8(image.width,image.height) ImageUInt8 blurred = new ImageUInt8(image.width,image.height); BlurImageOps.gaussian(image,blurred,-1,5,null);
Now add noise to the original image and filter it:
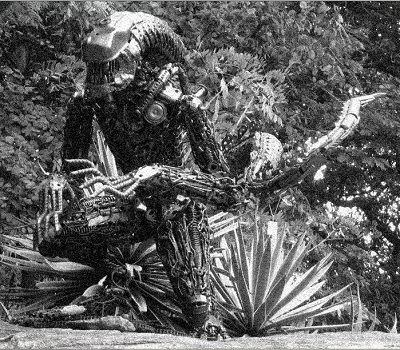

Add and remove noise:
Random rand = new Random(234); ImageUInt8noisy = image.clone(); GeneralizedImageOps.addGaussian(noisy,rand,20,0,255); ImageUInt8denoised = new ImageFloat32(ImageUInt8.width, ImageUInt8.height); int numLevels = 4; WaveletDenoiseFilter< ImageUInt8> denoiser = FactoryImageDenoise.waveletBayes(ImageUInt8.class,numLevels); denoiser.process(noisy,denoised);
Allocation of borders:

Code:
I
mageUInt8 canny = new ImageUInt8(image.width,image.height);
Image binarization:

Code:
ImageUInt8 binary = new ImageUInt8(image.width,image.height); float mean = PixelMath.sum(image)/(image.width*image.height); ThresholdImageOps.threshold(image, binary,mean,false);
Binarized image markup:
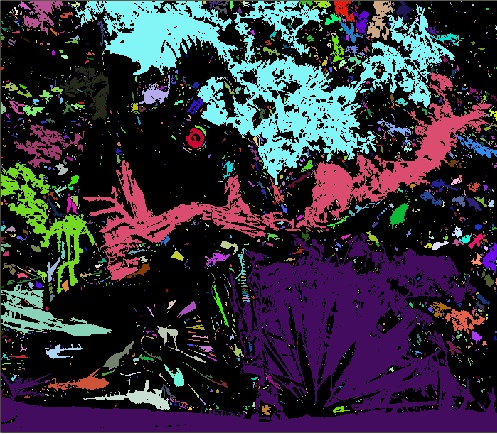
Code:
ImageSInt32 blobs = new ImageSInt32(image.width, image.height); int numBlobs = BinaryImageOps.labelBlobs4(binary,blobs);
Search points of interest:
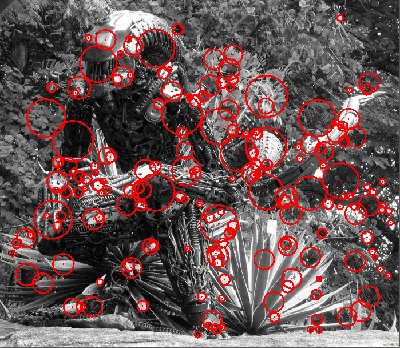
I will not give the code in order not to overload the article, an example is in the manual.
And then a natural question arises - where is Android? In order to use the library in Android, there is a class ConvertBitmap, containing methods for converting from Android format to BoofCV library format and back.
For example:
Bitmap map = BitmapFactory.decodeResource(getResources(), R.drawable.car5); ImageUInt8 image = ConvertBitmap.bitmapToGray(map, (ImageUInt8)null, null);
Conclusion:
Of course, this is only a small part of all the capabilities of the library. According to the functional BoofCV, of course loses to such monsters as opencv. However, the threshold for entering computer vision for Android is significantly reduced when using BoofCV.
At the same time, the library showed good performance, which allows it to be used in real-time systems. So the search and localization of the rectangle with the necessary parameters (for example, car number) takes about 0.2 - 0.5 seconds, depending on the size of the image. The library is well documented.