In the
previous part, we looked at creating a plugin for Pastebin, and also learned how to create actions for modules and associate them with a specific command in the menu. In this article we will expand the functionality of the plugin by adding authorization and a settings window.
Settings window
Let's start right away by creating a tab in the settings window. In “New-> Other-> Module Development”, select “Options Panel” and click “Next>”.
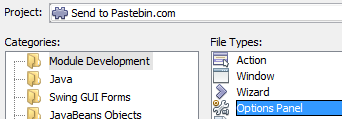
We are offered to choose the creation of a primary or secondary panel in the settings. We don’t need a primary one; we are completely satisfied with the place in the “Miscellaneous” panel, right? Select “Create Secondary Panel”, in the field “Primary Panel” set “Advanced”, specify the title and keywords, for example “Pastebin”.
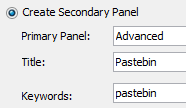
')
In the next step, check that the "Package" is correctly specified, and leave the "Class Name Prefix" by default. Click "Finish" and get the generated PastebinOptionsPanelController class and the PastebinPanel form. You can already run the module for review.

As you can see, the tab was created where needed, but it is still empty. Let's fix the situation.
For successful authorization in pastebin we need a login and password. Drag and drop to PastebinPanel JTextField and JPasswordField and arrange it more conveniently, calling them loginTextField and passwordField.

The settings window we created.
Saving and restoring settings
Opening the PastebinPanel class code, you can easily guess that the store () / load () methods are responsible for saving and restoring settings. Netbeans even shows us three possible options for committing actions in the commented lines. The first option is to save / load through the Preferences API, the second and third are using the Netbeans Platform. We will approach the second proposed method, since the third is obsolete. Write the code:
public static final String LOGIN_KEY = "PastebinLogin", PASSWORD_KEY = "PastebinPassword"; void load() { loginTextField.setText(NbPreferences.forModule(PastebinPanel.class).get(LOGIN_KEY, "")); passwordField.setText(NbPreferences.forModule(PastebinPanel.class).get(PASSWORD_KEY, "")); } void store() { NbPreferences.forModule(PastebinPanel.class).put(LOGIN_KEY, loginTextField.getText()); NbPreferences.forModule(PastebinPanel.class).put(PASSWORD_KEY, String.valueOf(passwordField.getPassword())); }
It's time to test performance. We start, we enter values, we click Ok, we come again and we see that everything is successfully saved. Well, with the saving and loading figured out, it was not difficult.
Pastebin authorization
In the last part, we already wrote the sending code to pastebin, so the
PastebinRequest and
PastebinSender classes remained with us. Remove the
append line
(“api_user_key”, "") from the PastebinRequest and add the methods:
public void setUserKey(String key) { append("api_user_key", key.trim()); } public String getUserKeyRequest(String user, String pass) { StringBuilder request = new StringBuilder(); request.append("api_dev_key=").append(API_DEV_KEY); request.append("&api_user_name=").append(encode(user)); request.append("&api_user_password=").append(encode(pass)); return request.toString(); }
The PastebinSender class will have to be slightly
rewritten .
Now in the PastebinAction class, add the following lines to the end of the setupRequest method:
String user = NbPreferences.forModule(PastebinPanel.class).get(PastebinPanel.LOGIN_KEY, ""); String pass = NbPreferences.forModule(PastebinPanel.class).get(PastebinPanel.PASSWORD_KEY, ""); String userKey = PastebinSender.getUserKey(request.getUserKeyRequest(user, pass)); request.setUserKey(userKey);
We start, enter our data in the settings and try to send the code. After a few seconds, a window appears with a link. We see that the sender of the code is no longer Guest, but we. Hooray, we got our way!
Localization
As in the previous article, we will not dwell on the English version of the plugin, so we will add the Russian language to it. If you did not forget to do this last time, now you should have two files: Bundle.properties and Bundle_ru_RU.properties. Opening the first file, you can see that the lines were automatically added there:
PastebinPanel.jLabel1.text = Login:
PastebinPanel.loginTextField.text =
PastebinPanel.jLabel2.text = Password:
PastebinPanel.passwordField.text =We don’t need the second and last lines, so we delete them. You will also need to remove the binding of remote lines to the components PastebinPanel. Open this class in Design mode, select the loginTextField and select “Customize Code” in the context menu. We give the code to this form:

You should do the same with passwordField.
Now in the Bundle_ru_RU.properties we add the lines:
PastebinPanel.jLabel1.text = Login:
PastebinPanel.jLabel2.text = Password:Run, see the result.

It remains only to create an NBM module. How to do this, I described in the last article.
Results
We learned how to create a settings panel for the module, save and restore settings. We also improved the pastebin.com client by adding authorization.
Link to the project received in this part
here.This article may also be helpful.