
It seems that Js is “just a scripting language” in which I will quickly add the necessary features on the page, without worrying about the quality of the code. Once it was true. Even the current Js and Html standards generally hold to this philosophy.
But times have changed! Now, Js create complex and intricate web applications that simply cannot help but run into the problems of their “big brothers” (server applications written in Java, C #, etc.). So, in order not to get bogged down in thousands of lines of “spaghetti code”, it is required to abandon the “just script” philosophy. You need to take Js as a tool with which you want to create a complex, modular and scalable system.
In the framework of the “serious language for serious tasks” philosophy, I want to start a cycle of small articles on the Js world from the side of a Java developer. Let's start with the tools and various design patterns.
')
1. Good IDE
Do not waste time digging in Js through a notebook. For serious code, you need a serious IDE. If you are sitting in IDEA, then js support is already out of the box, but what if you use Eclipse? For this case,
NetBeans unexpectedly fits. Yes, yes, this IDE has decent support for Js! Just run it in parallel with Eclipse and add not java sources to it, but folders with js, css and html (jsp).
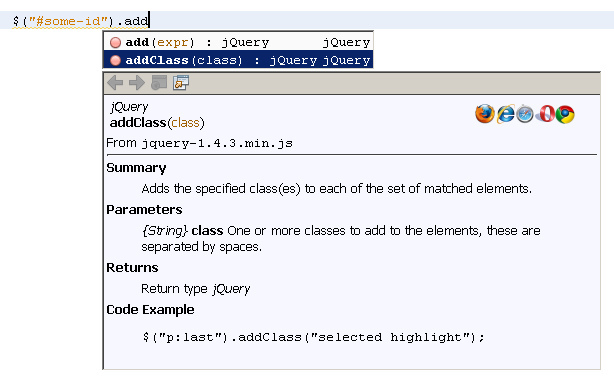
2. Logging
Can you imagine your server code without logging? Well then, how can you provide client code without logs? Use a logging library that supports different browsers. For example,
log4javascript .

3. Debag
It's all clear. For FireFox we set FireBug, in Opera and Chrome we use built-in debuggers.
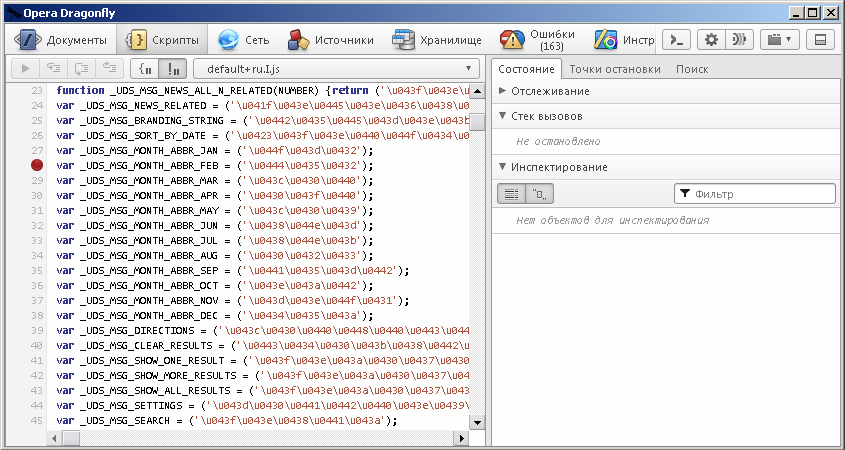
4. Refusal of global functions
Js are very disposed towards the same global context. But we know that in a large application modularity is needed, simple isolated contexts are needed. Therefore, remember the OOP: isolate the logic into separate classes, and do not spread it over dozens of global functions that can be called from anywhere, and which can always be accidentally blocked by creating a function with the same name.
An example of isolation of logic in the "class":
/** * javadoc * @param {String} str - */ NoteDocument = function(str){ // var self = this; // var data; // this.init = function(){ parse(str); }; // function parse(str){ ... } };
How to create an instance:
var doc = new NoteDocument("hello!"); doc.init();
It resembles Java code, doesn’t it? :)
5. Using packages
There is no package support in the language itself. Alas. So have to use any library.
An example of such a library:
function namespace(){}; namespace.prototype.useNamespace = function(newNamespace){ var namespaces = newNamespace.split("."); if (!this[namespaces[0]]){ this[namespaces[0]] = new namespace(); } if (namespaces.length > 1){ this[namespaces[0]].useNamespace(newNamespace.substring(namespaces[0].length+1)); } };
Next in the application we create the root package ...
var app = new namespace();
... and translate our classes into it:
app.useNamespace("model.note"); app.model.note.NoteDocument = function(str){ ... } ... var doc = new app.model.note.NoteDocument("hello!"); doc.init();
6. Unit testing
Yes, and this can also be done in Js! After all, tests are great! Use, for example, the
QUnit library.
An example of a unit test that is “almost like a JUnit test”:
app.useNamespace("model.note"); app.model.note.NoteDocumentTest = function(){ this.test_get_sections_and_blocks = function(){ var data = new TestNoteModel(); var doc = new app.model.note.NoteDocument(data.note);
7. Static code analysis
In the scripting language, you need to be careful, because you can easily skip the syntax error. Add a static code analyzer to the project that will do the dirty work for you to find silly typos with each build. For example,
jslint .

Results
In this article, we looked at a set of development tools and templates in Js that serve to improve code quality and support complex client applications. Have a nice work!