Containers (Container, Panel, TabPanel, ViewPort) and markup (BorderLayout, AccordionLayout, CardLayout)
Introduction
Well, let's start. Our ultimate goal will be to create an interface similar to
Microsoft Outlook 2010 . Why this particular interface? The reason is simple - because Ext.NET is often used in the corporate sector or close to it, and the user of this audience often wants to see something similar to Outlook. Plus, this markup is quite complicated and therefore interesting. Do not worry, you can do many other interfaces, but this is all somehow later.
At once I will make a reservation that we will not make a complete copy of the Outlook interface, we will confine ourselves to the most necessary.
')
To begin, create an empty ASP.NET application and name it "
OutlookInterfaceWithExtNet ".

Run "Package Manager Console (
Tools -> Library Package Manager -> Package Manager Console )". And type the following command "
PM -> Install-Package Ext.NET -Pre" . Using NuGet, we import Ext.NET and get almost the same application that was in the
last lesson.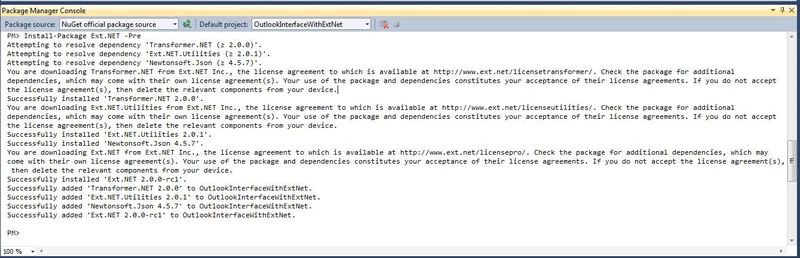
Let's correct “Web.config” not to add everywhere the link to the assembly with Ext.NET components.

Remove the file "Ext.NET.Default.aspx" from the project, in this project we will not need it.
ViewPort, BorderLayout and AccordionLayout
Now that all the preparations have been completed, we will copy the markup from the following example
http://examples.ext.net/#/ViewPort/Basic/Built_in_Markup/ to the new Default.aspx file. Remove the registration of the assembly, it is with us and is already registered in Web.config. We get the following markup:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title>Viewport with BorderLayout - Ext.NET Examples</title> </head> <body> <ext:ResourceManager runat="server" /> <ext:Viewport runat="server" Layout="BorderLayout"> <Items> <ext:Panel runat="server" Title="North" Region="North" Split="true" Height="150" BodyPadding="6" Html="North" Collapsible="true" /> <ext:Panel runat="server" Title="West" Region="West" Layout="AccordionLayout" Width="225" MinWidth="225" MaxWidth="400" Split="true" Collapsible="true"> <Items> <ext:Panel runat="server" Title="Navigation" Border="false" BodyPadding="6" Icon="FolderGo" Html="West" /> <ext:Panel runat="server" Title="Settings" Border="false" BodyPadding="6" Icon="FolderWrench" Html="Some settings in here" /> </Items> </ext:Panel> <ext:TabPanel runat="server" Region="Center"> <Items> <ext:Panel runat="server" Title="Center" Border="false" BodyPadding="6" Html="<h1>Viewport with BorderLayout</h1>" /> <ext:Panel runat="server" Title="Close Me" Closable="true" Border="false" BodyPadding="6" Html="Closeable Tab" /> </Items> </ext:TabPanel> <ext:Panel runat="server" Title="East" Region="East" Collapsible="true" Split="true" MinWidth="225" Width="225" Layout="Fit"> <Items> <ext:TabPanel runat="server" ActiveTabIndex="1" TabPosition="Bottom" Border="false"> <Items> <ext:Panel runat="server" Title="Tab 1" Border="false" BodyPadding="6" Html="East Tab 1" /> <ext:Panel runat="server" Title="Tab 2" Closable="true" Border="false" BodyPadding="6" Html="East Tab 2" /> </Items> </ext:TabPanel> </Items> </ext:Panel> <ext:Panel runat="server" Title="South" Region="South" Split="true" Collapsible="true" Height="150" BodyPadding="6" Html="South" /> </Items> </ext:Viewport> </body> </html>
We see on this page the following markup tree, the
important attributes are indicated in parentheses :
- Viewport
- Panel ( Region = "North" )
- Panel ( Layout = "AccordionLayout", Region = "West )
- TabPanel ( Region = "Center" )
- Panel ( Title = "Center" )
- Panel ( Title = "Close me" )
- Panel ( Region = "East" )
- TabPanel ( TabPosition = "Bottom" )
- Panel ( Title = "Tab 1" )
- Panel ( Title = "Tab 2" )
- Panel ( Region = "South" )
Before we begin to disassemble, let's see what is displayed in the browser. Make this page the default page and run. We will see the area marked up in 5 parts, and it occupies the entire area of ​​the page.
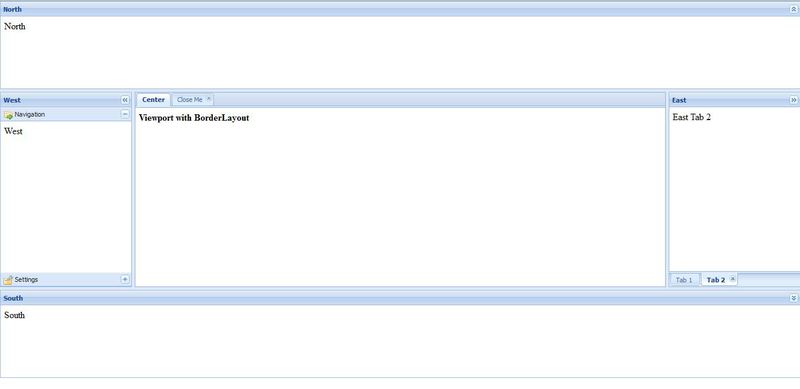
Now let's understand how it all happened. Let's start with the most important node - with ViewPort. Ext.NET has quite a few different containers. The main role is to place in it a certain number of other controls. All of them are inherited from the
AbstractContainer class and have a special Items collection, which contains child controls.
In our markup in the root of the tree, we see a special container, it is called "
ViewPort ", it has one interesting property that distinguishes it from other containers, it occupies the
entire accessible area on the page. You can change the size of the browser window in any way and the ViewPort will always stretch along with it, occupying all the available space. Therefore, it is convenient to use it as a base element in the markup. But it has one limitation, there can be
only one ViewPort on the page
, which is logical for itself, because you only have one browser window.
Now let's take a look at the
Layout attribute of the
ViewPort , which is set to "
border ". He has it for a reason and they are usually used together. Let's remove this attribute and see what happens.
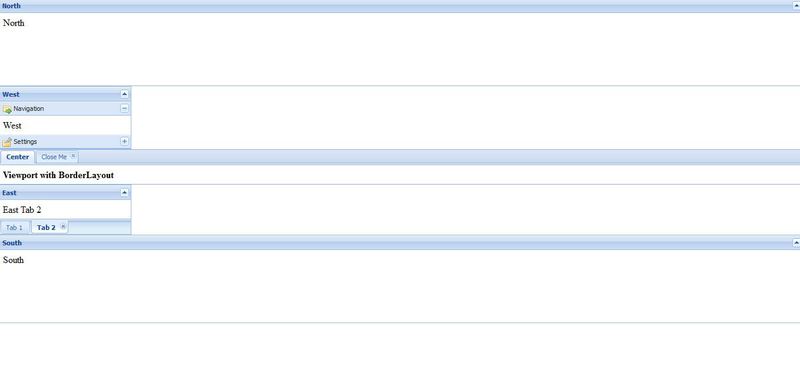
In principle, nothing terrible happened, only the Elements inside our ViewPort stood on top of each other. Typical behavior for block elements in HTML. The reason lies in the fact that the attribute
Layout (Layout) indicates the way the location of elements in the container. There is also
LayoutConfig , which performs fine-tuning markup. These properties are defined in the base class
"AbstractContainer" and all containers in Ext.NET inherit this property.
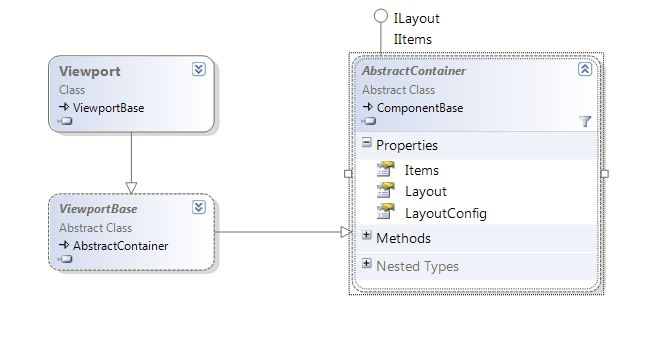
If you're wondering what other layouts are, you can look at
http://examples.ext.net in the Layout node. Now we will discuss only those that we need. You can read a good guide to markup, however, on ExtJS Pre,
http://rawberg.com/wp-content/uploads/ExtJS-Layouts.pdf . It details the main points of working with markup, which are also true for Ext.NET.
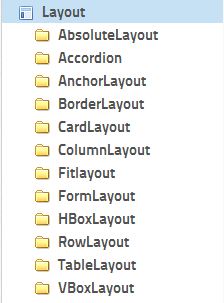
In our particular case, we use
BorderLayout , it places the child elements of the container, in our case, the
ViewPort, a maximum of five parts or at least one. To understand what principle it places them, let's look at the child elements of the ViewPort. It contains four Panel and one TabPanel and each has an attribute
" Region" , with a value from the set: "
North", "West", "Center", "East", "South" . As you probably already guessed, they point to 4 directions of the world and the central element, i.e. to place these elements inside the
ViewPort container. The markup "
Border " has the following rules:
- There must always be a child element with a Region = "Center" attribute . Therefore, there must be at least one element in this markup.
- This central element takes the space that remains after the allocation of space to all other elements involved in the markup.
In principle, there is nothing more difficult in
BorderLayout . Let's summarize a little:
- In Ext.NET, there are containers that inherit from AbstractContainer and contain a collection of child elements Items;
- Containers do not affect the location of elements; for this, the Layout and LayoutConfig properties are used;
- There is a special ViewPort container that occupies the entire accessible area in the browser window;
- There is a BorderLayout that allows placing its children in a container using the Region attribute.
I suppose that many people paid attention to the panel with the value Region = “West” and the title “West”, or rather, the value of its attribute Layout. Look at the display of its child elements. They are grouped in the so-called accordion. This is done using the value of the attribute already familiar to us - Layout = "accordion". In principle, this is nothing complicated, so we will not linger on
AccordionLayout .
Container, Panel, TabPanel
The Control
Panel is the main container in Ext.NET.
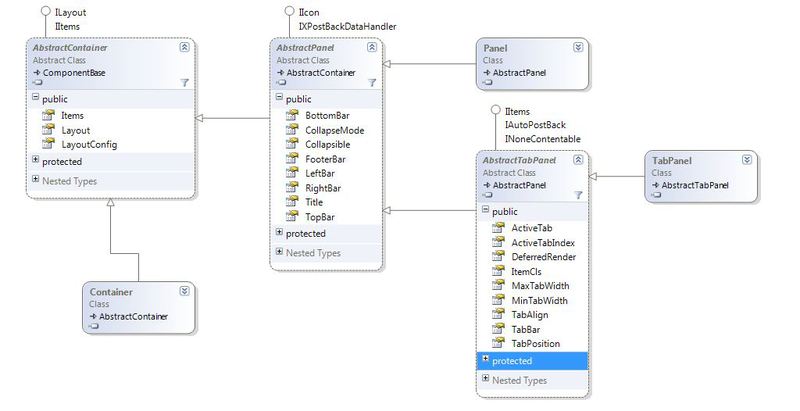
Consider the basic properties of the Panel class:
Property name
| Type of
| Inherited from
| Description
|
AnimCollapse
| bool
| AbstractPanel
| Indicates whether or not to minimize and collapse the panel. It makes sense only if the Collapsible property is true.
|
Bottombar
| Ext.Net.ToolbarCollection
| AbstractPanel
| A collection of items that will be displayed at the very bottom of the panel.
|
Closable
| bool
| AbstractPanel
| Can I close the panel
|
CollapseMode
| Ext.Net.CollapseMode
| AbstractPanel
| Determines how the panel minimizes button display. Takes one of the enum values ​​(Enum) CollapseMode: Default, Mini, Placeholder. It makes sense only if the Collapsible property is true. Default is 'Default'.
|
Collapsible
| bool
| AbstractPanel
| Indicates whether the panel can collapse. The default is 'false'.
|
Defaults
| Ext.Net.ParameterCollection
| AbstractContainer
| A set of parameters that will be added to each element of the container.
|
DefaultType
| String
| AbstractContainer
| Specifies the default xtype for children. The default is 'panel'. Xtype is a unique class identifier in ExtJS. Learn more docs.sencha.com/ext-js/4-1/# ! /Api/Ext.AbstractComponent-cfg-xtype
|
Footerbar
| Ext.Net.ToolbarCollection
| AbstractPanel
| The collection of items to be displayed at the bottom of the panel.
|
Items
| Ext.Net.ItemsCollection <AbstractComponent>
| AbstractContainer
| The set of components that will be displayed inside the container.
|
Layout
| String
| AbstractContainer
| Indicates the layout of elements in the container.
|
LayoutConfig
| Ext.Net.LayoutConfigCollection
| AbstractContainer
| Indicates the parameters / settings of the layout of elements in the container
|
Leftbar
| Ext.Net.ToolbarCollection
| AbstractPanel
| The collection of items to be displayed on the left side of the panel.
|
Rightbar
| Ext.Net.ToolbarCollection
| AbstractPanel
| The collection of items that will be displayed in the right pane.
|
Title
| String
| AbstractPanel
| Title bar. Default ''
|
Tools
| Ext.Net.ToolsCollection
| AbstractPanel
| A set of buttons that appear next to the title bar. Usually used to display the help buttons, minimize, close
|
Topbar
| Ext.Net.ToolbarCollection
| AbstractPanel
| The collection of items that will appear on top of the panel
|
To speed up client-side rendering, you can use
Container , a simpler implementation of AbstractContainer, instead of
Panel . Let's see what happens if we use Container, not Panel, as children of the ViewPort. The code we will use is the following:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head id="Head1" runat="server"> <title>Viewport with BorderLayout - Ext.NET Examples</title> </head> <body> <ext:ResourceManager ID="ResourceManager1" runat="server" /> <ext:Viewport ID="Viewport1" runat="server" Layout="BorderLayout"> <Items> <ext:Container ID="Panel1" runat="server" Title="North" Region="North" Split="true" Height="150" BodyPadding="6" Html="North" Collapsible="true" /> <ext:Container ID="Panel2" runat="server" Title="West" Region="West" Layout="AccordionLayout" Width="225" MinWidth="225" MaxWidth="400" Split="true" Collapsible="true"> <Items> <ext:Panel ID="Panel3" runat="server" Title="Navigation" Border="false" BodyPadding="6" Icon="FolderGo" Html="West" /> <ext:Panel ID="Panel4" runat="server" Title="Settings" Border="false" BodyPadding="6" Icon="FolderWrench" Html="Some settings in here" /> </Items> </ext:Container> <ext:TabPanel ID="TabPanel1" runat="server" Region="Center"> <Items> <ext:Container ID="Panel5" runat="server" Title="Center" Border="false" BodyPadding="6" Html="<h1>Viewport with BorderLayout</h1>" /> <ext:Container ID="Panel6" runat="server" Title="Close Me" Closable="true" Border="false" BodyPadding="6" Html="Closeable Tab" /> </Items> </ext:TabPanel> <ext:Container ID="Panel7" runat="server" Title="East" Region="East" Collapsible="true" Split="true" MinWidth="225" Width="225" Layout="Fit"> <Items> <ext:TabPanel ID="TabPanel2" runat="server" ActiveTabIndex="1" TabPosition="Bottom" Border="false"> <Items> <ext:Container ID="Panel8" runat="server" Title="Tab 1" Border="false" BodyPadding="6" Html="East Tab 1" /> <ext:Container ID="Panel9" runat="server" Title="Tab 2" Closable="true" Border="false" BodyPadding="6" Html="East Tab 2" /> </Items> </ext:TabPanel> </Items> </ext:Container> <ext:Container ID="Panel10" runat="server" Title="South" Region="South" Split="true" Collapsible="true" Height="150" BodyPadding="6" Html="South" /> </Items> </ext:Viewport> </body> </html>
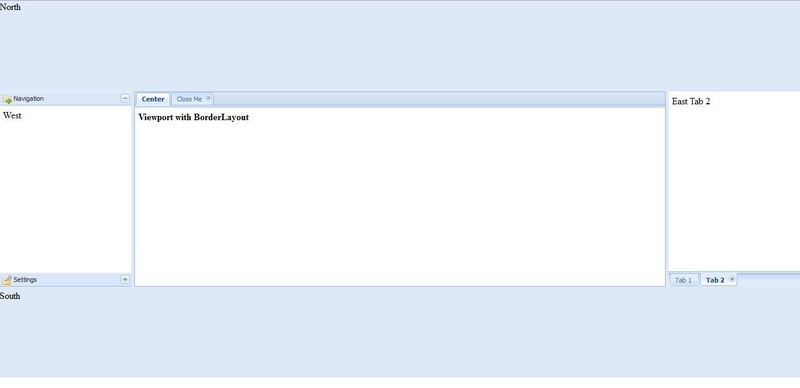
The dimensions are preserved and the contents of the elements remain. Purely visually, we lost only the headings of the panels, the border and the background became transparent. A small button was also lost, which allowed the panel to collapse to increase the space for the central element, its presence was ensured by the value of the following attribute -
Collapsible = "true". But this attribute is not supported in the
Container and we are deprived of this functionality.
Let's consider the results of the profiler in both cases - in the case of using the Panel and in the case of using the Container.
Profiling results using Container instead of Panel in ViewPort
Profiling results using Panel in ViewPortAs can be seen from the results, replacing
only 4 Panel to Container , reduced the time for rendering by more than 30ms
(494 ms instead of 530ms) and the number of calls to JavaScript functions, by 7000
(57236 instead of 64381) . Which is a pretty good result, considering that usually in Ext.NET applications several hundred panels are used. But if you are not sure about the gains and advantages of using Container instead of Panel, I recommend using Panel. Often this does not bring a fundamental gain in speed, because browsers are running faster, the power of client machines too, and this can add many times more potential problems. We will not save and leave the Panel.
The next container we meet is the “
TabPanel” tab bar. The control is a container for the Panel and each one is a tab. The value of the title is indicated in the "
Title " attribute of the container, and if you want the tab to be closed, then pay attention to the "
Closable " attribute. TabPanel in its implementation uses another interesting Layout - CardLayout. We will not dwell on it in detail. In short, it presents the child elements of the container as a stack of cards and displays only the top one to the user. Those. the user at a time is displayed only one element from its set of child elements. To understand, you can look at the
following example.Consider the basic properties of the TabPanel class (since TabPanel is a descendant of AbstractPanel, then all the properties described for the Panel are valid for it, but some will not work because they do not make sense):
Property name
| Type of
| Inherited from
| Description
|
ActiveTab
| Ext.Net.AbstractComponent
| AbstractTabPanel
| ID, index or directly tab, which will be displayed by default. Useful if you need the TabPanel to open, say 2, the tab
|
ActiveTabIndex
| int
| AbstractTabPanel
| Default tab index
|
DeferredRender
| bool
| AbstractTabPanel
| If True , tab elements will not be loaded into the browser until the tab is active or visible. This allows you to speed up performance with a large number of elements in the tabs. If False, the elements of all tabs will be loaded immediately, often reducing performance . Used in rare cases: when there are problems with the display of controls or when the user is annoyed with the delay when clicking on the tab. Default is true
|
ItemCls
| String
| AbstractTabPanel
| CSS class to be added to each tab. Default "x-tabpanel-child"
|
MaxTabWidth
| int
| AbstractTabPanel
| Max tab width
|
MinTabWidth
| int
| AbstractTabPanel
| Minimum tab width
|
TabAlign
| Ext.Net.TabAlign
| AbstractTabPanel
| Direction tabs. Either right to left (Ext.Net.TabAlign.Left), or from left to right (Ext.Net.TabAlign.Left). Ext.Net.TabAlign.Left default
|
TabPosition
| Ext.Net.TabPosition
| AbstractTabPanel
| Position of tab headers. Either on top (Ext.Net.TabPosition.Top) or (Ext.Net.TabPosition.Bottom). Ext.Net.TabPosition.Top default
|
Create basic basic markup
Now that we have the knowledge we have gained, we can start creating the most basic markup of our application. It will not be very much, but because we are just starting. All panels of our ViewPort remained in place, but in accordance with our requirements, they received due design. Let's look at the markup, which turned out in the end, you can insert it into Default.aspx and work with it yourself.
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head id="Head1" runat="server"> <title>«a la Outlook» , 1</title> </head> <body> <ext:ResourceManager ID="PageResourceManager" runat="server" /> <ext:Viewport ID="AppMainViewport" runat="server" Layout="BorderLayout"> <Items> <ext:Panel ID="MenuPanel" runat="server" Title="" Region="North" Height="100" Html=" " Collapsible="true"> <Tools> <ext:Tool Type="Help" runat="server"></ext:Tool> </Tools> </ext:Panel> <ext:Panel ID="LeftSideNavPanel" runat="server" Title="" Region="West" Layout="AccordionLayout" Width="225" MinWidth="225" MaxWidth="400" Split="true" Collapsible="true" CollapseMode="Placeholder"> <Items> <ext:Panel ID="MailLeftSideNavPanel" runat="server" Title="" Border="false" BodyPadding="6" Icon="Email" Html=" " /> <ext:Panel ID="CalendarLeftSideNavPanel" runat="server" Title="" Border="false" BodyPadding="6" Icon="Calendar" Html=" " /> <ext:Panel ID="ContactsLeftSideNavPanel" runat="server" Title="" Border="false" BodyPadding="6" Icon="FolderUser" Html=" " /> <ext:Panel ID="TasksLeftSideNavPanel" runat="server" Title="" Border="false" BodyPadding="6" Icon="Tick" Html=" " /> </Items> </ext:Panel> <ext:Panel ID="MainMailPanel" runat="server" Region="Center" Html=" "> </ext:Panel> <ext:Panel ID="TasksRightSidePanel" runat="server" Title=" " Region="East" Collapsible="true" CollapseMode="Placeholder" Split="true" MinWidth="225" Width="225" Html=" "> </ext:Panel> <ext:Panel ID="AppMainStatusPanel" runat="server" Region="South"> <BottomBar> <ext:StatusBar ID="AppMainStatusBar" runat="server" DefaultText=" "> </ext:StatusBar> </BottomBar> </ext:Panel> </Items> </ext:Viewport> </body> </html>
And if we run it, we get the following view of our main page:

There is nothing complicated in the markup, these are all components familiar to us. However, if you have any questions, please contact us.
On this we dwell. The foundation of our application is laid, although it is usually the same for all applications. In the next part we will take one more step - we will make the top menu (Ribbon) and in the process we will deal with Toobar, Button, SplitButton and other interesting components.
The project can be downloaded
here.Thank you for your attention and enjoy reading!