Introduction
Habra already has a couple of tutorials on the topic of cross-platform 3D engine “Unity 3D”, but still does not have lessons on the topic of 3D menus.
On this article I pushed the post
Unity3d script basics and
Unity3D for beginners - Tutorial 1 .
Also, a couple of people blamed the author on the topic “We are not living in that century”, so here you have a pinch of knowledge.
While doing this tutorial, I assumed that you were already familiar with the basics of JS and Unity 3D interface.
I will work with Vindusovskoy version of Unity 3D. Owners of other operating systems I think will understand.
Cooking
1) Have a basic idea on the menu, so to speak, mental preparation.
2) The models you need. You can find it
here (Google Sketchup). The format we need is collada.
3) Have a font for the menu text. You can take the standard of the fonts of Windows.
The first steps
I came up with a scene on “Western” themes.
Let's start with the cooking scene.
First create a terrain and select the size / height / quality of texturing (Terrain-> Set Resolution / Flatten Heightmap).
Next, select the texture and make the main landscape of the scene.
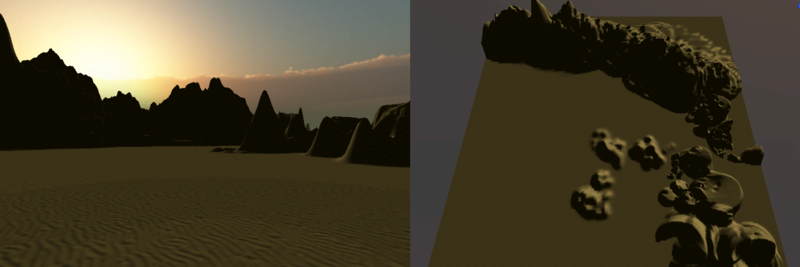
')
Then put the camera under the desired viewing angle, add light (Derectional light) and shadow it to it.
Add fog and sky (skybox), all this can be found in Edit-> Render Settings.
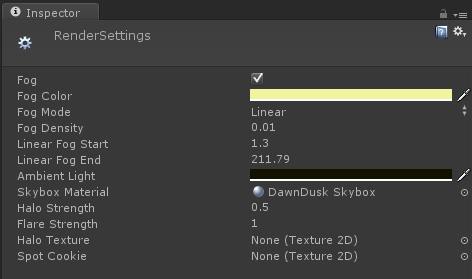
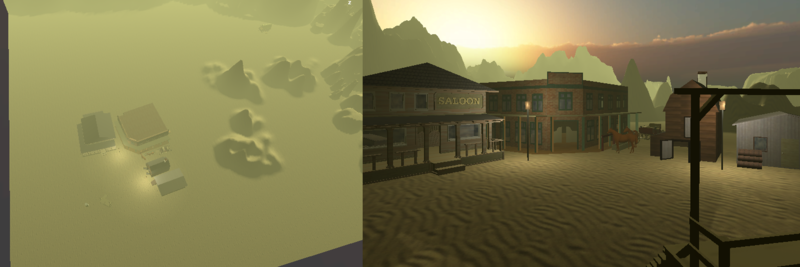
Models
First, unzip the downloaded archive, and transfer all the textures from the images to the Materials folder of your project (just select and drag on the folder in the unity window).
Next, import the model * .dae into Unity (Assets-> Import New Asset), and add it to the scene, just change the size to your liking.
IMPORTANT! If you do out of order, you will get an untextured model.
Menu Basis
The menu can be made with the help of models or 3D text, and it is possible from all at once.
I will talk about the text version, because it will be more awesome.
To create a 3D text with your own font, you need to add it to the project (Assets-> Import New Asset).
After selecting it, go to (GameObjects-> Create Other-> 3D Text).
You will create a soap text. To improve quality, change the Font Size, and then adjust the size.
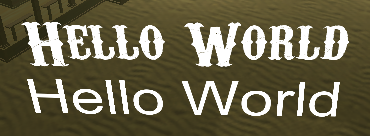
Further we will make it functional. To do this, copy the following code on JS'e and apply to all objects "3D text".
function OnMouseDown () { //1 if(name=="Play Game") { Application.LoadLevel("Test Scene"); } //2 if(name=="Options") { } if(name=="Quit") { Application.Quit(); } //3 } function OnMouseOver () { //1 animation.Play(); //4 }
1) Events with the manipulation of the mouse.
2) Load a scene called Test Scene, if you specify it when compiling the project (you can also specify the sequence number).
3) Exit the application if it is not compiled under the web, or does not run in the editor.
4) The method of animation menu, if you want one (Too dreary, so I will not tell. You can see in my finished project).
* name - the name of the object on which the script rests.
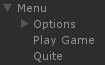
Menu navigation
Create a cylinder, and rename it to Menu. Make it transparent by applying any partially transparent material (as shown on the screenshot).

Let's put all our 3D text objects into the Menu object (Hierarchy window).
Also on the other side of the cylinder, create two 3D texts, and move them along the hierarchy to Menu. This will be a sub menu item.
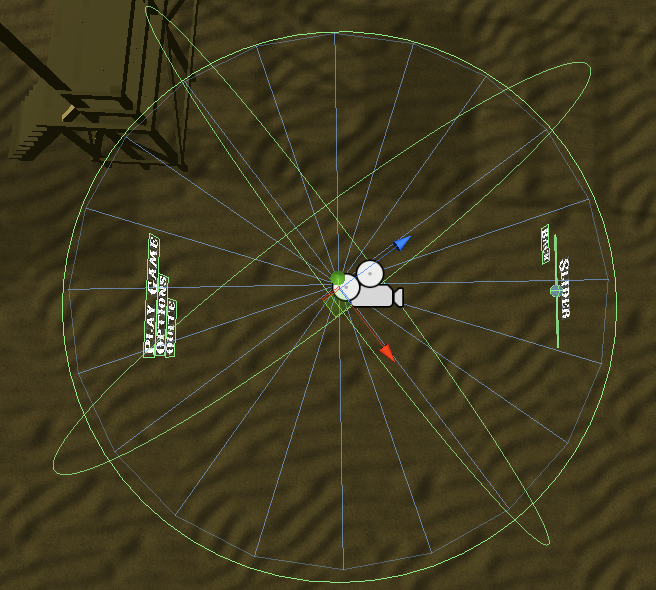
Update the script.
var menuRotation : GameObject; //1 function OnMouseDown () { if(name=="Play Game") { Application.LoadLevel("Test Scene"); } if(name=="Options" || name=="Back" ) { menuRotation.transform.Rotate(0, 180, 0); } //2 if(name=="Quit") { Application.Quit(); } } function OnMouseOver () { animation.Play(); }
1) Create a variable containing the object.
2) When you click on an object with the name Options, the command will rotate the menuRotation object by 180 *.
Move the cylinder in the Menu Rotation field of the Options button.
Now you have an almost finished menu. It remains only to create a slider.
Sliders
Sliders - the most dreary part of the lesson. Get ready for suffering and shamanism.
* I would be glad if someone posted their version of the slider, because my actually shamanic.
Create a noticeable Cube and stretch it to the similarity of the thread. Create a spotted Sphere and place it in the center of the Cube.
All this is located opposite the main menu, namely the Options submenu.
* For convenience, I renamed them to line_slider and sphere_slider.
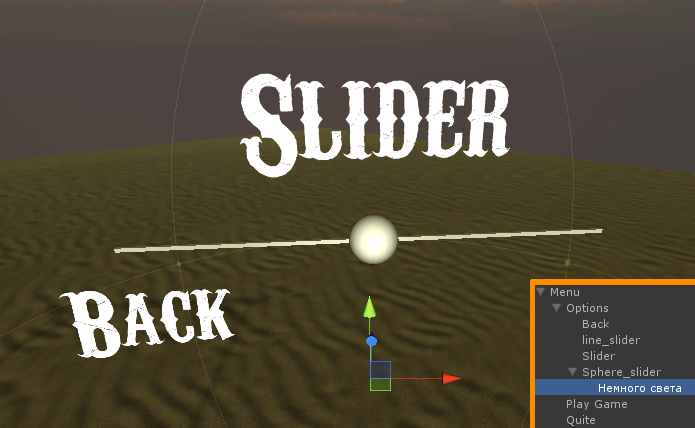
Create a new JS script and hook it to the Sphere (sphere_slider).
function OnMouseDrag () { //1 var translation : float = Input.GetAxis ("Mouse X"); //2 transform.Translate(translation, 0, 0); //3 print(transform.position.x.ToString()); //4 }
1) The event will be activated when you click (on Sphere / sphere_slider) and move the mouse.
2) Create a translation variable to which the X coordinates of the mouse are passed.
3) Move the Sphere / sphere_slider over the mouse.
ATTENTION. The coordinate along which the ball will move is different for everyone (it’s easiest to turn it with the X / red arrow along the movement path)
4) The string transform.position.x will give us the coordinate on which the object is currently located.
ATTENTION. The coordinate is still different (transform.position.x || y || z; or the Sphere / sphere_slider rotation).
According to the output in the console, we define the minimum and maximum on which the future slider will move.
As soon as you spot the necessary coordinates, update the old script.
function OnMouseDrag () { var translation : float = Input.GetAxis ("Mouse X") * 0.18; //1 if (transform.position.x < min || transform.position.x > max) { //2 if (transform.position.x < min) { transform.Translate(0.1, 0, 0); } //3 if (transform.position.x > max) { transform.Translate(-0.1, 0, 0); } //3 } else { transform.Translate(translation, 0, 0); } }
1) Still, we catch the X coordinate, but with a coefficient that lowers \ increases the speed of the ball.
ATTENTION! It is also unique for each user.
2) The maximum and minimum movement of the slider along the axes.
3) When going beyond the limits of the coordinate, it slightly decreases (in order to avoid the slider getting stuck in place).
* we change min and max for the limits received earlier.
Here the slider is almost ready. It remains only to teach him to return the value.
var bullet : float = 0; //1 function OnMouseDrag () { var translation : float = Input.GetAxis ("Mouse X") * 0.18; if (transform.position.x < min || transform.position.x > max) { //2 if (transform.position.x < min) { transform.Translate(0.1, 0, 0); } //3 if (transform.position.x > max) { transform.Translate(-0.1, 0, 0); } //3 } else { transform.Translate(translation, 0, 0); } bullet = (transform.position.x - min)*250;//2 }
1) Create a bullet variable.
2) Writes the slider values to the bullet variable.
That's all. Now you have a more or less functioning Unity 3D menu.
What happened with me -
tyk .
Thank you for reading the lesson.