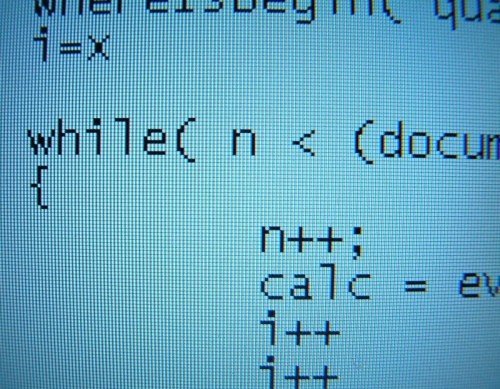
On the hub, several topics appeared about the one-liners in different languages that solved simple problems. I decided to publish several algorithms in C / C ++.
So let's go!
1. The Euclidean Algorithm
Recursive algorithm for finding the greatest common divisor.
int GCD(int a,int b) { return b?GCD(b,a%b):a; }
2. Finding the NOC
Recursive algorithm for finding the least common multiple.
int LCM(int a,int b) { return a/GCD(a,b) * b; }
3. Checking the number 2 ^ n
Algorithm for checking the number of degree 2.
int isPow2(int a) { return !(a&(a-1)); }
')
4. The function of the exchange of two variables
This algorithm works by using the symmetric distinction property that XOR possesses and does not require the presence of a third variable.
void swap(int *a, int *b) { *a ^= (*b ^= (*a ^= *b)); }
5. The exponential algorithm
The power of a number in linear time.
End of recursion condition: if the power of a number is 0, then a ^ 0 = 1.
int pow(int a,int n) { return (!n)?1:a*pow(a,n-1); }
6. Indian exponentiation algorithm
The power of the number in logarithmic time.
int powInd(int a,int n) { return (!n)?1:((n&1)?a:1)*powInd(a*a,n/2); }
7. Factor numbers
The factorial of a nonnegative integer n.
Continuation of recursion condition: factorial is the product of all natural numbers up to n inclusive.
End of recursion condition: if the number is 0, then 0! = 1.
int fac(int n) { return n?n*fac(n-1):1; }
8. Sum of digits
The condition for the continuation of recursion: the sum of digits of a number is equal to the last digit plus the sum of digits of a number without the last digit.
End of recursion condition: if the number is 0, then the sum of digits is 0.
int count(int a) { return (!a)?0:(a%10+count(a/10)); }
9. Fibonacci numbers
Fibonacci numbers are elements of a numerical sequence in which each successive number is equal to the sum of the two previous numbers.
int fib(int n) { return (n<=2)?1:(fib(n-1)+fib(n-2)); }
10. The next Fibonacci number
The function of finding the Fibonacci numbers.
int fibNext(int &f1,int &f2) { return f1=(f2+=f1)-f1; }
11. Mersenne numbers
Mersenne numbers - numbers of the form

int Mersen(int n) { return !(n&(n+1)); }
12. Min & Max
int max(int a,int b) { return (a>b)?a:b; } int min(int a,int b) { return (a>b)?b:a; }
13. Comparing two numbers
The function returns the difference between two numbers, so if the difference is greater than 0, then the number a is greater than b, if it is equal to 0, then the numbers are the same, otherwise the number a is smaller than b.
template <typename TYPE> int compare (const TYPE a, const TYPE b){ return ( a - b ); }
14. Raising the number 2 to the power n
With the help of the shift of unit by n bits, we calculate the two to the power n.
int pow2(int n) { return 1<<n; }
One-liners from comments
Finding the NOC from Lertmind
int lcm(int a, int b) { return (b < 1 ? (b ? lcm(b, a % b) : a) : (a / -lcm(-b, -a % b) * b)); }
The number of nonzero bits in the number of Mrrl
int NBit(unsigned int x){ return x==0 ? 0 : (x&1)+NBit(x>>1); }
The maximum degree of two, which divides n from Mrrl
int MaxDivPow2(int n){ return n&-n; }
Compare two numbers from Lertmind
int cmp(int a, int b) { return (a < b ? -1 : (a > b ? 1 : 0)); }
or pattern
template<typename T> int cmp(const T &a, const T &b) { return (a < b ? -1 : a > b); }
Find the k-th bit in the int * array (assuming sizeof (int) == 4) from Mrrl
int GetBit(int *a,int k){ return (a[k>>5]>>(k&31))&1; }
Waiting for more algorithms in comments ...