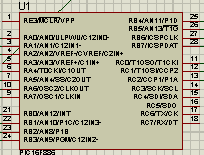
This article is aimed at beginners in learning programming family pic-controller based on the language of assembler. I took the pic16f886 microcontroller as a basis. For programming and modeling,
MPlab IDE (Microchip) and
Proteus (Labcenter) programs were used, respectively.
Briefly about the essence of the project
This project allows you to play
stone, scissors, paper for people with disabilities (Partial paralysis, the absence of one or several fingers or hands) The idea belongs to
meequz , my implementation
Project structure
Each player has 3 buttons at his disposal, with their corresponding inscriptions (stone, scissors, paper). The result of the selection and the score is displayed on a seven-segment board. The score goes to 9 points. Start a new game by pressing the button "Reset"
')
Pic controller programming
I repeat once again that for programming we will use a low-level machine-oriented language - assembly language
To begin, let us announce which microcontroller we use and reserve the general-purpose registers that we will need in the future:
LIST P=16f886
#include <p16f886.inc>
CBLOCK 0x20
IGROK_1
IGROK_2
KAMEN
NOJNITSI
BUMAGA
SCHET_1
SCHET_2
Reg_1
Reg_2
Reg_3
NINE
ENDC
Next, start the program itself and configure the input / output ports:
start ;
bsf STATUS, RP1 ; 3
bsf STATUS, RP0
clrf ANSEL ; \
clrf ANSELH
BCF STATUS, RP1 ; 1
BSF STATUS, RP0
movlw b'11100111' ; RA0,RA1,RA2,RA7,RA6,RA5 ,
movwf TRISA
movlw b'00000000' ;
movwf TRISB
movlw b'00000001' ; RC0 ,
movwf TRISC
bcf STATUS,RP0 ; 0
bcf STATUS,RP1
CLRF PORTA ;
CLRF PORTB
CLRF PORTC
movlw b'00000001'
movwf KAMEN
movlw b'00000010'
movwf NOJNITSI
movlw b'00000100'
movwf BUMAGA
movlw .9
movwf NINE
clrf IGROK_1
clrf IGROK_2
clrf SCHET_1
clrf SCHET_2
clrf W
bcf INTCON,6 ;
bcf INTCON,7
Commands:
movlw - sends a constant to the battery.
movwf - sends the contents of the battery to the register
bcf - clears the bit in the register
bsf - sets the bit in the register
clrf - clears the register
btfss - checks the bit in the register, skips the next command if the result is 1
btfsc - checks the bit in the register, skips the next command if the result is 0
The main cycle of the program. It checks the button press on both controllers:
GAME ;
BTFSS PORTA,0
CALL PRE_KANMEN_1
BTFSS PORTA,5
CALL PRE_KANMEN_2
BTFSS PORTA,1
CALL PRE_NOJNITSI_1
BTFSS PORTA,6
CALL PRE_NOJNITSI_2
BTFSS PORTA,2
CALL PRE_BUMAGA_1
BTFSS PORTA,7
CALL PRE_BUMAGA_2
GOTO PROVERKA
VOZVRAT ;
GOTO GAME
PROVERKA subroutine checks if element 1 was selected by player 1:
PROVERKA ;
BTFSC IGROK_1,0 ;
GOTO ONE
BTFSC IGROK_1,1 ;
GOTO TWO
BTFSC IGROK_1,2 ;
GOTO THREE
GOTO VOZVRAT
When a player presses a button, a transition to a certain subroutine occurs (for example, imagine that player 1 chose a stone):
PRE_KAMEN_1 ;
MOVLW .1
CALL KOD
MOVWF PORTB
BSF PORTA,3
MOVFW KAMEN
MOVWF IGROK_1
CLRF W
call delay
BCF PORTA,3
RETURN
The selected item is written to the register IGROK_1
After selecting player 1, it checks whether the selection of the element by player 2 and simultaneously compares the results of the selection. After comparing it goes to 1 of 3 subroutines:
1) The first player won
2) The second player won
3) Draw
ONE ;
BTFSC IGROK_2,0
GOTO NICHA
BTFSC IGROK_2,1
GOTO FIRST_WIN
BTFSC IGROK_2,2
GOTO SECOND_WIN
GOTO VOZVRAT
TWO ;
BTFSC IGROK_2,0
GOTO SECOND_WIN
BTFSC IGROK_2,1
GOTO NICHA
BTFSC IGROK_2,2
GOTO FIRST_WIN
GOTO VOZVRAT
THREE ;
BTFSC IGROK_2,0
GOTO FIRST_WIN
BTFSC IGROK_2,1
GOTO SECOND_WIN
BTFSC IGROK_2,2
GOTO NICHA
GOTO VOZVRAT
In order not to paint all 3 subroutines, imagine that the first player won:
FIRST_WIN ;
incf SCHET_1 ; 1
FIRST_W ;
MOVFW SCHET_1
CALL KOD2
MOVWF PORTB
BSF PORTC,1
NOP ;
NOP
NOP
NOP
NOP
BCF PORTC,1
MOVFW SCHET_2
CALL KOD2
MOVWF PORTB
BSF PORTC,2
NOP
NOP
NOP
NOP
NOP
BCF PORTC,2
BTFSC PORTC,0
GOTO FIRST_W
PROV ;
BTFSC PORTC,0 ; ""
GOTO PRED_GAME
GOTO PROV
The elements are displayed using the KOD routine:
KOD
addwf PCL,1;
retlw b'11111111';
retlw b'10100011'; ''
retlw b'11001001'; ''
retlw b'11000000'; ''
A rock -

Scissors -

Paper -

Numbers are displayed using the KOD2 subroutine:
KOD2
addwf PCL,1;
retlw b'11000000'; '0'
retlw b'11111001'; '1'
retlw b'10100100'; '2'
retlw b'10110000'; '3'
retlw b'10011001'; '4'
retlw b'10010010'; '5'
retlw b'10000010'; '6'
retlw b'11111000'; '7'
retlw b'10000000'; '8'
retlw b'10010000'; '9'
The final step of the program is to clear the registers IGROK_1 and IGROK_2, and check the account overflow:
PRED_GAME ;
CLRF IGROK_1
CLRF IGROK_2
BCF STATUS,2
MOVFW SCHET_1
SUBWF NINE,0
BTFSC STATUS,2
GOTO CLEAR
BCF STATUS,2
MOVFW SCHET_2
SUBWF NINE,0
BTFSC STATUS,2
GOTO CLEAR
GOTO GAME
CLEAR ;
CLRF SCHET_1
CLRF SCHET_2
GOTO GAME
Conclusion
The scheme of this project is compiled in the Proteus program:
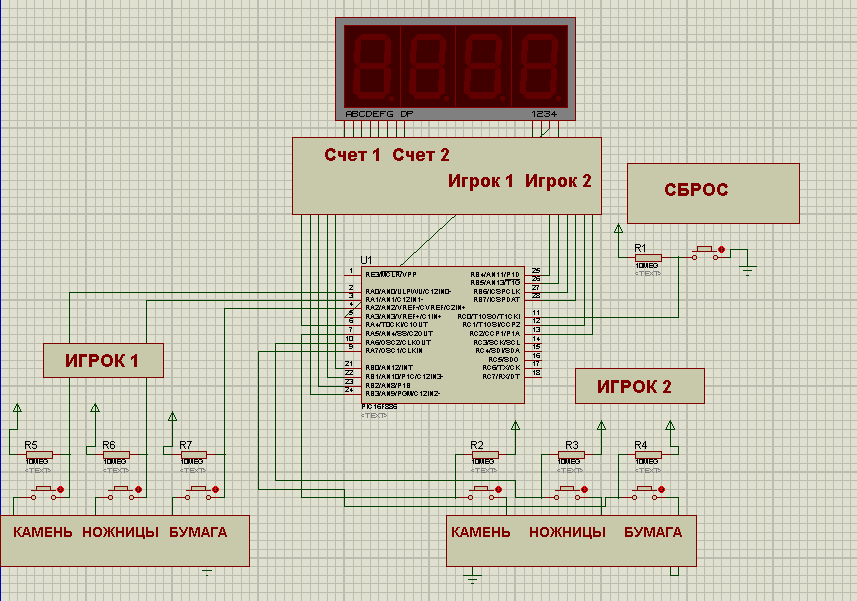
************************************************** ************************
For convenience, an
.asm file ,
.hex file, and
shemku in proteus