Hi, Habr. There are a number of tasks for which there is no need to use the Xcode GUI. The execution time of such tasks can be reduced at least by the time Xcode is started. Saving time may seem insignificant when working with one project, but if there are many projects, editing them can be very tedious. But the most important thing is that this approach opens up possibilities for automating work with projects.
I want to talk about a new tool whose goal is to solve the problem described. XcodeProject is a Ruby library that can be used to read and modify Xcode project data, as well as to build projects, archive and perform a number of automation tasks.
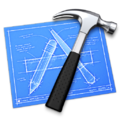
')
Installation
The library is a gem, its installation can be performed from the terminal with the following command:
gem install xcodeproject
Beginning of work
The simplest example of using a library that displays all the goals of a project:
require 'rubygems' require 'xcodeproject' proj = XcodeProject::Project.new('path/to/example.xcodeproj') proj.read.targets.each do |target| puts target.name end
First you need to create an object of type XcodeProject :: Project:
proj = XcodeProject::Project.new('path/to/example.xcodeproj')
Or you can find all Xcode projects in a given directory:
projs = XcodeProject::Project.find('path/to/dir')
Or according to a given pattern:
projs = XcodeProject::Project.find('*/**')
After the project object is created, you can read the data:
data = proj.read p data.target('example').config('Release').build_settings
Or change the data:
proj.change do |data| data.target('example').config('Release').build_settings['GCC_VERSION'] = 'com.apple.compilers.llvmgcc42' end
Files, groups and directories
Display all groups of the highest level:
data.main_group.children.each do |child| p child.name end
Display only files from the specified group:
group = data.group('path/from/main_group') group.files.each do |file| p file.name end
At any time you can get GroupPath groups. GroupPath is the path to the group relative to the project root (the project root is the Main Group, a hidden group that is the parent of all project groups) or the current group.
group.group_path
Directories are groups that are represented on your file system. For them, you can get FilePath - the real path in the file system to the directory with which the object is associated.
group.total_path
You can add a group to the project by specifying GroupPath to it, relative to the project root:
data.add_group('path/from/main_group')
Or relative to the current group:
group.add_group('path/from/current_group')
To add a directory to a project, you must specify FilePath:
data.add_dir('group_path/to/parent', '/file_path/to/dir') group.add_dir('/file_path/to/dir')
You can add a file to the project in the same way:
data.add_file('group_path/to/parent', '/file_path/to/file') group.add_file('/file_path/to/file')
You can also delete files and groups from the project:
data.remove_file('path/from/main_group') data.remove_group('path/from/main_group') group.remove_file('path/from/current_group') group.remove_group('path/from/current_group')
Goals
Getting the target object is simple:
target = data.target('example')
After the file is added to the project, you can use it to build a target:
file = main_group.add_file('/file_path/to/file') target.add_source(file)
You can exclude the file from the assembly as follows:
target.remove_source(file)
Build proct
XcodeProject uses XcodeBuilder to build projects.
First you need to create a rakefile. In the simplest case, it might look like this:
require 'rubygems' require 'xcodeproject' proj = XcodeProject::Project.new('path/to/example.xcodeproj') XcodeProject::Tasks::BuildTask.new(proj)
A number of tasks are now available, including building the project. A complete list of tasks can be obtained by running rake -T.
$ rake -T rake example:archive
Modify build options:
XcodeProject::Tasks::BuildTask.new(proj) do |t| t.target = "libexample" t.configuration = "Release" end
Get more information on XcodeBuilder can be on the
link .
Finally, a few words about the XcodeProject project. The project is under development and has only a small functionality presented in Xcode. The emergence of new features will be associated with real portebnostyami in them. The project is available under the MIT license, you can use the project code as you wish. Any help in the development of the project is welcome.
Project address on
github .