I recently wrote that Greensock, an excellent library for scripting animation in Flash,
now supports JavaScript . In this article I will demonstrate the basics of working with GSAP v12 (beta). The result will be something like this:
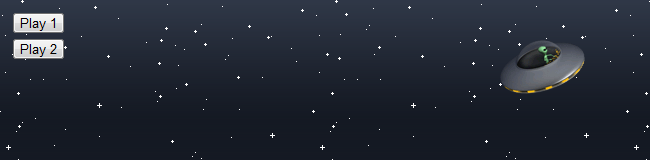
At once I will make a reservation that I took the graphics and idea for an example from the
documentation $ fx () , because which of us will refuse
to unload a UFO? :)
Scripts
First connect the necessary scripts:
< script type ="text/javascript" src ="js/jquery.min.js" ></ script ><br>< script type ="text/javascript" src ="js/TweenMax.min.js" ></ script ><br>< script type ="text/javascript" src ="js/TimelineMax.min.js" ></ script ><br>< script type ="text/javascript" src ="js/easing/EasePack.min.js" ></ script ><br>< script type ="text/javascript" src ="js/plugins/CSSPlugin.min.js" ></ script >
')
Why so much?
- JQuery we need in order to select elements. You can, of course, without him.
- TweenMax (60 Kb) is the main library of animation. There is also TweenLite (21 Kb), which includes only basic features.
- TimelineMax (16 Kb) is a timeline based library. TimelineLite weighs 9 Kb.
- EasePack (5 Kb) is a set of animation effects that control its character, for example, a smooth slowdown or “elastic” trembling.
- CSSPlugin (14 Kb) - allows you to animate CSS-properties of HTML-objects, both one by one and in whole
packs of classes.
Animation
We can begin to animate. The basic syntax is:
- TweenMax. from (object, duration, value) - from the specified value to the current
- TweenMax. to (object, duration, value) - from the current value to the specified
- TweenMax. fromTo (object, duration, value1, value2 ) - from the specified value to the specified value (in order not to be mistaken :)
An object can be any DOM element. The easiest way is to get it through the jQuery selector. The time is indicated in seconds. The value is specified as an object, for example, {value: 500} or {width: 300}. Convenient chip: if you take the value in quotes, the change will be relative, not absolute. Clearly:
TweenMax.to( $( '#sample' ), 1, {value: 300} ); // 1 300 <br>TweenMax.to( $( '#sample' ), 1, {value: '300' } ); // 1 300
Compare both options in the demo 1Animation of CSS properties is a bit more complicated (the main thing is not to get confused in curly braces):
TweenMax.to( $( '#bg1' ), 2, {css: {background-position: '-300px' }} );
You can specify properties one at a time, or you can specify a whole class. Then GSAP will compare both classes (current and specified), find the different properties and work them all. As usual, the price of convenience is the speed of execution.
Additionally, you can specify the number of repetitions of the animation (-1 means infinite repetition):
TweenMax.to($( '#bg1' ), 2, {css: {background-position: '-300px' }}, repeat: -1)
By default, all animations occur with a beautiful slowdown at the end. If another effect (or no) is required, this must be explicitly indicated:
TweenMax.to($( '#ufo' ), 2, {width: 300}, ease: Linear.easeNone)
Let's use the obtained knowledge and make an infinitely animated background with
blackjack and parallax:
TweenMax.to($( '#bg1' ), 9, {css:{backgroundPosition: "-314 0px" }, repeat:-1, ease:Linear.easeNone});<br>TweenMax.to($( '#bg2' ), 18, {css:{backgroundPosition: "-269 30px" }, repeat:-1, ease:Linear.easeNone});
View demo 2Sprites
With the help of sprite animation we turn on the side lights on the UFO. Our sprite consists of 4 frames:

Especially for this purpose was invented SteppedEase, animating not smoothly, but on the contrary - in spurts. Having set the number of jerks equal to the number of frames, we get a beautiful animation:
TweenMax.to($( '#ufo' ), 0.2, {css:{backgroundPosition : "340 0px" }, ease:SteppedEase.config(4), repeat:-1});
View demo 3Timeline
I will tell you in the second part of the article about how to combine animations in chains and manage the resulting sequences, but for now I will try to answer the questions of those present.
* All code examples are highlighted using Source Code Highlighter .