Suppose there is a task to make a form for filtering goods on a site of the following form:
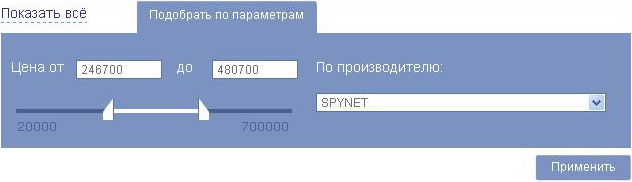
The site is managed by Umi.CMS, the required product catalog has been created, all properties of the goods are filled. It is necessary to cross-shape the form and make it work. There are no difficulties with the latter; tools necessary for filtering are present in Umi. but
Layout and programming of sliders to select a range from time to time causes a lot of trouble.
')
A good form of range selection should satisfy the following conditions:
1) The boundaries of the range should be managed by the project administrator. Prices are rising, everything is changing.
2) When crossing the sliders at the moment of dragging, the range should remain manageable.
3) Sliders should interact with text fields for entering values.
Adobe Flash has been selected as a suitable tool for implementing animation of the range sliders, which provides an excellent opportunity to draw the missing interface elements. And the possibilities of ActionScript and especially the package
external classes allow you to do what you need: make the sliders move predictably and organize data transfer for JavaScript and back in order to organize the normal operation of the form.
So, to make a working form of filtering based on the price range, you should go through the following three steps:
1) Preparing a swf file with sliders animation.
The interface for the sliders selection form will consist of the following components:
- Clips sliders: left and right. When preparing clips, it is desirable to take into account that when crossing them in a common part of the range, the left slider takes the form of the right and vice versa, which will preserve the integrity of the perception of the form. You can make two clips for the left and right slider with opposite shapes on different frames, or experiment with the display of one clip.
- The clips of the line on which the sliders are located: the lower main and upper, which will display the selected range.
- Text fields displaying the range boundaries defined in the administrative panel.
- Clip background. Any.
After creating and placing all the necessary components, we will prepare a file with the ActionScript class to organize its work. To move the sliders within the desired range, you should get some data:
The minimum and maximum limits of the range, the minimum and maximum values selected by the user (if the user has not chosen anything yet, they are equal to the limits of the range), the status of the selection indicates whether the user has selected the range or not yet.
This data allows you to get a package of classes ActionScript external. The ExternalInterface class allows you to register the methods necessary for exchanging data between a swf file and JavaScript code on a form page. For example,
if (ExternalInterface.available) { ExternalInterface.addCallback("getDiapasonData", getDiapasonData); ExternalInterface.addCallback("setDiapasonValue", setDiapasonValue); }
Here, in case of availability of the ExternalInterface, two methods are registered:
getDiapasonData - is responsible for receiving and initial processing of data necessary for the correct operation of the sliders. It is executed once after Javascript generates the necessary parameters for transfer to the swf-file.
private function getDiapasonData(begin_diapason:Number, next_diapason:Number, price_status:Number = 0, min_price:Number = 0, max_price:Number = 0):void {
setDiapasonValue is responsible for passing specific range values from a JavaScript function for future use. It is executed each time the textual values of the range are filled in the HTML form.
private function setDiapasonValue(temp_min_price:int, temp_max_price:int):void {
The parameters passed from the JavaScript function become full-fledged parameters of the ActionScript methods and are suitable for programming the animation of sliders.
To perform the inverse transfer of values from the ActionScript JavaScript function, the call method of the ExternalInterface class is used, which is passed the name of the necessary JavaScript function with parameters.
For example, the following methods are used to transfer the values of each slider from ActionScript to the corresponding JavaScript functions. There is a calculation of the price depending on the position of the slider, rounded up to 100 rubles:
private function setStartDiapason():void { var relation:Number = line_mc.x / 276; var current_diapason:Number = Math.round((start_diapason + Math.round((end_diapason - start_diapason) * relation)) / 100) * 100; ExternalInterface.call("setStartDiapason", current_diapason); } private function setEndDiapason():void { var relation:Number = (line_mc.x + line_mc.width) / 276; var current_diapason:Number = Math.round((start_diapason + Math.round((end_diapason - start_diapason) * relation)) / 100) * 100; ExternalInterface.call("setEndDiapason", current_diapason); }
2) Preparing the parameters for the range form in the Umi.CMS administration panel and setting up XSLT templates for them.
To ensure the full functionality of the price range form, it is necessary to provide the site administrator with the ability to independently set its boundaries: the minimum and maximum prices.
Prices change over time, and specifying the broad boundaries of the range degrades the accuracy of calculations in the implementation of animation. Moreover, for certain groups of goods, the price range is completely calculated.
To store user properties, Umi.CMS suggests creating pages of a special data type, for example, “General site parameters”. On this page, you can create a property group "Price Range", and in it place two properties of the numeric type:
The minimum price level (minimalnyj_uroven_cen) and the Maximum price level (maksimalnyj_uroven_cen). We remember the identifiers of these properties generated by the system, or we change them to more readable ones, and we also remember them.
After that, in the structure tree of the pages, create or find the General Site Settings page and indicate the values of these properties.
In the XSLT template, select the values of the price range and save in variables:
<xsl:variable name="minPriceValue" select="$commonParams//property[@name = 'minimalnyj_uroven_cen']/value" /> <xsl:variable name="maxPriceValue" select="$commonParams//property[@name = 'maksimalnyj_uroven_cen']/value" />
Here commonParams is a variable that stores information about the properties of the General Site Settings page.
Directly in the XSLT template we place the following javascript code
<script type="text/javascript"> // , min_price_product = parseFloat('<xsl:value-of select="$minPriceValue" />'); max_price_product = parseFloat('<xsl:value-of select="$maxPriceValue" />') + 100; start_diapason_price = min_price_product; end_diapason_price = max_price_product - 100; if(start_diapason_price > 0) { current_start_diapason_price = start_diapason_price; } else { current_start_diapason_price = min_price_product; start_diapason_price = min_price_product; } if(end_diapason_price > 0) { current_end_diapason_price = end_diapason_price; } else { current_end_diapason_price = max_price_product; end_diapason_price = max_price_product; } // , <xsl:if test="$min_price !='' or $max_price !=''"> price_status = 1; min_price = parseFloat('<xsl:value-of select="$min_price" />'); max_price = parseFloat('<xsl:value-of select="$max_price" />'); </xsl:if> </script>
Here you can select the values of the price range from the administrative panel and prepare the necessary variables for transfer to the swf-file with the animation of the sliders.
Generating JavaScript code directly in the XSLT template is not a very pleasant experience, since some JavaScript constructs cannot be written without being further processed in accordance with the XSLT rules.
The Umi.CMS template engine jealously ensures that the template syntax is clean, but this is the simplest way to get the values of variables from the administrative panel.
In addition, these variables are used further in the template to solve other tasks that are not related to the current one.
3) Preparing JavaScript code to organize the interaction between the form and the sliders.
You need to prepare data exchange functions with ActionScript code. They can be placed in a separate javascript file. There is also the initialization of the necessary variables and the launch of the function of initial setting the positions of the sliders after the page loads.
For the latter purpose, the jQuery library is used, which must be pre-connected, and since its cross-browser compatibility is not recognized by all browsers, you can supplement the call to the initialization function by assigning an onLoad event handler to the body tag.
There are no special libraries for organizing the interaction of JavaScript and ActionScript. Everything is already there and is ready to be used directly in the languages themselves.
The list of required JavaScript functions might look like this:
The values set by these functions are further used to select products in the specified price range.
After completing the above steps, the site visitor receives a product filtering form, in which he can set the price range using the swf file sliders or the usual text input fields.
Approximately according to this scheme, you can organize the interaction of Umi.CMS with Flash-videos, using JavaScript as an intermediary.