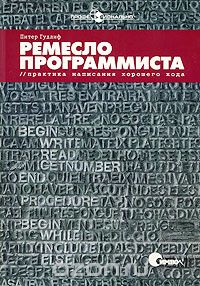
This post is an excerpt of the “golden rules” from the remarkable book by Peter Goodlyfe
“The Craft of the Programmer” .
Someone will refresh the memory, someone will check as a check-list, and someone will be interested and read the book. Because The post turned out to be quite voluminous, you can add it to your bookmarks and periodically return to it.
Chapter 1. Keep the defense
Do not make any assumptions. Formally unrecognized assumptions often serve as the cause of failures, especially as the code grows.
The more haste, the less speed. Always think what you are going to type from the keyboard.
')
Do not believe anyone. Anyone, including yourself, can make mistakes in the logic of your program. Treat all input and output data with suspicion until you verify that they are valid.
Compiler warnings help identify a lot of stupid errors. Always include their output. Keep your code compiled silently.
Take care of all limited resources. Carefully organize their capture and release.
Chapter 2. Thin calculation
Understand who will actually read your source code: other programmers. Write with the expectation of them.
Find out what code standards there is for your chosen language, and learn each of them in practice. Evaluate the advantages and disadvantages of each.
Choose one correct coding style and stick to it all the time.
If there is a coding standard in your group, stick to it. Leave your own favorite style.
Say no to religious wars. Do not join them. Step aside.
Chapter 3. What is in my name?
Learn to give objects transparent names — they must clearly describe what lies behind them.
To come up with a good name, the main thing is to clearly understand for whom it is intended. Only in this case the name can become meaningful. If you can't think of a good name for an object, ask yourself if its purpose is clear.
Learn the rules for forming names in the language you work with. It is even more important to study the idioms of this language. Are there standard ways of forming names? So use them.
The clarity of the name is preferable to its brevity.
The relative merits of short and long names should be considered, especially depending on the scope of the variable.
A naming rule that distinguishes between variable names and type names is preferable.
Let the function names from the external point of view, in the form of phrases expressing actions. Describe the logical operation, not the way to implement it.
Avoid unnecessary words in the names. In particular, in type names — words such as class, data, object, and type.
Give namespaces and packages names that are logically related to content.
Macros in C / C ++ are always capitalized to be clearly visible, and their names are carefully chosen to avoid conflicts. Never capitalize other objects.
Choose a uniform naming system and apply it consistently.
The extent to which the name is specified depends on the context in which it is used. When creating names, consider contextual information.
Chapter 4. Literary Criticism
Do not write code that needs external documentation. He is unreliable. Write a code that is understandable without external documentation.
Write a code that a normal person can read, and without stress. The compiler will cope somehow.
Avoid the magic numbers. Use named constants.
Important parts of the code should stand out and be easily readable. Hide everything that should not interest customers.
Try to group related information. Make this grouping visible with the help of language tools.
Do not display meaningless error messages. Depending on the context, provide the most relevant information.
Put comments in the code only if you can not facilitate his understanding in other ways.
Use the tools of competent documentation for the automatic generation of documentation for your code.
Chapter 5. Marginal Notes
Learn to write just as many comments as you need. Give preference to quality rather than quantity.
Take the trouble to have your code do not require support in the form of a lot of comments.
Good comments explain why, not how.
One source for each fact. Do not copy the code in the comments.
When you find that you are writing wordy comments describing your code, stop and think. Is this a sign that there is some higher order problem?
Think what you write in the comments; do not press thoughtlessly on the keys. Read the comment again in the context of the code. Does it contain information?
Comments are part of the code narration. Place them so that the reading order is natural.
Supply each source code file with a prologue in the form of a comment.
Comments should concern the present, not the past. Do not describe what has changed, and do not talk about what happened before.
If you change the code, check the correctness of the comments next to it.
Chapter 6. It is human nature to err
Error handling is a serious matter. It depends on the stability of your code.
Do not leave a failed situation without attention. If you do not know how to deal with the problem, signal the failure to the calling code. Do not sweep the garbage under the carpet in the hope that everything will somehow manage.
Never neglect the incoming error messages. If there is a channel for error messages, then there are reasons for this.
Treat all errors in the most favorable context when it becomes clear how to handle it correctly.
Ignoring errors does not save your time. You will spend more time figuring out the reasons for the incorrect behavior of the program than you would need to write an error handler.
If you write code that can fail, write code at the same time to detect and handle errors. Do not postpone it for the future. If you still have to postpone processing, at least write a snap-in for error detection.
Chapter 7. Programmer Tools
Learn your standard tools far and wide. The time you spend studying them will immediately pay for itself.
Treat programming tools pragmatically; use them only if they make your life easier.
Find out what kinds of tools exist. Find out where you can get them, even if you don't need them at the moment.
For each task there is a tool. Do not click nuts sledgehammer.
Watch out for the latest version of your tool, but be careful when upgrading.
The choice of code editor is crucial; it has a huge impact on how you will write code.
Learn a few languages. In each you will find a special way to solve problems. Treat them as a set of tools and choose the one that is most effective in a particular situation.
Chapter 8. Test Time
Testing can reveal only the presence of errors. It can not prove the absence of faults. Do not give in to the false sense of calm, if the code has passed a series of inadequate tests.
Test every piece of code you write. Don't expect someone else to do it for you.
To make testing effective, it needs to be started in advance, when the detected errors still cannot do much harm. Test code can be written earlier than working!
Write tests for all identified errors.
Run your tests as often as possible.
Reading the code is very easy to be deceived and believe that it works correctly. If you have written the code, then when reading it you will see what you were going to write, and not what you actually wrote. Learn to read code with cynical disbelief.
Write a complete test suite, each testing a specific aspect of the code. Fifteen tests that demonstrate the same error are less useful than 15 tests that demonstrate 15 different errors.
The code architecture should facilitate its testing.
As far as possible, automate code testing. This is faster and easier than doing manual tests, and much more reliable: it’s more likely that tests will be performed regularly.
Perform testing automatically during the build procedure.
Chapter 9. Search for errors
Compile the code with all warning messages turned on by the compiler. Thereby, you will discover potential problems before you actually come across them.
Follow the golden rule of debugging: think with your head.
Set a reasonable time limit on "unsystematic" debugging, and if it fails, go to a more methodical way.
Study the code being debugged - it is difficult to find errors in the code that you do not understand.
When you're looking for a mistake, disbelieve anyone. Check out the most incredible reasons instead of rejecting them right away. Do not take anything for granted.
If the product build fails, look at the first compiler error. Subsequent messages are much less credible.
Debugging is a methodical job, slowly narrowing the ring around the location of the error. You should not treat it as a game of ukadayku.
The first step in establishing the location of the error is to determine how to play it with confidence.
Start from a known place, for example, from a program crash point. Then move in the opposite direction towards the cause of the crash.
If it seemed to you that you found the cause of the error, examine it thoroughly and make sure that you were right. Do not accept recklessly the first hypothesis.
Debugging ends only when you prove that the error is fixed and the problem is solved forever.
Take extreme care when correcting errors. Make sure that your modification does not cripple anything else.
Correcting the error, check if it is repeated in close sections of the code. Destroy the error once and for all: fix all its duplicates immediately.
From each corrected error, draw conclusions. Could it have been avoided? Could she have been faster?
Faced with behavior that you cannot explain, use debuggers sparingly. Do not get used to immediately rush to them without first trying to understand how your code works.
Chapter 10. The Code That Jack Built
Consider the build system as part of the source tree and share them together. They are closely related.
All programmers participating in the project should use a single build system. Otherwise, they will all be assembled in different software packages.
The correct build system allows you to repeatedly create physically identical binary files.
You should be able to extract the source tree of three years ago and properly assemble it again.
The correct system looks like a single operation. Just press the button or execute one command.
For each assembly rule, write the appropriate purge rule that cancels the entire operation.
Organize an automatic build procedure for your software product. Check with its help the performance of your code.
Final assemblies are always run from pure source code. Take care that you can then always get this clean source code from the archive or version control system.
Test the final configuration of your application, not just the working builds. Small differences between them can negatively affect the code behavior.
Chapter 11. Need for Speed
You need to think about the effectiveness of the program from the very beginning - do not expect that at the end of the development you will be able to increase it at the cost of small changes.
The correctness of the code is much more important than its speed. What is the use of getting results quickly if it is incorrect!
Consider optimization alternatives; Will you not be able to improve the effectiveness of the program in other ways?
Find out if there really is a need to optimize the code, but it’s better to write efficient, high-quality code right from the start.
Optimize the code separately from any other work so that the results of one work do not affect the other.
Optimize final versions of the program, not intermediate builds.
Carefully select the input data for profiling to reflect the real world. Otherwise, it may turn out that you optimize those parts of the program that are not normally executed.
Do not limit yourself to the profiler when looking for the reasons for the lack of effectiveness of the program; they may be deeper.
Be sure to take measurements before and after optimization.
It is better to replace the slow algorithm with a fast one, than to try to improve the implementation of the existing algorithm.
Chapter 12. Insecurity Complex
Keep in mind what important resources you have. Do you have any particularly sensitive information or special features that you can hunt? Protect them.
The more complex the computer system, the more likely it is that there are gaps in its security system. Therefore, write as simple as possible software!
Do not pass by vulnerabilities and do not consider yourself invincible. Somewhere there must be someone who is trying to apply an exploit to your code.
Run only programs from trusted sources on your computer. Establish a clear policy for determining the reliability of sources.
Security is an important aspect of the architecture of any software product. It would be a mistake not to take care of it in the early stages of development.
When designing a program, rely only on well-known, protected third-party components.
Get ready for the fact that your system will be attacked, and design with this in mind all its parts.
Chapter 13. The Importance of Design
Programming is a design job. This is a creative and artistic act, not a mechanical code writing.
Think before you knock on the keys; make a clear project. Otherwise, you will not have code, but chaos.
Less is better. Your goal should be a simple code that solves large problems with a small size.
It’s hard to make a simple thing. If the structure of the code seems obvious, do not think that it was easy.
Design such modules that are internally connected and minimally interconnected. Decomposition should reflect the correct breakdown of the task into parts.
Draw a line that no one should overstep: define clear APIs and interfaces.
Design with the subsequent expansion, but do not overdo it, otherwise you will write not the program, but the OS.
Do it once. Do well. Avoid duplication.
Solve the problems of code portability at the design stage, and not by reworking the finished code.
Be pragmatic about design tools and methodologies: use them when it’s really useful, but don’t become their slaves.
Chapter 14. Software Architecture
The architecture has the greatest influence on the design and further development of the software system. For this reason, it is important to correctly identify it at the earliest stages of development.
Keep a description of the system architecture in a place where it is available to anyone you may need: a programmer responsible for maintenance and installation, managers, and possibly clients.
Architecture specification is an important tool for reporting on the status of your system. Make sure that it matches the state of the software.
All design decisions for the software product take in the context of the architecture. Be careful not to deviate from the system vision and strategy. Do not make small changes to anything not related.
Good system architecture should be simple. A description consisting of one paragraph and one simple diagram is enough.
The architecture defines the most important components of the system and their interaction. She does not describe their device.
Good architecture leaves room for maneuver, expansion, modification. But her community does not go beyond reasonable limits.
Understand the basic architectural styles and appreciate their strengths and weaknesses. This will help you more sympathetic to the software products that you encounter, and properly design the system.
Chapter 15. Software - evolution or revolution?
Remember how easily the modified code degrades. Avoid modifications, after which the system is in the worst condition.
Learn to detect broken code. Examine its symptoms and handle decomposed code with extreme caution.
Be good at writing code. Good programmers think about how their code will look like in a few years, and not about how much effort they need to make today.
Write a new code with the possible need to modify it in the future. Make it understandable, extensible and simple.
Be careful when modifying. Be aware of whether someone is modifying a nearby code.
Discuss dangerous changes, especially when preparing to release a new version. Even the simplest modifications can break other code.
Do not mess the code thoughtlessly. Stop and think about what you do.
Carefully test all modifications you have made, no matter how simple they are. It is very easy to miss a stupid mistake.
Chapter 16. Encoders
Find out what type of programmers you are. Determine how you can profitably use your good qualities and compensate for the bad ones.
Chapter 17. Together we are power
The ability to work in a team is an important quality of a highly qualified software developer.
The resultant code is influenced both by relations within the team and external contacts. Watch how they affect your work.
Organize your team in accordance with the code you are going to build, and not vice versa - the code in accordance with the existing team.
The presence of channels for effective communication is vital for the normal work of the team. Such channels need to be organized and maintained. A good programmer should be able to communicate well.
No programmer owns any part of the code. Each of the participants has access to the entire code and can modify it as needed.
Successful teams develop and work purposefully; their occurrence cannot be attributed to chance.
Do not lose information when people leave the team. Organize its transfer and get all the important data from them, including code documentation, testing tools and maintenance instructions.
Chapter 18. Source Code Protection
The code has value. Treat him with respect and care.
Version control system is an important software development tool. It is necessary for the reliable work of the team.
Be careful about the repository. Take care not to write in it a non-working code that does not allow other developers to work.
Backup your data. Develop a recovery strategy without waiting for the crash.
Chapter 19. Specifications
The software development process requires not only the availability of specifications, but their high quality.
Specifications are an important communication mechanism between software developers. They are used to record information that should not be lost or forgotten.
Software requirements need to be set in advance so that no unfulfilled expectations arise in order to prevent the expansion of functionality and reduce the concerns of developers.
If your programming task is not clearly defined, do not start coding without writing specifications and agreeing on them.
Use the tools of competent programming for the preparation of technical documentation. Do not write in a text editor a document that quickly becomes obsolete.
When making a specification, think about its content. Choose a structure and vocabulary that is understandable to the audience, and make sure that the document is correct, complete and contains its own description.
Avoid making specifications dangerous and unprofessional. If there is not enough time to write specifications, it is most likely not enough to write code.
Chapter 20. Shooting Review
Code reviewing is an excellent tool for finding and fixing hidden errors, improving the quality of code, strengthening collective responsibility for code, and disseminating knowledge.
When creating a system, find out if you need to conduct a review, and if so, what code.
Carefully select the code for review. If you cannot review the entire code, make an informed choice. No need to select at random and lose precious time.
Whoever the code is written, it is subject to review and careful study by colleagues. Get review of your code.
The success of the review is highly dependent on the positive position of the author and reviewers. The purpose of the review is to work together to improve the code, and not to appoint the perpetrators or to justify the decisions made during the implementation.
If you don’t know how good code should look like, you cannot reasonably judge the quality of the work of other programmers.
Chapter 21. How long is the string?
Estimating the duration of a software product development is a fact-based conjecture. For each evaluation there is some measure of your confidence in it.
Estimating program development time is a really difficult task. Avoid underestimating the amount of work to be done. Consider the possible consequences of incorrect evaluation.
Everyone (including you) wants shortened schedules. Do not be fooled about what you can really do in the time allotted to you. Do not make unrealistic promises when you need to provide a code ready for delivery.
Time estimates need to be applied to small tasks whose workload is easy to understand. Unit of measure shall be man-hours or man-days.
Distinguish whose work you rate by time: your own (on a system that you understand well) or someone else's (someone who may need to study the system).
Do not do evaluations alone. Find out the opinions of other people to clarify their estimates.
Schedule work so that there is time to bring the code in order. Plan for the return of technical debt.
Constantly check your progress with the plan. Then there will be no suddenly detected lag.
Chapter 22. Recipe Program
Good programmers know how to program - own the methods and techniques of work.
Our software projects are determined by the styles and processes that we apply. They inevitably affect the form and quality of the code.
Without a development process, the team is in a state of anarchy. Programs appear due to luck, and not as a result of targeted actions.
The choice of recipe program can be determined by many reasons that must be compelling. Motivating your choice says a lot about the maturity of your organization.
The process you are taking should not be too formal and difficult to implement. Usually, the characteristics of a good process are just the opposite characteristics. However, there must be a certain process.
The selection process is vital. Unsuccessful projects in most cases turn out to be such non-technical reasons. Almost always one of the main reasons is the poor organization of the process.
Chapter 23. Beyond the Possible
There are different types of programming in different subject areas. Each area has its own particular problems and requires specific skills and experience.
Know your programming area. Cut her specifics. Learn to write great programs that meet its requirements.
Chapter 24. What's Next?
To become a good programmer, you need to choose the right position - the angle from which the creation of software is considered.
Practice improving your skills. Do not lose the love of programming and the desire to be a master in it.