So, we have already talked
about the origin of the Android OS architecture and
about the patterns implemented in this architecture . Now it's time to talk about what the Android application is made of.
This article will present the main "characters" of the architecture of the Android application.
In general, the Android application consists of:
Java classes
The following diagram shows the hierarchy of the main classes from the Android SDK, with which the developer has to deal:
')
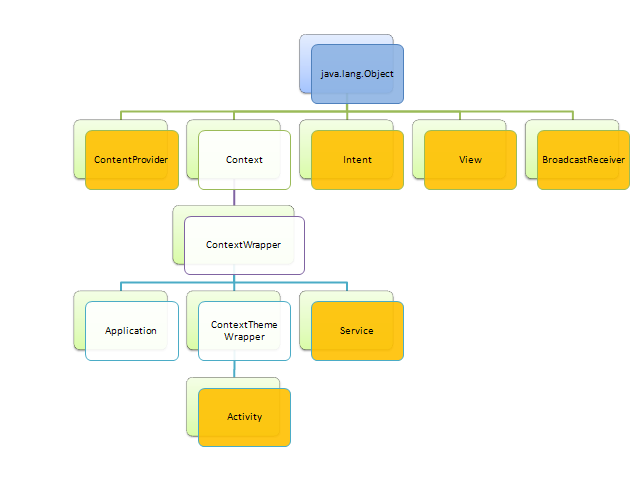
In fact, there are many more classes, but these are the main ones. Dedicated yellow - those with which the developer works directly (in particular, inherited from them). The rest are just as important, but less commonly used directly.
View is the base class for all user interface widgets (GUI widgets). The interface of an Android application is a tree of instances of the heirs of this class. You can create this tree programmatically, but this is wrong. The user interface is defined using XML (layer files, layout files), and at runtime it automatically turns (inflate, the term Android) into a tree of corresponding objects.
The
Activity class and its subclasses contain the logic behind the user interface. On closer inspection, this class corresponds to the ViewModel in the
Model-View-ViewModel architectural pattern (MVVM). The relationship between the Activity subclass and the user interface is a one-to-one relationship; Normally, each Activity subclass has only one UI layer associated with it, and vice versa. Activity has a life cycle.
During the life cycle, an Activity can be in one of three states:
- Active and running - this user interface is in the foreground (technically speaking - at the top of the activation stack)
- Suspended - if this user interface has lost focus, but is still visible. In this state, no code is executed.
- Completed - if the user interface is invisible. In this state, the code is not executed.
The activation code is executed
only when the corresponding user interface is visible and has focus. There is no guarantee that the Activity object and its associated objects are in memory when the activation is paused or completed (it is very important to remember this; we have previously discussed this point of memory management in Android).
The
ContentProvider class and its subclasses represent model in the MVVM architecture. In most practical cases, this is a wrapper over a SQLite database with a slightly bizarre URI-based access method. Theoretically, no one bothers the developer to create a ContentProvider based on something other than the database. However, the content provider’s existing query () method returns a
Cursor object, which is very similar to the JDBC
ResultSet interface and how it works. Therefore, it is unlikely that anyone will doubt that the real purpose of content providers is to encapsulate a database.
I do not know how the Android team came to this design, but, in my opinion, there are two good, but not very compatible, ideas here.
And that's why I think so. The basic idea of ​​content providers seems to be based on the architecture of AJAX applications. AJAX applications typically use the MVVM architecture, where the model is represented as a server-side URI (however, this has changed with HTML5, which allows you to store data locally). In fact, the fact that content providers are requested using a URI and creating an extension using MIME types indicates that AJAX is at the core. Let me remind you, the guys from Google created a large number of AJAX applications, such as Gmail, Google Docs, etc., so it’s quite natural that the ideas were borrowed from the AJAX architecture.
Perhaps someone else came up with another great idea: how great it would be to have a full-fledged relational base on a mobile device! (I note that it was around 2005, when mobile phones were much weaker than they are now). And, as a result, they combined two good ideas into one ContentProvider class. As it usually happens in software development, combining two good ideas does not always result in a good idea; in the case of Android, we have a somewhat discouraging design of content providers.
Class
Service and its subclasses I find it difficult to somehow classify. I think the guys at Google are experiencing the same difficulties (please read their documentation). Their classification basically says what this class is not. I personally think that a service is a type of Model that serves slightly different uses than ContentProvider.
In my opinion, the architectural design of the Android Service is inspired by
OSGI services .
I think the services were created by the guys from Google as a solution to a logical problem that arose because of the Android thread model.
Think about it: Activity is active and only runs when its user interface is in the foreground. As soon as the interface of another Activity closes the current one with itself, the latter stops, even if it did something. And what if you need to perform an operation, even if the process that performs it is not in the foreground? With the help of Activity you can not do this. You cannot do this with the help of ContentProvider, since they do not have their own life cycle, and they can be executed only while the Activity that uses it is active.
And here services come to the rescue. They can be performed even when the process in which they operate is not in the foreground. So, if you are developing an activity that performs a time-stretched operation that should complete even working in the background, you must create a Service that implements this operation and start it from the Activity.
Service also has a life cycle. This means that it can be instantiated and launched by an Android application for some condition (we will discuss this later).
As I already mentioned, Service, like model, has more general objectives than ContentProvier. It can use a database, but its API is not associated with a database, as in the case of a ContentProvider. In most cases, services are used to communicate with external servers.
The
BroadcastReceiver class and its subclasses represent a “subscriber” in the
publisher / subscriber interaction mechanism implemented in the Android architecture.
We have already talked about the mechanisms of interaction in the previous article.
Of course, a developer for Android is not limited to just the extension of classes from the Android SDK. He can write his own classes as he wants. But they will all be only helper classes for classes from the Andoird SDK.
Android Manifesto
Android Manifesto is another important part of the Android application. The idea was inspired by
plug-in manifests for Eclipse .
The Android manifest is an XML file and performs several functions. This is how Google describes them:
- Specifies the name of the Java application package. The package name is a unique identifier for the application.
- Describes the components of the application - activites, services, broadcasters and content providers. Defines the class names that implement each of the components and announce their capabilities (for example, which Intent messages they can process). These ads allow the Android system to know which components and under what conditions can be launched.
- It predetermines the processes that the application components will contain.
- Declares the permissions that an application must have to access secure parts of the API and interact with other applications.
- It also declares the permissions that are required to access application components.
- Lists Instrumentation classes that provide profiling and other information while the application is running. These ads are present in the manifest only while the application is being developed and tested; they are deleted before the application is published.
- Announces the minimum level of the Android API that the application requires.
- Lists the libraries with which the application should be associated.
Pay attention to the second paragraph. This means that if a certain class extends an Activity, ContentProvider, BroadcastReceiver or Service in your application, this class cannot be used until it is described in the manifest.
Resources
Every modern GUI application uses resources in one form or another. Android apps are no exception. They use the following types of resources:
- Images
- GUI Layers (XML Files)
- Menu declarations (XML files)
- Text strings
The way in which resources are associated with an Android application is something unusual. As a rule, in Java resources are identified by strings. Such strings may contain, for example, the path and name of the file containing the image, or the ID of the given string, etc. The problem is that errors in such links cannot be detected during the translation of the code.
Let's consider the following example. The file named mybutton.png contains the image for the button. The developer makes a mistake and dials mybuton.png, referring to the resource from the code. As a result, the code tries to use a non-existent resource, but the compilation will succeed. An error can only be detected during testing (or it may not be detected at all).
The guys from Google have found an elegant solution to this problem. When building an Android application, a special Java class is generated with the name
R (just one letter). This class contains several static final data sets. Each such data set is a link to a separate resource. These links are used in the application code to communicate with resources. Now every error in the link to the resources manifests itself in the compilation process.
Files
The Android application uses several different file types:
- General Purpose Files
- DB files
- Opaque Binary Blob ( OBB ) files (they are an encrypted file system that can be mounted for an application)
- Cached files
Although, of course, all of them are only Linux files, it is understood to consider them as separate file types only in the context of processing them with various APIs and separate storage. They are also separated from files stored in internal or external storage (the latter may be absent or disappear / appear at any time).
The API for working with files is implemented by the
Context class, from which the Activity and Service classes are generated. This class has already been discussed by us
here .
That's all for today. In the next article we will talk about how the different parts of the Android application interact with each other.
Previous articles:
Next article:
Android application architecture. Part IV - integration level