Good day!
What is Sinatra
Sinatra is a small but rather interesting DSL (Domain-specific language) framework written in Ruby. Unlike Ruby on Rails, it does not follow the typical MVC pattern (Model - View - Controlller). Sinatra was created so that “a programmer could quickly create a web application written in Ruby with minimal effort.
In order to use Sinatra, we don’t need to install it. You can do this with the following command in RubyGems:
')
gem install sinatraWe also need a gem called
Shotgun . We need it in order not to restart the server manually once again. Shotgun will do it for us. Let's install it:
gem install shotgunWell that's all! We have everything necessary for work. The time has come for creativity. Let's start ...
Hello, Sinatra!
And we will write a simple application that welcomes itself. I sincerely hope that Frank will not turn over in his grave. So, here it is, our application:
require "sinatra" get "/" do "Hello, Sinatra!" end
It remains only to start the server. You can do this by typing the following command:
ruby -rubygems path_to_file \ app.rbNow we can open the browser. In Omnibox you need to dial the address
localhost: 4567 . Voila!
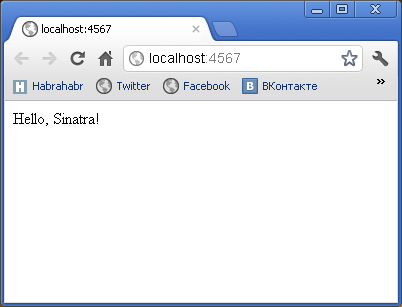
Congratulations! We just wrote the first web application using Sinatra.
Debriefing
Despite the fact that it was the simplest example, it would not hurt to figure out what our code does.
From the beginning I would like to note that when developing web applications I use Ruby version 1.9. In case you are using version 1.8., You need to add another line to your code. Here she is:
require "rubygems"
Moving on. We say to our application that if a user in the address bar drives in the address of our site, then it hits this page, i.e. home, where we welcome Frank. If the user specifies in the address bar, for example,
/ about , then he will get the following error:
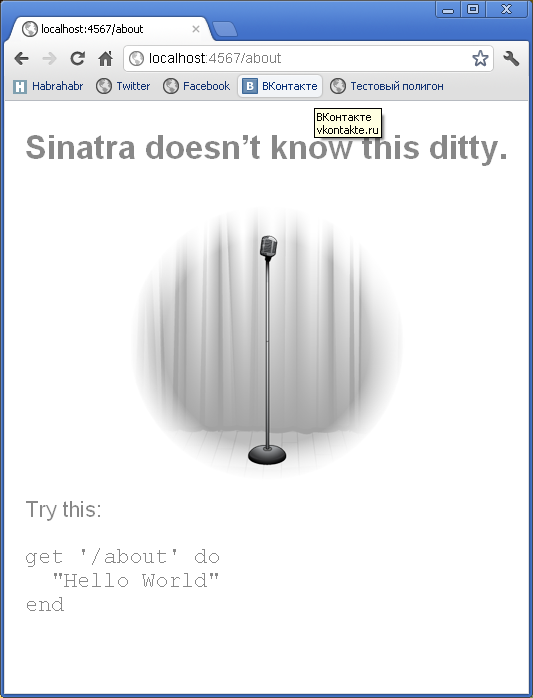
Let's handle the
Not Found error. This can be done in the following way:
not_found do status 404 "Something wrong! Try to type URL correctly or call to UFO." end
Now we type in the address bar
localhost: 4567 / about and see our page with you that tells the user that something wrong has happened.
Hello,% username%
It's time to remember that we met with Frank, but he is not with us. God forbid, of course. But we can make our browser greet us. This can be done using the
name parameter. Its processing will look like this:
get "/hello/:name" do "Hello, #{params[:name]}." end
It remains only to dial
localhost: 4567 / hello / your_name and you will see a greeting in your address. For example:
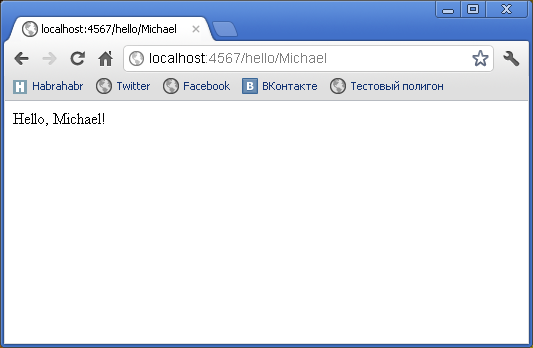
I am where you are
It is with this expression that we can characterize the situation when we need, for example, to give the user only one page, but no matter what address he dials. This is done by specifying an asterisk after the slash. Like this:
get "/*" do "It's the main page of this site you can see right now!" end
Now wherever you want to go, you'll see the same page. By the way, here it is:




The value of the parameter specified in the URI can be obtained using the
splat attribute. Here so:
get "/*" do "I don't know what is the #{params[:splat]}. It's what you typed." end
Voila! So that we do not type in the address bar, but see the dialed URI.


Conclusion
That's all for today. Despite the size of the topic, we dismantled some routing schemes in Sinatra. I hope that it will awaken in you the desire to quickly get acquainted with this framework, which allows you to write web applications with unprecedented speed. This is only a starting point and no more.
Where to go next
Introduction to Sinatra .
The official site of Sinatra .
Singing with Sinatra .