Some time ago I turned up an inexpensive
VFD- screen. From it, I made something like a watch, connecting to the home server.

Such displays look quite beautiful, and using them is very simple, so they are suitable for many projects.
')
I used the IEE 036X2-122-09220 display (aka IEE 36199-01), they can be found in ibei. Most likely, working with any other VFD from IEE will not be different (as well as with many other similar displays, for example, from cash registers).
The display supports three parallel interfaces (Intel 8041/42 and Motorola 6821 processor buses, HD44780 emulation mode) and the usual asynchronous serial input with RS-232 levels. Parallel interfaces are convenient for embedding the display in ready-made devices, it’s more convenient for us to use serial input.
Display Connector:
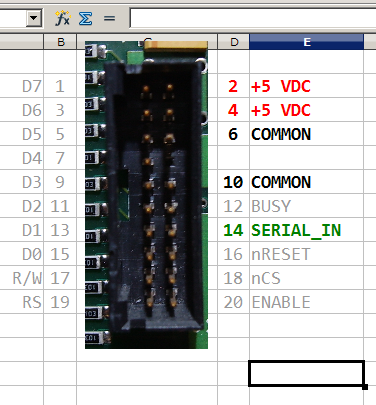
We need only +5 VDC (power), COMMON (ground) and SERIAL_IN (serial input). The remaining pins are used for parallel interfaces, they are already pulled to power and you can leave them hanging.
The display can be powered from a USB port or a PC power supply. For communication, the easiest way is to use the COM port.

However, naturally, it is better to somehow cling to the USB. For example, using the
popular AVR-CDC adapter on Tine 2313 , supplemented with a level converter on the MAX232. True, I had problems with this adapter when I switched to 64-bit Debian (the device seemed to be properly picked up by the system, but did not work). Such a USB-COM tail on PL2303 and MAX212, bought in one of the Chinese online stores, came to the rescue.
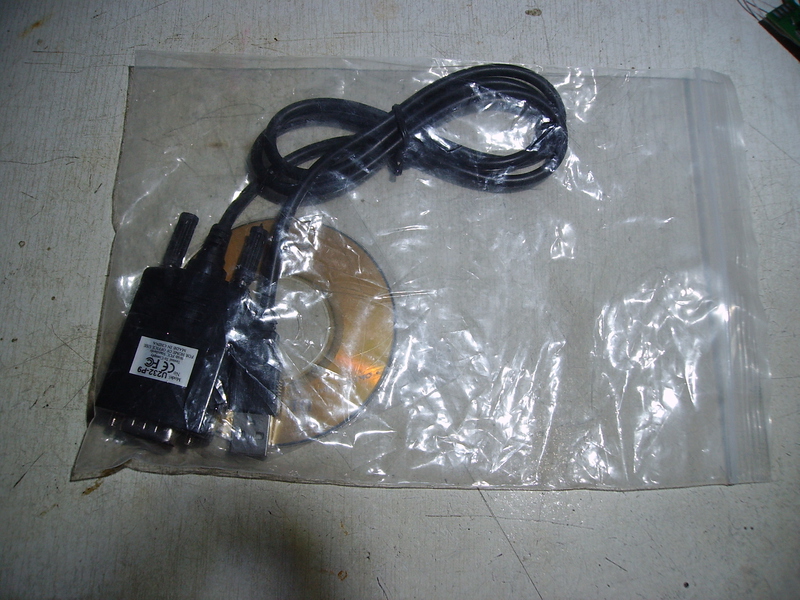
The tail I gutted and connected the display power +5 VDC and COMMON directly to USB (red and black wires, respectively), and SERIAL_IN - to pin 3 (TxD) of the COM port.

On the power hung capacitor 100 microfarads (although this is not necessary).
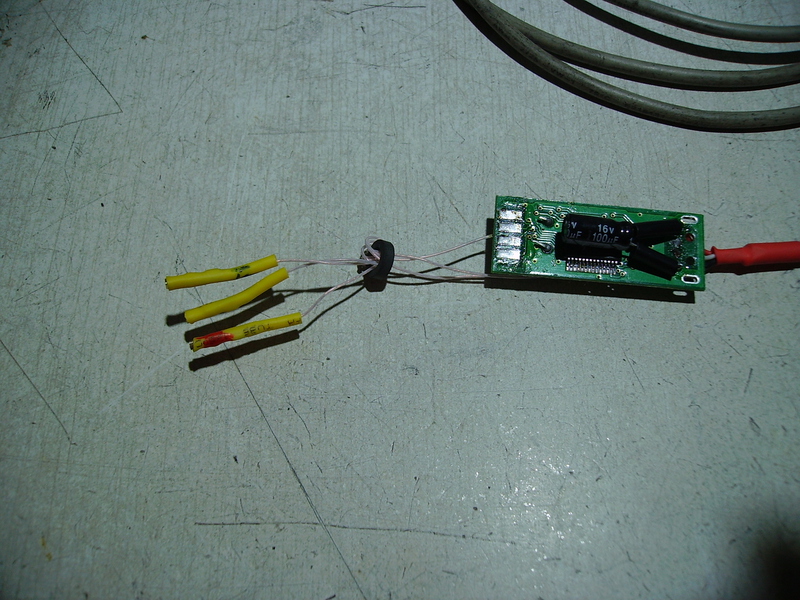
To check the screen, suitable, for example, microcom.
sudo apt-get install microcom
sudo microcom -s 9600 -p /dev/ttyUSB0
/ dev / ttyUSB0 - this is for adapters on PL2303, FT232, etc. For the COM port of the computer, most likely it will be / dev / ttyS0, but adapters like AVR-CDC live in / dev / ttyACM0, etc.
9600 is the speed of the serial input of the display, chosen by the jumpers on it.
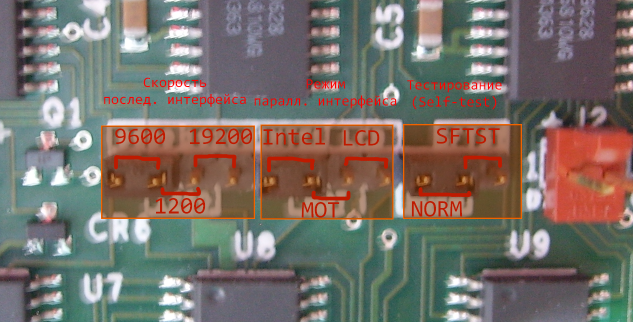
The character set that the display uses is similar to ASCII. We type something in microcom - it should appear on the display.
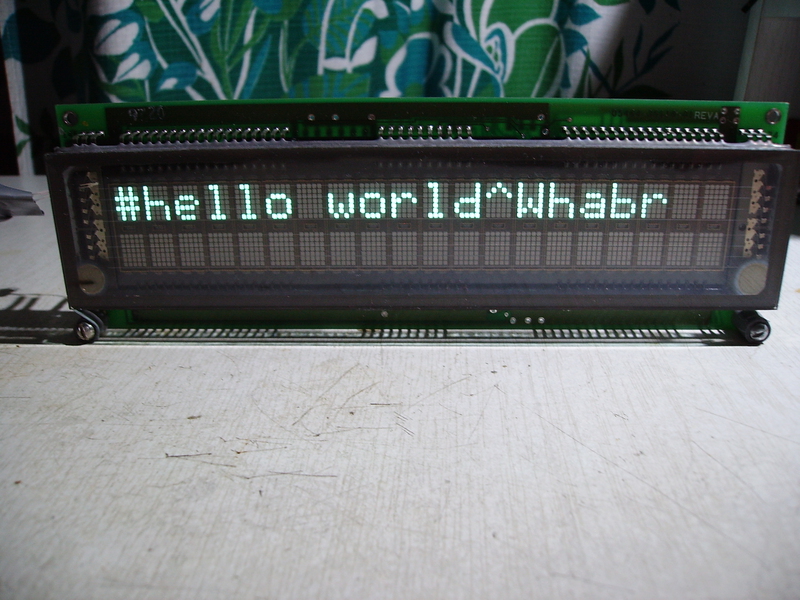
To control the display, you can adapt something like
lcd4linux ,
lcdproc , etc. But their capabilities can often be insufficient, in general, it is easier to write yourself)
We will write on the pearl, because he is concise and clear.
To begin with, in order to display the data in real time, we will need to tear off the buffering.
$| = 1;
Now the pearl will automatically reset the buffer.
The display is controlled by special characters. For example, 0x0F - show the cursor, 0x15 - clear the screen. For more information, see the documentation referenced below.
We will write a watch.
#!/usr/bin/perl use strict; use warnings; use LWP::Simple; # sub display_init() { $| = 1; # binmode(STDOUT,':raw'); # print pack("C",0x14); # print pack("C",0x0e); # print pack("CCCCCCC",0x18,0xf6,0x00,0x04,0x44,0x40,0x00); # } # sub display_goto() { my ($x, $y) = @_; my $pos = $x + $y*20; print pack("CC",0x1b,$pos); } # sub get_weather() { my ($city) = @_; my $url = "http://www.google.com/ig/api?weather=$city"; my $data = get $url or return undef; if($data =~ /<current_conditions>(.+?)<\/current_conditions>/) { my $weather = $1; my %info; while($weather =~ /<(.+?) data="(.+?)"\/>/g) { $info{$1} = $2; } return \%info; } return undef; } # sub display_time() { my($sec,$min,$hour,$mday,$mon,$year,$wday,$yday,$isdst) = localtime(time()); my @abbr = qw( Jan Feb Mar Apr May Jun Jul Aug Sep Oct Nov Dec ); printf "%s %02d %02d:%02d:%02d",$abbr[$mon],$mday,$hour,$min,$sec; } # sub display_weather { my $city = shift; my $info = &get_weather($city); if(!$info) { print "Err!"; return; } my $temp = int($info->{temp_c}); printf("%+3d%s",$temp,chr(0xf6)); } &display_init(); my $last_weather_update = 0; while(1) { # &display_goto(0,0); &display_time(); # ( ) if(time() - $last_weather_update > 30*60) { &display_goto(16,0); &display_weather("Novosibirsk"); $last_weather_update = time(); } sleep 1; }
Let's run.
./vfd_clock.pl | sudo microcom -s 9600 -p /dev/ttyUSB0
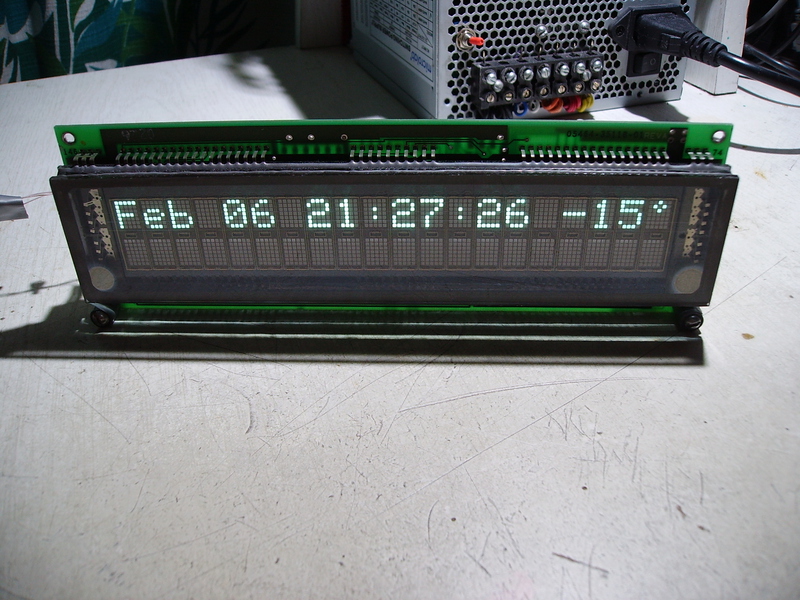
Here is my current version. With the display of LA, free memory, disk space and online on the minecraft server.

That's how you can make a beautiful garbage. Thanks for attention.
Documentation on the display.
My
control program .