Introduction
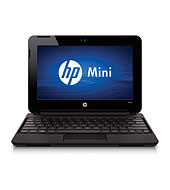
About a year and a half ago, I became the proud owner of the HP Mini 110-3155sr. The machine pleased everyone, but there was one problem that eventually got more and more boring - the lack of Home, End, PgUp, PgDown buttons. How I solved this problem with a small Python program - under the cat.
The decision comes to mind quickly enough. If there are no buttons, it means that you need to create some hot keys for them. I did not find how to do it using system tools, so I started sorting through various programs of this kind. Despite the fact that there are really many such programs, I did not find the one that would suit me. Some suggested executing commands on hotkeys, in others there was no convenient keyboard shortcut at all.
In the end, I decided to write a small utility with my own hands. In addition, it became a good idea as a “baptism of fire” in Python — I started learning quite recently, and it became interesting how quickly (if at all) you could do such a thing.
Formulation of the problem
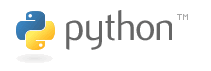
So, you need to write a program that will respond to keystrokes, and generate others. In my particular case, you need to generate clicks of all four buttons - Home, End, PgUp, PgDown. After sorting through a heap of possible combinations, discarding existing and important ones for me, I decided to dwell on the Alt + arrow combinations, namely:
- Alt + Left == Home
- Alt + Right == End
- Alt + Up == Page Up
- Alt + Down == Page Down
What do we use?
Python 2.7 - this version is fairly well supported, as I managed to make sure;
Python for Windows Extentions (build 216) - to use WinAPI;
pyHook 1.5.1 - a convenient wrapper for using system hooks;
py2exe 0.6.9 (optional) - to create a standalone-executable file.
')
Solution idea
The general idea is quite simple: hang up the system hook, track clicks. If the Alt + arrow is pressed, we generate the corresponding system message on pressing the button.
Gingerbread and rake
- There are several points that require special attention:
- The Alt key is distinguished from the others by the fact that if it is pressed, any other system message about a button being pressed will be “system”. This means that, directly from the arrow click message, we can find out if the Alt button is currently pressed or not.
- If we try to press the PgUp button while pressing the alto, nothing will happen. Similarly, with the generation of system messages. The solution is simple: programmatically “release” Alt before generation, and then “push” after.
- Pressing (keystroke) each button generates two messages - pressing (key down) and releasing (key up). It is necessary to distinguish them, otherwise for one keystroke there will be two simulations of pressing the button we need.
- The message processing function returns True or False, and depending on this, the message is either transmitted further along the chain of other system hooks or is not transmitted. This way you can control the flow of system messages.
Program code
Result
Such a program really simplified life. True, it works far from "perfect."
Firstly, the Alt button has lost the fullness of its functionality - now it will not work just to access the application menu by pressing it. However, the same Alt + F4 works fine. However, all the main system hotkeys are already busy, so I had to sacrifice something in any case.
Secondly, the following bug was found: the Alt + Tab combination works, but when the Alt button is released, the switch window does not disappear. So far I do not know how to fix it, I hope someone will tell.
findings
The global conclusion is “Python is cool” (remember, this is the first thing I wrote on python). Indeed, the necessary libraries are easily installed in two clicks. It's nice that you can easily use what has been written by others.
The smaller conclusion concerns directly pyHook - the package is quite convenient. Several ideas have already appeared on how to apply it:
- based on the movement of the cursor, try to determine (taking, for example, 1 pixel ~ 1 mm), what distance the mouse travels (per hour, per day, for the entire observation time), its average speed, etc .;
- write the usual keyboard keyhook in the output to some log file (I have long wanted to feel like a spy :));
- compile a frequency dictionary of his speech. Essentially the same keyhook, only combining individual letters into words, and counting their frequency. The idea of
stealing is inspired by a long-standing correspondence with Skiminok - he made a similar plugin for QIP.
UPD: lxyd suggested that for all of the above, there is an AutoHotkey program. The article came out in the style of “how I invented my own bicycle, which nobody needs”.