At HabraHabr, a new GTK blog has finally appeared. Join now! :-)Hi% username%!
The network has terrible rumors about this framework, but with a series of articles about it on HabraHabr, I will try to destroy the stereotypes that have been formed.
')
GTK + is a framework for creating cross-platform graphical user interface (GUI). Along with Qt, it is one of the two most popular libraries for the X Window System today.
Initially, this library was part of the GIMP graphical editor, but later became independent and became popular. GTK + is free software, distributed under the GNU LGPL and allows you to create both free and proprietary software.
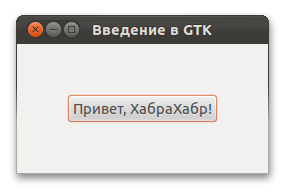
How it works
GTK + is written in C, but despite this, is object-oriented. You can also use wrappers for the following languages: Ada, C, C ++, C #, D, Erlang, Fortran, GOB, Genie, Haskell, FreeBASIC, Free Pascal, Java, JavaScript, Lua, OCaml, Perl, PHP, PureBasic, Python, R , Ruby, Smalltalk, Tcl, Vala.
Inside GTK + consists of two components: GTK, which contains a set of widgets (button, label, etc.) and GDK, which is busy displaying the result on the screen.
Appearance of applications can be changed by the programmer and / or user. By default, applications look native, i.e. just like other apps in this system. In addition, starting with version 3.0, you can change the appearance of elements using CSS.
Making "Hello, World"
For a start, let's take the following preparation as a basis:
#include <gtk/gtk.h> int main( int argc, char *argv[]) { gtk_init(&argc, &argv); gtk_main(); return 0; }
Please do not use single comments (//) if, like me, I decided to write in C.
Let's first create our application window. There are several types of windows in GTK, but we need the usual GtkWindow. Paste the following code into our blank:
GtkWidget *window; window = gtk_window_new(GTK_WINDOW_TOPLEVEL); gtk_window_set_title(GTK_WINDOW(window), " GTK"); gtk_container_set_border_width (GTK_CONTAINER(window), 50);
Before continuing, a few words about the packaging.
In GTK +, it is customary to place widgets not by coordinates, but to pack them into special containers. The window we created is also a container that can contain only
one widget. This is enough for us, but otherwise we would have to add a special widget container, which can be a grid, as well as horizontal or vertical fields. But I will tell you more about this in the next topic.
While we stop at the window. Let me remind you that the window is a container to which we have specified the border thickness of 50 pixels. What does it mean?
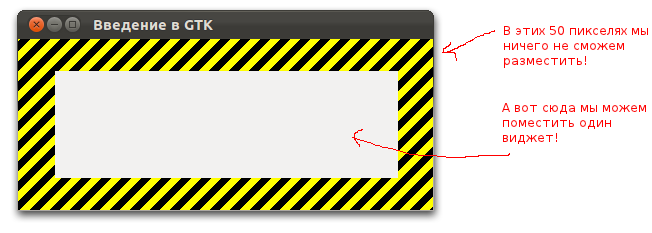
This means that we create a kind of invisible frame around this container and therefore we will not be able to place anything in this area.
Now consider the signals.
We can associate a specific event that should occur with the widget with its function.
g_signal_connect(G_OBJECT(window), "destroy", G_CALLBACK(gtk_main_quit), NULL);
In this case, this is the standard gtk_main_quit
gtk_main_quit()
, which safely completes our application.
Now we will create a button, by clicking on which a window will appear with the inscription “And hello to you,%% username!”.
A button (GtkButton) is also a container, which can also contain one widget. In order not to complicate the code by creating a label and placing it in a button, let's do it like this:
GtkWidget *button; button = gtk_button_new_with_label(", !"); gtk_container_add(GTK_CONTAINER(window), button); g_signal_connect(GTK_BUTTON(button), "clicked", G_CALLBACK(welcome), NULL);
It will look like this:
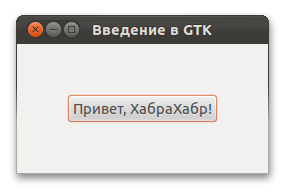
Now the implementation of the
welcome
function:
/* */ void welcome (GtkButton *button, gpointer data) { /* */ GtkWidget *dialog; GtkWidget *label; GtkWidget *content_area; /* */ dialog = gtk_dialog_new_with_buttons(" LOL!!!111", NULL, GTK_DIALOG_MODAL | GTK_DIALOG_DESTROY_WITH_PARENT, GTK_STOCK_OK, GTK_RESPONSE_ACCEPT, NULL); /* , */ content_area = gtk_dialog_get_content_area(GTK_DIALOG(dialog)); /* */ label = gtk_label_new("\n\n , %username!%"); gtk_container_add(GTK_CONTAINER(content_area), label); gtk_widget_show(label); /* */ gtk_dialog_run(GTK_DIALOG(dialog)); /* */ gtk_widget_destroy(dialog); }
Result after clicking:

So simply and quickly we created a work program. In the following topics I will talk about using Glade (a program to draw widgets without code) and creating your own widgets.
Compilation
gcc file_name.c -o file_name `pkg-config --cflags --libs gtk+-3.0`
Do not forget to install the GTK for the developer (ends with "-dev").
Anything else?
Yes, perhaps, here are some links:
www.gtk.org - official site
www.gtkforums.com - English forum dedicated to GTK
developer.gnome.org - information for developers under the GNOME environment. It contains a lot of useful information about GTK, including in Russian.
UPD: Full PasteBin example code -
pastebin.com/iPttWBne 