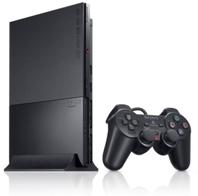
When I started writing this article, I realized that the chapters will be released on the controller more:
This is the joystick itself, the second joystick, vibration and Multitap (a device for connecting 4 joysticks in one port) - I have it.
For the joystick itself, there are two libraries, each of which provides its own information.
I use 2 original Dual Shock 2 joysticks, however, I have two non-original joysticks (they are used to connect to the computer through a special device or through a multitap for four of us), which I will mention separately.
')
Each controller has a so-called terminal controller - this is the actual port through which you access the controller.
To control the controllers there is a special library libpad, which provides data from the buttons, changing the mode of the controller and vibration.
There is also a “regular” libmtap library. Both libraries can work with multitap.
The library supports the following controllers from PS1 and PS2:
Regular digital controller (no analogues):
ID = 4 ;
Dual Shock 1 and Dual Shock 2:
ID = 7 ;
NeGcon controller:
ID = 2 ;
Normal analog joystick:
ID = 5 ;
Namco pistol :
id = 6 ;
Jog controller and fishing controller (here, apparently, includes a guitar):
Spec. extended id .
About the rest I will replenish information along the way.
Port and slot
The concepts port and slot are different things in PS2.
The port points to the main controllers - the ones for the first and second joysticks on the PS2 itself.
The slot is used most of the time when you have a MultiTap. Multitap needed to connect multiple joysticks in one port (maximum - 4). Usually in games this is displayed as Joystick 1A, Joystick 1B, etc.
If you do not have a multitap, then, in principle, you should not bother: port 0, slot 0 is the first joystick, port 1, slot 0 is the second joystick.
With multitap it will be different: if you have, say, a multitap is inserted into the second port and a joystick is connected to it in slot A, then you need to access it using port 1, slot 0.
This can be seen in sufficient detail in the image:
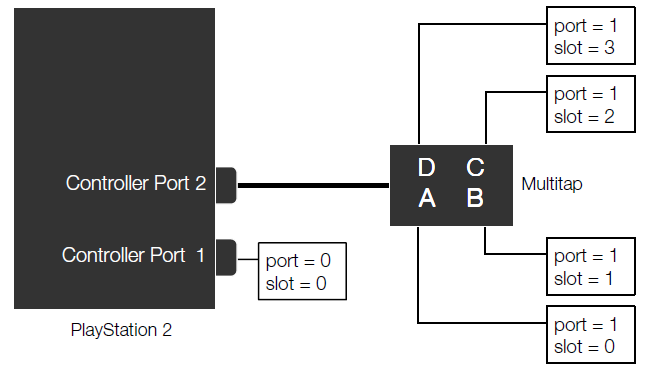
For the future I will say that there is also work with memory cards.
Joystick work
To use libpad, you need to connect the library “libpad.a” and the header file “libpad.h”.
To start, use the “sceSifLoadModule ()” function during the application start.
The “–lpad” parameter should be added to the makefile.
Basic procedures for using controllers:
scePadInit () - library initialization. The parameter is 0.
scePadPortOpen () - opening the controller port. Returns Its parameters are: port, slot, buffer to be used. Must be 64 bytes. Declared as:
static u_long128 pad_dma_buf[scePadDmaBufferMax] __attribute__((aligned (64)));
Where scePadDmaBufferMax is a constant that should not be declared.
Returns an int function by which you can check that the joystick is inserted - if it is not there, then the parameter will be 0;
Next, the library begins to automatically communicate with the controller when the controller is on and open. Further there are two functions:
scePadRead () - used to read buttons.
The function has 3 parameters: port and slot and buffer to save the result.
The buffer is unsigned char buffer [32];
The function also returns the result. And if it is equal to zero, then the reading has stopped.
Then we just need to read the buffer, although in fact it is not easy.
scePadGetState () - with it you can check whether the controller is in a calm state. Accepts the parameters of the port and slot.
Returns the status of the controller. If the status is greater than and equal to zero, and also less than or equal to 7, then something has happened.
The scePadPortClose () function is used to close the controllers, where the parameters are port and slot, and after scePadEnd () it turns off the library. Usually these two functions are not used.
I will add that all these functions are also used to read analogues, only if the mode is on.
The most banal example would look like this:
main(){<br> unsigned char rdata[32];<br> u_long128 pad_dma_buff[scePadDmaBufferMax] __attribute__((aligned(64)));<br> scePadInit();<br> scePadPortOpen(0, 0, pad_dma_buff);<br> while (1) {<br> scePadRead(0,0,rdata);<br> // <br> }<br> scePadPortClose(0,0);<br> scePadEnd();<br>} <br> * This source code was highlighted with Source Code Highlighter .
Work with DualShock and button touch sensitivity
First you need to turn on the analog mode with the scePadSetMainMode () function. Accepts parameters port, slot, 1 and 0. Returns the result as 0 or 1, which means it is not included or enabled, respectively.
To ensure that the controller is indeed DualShock, you need to call the scePadInfoPressMode () function. Takes parameters of the port and slot, returns the result in the form of 0 or 1.
The inclusion of the mode itself is done by the scePadEnterPressMode () function. Accepts parameters and returns as well as the previous function.
Further, through the scePadRead () function in the buffer, we receive an additional one byte, in which the degree of pressing of the buttons is displayed from 0 to 255. Where 0 is equal to the fact that the button is not pressed.
I will say, note that my first joystick sometimes shows the very force of pressing up to 3.
According to the specification, a completely normal movement error of the analog is plus or minus 25.
Using the scePadExitPressMode () function, you can exit the key sensitivity mode. It takes the port and slot. You may need to switch to the menu.
If the user during the sensitive mode presses the analog button, he will switch to the normal digital mode without sensitivity. Pressing the analog button
again will not switch the joystick back to sensitivity mode.
Vibration
Vibration in the joystick is quite simple and at the same time not.
You can connect to it a separate library, and you can use only two functions that are included in libpad.
I will tell you about another vibration library next time, and here I’ll talk about rather simple two functions, these are scePadSetActAlign () and scePadSetActDirect (), which accept a port, a slot and 6 bytes of twisting.
In the first byte, you specify a strong motor or a weak motor.
A weak motor takes in the second byte the values ​​0 or 1, which means stop or torsion. A strong motor takes in the second byte a value from 1 to 255 or 0, which means torsion force or stop.
The scePadSetActDirect () function returns true or false, indicating that the vibration operation has been performed. The remaining bytes are preferably filled with the value 0xff if you do not use them.
After that, the user's joystick will receive 10 current units for vibration operation.
To increase the number of current units to 20, simply fill in the remaining values ​​of 0x00.
Important!You need to know that each port is limited to a maximum number of current units equal to 60. If you try to give more than 60, then the port can be blocked before the console reboots (the internal limiter works).
Thus, if I have a multitap, and I give 20 units of current to joysticks 1A, 1B and 1C, then I cannot do this to the 1D joystick, otherwise I can block the port. Therefore, I do not advise you to use an increased current supply.
I also add that these functions cannot be used for long vibrations.
Asynchronous functions
The following functions are asynchronous. They may not return the result to you at the same time. In this case, you can get the result of their execution through the scePadGetState () or scePadGetReqState () functions.
scePadSetMainMode - switching input modes;
scePadSetActAlign - vibration function;
scePadEnterPressMode - enter button touch sensitivity mode;
scePadExitPressMode - exit from button touch sensitivity mode.
Closer to the end
In general, in principle, the application will continue to work with the port and slot, even if the joystick is removed (turned off). Programmatically check that the joystick is deleted can be done using scePadGetState (), which will return scePadStateDisconnect.
Before each frame, it is advised to check the state of the controller so that there is no situation when the joystick is accidentally turned off.
Also, depending on the version of the DualShock or DualShock 2 controllers, the specification of analogs may change and you should not hope for the automatic calibration of the joystick. Therefore, most often it is necessary to make corrections to the fact that the analog has not stopped at the point 127x127.
That's all. See you in the next, more detailed article about joysticks.
PS : Seeing the relevance of the topic, I wrote this article half the night and morning. If the SDK is ready for the next article, then instead of the joysticks there will be a description of the installation.
Update : The scePadInit () function is needed only in the use of EE, which I am talking about. IOP initializes the library itself.