In this article I want to describe how to create a minimal application that publishes something in the timeline, as well as tell about the difficulties that may arise along the way.
First of all, it should be said that at the moment facebook timeline is in beta and is available only to developers, so the first thing to do is activate the timeline for your account. Many instructions have been written on this topic, for example
www.makeuseof.com/tag/enable-facebooks-timeline-profile .
After completing all the actions from the instructions, and after waiting a few minutes, click on your name, the long-awaited timeline should open instead of the wall.
Step 1. Customize facebook app
This is the most important step, it is there that unforeseen complications can arise. Having created the application (https://developers.facebook.com/apps), first of all we specify “App Domain” in the form of test.com or news.test.com. Further, in the section "Website" you need to fill out a link to your website, already in the form of
news.test.com . Then go to the “Advanced” settings and make sure that “Sandbox Mode” is set to “Disabled”. If you do not disable the sandbox mode, you will encounter errors with the login, for example, when calling the getLoginStatus API function with a request for publication rights, the callback will not work.
Go to the section "Open Graph", and add the action read article, clicking "get started" we will proceed to the setting of the verb read, everything is intuitively clear, and there are no pitfalls. Save and proceed to setting up the object. The next page will be the “Aggregations” setting - an important enough setting, for each verb created, aggregation must be configured. Otherwise, facebook will react a little unobviously - when you try to request permission for publish_actions, a dialog opens that prompts you to log in to your application, but there will not be a word about publications in the timeline and the corresponding permissions will not be obtained. Having set up the aggregation, you need to make sure that there are “thumbnails” for it, without them the error with the auth dialogue can repeat. In the Open graph -> Aggregations settings, click preview and make sure that the aggregation has a thumbnail (if there is none, you can add it in the dialog that opens). To create facebook thumbnails, you need to feed a link to the sample article. How to create the right “article” will be discussed below.
Step 2. Create an object that will be published on the timeline
Under the object, there is a view to the usual web-page accessible from the Internet. Suppose we want to publish information about an article read by a user, that is, to place a note on a “read article” on the timeline, which means that our page will be an article. How the publishing process takes place - using the facebook API in timeline, a link to the object is sent (a web page with the article), then facebook processes this page and creates an object in its graph. In order for facebook to properly process our article, it must contain the correct open graph tags. Here is an example of the "correct page"
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta property="fb:app_id" content="{id }"/>
<meta property="og:type" content="{namespace }:article"/>
<meta property="og:url" content="http://{ }/Article.html"/>
<meta property="og:title" content="article about article"/>
<meta property="og:description" content="cool article"/>
<meta property="og:image" content="{ }"/>
<title>Article</title>
</head>
<body>
<h2>just article about article</h2>
Some text ...
</body>
</html>
')
Check the page for the correctness of the open graph tags, you can use the utility facebook debugger.
Having created the correct object that can be published in the timeline, set up a preview for the aggregations (see the instructions in the previous paragraph).
Having entered the open graph settings and set up a preview, the dialog for adding thumbnails will look something like this

Step 3. We request publication rights in the timeline.
Here is the minimum code to enable the FB API and request publishing rights on the timeline:
<html>
<head>
<title>log in</title>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js" type="text/javascript"></script>
</head>
<body>
<!-- Fb API Initialisation -->
<div id="fb-root"></div>
<script>
window.fbAsyncInit = function () {
FB.init({
appId: '{fb app id}', // App ID
status: true, // check login status
cookie: true, // enable cookies to allow the server to access the session
oauth: true, // enable OAuth 2.0
xfbml: true // parse XFBML
});
};
// Load the SDK Asynchronously
(function (d) {
var js, id = 'facebook-jssdk'; if (d.getElementById(id)) { return; }
js = d.createElement('script'); js.id = id; js.async = true;
js.src = "//connect.facebook.net/en_US/all.js";
d.getElementsByTagName('head')[0].appendChild(js);
} (document));
</script>
<script type="text/javascript">
$(document).ready(function () {
$("#post").click(function () {
FB.login(function (response) {
if (response.authResponse) {
console.log('logged in');
} else {
console.log('failed.');
}
}, { scope: 'publish_actions' });
});
});
</script>
<input id="post" type='button' value='post' />
</body>
</html>
Clicking on the button will open a dialog in which it will be written that this application is going to write in your timeline, here is an example:
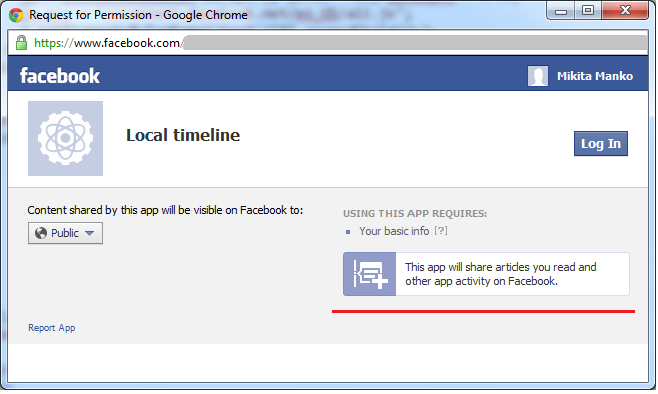
If there is no underlined request, then the application is configured incorrectly (in this case, you should re-read the previous steps, and make sure that for all the "verbs" there are "aggregations" with sliders).
You can delete the application in Account Settings -> Apps, you can check there and what rights are granted to the application.
Step 4. Publish in timeline
Let's add the necessary api call and it will turn out:
<html>
<head>
<title>log in</title>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js" type="text/javascript"></script>
</head>
<body>
<!-- Fb API Initialisation -->
<div id="fb-root"></div>
<script>
window.fbAsyncInit = function () {
FB.init({
appId: '{your fb app id}', // App ID
status: true, // check login status
cookie: true, // enable cookies to allow the server to access the session
oauth: true, // enable OAuth 2.0
xfbml: true // parse XFBML
});
};
// Load the SDK Asynchronously
(function (d) {
var js, id = 'facebook-jssdk'; if (d.getElementById(id)) { return; }
js = d.createElement('script'); js.id = id; js.async = true;
js.src = "//connect.facebook.net/en_US/all.js";
d.getElementsByTagName('head')[0].appendChild(js);
} (document));
</script>
<script type="text/javascript">
$(document).ready(function () {
$("#post").click(function () {
FB.login(function (response) {
if (response.authResponse) {
console.log('logged in. Try to publish in timeline');
var verb = '{your_app_namespace}:read';
var objectType = 'article';
var permalink = link to article';
FB.api('/me/' + verb + '?' + objectType + '=' + permalink, 'post', function (result) {
console.log('published with id = ' + result.id);
});
} else {
console.log('failed.');
}
}, { scope: 'publish_actions' });
});
});
</script>
<input id="post" type='button' value='post' />
</body>
</html>
In the callback comes the result of the publication, with id in facebook graph (the created record can be deleted, for example). If the inscription "'published with id ..." is displayed in the browser console, then everything is set up correctly. To see the publication you need to click on your login (top right), the timeline will open, then you can click Activity log (for more convenient viewing of the added actions). With the next publication, several “read articles” will be grouped into one aggregation. At the timeline itself, it looks like this:
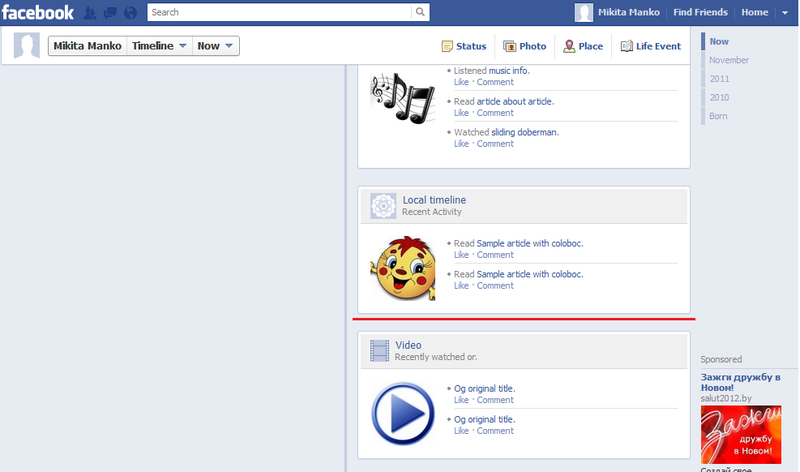
In the FB API call there is a verb - verb, in this case it is the namespace of your application, then a colon and a verb. If, when creating a verb (for example, watch), facebook prompts you to choose from existing ones, an original verb will be created that is not tied to your application, in which case the verb should be equal to neither “namespace: watch” but “video.watches”, you can find out clicking get code in the open graph settings of the application.
If everything works, then you should check the work in IE, at least in IE 8. Surprisingly, even this code, taken from the facebook instructions, refused to work in IE (with commented out console.log). At the time of this writing, the error in the FB plugin in IE was not reproduced, but if you have a problem with permissions in IE, here is a useful link
gkirok.blogspot.com/2011/11/ie-facebook-connect.html .
Perhaps, I forgot to mention some rakes, which I became myself, but basically they are all solved very simply - we drive a mistake into Google and are looking for an answer to stackoverflow. If fb.getloginstatus is called and nothing happens (even there are no errors), we go to the network tab and look at the answer from facebook and drive it into Google again.
In addition, you should not forget that you can only request publish_action from administrators / developers / testers of your application, this situation will continue while the facebook timeline is in demo. You can send someone an invitation to become the admin / developer of your application from the configuration of the “roles” in the application itself.
Happy all!