Introduction

This article discusses the process of writing programs using SL4A on the example of a program to synchronize files via FTP in Python in the Ubuntu operating system. It shows the configuration of the operating system for developing SL4A applications, writing the application itself, distributing the application using barcodes, and packaging the application into an .apk file.
Few words about what SL4A is and what it is eaten with
The SL4A project (Scripting Layer for Android), which was born thanks to Damon Kohler and Google’s
20% of free working time , makes it possible to program under the Android platform in various interpreted programming languages ​​(currently Python, Perl, JRuby, Lua, BeanShell, JavaScript, Tcl and Shell).
On Habré already written about SL4A. For example,
here it was described how to write an application in Python to check karma for Habré, and
here with the help of Lua a script was created that disables the phone’s communications for the night.
SL4A is well suited for teaching programming, for writing prototypes of programs, for solving tasks of automation of actions on the Android platform. Large serious programs are not written on SL4A, but it fits well for creating mini-programs.
Check karma on a habre, send a text message to my wife, to ask him to start warming up dinner
a couple of kilometers from home ; waking up in an electric train when approaching the required station, or even
controlling a real rocket - all this can be implemented with the help of SL4A with a minimum amount of code and elapsed time.
For comparison, the simplest program in Java and Python is shown (examples are taken from the presentation of
PyCon 2011 ):
package com.spodon.pycon; import android.app.Activity; import android.app.AlertDialog; import android.content.DialogInterface; import android.os.Bundle; import android.widget.EditText; import android.widget.Toast; public class Demo extends Activity { private EditText mEditText = null; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Hello!"); builder.setMessage("What is your name?"); mEditText = new EditText(this); builder.setView(mEditText); builder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { Toast.makeText(Demo.this, "Cancelled", Toast.LENGTH_SHORT).show(); } }); ...
The same Python program takes only 4 (!) Lines:
import android droid = android.Android() name = droid.getInput("Hello!", "What is your name?") droid.makeToast("Hello, %s" % name.result)
The difference, as they say, is obvious. Of course, not without drawbacks. About them at the end of the article.
')
Main part
Installing SL4A on the phone
So, let's start writing the synchronization application itself.
To use SL4A, you need to install an application on your phone. You can take it
from here or from the barcode:
Directly in the SL4A application there is only a Shell interpreter. To use other programming languages, you must install additional applications. The Python interpreter is
here .
Configure the operating system to work with SL4A
For the file synchronization application to work, an ftp server must be installed on the computer. For Ubuntu, there is a vsftpd application, which is a free and easy to configure ftp server. To install, just enter the following command in the terminal:
sudo apt-get install vsftpd
The server settings file is located in /etc/vsftpd.conf, in it you need to add permission to write files by uncommenting the line
#write_enable=YES
And restart the server:
$sudo service vsftpd restart
SL4A applications can be created directly on the phone, but it’s extremely tedious even for small applications to learn how to dial a code on the virtual keyboard, it’s much easier to write applications on a computer and then download them to the phone.
To run SL4A applications written on a computer, you must first start the SL4A server on your phone (on the SL4A application home page, click Menu - Interpreters - Menu - Start Server). We will launch a private server. A notification will appear that the server is running, and there it will be written what port the server uses.
Comment: There is a choice of starting a public server. When starting a public server, anyone who will have access to your IP address will be able to control your phone. When starting a private server and setting environment variables as shown below, the scripts running on the computer will work without changes on the phone. For the private server to work, it is necessary that the
Android SDK is installed.
Redirect all local traffic arriving on port 9999 to the Android device (assuming the server is listening on port 46136):
$ adb forward tcp:9999 tcp:46136
It remains to create an environment variable and the configuration is complete:
$ AP_PORT=9999
It remains to add the
android.py file to the folder with the Python libraries, and now you can write applications on your computer, run them, and you can see the result immediately on your phone. To run helloWorld on an Android device, it is now enough to enter into the Python interpreter:
>>>import android >>>droid = android.Android() >>>droid.makeToast("Hello, world!")
The first line imports the android library, then creates a droid object, with which the Android API is used. The last line displays the message “Hello, World!” On the device screen.
Now is the time to get to know the
API that SL4A provides.
Source code of the program
The program synchronizes files in selected folders on your Android device and computer in the background. Checking the appearance of new files occurs every 30 seconds. Most of the program is practically no different from a personal computer application with similar functionality. For clarity, when exception handling is omitted.
With the help of the already known droid.makeToast (), the synchronization progress messages are displayed on the Android device screen.
Application launch
After the script has been edited, you can put it into your Android device using the command
$ adb push ftp_sync.py /sdcard/sl4a/scripts
To launch the script from a computer, it is convenient to create an alias that runs the application on the phone. To do this, add a line to ~ / .bashrc:
alias andrun='adb shell am start -a com.googlecode.android_scripting.action.LAUNCH_FOREGROUND_SCRIPT -n com.googlecode.android_scripting/.activity.ScriptingLayerServiceLauncher -e com.googlecode.android_scripting.extra.SCRIPT_PATH'
Then the script itself can be run with the command
andrun /mnt/sdcard/sl4a/scripts/ftp_sync.py
Application distribution
Distributing the application using barcode
A simple and original way to distribute small SL4A applications is to transfer them using barcodes. You can create a barcode with the application, for example,
here (select type - text, size - L). This is what our application looks like:
To install the script in the Android device, just run the SL4A application, press menu - add - scan barcode.
Packaging an application into a .apk file
SL4A scripts can also be packaged into regular .apk files. To do this, download the
template file of
the SL4A project and import it into eclipse. For unknown reasons, the project initially lacks the gen folder. It must be created manually in the root directory of the project. After that, in Windows - Preferences - Java - Build Path - Class Variables, create a variable ANDROID_SDK that points to the folder where the Android SDK was installed.
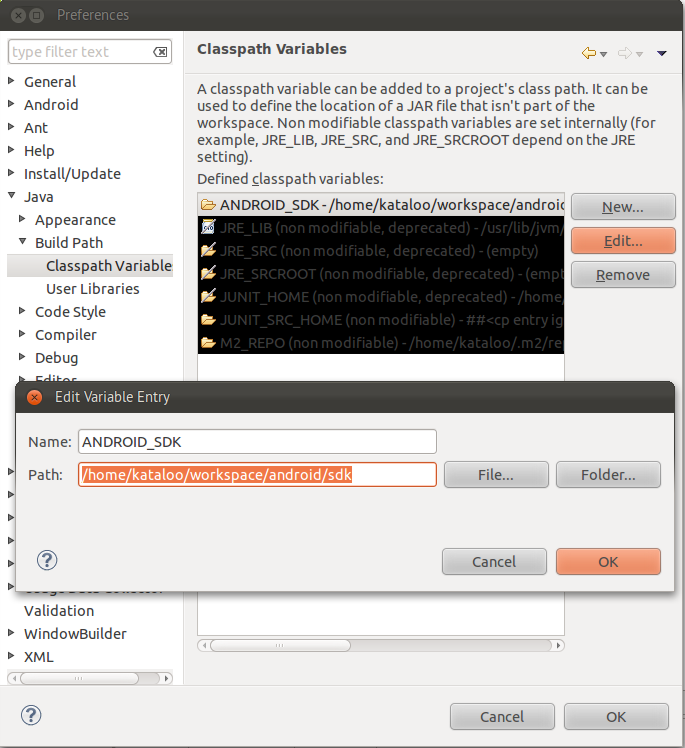
Next, in the res / raw folder, we replace the contents of script.py with the contents of our program. Then click Project-Build in the menu, and if everything went fine, we are ready to build the application into an .apk file. Go to File - Export, choose Android - Export Android Application, then select our project.
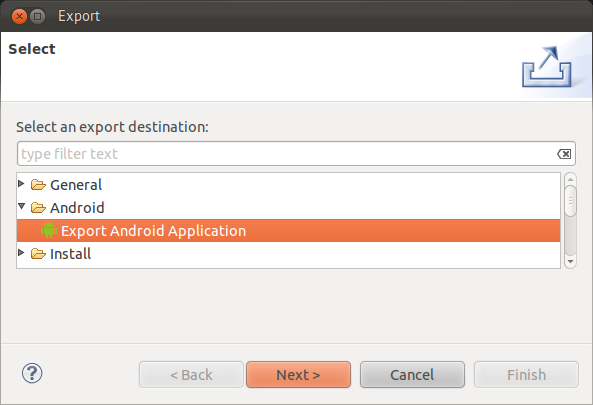
Every Android application must be digitally signed. If it was not previously created, you must create it using the dialog box. The last dialog box asks where to save the generated .apk file. Install the application on the phone:
$ adb install ftp_sync.apk
You can rename the script by selecting in the src com.dummy.fooforandroid directory and clicking Rename in the Refactor menu. The name must contain at least two points. After that, you need to change the name of the application in AndroidManifest.xml. Application icons are res / drawable. If desired, they can also be changed.
The GUI interface for SL4A is created using HTML markup and webView.
Here is an example of creating such an interface.
Conclusion
Creating applications using SL4A for people who are not familiar with Java and mobile development for Android, is not particularly difficult. The Android platform is remarkable for its openness and availability. Including programming for it. SL4A brings a new level of accessibility to the development of Android applications, making it possible to use various programming languages.
Disadvantages SL4A
- SL4A is currently only in the alpha version stage, now there are quite a few bugs
- the ability to create a GUI is limited by webView tools (html markup) and standard dialog boxes. As kAIST suggests, starting with version r5 (for the time being unofficial), there is another possibility to create a GUI interface - FullScreenUI
- performance of applications using SL4A significantly loses to applications written in Java
- limited API capabilities
Links
Android-scripting project code.google.com on code.google.com
SL4A and Python
Pro Android Scripting with SL4A: Writing Android Native Apps Using Pythonpython for SL4AAPI description