Wandered on the Internet in search of a decent manual. I found, in my opinion, decent, but, as usual in Android programming circles, it is, of course, in English. So I decided to contribute to the distribution of manuals in this environment in Russian. I will study it and, in passing, translate here.
Let me remind you that the article is a translation of
this article with the permission of its author.
The continuation of the article is
here . It discusses customization lists and tips for optimizing them.
The manual is written under Android 4, Eclipse 3.7, Java 1.6. The source can be found
here .
')
Lists are a common thing in modern mobile devices. We can navigate through the list and select the item we need to open something. And Android has it.
ListActivity
You can directly use ListView in your project, just like any other user interface component. If the main goal of your Activity is to display a list, then you can extend the Activity class with the ListActivity class, which will greatly simplify the use of ListView.
ListActivity extends Activity to simplify the use of lists. For example, you already have a predefined method that handles clicking on a list item.
ListActivity contains a ListAdapter responsible for content management. The adapter must be set in the onCreate () method of your activity via the setListAdapter () method.
When you click on a list item, the onListItemClick () method is called. This method allows you to access the selected item.
Android already has some predefined templates for use in Adapters, for example, “android.R.layout.simple_list_item1”. If you do not want to use a predefined template, your template should have an identifier "@android: id / list", which will be called a ListView. For example:
<ListView android:id="@+android:id/list" android:layout_width="match_parent" android:layout_height="wrap_content" > </ListView>
You can also use the view with id "@android: id / empty". This view is displayed when the list is empty.
Adapter
What is an adapter?
ListView takes content to display via adapter. The adapter is extended by the BaseAdapter class and is responsible for the data model for the list and for the location of this data in its elements.
There are two standard adapters in Android: ArrayAdapter and CursorAdapter. An ArrayAdapter manages data based on arrays or lists, while the SimpleCursorAdapter manages content from a database. You can also develop your own Adapter that extends these two classes or the BaseAdapter class.
The most important Adapter method is getView (). It is called for each item in the list to determine how and what data should be displayed in it. getVew () also contains the convertView parameter, which allows you to re-use an already existing list item that is no longer displayed, because the user scrolled it from the visible part of the display. If convertView is not empty, it can be used again to not load the template, which leads to a significant performance increase, because loading the XML file is quite resource intensive.
ListViews and performance
Displaying large amounts of data on a mobile device should be implemented as efficiently as possible. Therefore, the ListView creates views (widgets) as needed and reinforces them to the view hierarchy. The Standard ListView Adapter deletes views, for example, if an item is no longer displayed, it will be erased and only its contents will change. If you use your adapter, you should also consider this in order to avoid performance problems.
Example: Simple ListActivity
Now we will create as simple a list as possible. We use the standard class Adapter - ArrayAdapter and a view predefined by Android.
Create a new de.vogella.android.listactivity project with MyListActivity. Do not modify the main.xml template. Create the following Activity:
package de.vogella.android.listactivity; import android.app.ListActivity; import android.os.Bundle; import android.view.View; import android.widget.ArrayAdapter; import android.widget.ListView; import android.widget.Toast; public class MyListActivity extends ListActivity { public void onCreate(Bundle icicle) { super.onCreate(icicle); String[] values = new String[] { "Android", "iPhone", "WindowsMobile", "Blackberry", "WebOS", "Ubuntu", "Windows7", "Max OS X", "Linux", "OS/2" }; ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, values); setListAdapter(adapter); } @Override protected void onListItemClick(ListView l, View v, int position, long id) { String item = (String) getListAdapter().getItem(position); Toast.makeText(this, item + " ", Toast.LENGTH_LONG).show(); } }
Notice that we do not use setContentView (). ListActivity uses the ListView template by default if no other template is specified explicitly.
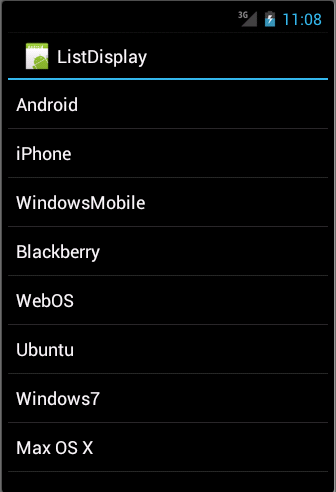
By pressing one of the elements of the list, a standard “Toast” will be displayed with information about which element was selected.
I apologize for the repost. Initially not noted as a translation. Many thanks to
jeston for the tip, accepted and learned from mistakes.