
This post is the beginning of a series of articles on optimizing and improving the performance of GWT applications. Since I have accumulated quite a lot of material, I decided to split it into 2-3 parts.
Let us proceed to the description of what awaits us in the first article.
Issues addressed
In the first part of a series of articles on optimizing GWT applications, I’ll tell you about the following things:
- Separation of client code, on-demand loading
- Getting rid of the use of heavy classes on the client
- Resource caching
- GWT RPC performance issues using REST
- Features of the impact of layout on the application
- Transmission Optimization
- Using Scheduler
- Using dispatchers to aggregate server requests
And now in detail about each of the points mentioned.
Separation of client code, on-demand loading
GWT has a built-in mechanism that allows the developer to "cut" the application into parts. It is necessary, first of all, for the following purposes:
- Reducing the initial size of the loaded data
- Using the principle of deferred loading - the necessary data will be loaded by the application as needed
To divide the client code into parts, use the GWT.runAsync method call.
An example of using runAsync:
')
GWT.runAsync(new RunAsyncCallback() { public void onSuccess() { new MySettingsDialog().show(); } public void onFailure(Throwable ohNoes) {
In this case, the GWT compiler takes on the responsibility not only to do work on the separation of client code, but also to analyze how to cluster the code. It is guaranteed that the separation will be correct. However, separation may not occur. The reason for this may be cross-references in the code.
In the GWT Can Do What presentation with Google I / O, you can see a comparison of the download speed of the application before and after cutting it into pieces using runAsync:
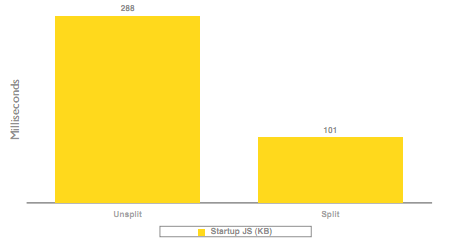
Thus, using runAsync can seriously reduce the initial application load time and reduce the amount of data transferred to the client.
It is worth paying attention to the class AsyncProxy. Probably, I will give his description in the second part of the article; now you can familiarize yourself with it by its
documentation .
Getting rid of the use of heavy classes on the client
On the Google Web Toolkit group page, you can find a
discussion on how to reduce the size of a compiled GWT application. In particular, the approach is noted, which consists in the rejection of the use of "heavy" classes on the client side, which entail the inclusion of a large amount of code on the client side.
First of all, you can use the example of using the Comparator on the client. Using it entails the inclusion in the client part of the ~ 10 KB code associated classes.
Using RequestFactory leads to a further increase in the client - in the discussion mentioned above, the client part increased by 150 Kb.
It should also be careful in the case of using external modules - it is possible that their inclusion in the project will entail a significant increase in the client part due to the addition of many other classes.
Resource caching
HTTP requests from the client side are significant limitations to the performance of the client side of the application. If the application requests each resource separately, the charge for downloading all resources will be large.
To prevent client application from slowing down, a resource caching approach is used.
GWT has a mechanism called ClientBundle. ClientBundle caches various types of resources (text, graphics, CSS, and others). You can read about ClientBundle
here .
Advantages of the approach used in ClientBundle:
- The required resource will be loaded once instead of being loaded each time it is used.
- The total amount of stored resources will be less
How does minimizing resource storage in the case of ClientBundle?
Let's return to the GWT Can Do What presentation. In it we can see the following illustration:
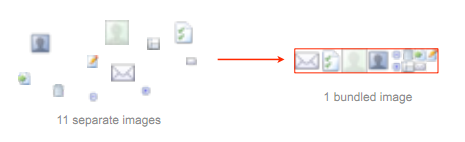
Thus, overhead is reduced to store data of the same type.
How can resources be reduced as a result?
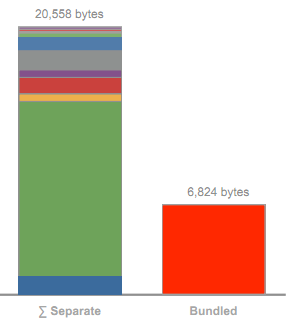
GWT RPC performance issues using REST
GWT RPC is a really convenient tool. Providing an interface for organizing client and server code for a service, having support from IDE tools, it is one of the amazing features of building AJAX applications in GWT. However, it is worth considering whether it suits you.
The main disadvantages of using GWT RPC are the addition of too much redundant data to the request and the complexity of debugging.
As a solution to these problems, use of REST can be noted. In the
article from Zack Grossbart you can find an example of using REST for GWT projects.
At the same time, his
article 4 More GWT Anti-patterns highlights the main advantages of REST:
- REST makes the developer think about the API. The API can also be provided to external services and applications, adding additional convenience and functionality for interaction.
- Convenience Testing
- Clear separation of client and server
Features of the impact of layout on the application
Very often, gwt developers use an auto-fit layout. Examples of this are the use in components height = "100%". It is worth saying that ontentHeight, offsetHeight are heavyweight operations. Below is the statistics for the execution time of the offsetHeight operation in IE, taken from the Measure in Milliseconds presentation with Google I / O 2009:

Not only is the result of the operation poorly predictable; its peak value for 18 measurements reached a value of about 85 ms.
Certain time is taken by the full processing of the resize event. Often, the resizing operation in the forced layout case takes time several times longer than the style recalculation.
How can you get rid of this problem? Must use CSS. Layout defined with CSS will be rendered much faster, depriving us of having to call heavyweight methods such as offsetHeight for the entire DOM hierarchy of components.
You can use the updated DockPanel, not using javaScript during resize.
It is not recommended to create and store large trees of embedded widgets. Widgets have an additional overhead, which negatively affects the memory used and the size of the client (as a result, and speed). As a solution, you can use HTMLPanel. To track the number of widgets on the pages there is an InspectorWidget tool. About the properties of the plugin can be found on its
page .
Transmission Optimization
Objects being serializable are constantly transmitted between the client and the server. The developer must ask himself - are only the most necessary data transmitted? Large object graphs, nested sub-objects, and unnecessary parameter sets take precious time and create an extra load. Transfer only what you need on the client. Often - just what the user sees. Everything you need will be available if necessary in the data. Of course, this approach should not contradict the policy of caching resources on the client side.
Using Scheduler
GWT has a deferred call mechanism. Previously, it was called DeferredCommand, but was declared deprecated. Now the mechanism is called Scheduler.
Scheduler actually provides a class for asynchronous execution of a certain action. It is guaranteed that the action will be performed after the end of the event loop browser.
Benefits:
- Does not block the thread of execution.
- Allows you to first finish all UI events.
Quite often, Scheduler is used for late construction of some objects. However, its proper use will in some cases make the application UI faster due to more prioritization of UI events.
Using dispatchers to aggregate server requests
Each call to the server imposes a certain delay in execution. If we need to make frequent multiple calls to the server, we can aggregate them into some batch units.
Starting with version 2.3, the GWT feature has been introduced using RequestFactory:
requestContext.method1().to(new Receiver<T>(){...}); requestContext.method2().to(new Receiver<T>(){...}); requestContext.fire(new Receiver<Void>(){...});
The advantages of this approach are obvious - the number of calls is significantly reduced, and, as a result, the execution delay.
In case we can cache server responses, we can use caching dispatchers. You can learn about their use by clicking on the
link .
Finally
GWT is a powerful tool that allows you to create an excellent cross-browser interface and is widely used already outside of Google. Nevertheless, some of its most convenient tools impose serious limitations on the performance of the application. You must carefully optimize the speed of the application, so that users are also satisfied with the work with it.
In the next article I plan to continue to talk about the features of improving performance and optimizing GWT applications. In particular, ways to improve the speed of GWT RPC, JS Shrink, features of applying native code on the client, tips for reducing the size of the script will be considered; It is also planned to talk about the optimization of compilation speed and give some tips for the interface layout.
Thanks for attention!
Useful resources:
- static.googleusercontent.com/external_content/untrusted_dlcp/www.google.com/en//events/io/2011/static/presofiles/drfibonacci_devtools_high_performance_gwt.pdf
- dl.google.com/io/2009/pres/W_1115_GWTCanDoWhat.pdf
- dl.google.com/io/2009/pres/W_1230_MeasureinMilliseconds-PerformanceTipsforGoogleWebToolkit.pdf
- turbomanage.wordpress.com/2010/07/12/caching-batching-dispatcher-for-gwt-dispatch
- www.zackgrossbart.com/hackito/antiptrn-gwt
- www.zackgrossbart.com/hackito/antiptrn-gwt2
- www.zackgrossbart.com/hackito/gwt-rest
- habrahabr.ru/blogs/gwt/99614