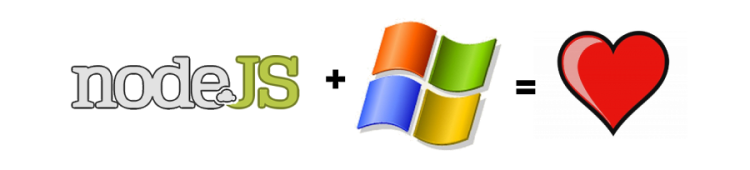
In this article we will discuss the most important questions for novice web programmers and those who are thinking about studying Node.js, namely:
- how to install the Node.js work environment on your computer;
- how to write code;
- how to debug;
- how to deploy what happened on a remote server.
And the performance tests at the end of the article may give an answer to the question of why you may actually need to study Node.js.
Node.js is an event-oriented Javascript framework for creating network applications. The basic idea is that during the execution of the code, nothing is blocked - there are no operations that are waiting for something, such as transferring data, user input or establishing a connection. Everything is built on events that occur at the moment of the onset of what synchronous operations are waiting for. This gives a significant, sometimes tenfold, performance advantage compared to old synchronous systems. Since version 0.6.0, which was released in November 2011, the Node.js build for Windows has been declared stable.
Install the working environment
')
First you need to download and install the
Web Platform Installer , run it, click on “Options” and in the “Display additional scenarios” field add a link to Helicon Zoo Feed:
http://www.helicontech.com/zoo/feed/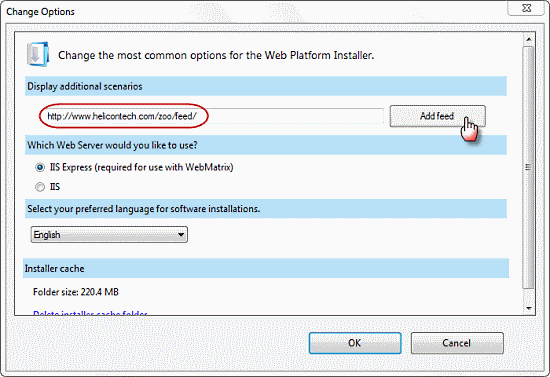
After that, the Zoo tab will appear in the Web Platform Installer:
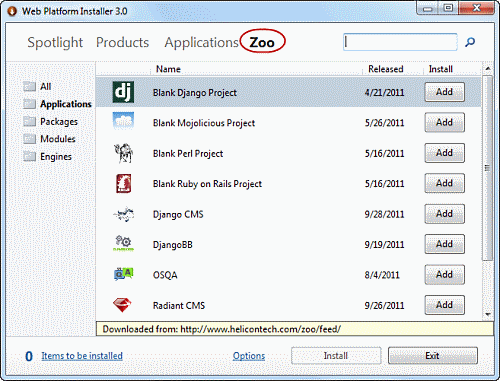
Installing Node.js
On the
Zoo tab
-> Engines there is a list of all available web engines, among them Node.js. However, we recommend installing the Node.js package into which, besides the node itself, some other useful modules are included, so that
Zoo -> Packages -> Node.js Hosting Package and click Add, Install.
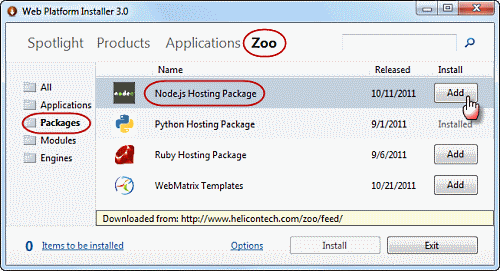
You can see all currently available web frameworks and applications in the
gallery Helicon Zoo . After you agree to the license agreements, the download and installation of IIS (if not already installed), the Helicon Zoo Module, as well as the node.exe for Windows itself will begin.
An important component of the system is the Node Package Manager (npm) package manager, which you will need to install additional modules. Unfortunately, the current version of npm on Windows is unstable. But there is its analogue - the
ryppi.py utility, which can be used in the same way as npm. ryppi.py is written in Python, so the Node.js Hosting Package installation will pull the python along with it. In the future, if npm will work stably on Windows, we will replace ryppi.py with it, which will save 80mb of disk space on the python installation.
Installing WebMatrix Templates
So, we installed Node.js, now to start writing applications for it, you can use the templates for WebMatrix. These templates allow you to create blank applications-blanks that can be used for further development.
To install them, select
Zoo -> Packages -> WebMatrix Templates .

If you do not have WebMatrix installed - it does not matter, it will be downloaded and installed automatically during the installation of templates for it. After installation, run WebMatrix and select
Site from Template on the main
page :
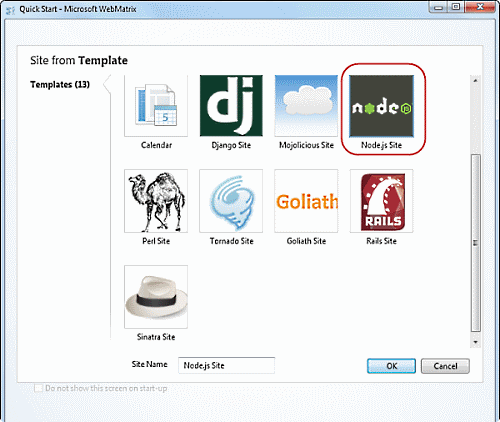
As seen in the screenshot, Node.js is not the only framework for which WebMatrix templates are available.
After creating Node.js Site, if you go to the specified URL or click "Run", you will see a simple "Hello, World!".
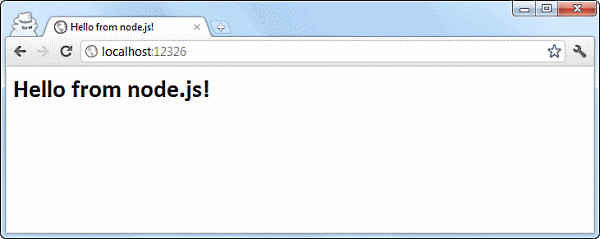
By default, the newly created website contains an
express framework for easier creation of web applications. He himself and his dependencies are located in the node_modules directory under the site, which will be convenient for deploying the application on a remote server.
The public directory is intended for storing static files. Any file placed in this directory will be processed directly by IIS as a static file, without leading to the Node.js call. This is especially important to avoid accidentally running client * .js files on the server.
The web.config file contains in particular URL rewrite rules for static files. Initially, any request is checked for the presence of such a static file in the public directory. This is necessary for some web applications that like to mix static and dynamic resources in one directory, often the root. If your application is not exposed to this, unambiguously vicious practice, then remove the rules for Microsoft URL Rewrite from the web.config file and refer to the static files, indicating the public directory explicitly.
And the web.config file contains the configuration directives needed to run Node.js and the Helicon Zoo Module on this site.
We write the first application
One of the charms of Node.js is that JavaScript is a well-known language that is widely used in web development. This means that you will not have problems with the choice of the editor. In our case, the free WebMatrix is ​​quite suitable for a start.
To demonstrate the capabilities of asynchronous web frameworks, chat is usually the first thing to do. So the most famous demo application on Node.js is a chat on
http://chat.nodejs.org/ , its
source codes are available for study.
We also decided to make the most simple chat, so that its source fully fit to the page of the habr. There are no users, no sessions, no scrolling or formatting of messages, there is only the simplest asynchronous transfer of messages to demonstrate the work of long-polling.
For work we will use the Node.js Site created by us earlier. You will need to edit the server.js and index.html files.
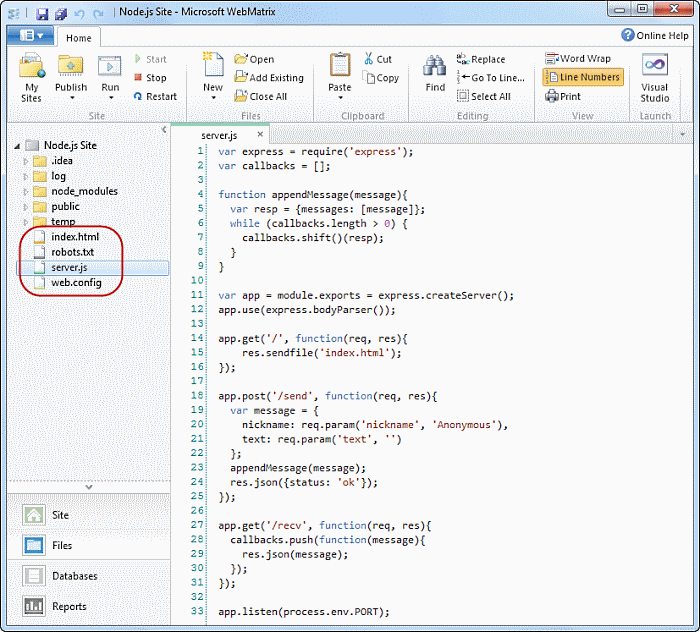
Here are the sources for the server.js file:
var express = require('express'); var callbacks = []; // function appendMessage(message){ var resp = {messages: [message]}; while (callbacks.length > 0) { callbacks.shift()(resp); } } // express var app = module.exports = express.createServer(); app.use(express.bodyParser()); // index.html app.get('/', function(req, res){ res.sendfile('index.html'); }); // app.post('/send', function(req, res){ var message = { nickname: req.param('nickname', 'Anonymous'), text: req.param('text', '') }; appendMessage(message); res.json({status: 'ok'}); }); // app.get('/recv', function(req, res){ callbacks.push(function(message){ res.json(message); }); }); // app.listen(process.env.PORT);
and index.html
<html> <head> <title>Node.js Zoo Chat</title> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.6/jquery.min.js" type="text/javascript"></script> <script type="text/javascript"> </script> </head> <body> <h1>Node.js Zoo Chat</h1> <form action="/send" method="post" id="send"> <label for="nickname">Nickname:</label> <input name="nickname" size="10" id="nickname" /> <label for="text">Message:</label> <input name="text" size="40" id="text" /> <input type="submit"> </form> <div id="messages"></div> </body> </html>
For the changes to take effect, you must click Restart and then Run:

Now you can make sure that the chat works by running it in two different browsers:
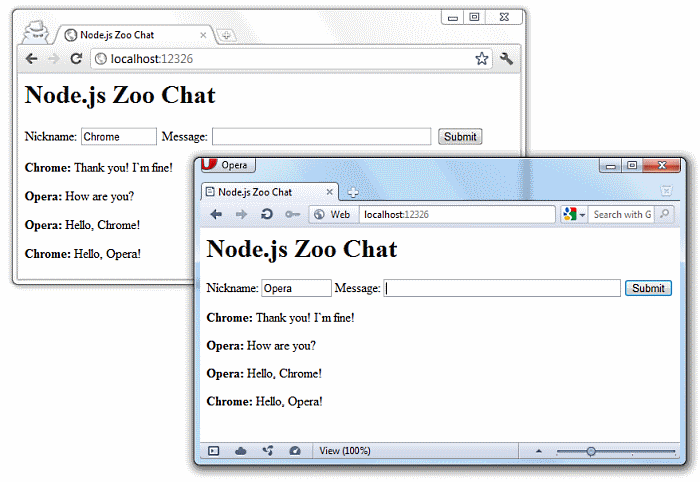
Module installation
For any web framework, perhaps the most important characteristic is the availability of various modules and the use of third-party technologies. In the current implementation, the Node Package Manager (Node PackageManager) under Windows is unstable, although Windows support is already announced and the situation may change in the near future. At the moment, you can use the package manager written in Python - ryppi.py. Here you need to remember one subtlety - ryppi.py always installs the modules in the node_modules directory of the current directory where it is called. Those. to put the module under the site you need to go to the site directory and call the command there:
C:\>cd "C:\My Web Sites\Node.js Site" C:\My Web Sites\Node.js Site>ryppi.py install mongodb Installing http://registry.npmjs.org/mongodb/-/mongodb-0.9.7-0.tgz into .\node_modules\mongodb ... Checking dependencies for mongodb ... All done.
It should also be noted that not all of the existing modules work under Windows. So, for example, the great, in my opinion,
node-sync library, written
octave by the habrow user, will not work under Windows. The library allows in many cases to get rid of the bulky paradigm of callbacks, without losing the asynchronous nature of Node, but it is based on the implementation of
node-fibers , which is not ported under Windows. I hope that in the future support for the fibers (fibers) will be built into Node.js directly.
And yet most of the modules will work stably on Windows.
CoffeScript
If you start writing a more or less large project on Node.js, sooner or later you will encounter the fact that JavaScript is not a very friendly language. A swarm of curly braces, a mass of unnecessary constructions - all this does not add readability to the program and complicates further work on the code. Fortunately, you are not the first to deal with this and the problem has already been solved. So there are many derivative languages ​​based on JavaScript or extending it. Here, for example, a short list for review:
http://altjs.org/You can take CoffeeScript, as the most popular at the moment. Code written in CoffeeScript is simpler and easier to read. Then this code is compiled into regular JavaScript and executed. A JavaScript code, on the contrary, can be converted to CoffeeScript. For example, the script server.js from our chat in CoffeeScript looks like this:
express = require("express") callbacks = []
Learn more about CoffeeScript:
http://jashkenas.github.com/coffee-script/ (English)Install CoffeeScript:
rippy.py install coffe-scriptDebugging Node.js Applications
There is a good tool for debugging applications on Node.js - node-inspector. It is already included in the node_modules directory, which is in the template of the Node.js site. The node-inspector works like this:
- the application to be debugged runs in debug mode (call node.exe with the --debug or --debug-brk parameter);
- runs node-inspector, which in essence also has a web application;
- using the WebSocket protocol, these two applications communicate with each other to transfer debug data;
- the browser opens the page with the debugged application;
- The node-inspector interface with debug information opens in a webkit-compatible browser, its interface is similar to the Web Inspector in Google Chrome or Safari.
In the root directory of the node.js site from the template there is a file start_debug.cmd, which starts debugging for the current application and opens pages in the browser for debugging.
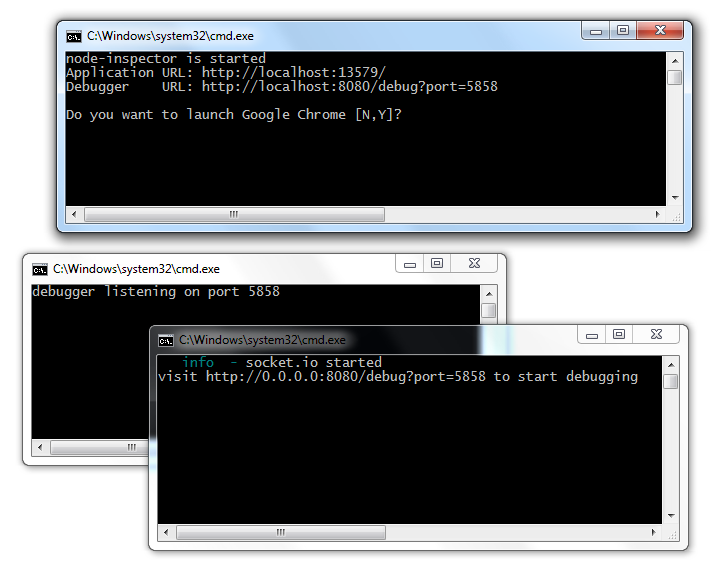
The debugger in the browser looks like this:
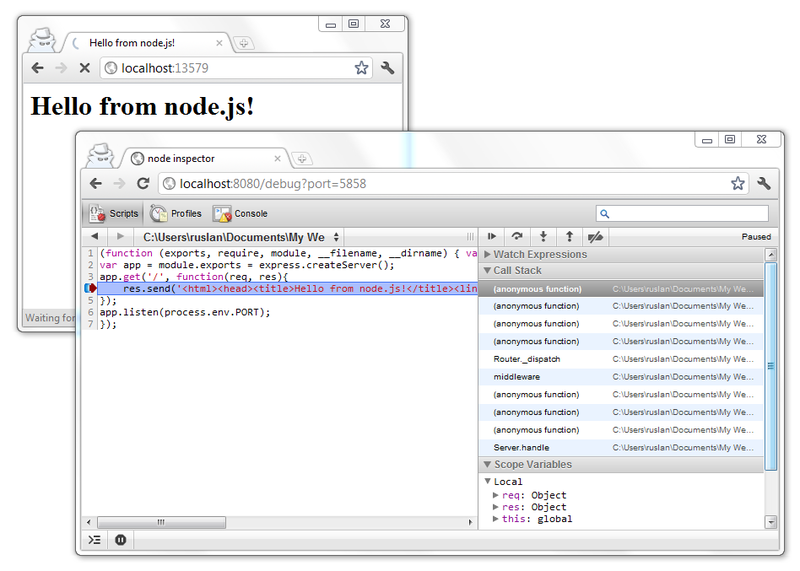
Deploy to server
So, we have written a web application and now we want to post it online. To do this, we need a server, and now there is nothing easier than setting up a Windows server to work with Node.js. We only need to repeat a few steps from the beginning of the article, which we did to deploy the working environment. Namely: install the Microsoft Web Platform Installer, add the Helicon Zoo feed to it and install the Node.js Hosting Package from the Zoo repository. All - the server is ready to accept our application. From server platforms are supported Windows 2008 and 2008 R2, 32 and 64 bit versions.
Now you only need to create an empty web site on the server using the IIS manager or hosting panel, if we are hosting, and copy our application to the site via FTP or WebDeploy. In the case of WebDeploy, the necessary rights to the folders will also be distributed. You can also use Git or another version control system, but this is outside the scope of this article.
Helicon Zoo Module was originally developed with the calculation of configuring hosting solutions. So all applications under it are divided and do not overlap. The module itself with the default settings works in automatic mode, creating one worker (process handler) when the load is low or adding workers up to the number of cores to give maximum performance if the load on the application increases.
In Helicon Zoo the concept of engines (engines) and applications (applications) is used. So in the engines it is determined what to run and how, by what protocol and on which port, how many minimum and maximum workers are allowed and similar global settings that are set globally in the applicationHost.config file. Then, already under the site, you can create an application that uses a specific engine and pass it the necessary parameters for the operation of this application. This allows you to separate the work of the hosting administrator from customers, and customers from each other.
Node.js performance tests
Test machine as a server - Core 2 Quad 2.4 Ghz, 8 Gb RAM, gigabit network. To generate the load, a more powerful computer and Apache Benchmark were used with the command “ab.exe -n 100000 -c 100 –k”. Ubuntu 11.04 Server x64 was used to test Apache and Nginx. IIS 7 tests worked on Windows Server 2008 R2. Any virtualok - honest iron.
Three tests were performed. In the first, Node.js should have just displayed the current time on the page in high resolution. Time is needed to ensure that the answers do not come from the cache. In the second test, read from the MySQL database, in the third record in the database.
Here are the results (the value on the graphs - requests per second):
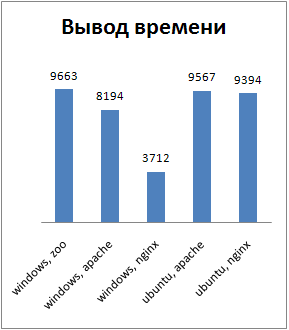

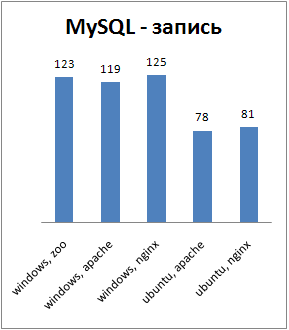
Impressive, isn't it? Now a few explanations of what these tests measure. Calling them performance tests is probably not quite right, we are not measuring different processors. The processor can have performance, while web servers are more likely to have the opposite result - how much processor time they spent on each request.
Thus, in the first test, the net overhead costs of processing the request by each specific web server and their ability to use processor resources are measured. Faster this bunch of technologies on this processor to return the answer is simply not able to. In this test, Nginx is far behind on Windows because in this system Nginx opens a new connection with the backend on every request. But on the contrary, Apache on Windows pleased me with pooling connections and real streams.
The second and third tests show how the share of web server overhead for processing a request changes as the “weight” of the request increases. However, they are now more influenced by many other factors, such as the performance of the file system, database drivers, and the database itself. For the experiment, we also tested a bunch of Windows + Zoo + MongoDB, just to see the difference with Mongo. Her results - 6793 z / s for reading and 2906 z / s for writing. Impressive, especially recording speed.
Another interesting fact is that the hardware and software base used in these tests is the same as when testing Django in
this article . So the results of these tests can be compared. Of course, the Node.js scripts are much more lightweight, we did not use templates, ORM, etc. but still there is reason to think.
At the request of readers lay out detailed graphics ab. Only the first test was re-measured, where there is a simple time display, because the overhead of the web server itself is best seen on it. Configuration files and test js scripts can be found
here . There only inkludy, everything else is by default. Horizontal scale - requests, vertical - response time in milliseconds.
Windows, IIS7 + Zoo, "time output":
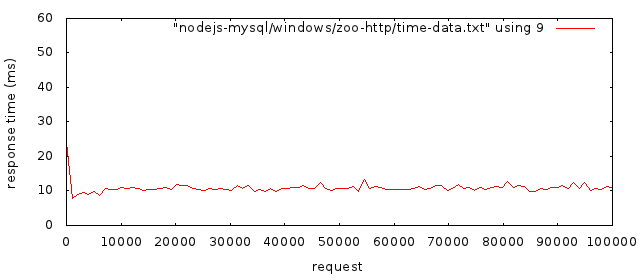
Ubuntu, Apache, "time output":
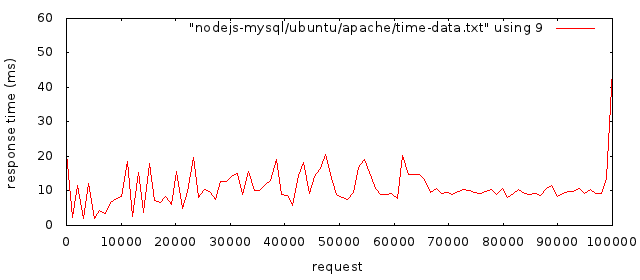
Ubuntu, Nginx, "time output":
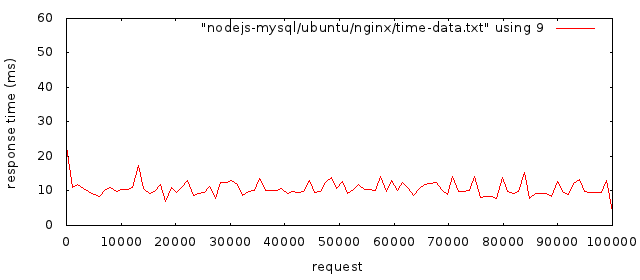
findings
I think Node.js is a very promising technology. It has impressive performance and flexibility. It is especially nice that Node.js is equally good on both Unix and Windows, and uses the right technological solutions for each of these operating systems, which can be clearly seen from the test results.
Soon we plan to add support for Erlang and Java in Helicon Zoo. It will be interesting to compare the performance of these technologies. In the meantime, Node.js is the clear leader in speed among the supported web frameworks.
PS:
Special thanks to
rukeba and
XaocCPS for their help in publishing this article.