Suppose we have 100-150 client machines (well, for example, information terminals) and we need to periodically send some commands to execute (update, clear, reboot, etc.), you can use l2tp tunnels, you can use ssh, but we will simpler! We will use the usual get requests to our server.
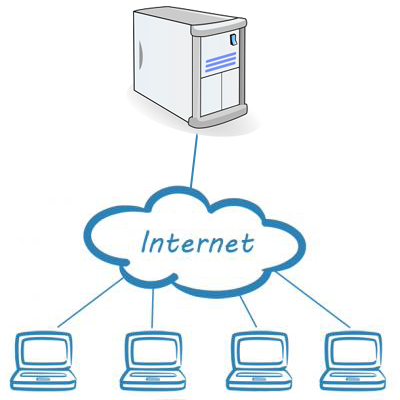
In this article, we will look at the simplest example of implementation, but it is easily completed and reworked for personal needs.
And so, on the server we need Apache + php + MySQL. On clients we have enough bash'a and wget'a.
')
I'll start with the description of the client part.
Each client will have a unique ID, it will be needed to communicate with the database. The ID can either be statically assigned to each client, or generated from such system parameters as the serial number of the processor or MAC, or the hard drive serial number and the like, or take the md5 sum from everything at once. Here is one of the options for implementation.
#!/bin/bash
scpu=`lshw -C cpu|grep serial|awk '{print $2}'`
macu=`ifconfig eth0|grep HWaddr|awk '{print $5}'`
mbs=`lshw|grep "configuration: uuid"|awk '{print $2}'`
echo $scpu $macu $mbs>/tmp/idu.key
md5sum /tmp/idu.key|awk '{print $1}'
But the script to collect commands from the north, it will run cron'om at the intervals we need. For example, once a minute.
#!/bin/bash
idu=`/scripts/idu.sh
wget --no-check-certificate -q -O /tmp/task.md 192.168.3.92/id_task.php$
echo "TASK `cat /tmp/task.md`"
task=`cat /tmp/task.md`
task1="11"
if [[ "$task1" < "$task" ]]
then
touch /opt/apps/tasks/`echo $task`
fi
Its meaning is that it gives a request to the database, and that one passes the value of the delivered task, after which the script creates a file of the same name which will subsequently be processed by the following script. This system is made so that the script queries and execution can work independently. In our case, this was done so that tasks could be set not only by the server, but also by the terminal GUI and some other system scripts.
Actually, an example script processing task.
#!/bin/bash
taskfile="/opt/apps/tasks/open_l2tp.tsk"
if [ -f $taskfile ]
then
echo "Open L2PT"
rm $taskfile
/etc/init.d/xl2tpd start
fi
#
As you can see, everything is implemented very simply.
Now let's talk about the server side.
First, we have a table with information on customers, it wakes look like this.
NAME OP ID
term1 ( 41) 7d6f4f92f10a9b3bb3
term2 ( 32) 2b0fa075e3ca1b4ee9
In our example, this table is not useful to us, but in practice it is very useful. The first column is the name of the client machine (hostname), the second is the description, the third is its ID, by which the server identifies it.
Secondly, the base in which we will set tasks for customers. It looks like this.
ID TASK STAT
2b0fa075e3ca1b4ee9 open_l2tp.tsk is_done
7d6f4f92f10a9b3bb3 open_l2tp.tsk wait
In it, we will also have 3 columns. The first is the ID, the second is the task set, the third is the execution status.
Add an entry to the table with the status “wait”, the client takes the task and changes the status to “is done”. After that, the record can be sent or deleted, or simply left in this table. And we will be processing a simple php script to process requests to this database.
<?
$ID = $_GET['ID'];
$dbcnx=@mysql_connect(localhost,base,*********);
mysql_select_db(TERM, $dbcnx);
$ath=mysql_query("select * from term_task where ID='$ID' and STATUS='wait';");
$term=mysql_fetch_array($ath);
$TASK=$term['TASK'];
$ath=mysql_query("UPDATE term_task SET STATUS='is done', DATA_S='$DATE' WHERE ID='$ID' and STATUS='wait';");
echo $TASK;
?>
If the information is important and secret, then https can be used, and if the information is completely secret, the transmitted data can be encrypted with AES-256 and the like. For example using mcrypt.
Well, that's all. I hope someone will find this useful.