The hour is not far when PDF documents can be
fully displayed using Javascript . In this case, the reverse possibility, namely the use of Javascript in PDF documents, has existed for a very long time. This will be discussed in this article.
Any software contains some actively used functionality and a significant proportion of rarely used functionality. You can figure out whether all the features of the operating system (or Microsoft Word, or your IDE), we use in our daily work or even use it at least once in a lifetime. As a rule, not all.
PDF format is no exception. We are all accustomed to text and images in PDF documents, but this is only a small part of what can be used. In particular, the PDF format includes various possibilities for creating documents with dynamic content depending on the reader and his actions. One such feature is the use of javascript.
Javascript to PDF is most often used for the following tasks:
- To change the content of the document depending on certain events. For example, hide part of the document when sending to print. Or, when opening a document, automatically fill in part of the form fields.
- To limit the reader's actions. For example, to validate input values when filling out forms.
To allow PDF viewers to interpret Javascript code, a special
Javascript API is defined. The API was originally developed only for the Adobe Acrobat family of products, but alternative viewers, as a rule, also support some basic subset of this API.
')
Consider a number of practical examples of using Javascript in PDF documents.
Hello world
Let's start the discussion of the topic with the traditional Hello World example. This and the following examples use the C # language and the
Docotic.Pdf library for working with PDF documents. A link to the source code with the code of all the examples is given at the end of the article.
using BitMiracle.Docotic.Pdf; namespace JavascriptInPdf { public static class Demo { public static void Main(string[] args) { PdfDocument pdf = new PdfDocument(); pdf.OnOpenDocument = pdf.CreateJavaScriptAction("app.alert(\", !\", 3);"); pdf.Save("Hello world.pdf"); } } }
If you open a document created by this code in Adobe Reader, you will see something like the following:
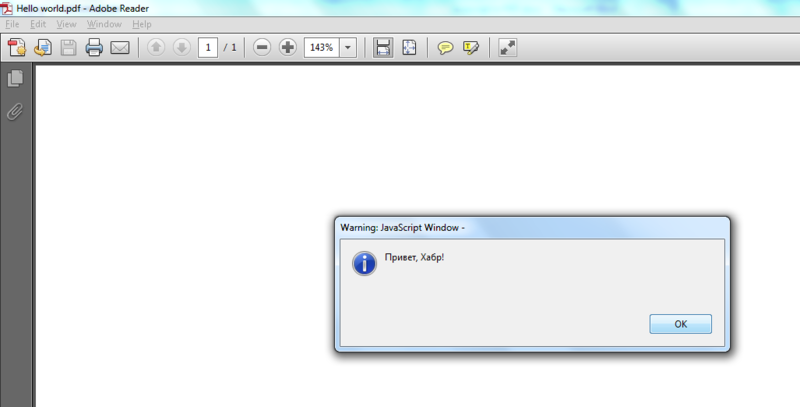
What happens in the example? The bottom line is
pdf.OnOpenDocument = pdf.CreateJavaScriptAction("app.alert(\", !\", 3);");
The PDF format includes support for actions — these are actions that occur on a particular event. For example, when in the table of contents in some PDF document we click on the link with the page number, a certain action is triggered to go to the corresponding page:

For Javascript, there is also an appropriate action. We create it using the
PdfDocument.CreateJavaScriptAction method, which is
passed JS code as a parameter. We attach the created action to the
OnOpenDocument event that occurs when the viewer opens the document.
Directly Javascript code looks like this:
app.alert(", !", 3);
The static
app class is part of the Javascript API and provides a set of methods for interacting with the viewer application. In particular, it contains several overloads of the
alert method to display a modal dialog with a message. In this example, an overload is used with the second optional parameter, the index of the dialog icon, the value 3 corresponds to the Status Icon.
From simple to complex
Consider a more realistic example.
Many PDF documents describe some forms to fill out - this could be an agreement to open a bank deposit, a visa application form or a foreign country. passports, vacation application, etc. Quite convenient, since such a form can be filled directly in the viewer and saved or printed. Authors of PDF documents containing forms can make it easier for the user to complete them using Javascript.
Real documents often contain fields for entering the due date. For example, it might look like this:
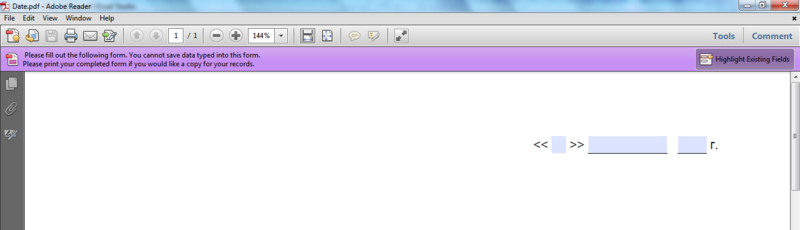
In principle, creating a document, you can stop there. However, you can go a little further and slightly simplify the task that fills - for example, setting the default date to the current one - in 99% of cases this is exactly what is needed.
Set the default date
C # code for creating fields for the date, as in the screenshot, in this case is not so interesting. Consider only the part relating to Javascript. It is necessary to set the current date in the fields when opening the document; this is done like this:
function setDay(date) { var dayField = this.getField("day"); if (dayField.value.length == 0) { dayField.value = util.printd("dd", date); } } function setMonth(date) { var monthField = this.getField("month"); if (monthField.value.length == 0) { monthField.value = util.printd("date(ru){MMMM}", date, true); } } function setYear(date) { var yearField = this.getField("year"); if (yearField.value.length == 0) { yearField.value = util.printd("yyyy", date); } } function setCurrentDate() { var now = new Date(); setDay(now); setMonth(now); setYear(now); } setCurrentDate();
Compared to the previous example, the JS code has increased in volume, so using it directly in the C # line was inconvenient because of the need to escape quotes and insert line breaks. Therefore, we put this code into resources, then the script will be used like this:
pdf.OnOpenDocument = pdf.CreateJavaScriptAction(Resources.SetCurrentDate);
As a result, when opening a document, we will see something like the following picture:
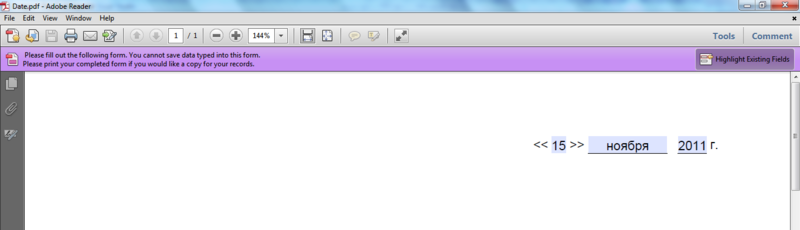
Pay attention to the code for setting the month - we use the specific overload of the method
util.printd to output the localized month.
monthField.value = util.printd("date(ru){MMMM}", date, true);
This gives excellent results in Adobe Reader, but, unfortunately, it is not guaranteed that other viewers will correctly support such specific designs. When designing a document that needs to be considered. It may be worth replacing this code with a more verbose one (independent getting the name of the month in the right case), but supported by a large number of viewers.
In this example, it is also important that the values are set only to empty fields. Without these checks, a situation may arise when the user saves the completed form, and when opening such a saved form, the date will be changed to the current one.
Validation of Input Values
If you still need to change the date when filling out the form, it makes sense to allow entering only numbers in the fields for the day and year. Use for this the following Javascript code:
function validateNumeric(event) { var validCharacters = "0123456789"; for (var i = 0; i < event.change.length; i++) { if (validCharacters.indexOf(event.change.charAt(i)) == -1) { app.beep(0); event.rc = false; break; } } } validateNumeric(event);
In the C # code, we use the
OnKeyPress event of the controls for checking the entered character:
PdfJavaScriptAction validateNumericAction = m_document.CreateJavaScriptAction(Resources.ValidateNumeric); dayTextBox.OnKeyPress = validateNumericAction; yearTextBox.OnKeyPress = validateNumericAction;
After that, in the fields for the day and year it will be impossible to enter any character other than a digit. Paste string from the clipboard that contains an invalid character will also fail.
Sync field values
It often happens that the same information in the document must be specified several times. And in the case of PDF documents using Javascript, you can save the user from repeating the same actions.
Suppose there is a document of the following form:

We modify it so that when one of the fields changes with the full name, the other is updated.
We use a simple Javascript function:
function synchronizeFields(sourceFieldName, destinationFieldName) { var source = this.getField(sourceFieldName); var destination = this.getField(destinationFieldName); if (source != null && destination != null) { destination.value = source.value; } }
And also the following C # code:
PdfDocument pdf = new PdfDocument(“Names.pdf”); pdf.SharedScripts.Add( pdf.CreateJavaScriptAction(Resources.SynchronizeFields) ); pdf.GetControl("name0").OnLostFocus = pdf.CreateJavaScriptAction("synchronizeFields(\"name0\", \"name1\");"); pdf.GetControl("name1").OnLostFocus = pdf.CreateJavaScriptAction("synchronizeFields(\"name1\", \"name0\");"); pdf.Save("NamesModified.pdf");
Now if any of the textboxes lose focus, the value of the other will be updated. Pay attention to the technique that was not met before - general Javascript code is placed in the
PdfDocument.SharedScripts collection, and then we are able to call a function defined in the general code from specific actions.
Summarize
Javascript can be used not only in web development, but also in areas such as the design of PDF documents. A little extra effort and PDF documents created will delight the reader no less than a program with a convenient and thoughtful interface - a sophisticated user.
And yet such opportunities are secondary compared to the contents of the document, so the practical value of Javascript in PDF is not so high. In addition, a number of problems are associated with this:
- It is more difficult to write Javascript code than in the case of conventional web development. You need to create and open a document to check the correctness of the written code.
- Javascript is fully supported only by Adobe viewers. In alternative viewers, support is significantly limited or absent altogether.
- Javascript can be disabled in the PDF viewer.
- Theoretically, the execution of Javascript scripts in the document is unsafe, and various vulnerabilities are periodically detected.
Armed with the described information, do not be surprised if the PDF document that opens one day offers to play chess, and at this time will quietly remove Foxit Reader from the system;)
Download the code of examples from the article
here .