Introduction
Horizontal scrolling of screens is a convenient and fashionable thing. On Habré was already a
topic dedicated to this topic. However, using the ViewFlipper does not make it easy to achieve a snap effect when the screens move with the finger. Also need a mechanism for automatic screen adjustment in one direction or another. He could not find an example where this would be well explained and implemented. In the comments
offered to see the source code of Google code, where it is implemented. However, it would be more convenient to use a ready-made solution. Such a mechanism is implemented in the Android
Support Package . You can use this library for versions of Android 1.6 and later. Specifically, we will need the ViewPager and PagerAdapter classes.
Presetting
To connect the library to the project, perform the following steps.
Go to the directory where the Android SDK is installed, launch the SDK Manager and select Android Support package from the list of components available for installation. Install it. All you need to be in the following directory: <directory with sdk> / extras / android / support /. In the v4 folder there will be a .jar file, which you need to connect to the project. If you are working in Eclipse, then in the Package Explorer we click the right click on the project, select Build Path -> Add External Archives. In the dialog box that appears, go to the downloaded .jar file, select it and click OK. Everything, the library is connected to the project and is ready to use.
I would like to note that problems may arise if you use the old version of the SDK Manager. My specified support package did not appear in the list of available downloads. After updating the SDK, the problem was solved.
Development
By following the instructions at the above link, and installing the library, you can proceed to the implementation of the task.
ViewPager works like a ListView. It takes the data to display from the PagerAdapter. We will need to inherit our class from the PagerAdapter and implement some methods in it. Adding and deleting screens is implemented using the methods instantiateItem () and destroyItem (), respectively. View to display can be created directly in the adapter. This approach is good because ViewPager can be configured so that the adapter does not store all the screens at once. By default, the adapter stores the current screen, and one by one to the left and right of it. This can save memory if the contents of the screens are too complex. However, in this example, we will create all the screens in the application's activity, add them to the list, and then pass this list to the adapter. The adapter will not create the view itself, but will take them from the list.
The adapter code is the following.
public class SamplePagerAdapter extends PagerAdapter{ List<View> pages = null; public SamplePagerAdapter(List<View> pages){ this.pages = pages; } @Override public Object instantiateItem(View collection, int position){ View v = pages.get(position); ((ViewPager) collection).addView(v, 0); return v; } @Override public void destroyItem(View collection, int position, Object view){ ((ViewPager) collection).removeView((View) view); } @Override public int getCount(){ return pages.size(); } @Override public boolean isViewFromObject(View view, Object object){ return view.equals(object); } @Override public void finishUpdate(View arg0){ } @Override public void restoreState(Parcelable arg0, ClassLoader arg1){ } @Override public Parcelable saveState(){ return null; } @Override public void startUpdate(View arg0){ } }
In the adapter, we needed to define our own implementation for the instantiateItem (), destroyItem (), getCount () and isViewFromObject () methods. For other methods, the body can be left empty.
Create a page.xml markup file and describe the contents of the screen in it as follows:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:padding="10dip" android:background="#ddd"> <TextView android:id="@+id/text_view" android:layout_width="fill_parent" android:layout_height="fill_parent" android:layout_centerInParent="true" android:gravity="center" android:background="#888" android:textColor="#fff" android:textSize="30dip"/> </RelativeLayout>
In the application's activity, as mentioned above, we will create several View, which will be our screens, add them to the list and pass the list to the adapter. Then we will create a ViewPager and install the received adapter for it.
')
Activity code:
public class ViewPagerSampleActivity extends Activity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); LayoutInflater inflater = LayoutInflater.from(this); List<View> pages = new ArrayList<View>(); View page = inflater.inflate(R.layout.page, null); TextView textView = (TextView) page.findViewById(R.id.text_view); textView.setText(" 1"); pages.add(page); page = inflater.inflate(R.layout.page, null); textView = (TextView) page.findViewById(R.id.text_view); textView.setText(" 2"); pages.add(page); page = inflater.inflate(R.layout.page, null); textView = (TextView) page.findViewById(R.id.text_view); textView.setText(" 3"); pages.add(page); SamplePagerAdapter pagerAdapter = new SamplePagerAdapter(pages); ViewPager viewPager = new ViewPager(this); viewPager.setAdapter(pagerAdapter); viewPager.setCurrentItem(1); setContentView(viewPager); } }
As you can see from the code, we created three screens. For each of them, we indicated what he counts. Having installed the adapter for the ViewPager, let us indicate that the screen with the index 1 will now be displayed, i.e. on which is written "Page 2".
Screenshot of the application, while scrolling the screen:
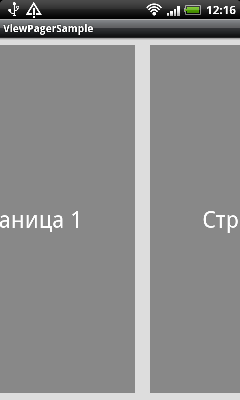
Conclusion
As you can see from the example, using a ViewPager and a PagerAdapter is quite easy. When sliding screens, the image is tied to the position of the finger. Works automatic fine-tuning in any of the parties. Clicking on child elements (various buttons, list elements, if present) is handled correctly.
And finally, a link to
an article that tells about ViewPager.