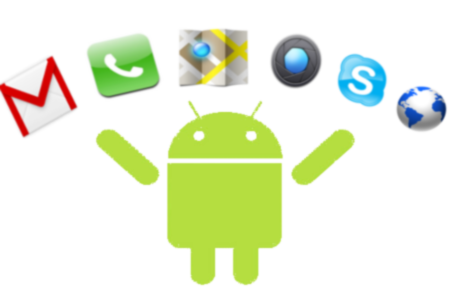
Introduction
Objects of the
Intent type can be used to communicate between individual parts of an Android application, or between different system applications. The power of the mechanism of intents is that it can be used to access any application installed on the system. This allows you to reuse some components and significantly reduce development time.
In this article, I will show some of the possibilities for using the Intent class to refer to system applications.
So what can be done with Intenta:
1. Call
Intent dialIntent = new Intent(Intent.ACTION_CALL, Uri.parse("tel:" + "123456789")); startActivity(dialIntent);
You must also add permission to make calls:
<uses-permission android:name="android.permission.CALL_PHONE" />
Or permission for emergency calls:
<uses-permission android:name="android.permission.CALL_PRIVILEGED" />
Calls can also be made by specifying the first parameter as
ACTION_DIAL . This will open the phone book with the specified number, but the call itself will not be automatically made. This parameter does not need permissions in the manifest.
')
2. Send SMS
Intent smsIntent = new Intent(Intent.ACTION_VIEW); smsIntent.setType("vnd.android-dir/mms-sms"); smsIntent.putExtra("sms_body", "Some SMS text"); startActivity(smsIntent);
Because Since this code does not send SMS directly, but launches standard activation for sending SMS, then no permissions need to be specified.
3. Get a contact from the phone book
Intent pickIntent = new Intent(Intent.ACTION_PICK, android.provider.ContactsContract.Contacts.CONTENT_URI); startActivityForResult(pickIntent, PICK_RESULT);
This code will open the standard contacts application. The
startActivityForResult method allows you to get the uri of the selected contact from the
Intent data parameter of the overridden
onActivityResult method.
The above code shows in the contacts application at once all the user's contacts, including email. If you only need to select a contact from the phone book, you can use the following code:
Intent pickIntent = new Intent(Intent.ACTION_GET_CONTENT); pickIntent.setType(ContactsContract.CommonDataKinds.Phone.CONTENT_ITEM_TYPE); startActivityForResult(pickIntent, PICK_RESULT);
To read the list of contacts, you must also add permission to the manifest:
<uses-permission android:name="android.permission.READ_CONTACTS" />
4. Open link in browser
Intent browseIntent = new Intent(Intent.ACTION_VIEW, Uri.parse("http://www.google.com")); startActivity(browseIntent);
This code will open the link passed in the parameter in one of the installed browsers. In this case, the user can choose the browser to view. If the link leads to YouTube, you can immediately open the video with a standard YouTube application.
With this intent, you can also open the standard Android Market client with a link to any application. For this, it is necessary to pass the link of the form
market: // search? Q = search query to the
uri parameter, where the
search query must be replaced with the corresponding search query.
Internet permissions do not need to be specified, because The network is not used directly, but through the application browser.
5. Share the content / write a letter
Intent shareIntent = new Intent(Intent.ACTION_SEND); shareIntent.setType("text/plain"); shareIntent.putExtra(Intent.EXTRA_SUBJECT, "Content subject"); shareIntent.putExtra(Intent.EXTRA_TEXT, "Content text"); startActivity(Intent.createChooser(shareIntent, "Sharing something."));
Intent can set the theme and text content that you want to share. The
Intent.createChooser () method creates a dialog for selecting the appropriate application.
If you need to share media content (image / video), then you need to specify the appropriate content type in the
setType method, for example,
mailIntent.setType (“image / png”); , and add the content itself to extra intensity:
shareIntent.putExtra(Intent.EXTRA_STREAM, imageUri);
With this intent you can also send email to a specific address:
shareIntent.putExtra(Intent.EXTRA_EMAIL, new String[] { mail });
6. Open the map at specific coordinates or request
String uri = "geo:"+ latitude + "," + longitude; Intent mapIntent = new Intent(android.content.Intent.ACTION_VIEW, Uri.parse(uri)); startActivity(mapIntent);
Uri can be modified by specifying other parameters:
- geo: latitude, longitude
- geo: latitude, longitude? z = zoom The parameter z is the zoom level when the map is displayed (from 0 to 23)
- geo: 0,0? q = my + street + address
- geo: 0.0? q = business + near + city
7. Take a picture with the camera
Intent cameraIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); startActivityForResult(cameraIntent, CAMERA_RESULT);
This code starts the standard camera application. The image received from the camera can be processed in the
onActivityResult method:
@Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { if (requestCode == CAMERA_RESULT) { Bitmap thumbnail = (Bitmap) data.getExtras().get("data"); ImageView ivCamera = (ImageView) findViewById(R.id.iv_camera); ivCamera.setImageBitmap(thumbnail); } }
The image obtained from the camera can be saved in a specific directory by adding a parameter to the intent:
File photo = new File(Environment.getExternalStorageDirectory(), "Pic.jpg"); cameraIntent.putExtra(MediaStore.EXTRA_OUTPUT, Uri.fromFile(new File(filePath)));
To use this intent, you do not need permission
android.permission.CAMERA , because the camera is not used directly, but only the built-in camera application is called. However, it is recommended to add the following line to AndroidManifest:
<uses-feature android:name="android.hardware.camera"></uses-feature>
Thus, people with devices without a built-in camera (apparently, this also happens) will not see your application on the market.
8. Call any contact via Skype
To make a call via Skype intent, you must specify the specific name of the activation and the package to which it will apply. This complicates the code a bit because in two different versions of Skype, the package names are different. It is necessary to check which version of Skype client is installed on the system, and whether it is installed at all. For verification, you can use the following method:
public static boolean isIntentAvailable(Context context, Intent intent) { final PackageManager packageManager = context.getPackageManager(); List<ResolveInfo> list = packageManager.queryIntentActivities( intent, PackageManager.MATCH_DEFAULT_ONLY); return list.size() > 0; }
This method checks the system for the presence of an application capable of responding to a specific intent. Using this method, the call code via Skype will look like this:
private static final String SKYPE_PATH_GENERAL = "com.skype.raider"; private static final String SKYPE_PATH_OLD = "com.skype.raider.contactsync.ContactSkypeOutCallStartActivity"; private static final String SKYPE_PATH_NEW = "com.skype.raider.Main"; Intent skypeIntent = new Intent().setAction("android.intent.action.CALL_PRIVILEGED"); skypeIntent.setData(Uri.parse("tel:" + skype)); if (isIntentAvailable(context, skypeIntent.setClassName(SKYPE_PATH_GENERAL, SKYPE_PATH_NEW))) { context.startActivity(skypeIntent); } else if (isIntentAvailable(context, skypeIntent.setClassName(SKYPE_PATH_GENERAL, SKYPE_PATH_OLD))) { context.startActivity(skypeIntent); } else { Toast.makeText(context, " Skype .", Toast.LENGTH_LONG).show(); }
A simpler method for checking the presence of an application in the system is to intercept an
ActivityNotFoundException , which will be
thrown in the
startActivity method. However, this will only react to the problem, but not prevent it. For example, using the above
isIntentAvailable method,
you can make the corresponding control inactive in time, or redirect the user to the Android Market to install the corresponding application.
9. Use Speech to Text for voice recognition.
Intent speechIntent = new Intent(RecognizerIntent.ACTION_RECOGNIZE_SPEECH); speechIntent.putExtra(RecognizerIntent.EXTRA_LANGUAGE_MODEL, RecognizerIntent.LANGUAGE_MODEL_FREE_FORM); speechIntent.putExtra(RecognizerIntent.EXTRA_PROMPT, "Speak please"); startActivityForResult(speechIntent, RESULT_SPEECH_TO_TEXT);
This code starts the speech recognition application. If you say something, the system processes the voice and returns the result to the
onActivityResult method:
@Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { if (requestCode == RESULT_SPEECH_TO_TEXT && resultCode == RESULT_OK) { ArrayList<String> matches = data.getStringArrayListExtra(RecognizerIntent.EXTRA_RESULTS); TextView tvSpeech = (TextView) findViewById(R.id.tv_speech); tvSpeech.setText(matches.get(0)); } }
Conclusion
The mechanism of intents provides great opportunities for the developer. In this article I wanted to show examples of actions that can be implemented more easily with the help of intents. I hope it will save someone time to develop.
Perhaps there are other interesting uses for intents. I propose to share them in the comments.