Strategies implemented in the starter pack are only a starting point for creating your own bot, in fact, this is one of the worst strategies. At the same time, the starter pack includes useful features that help you develop a better strategy. This article will guide you through a series of improvements package. With each completed step, your bot will become smarter, and your rating will start to grow.
Prerequisites
For this article, we will use the start package in Java (note: translator: we also need Python, because the tools - the tester bot and a set of useful scripts are written on it, the links are at the end of the article). Also you will need tools.
Install
Create a directory in which you will work with the starter pack (bot) and tools. You should have something like this:
C:\aichallenge>tree Folder PATH listing C:. +----tools +---mapgen +---maps | +---maze | +---multi_hill_maze | +---symmetric_random_walk +---sample_bots | +---csharp | +---java | +---php | | +---tests | +---python +---submission_test +---visualizer +---data | +---img +---js C:\aichallenge>dir /b AbstractSystemInputParser.java AbstractSystemInputReader.java Aim.java Ants.java Bot.java Ilk.java make.cmd Makefile Manifest.txt MyBot.java Order.java Tile.java tools
')
Check
Make sure that everything works as it should by launching a test game (use the shell script play_one_game.cmd or play_one_game.sh depending on your OS). Among the tools is the utility “playgame.py”, which will help us test our bot. Its use is clearly shown in the play_one_game.cmd shell script (play_one_game.sh).
Create a shell script to test the bot.
Now we will make our shell script, which will use the new map and our bot.
For Windows: create tutorial.cmd
C:\aichallenge>notepad tutorial.cmd
For Linux: create tutorial.sh
user@localhost:~$ gedit tutorial.sh
then assign rights
user@localhost:~$ chmod u+x tutorial.sh
In it we register:
python tools/playgame.py "java -jar MyBot.jar" "python tools/sample_bots/python/HunterBot.py" --map_file tools/maps/example/tutorial1.map --log_dir game_logs --turns 60 --scenario --food none --player_seed 7 --verbose -e
- The first two options are bots that we launch. We use our bot and HunterBot as an opponent.
- The option --map_file sets the used map. Choose a card with 2 anthills.
- The option --log_dir sets the location where the viewer file will be saved and, optionally, the I / O logs of the bot and its errors.
- Option --turns sets the maximum number of moves for the game. Since no additional data is required, we will stop at 60 steps.
- The --scenario option allows you to place the starting points with food on the map. Specify the placement of food for this tutorial.
- Option --food none allows you to turn off the appearance of food during the game. Again, this option is used as part of the tutorial.
- The --player_seed option ensures that you can get the same result as in this tutorial. HunterBot uses this value to initialize a random number generator, so it will always take the same steps. (Note: if you want to use game replays to debug your bot, then you should also apply this strategy).
- The option --verbose will display general statistics on the game, which allows you to monitor the progress of the game.
- The --e option sends all bot errors to the console, so if you make a mistake while studying - you can see a message about it.
Do not forget that the Java bot must be compiled. Both bots - both HunterBot and the bot reproduced in the textbook will be deterministic, i.e. with the same input, they will produce the same moves. This means that if you follow the instructions completely, you will get exactly the same result as in the textbook.
Let's run our bots using shell skrpita and see how the bot works from the starter pack.
You should see a similar conclusion:
running for 60 turns ant_count c_turns climb? cutoff food r_turn ranking_bots s_alive s_hills score w_turn winning turn 0 stats: [1,1,0] 0 [1,1] - 18 0 None [1,1] [1,1] [1,1] 0 None turn 1 stats: [1,1,0] 0 [1,1] - 16 1 [0,0] [1,1] [1,1] [1,1] 1 [0,1] turn 2 stats: [2,1,0] 0 [1,1] - 16 1 [0,0] [1,1] [1,1] [1,1] 1 [0,1] turn 3 stats: [2,2,0] 0 [1,1] - 15 1 [0,0] [1,1] [1,1] [1,1] 1 [0,1] turn 4 stats: [2,3,0] 0 [1,1] - 14 1 [0,0] [1,1] [1,1] [1,1] 1 [0,1] turn 5 stats: [2,4,0] 0 [1,1] - 14 1 [0,0] [1,1] [1,1] [1,1] 1 [0,1] turn 6 stats: [2,4,0] 0 [1,1] - 14 1 [0,0] [1,1] [1,1] [1,1] 1 [0,1] turn 7 stats: [2,4,0] 0 [1,1] - 14 1 [0,0] [1,1] [1,1] [1,1] 1 [0,1] turn 8 bot 0 eliminated turn 8 stats: [0,4,0] 0 [0,1] - 14 1 [0,0] [0,1] [1,1] [1,1] 1 [0,1] score 1 3 status eliminated survived playerturns 8 8
The game lasted only 8 steps, which is suspiciously fast. It seems that player 0 (our bot) was destroyed. After the game ends, the browser should start to show what happened in the visualizer.
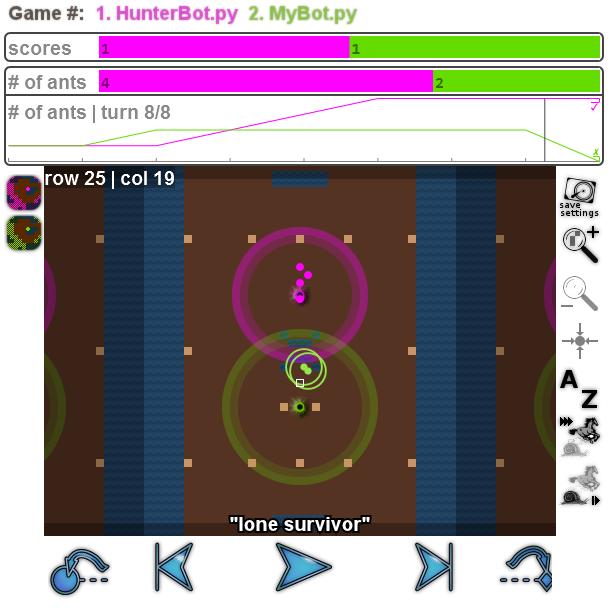
You can see that the strategy of the original bot is terrible - it destroyed itself by pushing two ants. Well, this will be our first improvement.
Step 1. Avoid collisions
Plan
To prevent collisions, do the following:
- Forbid ants from moving on other ants.
- Prohibit 2m ants from moving to one point.
- Track information about all our ants.
Implementation
To keep track of where our ants are, effectively use the HashMap structure. This data structure will be stored by the position of the ants and will allow you to check whether this position is already in use. Each key HashMap value will contain a Tile object. Tile - object with the position of the object on the map. The key is the position of the intended step, and the value is the current position. This way, we can check the HashMap before taking a step to make sure that the two ants do not go to one point. With each movement we will update the HashMap.
Since this check is useful later in the tutorial, we will make a function that checks whether a given step is possible. It will return a boolean value (true or false).
Code
We will omit the comments in the original bot and place the new code:
public boolean doMoveDirection(Ants ants, HashMap<Tile, Tile> orders, Tile antLoc, Aim direction) {
The
doMoveDirection
function gets the ant position (Tile object) and direction (Aim object - N | E | S | W) and tries to perform a step. This function is located outside the
doTurn
function, so we have to pass the Ants and HashMap object to it with our orders. We will use some predefined functions from the bot that can help us:
-
ants.getTile(Tile, Aim)
takes the position (Tile object) and direction (Aim object) and returns the new position (Tile object). She will take care of closing the card, so we don’t have to worry about it.
-
ants.getIlk(Tile)
- takes a position (Tile object) and returns an Ilk object (the type of the map point in the specified position). Next, call the function
isUnoccupied()
to make sure that there is an opportunity to make a move.
-
Ilk.isUnoccupied
takes the position and lets us know if this move is possible. This is better than the earlier
Ilk.isPassable
, because it will not allow you to go to food or to other ants.
- HashMap with orders is used to track the planned steps. In an
if
expression, the condition
!orders.containsKey(newLoc)
checks for the existence of the specified key and helps avoid collisions.
results
Finally, run our bot again and see what happened.
C:\aichallenge>tutorial.cmd running for 60 turns ant_count c_turns climb? cutoff food r_turn ranking_bots s_alive s_hills score w_turn winning turn 0 stats: [1,1,0] 0 [1,1] - 18 0 None [1,1] [1,1] [1,1] 0 None turn 1 stats: [1,1,0] 0 [1,1] - 16 1 [0,0] [1,1] [1,1] [1,1] 1 [0,1] turn 2 stats: [2,1,0] 0 [1,1] - 16 1 [0,0] [1,1] [1,1] [1,1] 1 [0,1] ... turn 60 stats: [1,5,0] 0 [1,1] - 12 1 [0,0] [1,1] [1,1] [1,1] 1 [0,1] score 1 1 status survived survived playerturns 60 60

Better, but still bad. Only one ant comes out and fights with HunterBot, however, we avoided suicide, and this is already an improvement. In addition, we have created a helper function that will be useful in the future.
Links
Start package
can be found here .
Tools
for Windows ,
for Linux / MacOS .
Translated articles:
Ants Tutorial ,
Step 1: Avoiding collisions .
PS On the site, examples for Java have just started to appear (they are now completed at the 1st step), so for now I’ve brought the translation to the end of the first step.
PPS If I liked the translation, I will continue to translate as Java examples are added to the next steps.
PPPS Thanks to an unknown friend for an invite and the opportunity to submit an article for general review.