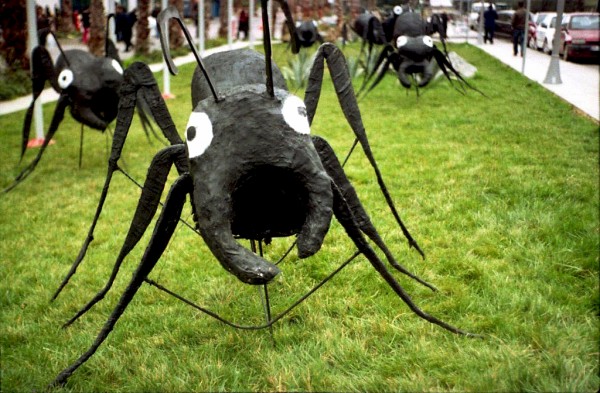
The Google AI Challenge Ants is starting very soon. Two days ago, there was already an announcement on the Habré about this contest:
http://habrahabr.ru/blogs/sport_programming/130457/
. For those who have a desire to participate, or at least just find out in more detail how this is done, I wrote this “Quick Start”.
From the article you will learn how to start a game on your machine, write your first bot and run two copies to fight against each other.I use
Linux (Debian) , but the site also has a kit for
Windows (Python must be installed on the system).
I will give an example of a bot in the
Perl language , but this does not in any way cancel the usefulness of the article for those who write in another language.
Bundled from aichallenge have ready-made bots written in languages: Java, PHP, C #, Python.
Moreover, now on the site you can download a starter pack for writing your bot in languages:
C #, C ++, Common Lisp, D, Go, Java, JavaScript, Lua, OCaml, Pascal, Perl, PHP, Python, Ruby, Scala.
These languages will be added soon:
C, Clojure, CoffeeScript, Erlang, Groovy, Haskell, Visual Basic.
')
And yes. You can simultaneously launch a battle bots written in different programming languages.
Parting words
Even if you have little programming experience and you think that nothing shines for you, I still recommend trying your hand at least on a local machine. At a minimum, you will see a sample of a good programming style and you will get such a useful programming skill as working with someone else’s code.
And by the way, Google promises some special prizes for first-year students.
Well, let's get started.
What you need to start?
First of all, here is the link to the official website, where there is all the useful information:
http://beta.aichallenge.org/To write your bot you will need a
starter pack . On the page
http://beta.aichallenge.org/starter_packages.php choose your programming language and download the archive. I chose Perl:
$ wget http://beta.aichallenge.org/starter_packages/perl_starter_package.zip
Unpack, for example, in the MyBot directory:
$ unzip -d MyBot perl_starter_package.zip Archive: perl_starter_package.zip inflating: MyBot/Ants.pm inflating: MyBot/MyBot.pl inflating: MyBot/MyBot.pm inflating: MyBot/Position.pm inflating: MyBot/README.md
This is a simple but already working version of the bot! You can run it in action. But first I will tell you what it is for.
The battle takes place in a rectangular area. On the plot there is land, water, food, anthills and, in fact, the main characters - ants.
Turn-based game.
Your bot is a program that, in an infinite loop (one cycle - one step), gets the state of the playing field and returns a new position to its ants.
Your bot is the collective mind of all your ants, it knows everything your ants know. At each cycle, the bot for each of your ant must determine the next step, where the ant will go: north, south, east, west, or remain in place. Everything. This is the whole essence of the game.
Now we turn to the implementation. The bot implementation is in the
MyBot.pm file.
The
Ants.pm and
Position.pm files are the necessary files for interacting with the game world. File
MyBot.pl - file to run the bot.
All work on writing a bot will occur in the file MyBot.pm.
And the main function here is:
sub create_orders { my $self = shift; for my $ant ($self->my_ants) { my @food = $self->nearby_food($ant); for my $f (@food) { my $direction = $self->direction($ant, $f); next unless $self->passable( Position->from($ant)->move($direction) ); $self->issue_order( $ant, $direction ); last; } } }
This is exactly the function that is called at each step.
In it for every ant of all your ants
for my $ant ($self->my_ants) {
something is being done ... and as a result, an indication is given to the system:
$self->issue_order( $ant, $direction );
that this ant will go this way.
Everything. And whoever goes where is your imagination. In this example, the ant will go for the first available food.
But I promised that we will try to write your bot. And so that the lesson is simple, we will write the simplest, but also the most useless option - the ant will walk at random.
But before you start, you need to figure out where we will look at the battles of our bots.
The official site offers a special kit:
Ants game visualizer , written in Python.
Linux / MacOS version:
http://beta.aichallenge.org/tools.tar.bz2Windows version:
http://beta.aichallenge.org/tools.zipTake:
$ wget http://beta.aichallenge.org/tools.tar.bz2
Unpack:
$ tar -xjvf tools.tar.bz2
We look. At the root of the folder there are two shell scripts with speaking names:
play_one_game.sh
play_one_game_live.shTo watch the process, we need the
play_one_game_live.sh file.
Let's look carefully:
When you start the game:
$ ./play_one_game_live.sh
A visualizer will open, in which you will see a large field with 4 anthills and you can watch the battle.
As you can see from the code, there will be 4 python-bots that come with the visualizer to fight here. In addition to the python bots, as I said, there are bots written in C #, Java and PHP.
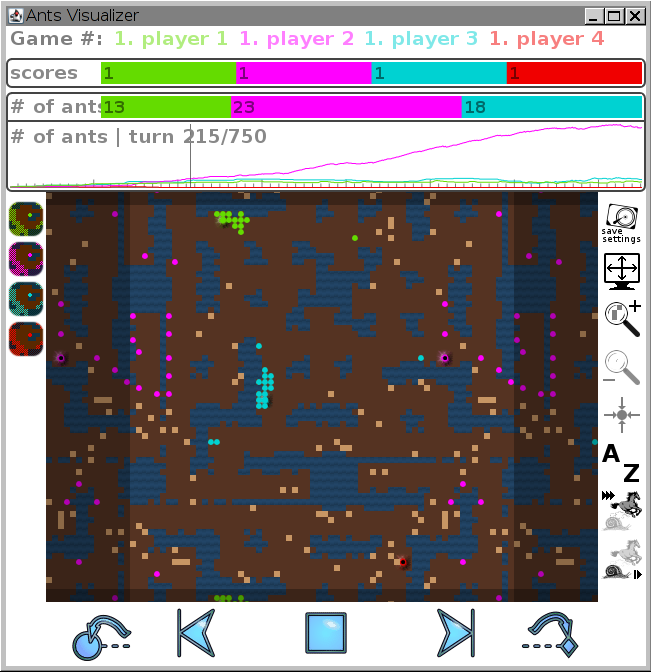
The first step has been taken. Fine! What's next?
And then we want to connect your Perl-bot to the game. We will change the script.
The line
"python sample_bots / python / HunterBot.py" is a command to launch your bot. The result of this command should be a running process that will accept input data and produce a result. Thus, there can be any of your programs written in any programming language.
In my case - the program that launches my bot -
MyBot.pl .
Create the
sample_bots / perl directory and copy all the necessary files of our bot from the starter kit to this directory:
$ cp MyBot/*.pl MyBot/*.pm tools/sample_bots/perl/
Bot there. Choose a card easier. Maps are in the maps directory. Each card is a text file. The first three lines are the size and number of players on the map.
Be careful, run exactly as many players as indicated in the map!
I chose a small card with two players:
maps / example / tutorial1.mapI make changes to the startup file
play_one_game_live.sh :
All is ready. We can run and watch the game of our bot.
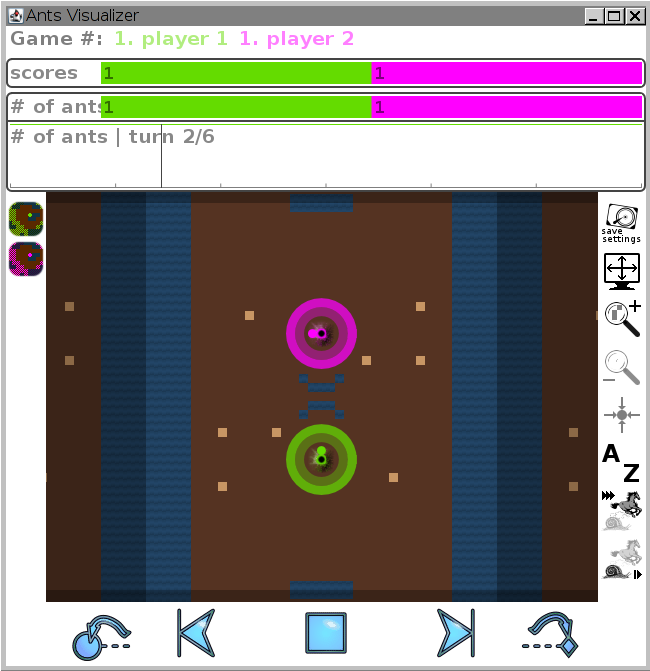
We write a bot that walks randomly
We proceed to the implementation of the task. Open the file MyBot.pm and delete all unnecessary from the
sub create_orders function. The starting point will be:
sub create_orders { my $self = shift; for my $ant ($self->my_ants) { $self->issue_order( $ant, $direction ); } }
She is not working yet, because we have not defined
$ direction . If you look at the function code
issue_order (it is in the Ants.pm file), then you will find out that it takes the letter
N, S, W or E (north, south, west or east) as the second argument.
Create an array of letters, and for each ant, take an arbitrary element of this array. Thus, each ant at each step will go in a direction unknown to anyone.
The perl language gives the temptation to write it all in a couple of lines, but since The article is designed for a different type and level of programmers, then I will sign it like this:
sub create_orders { my $self = shift; my @directions = qw(NSWE); for my $ant ($self->my_ants) { my $index = int (rand (4)); $self->issue_order( $ant, $directions[$index] ); } }
We remain, we choose our opponent, we launch and observe how our blind kittens are devoured by the evil, ruthless ants of the opponent.
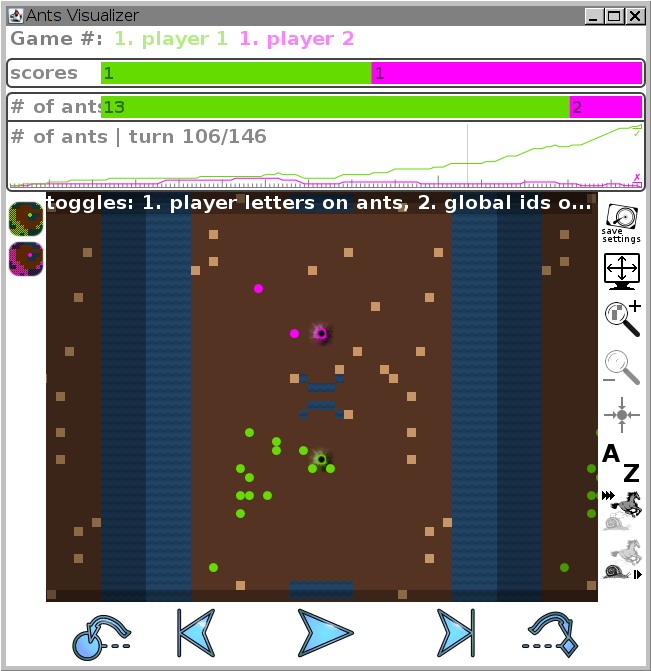
Well. The bot is ready, albeit stupid, but its. Things are easy: add intelligence bot, teach him to search for food, to fight, and you can send him to win. Here our paths diverge.
Options for finding food and rivals leave for self-study. See the Ants.pm file.
And in conclusion, I want to say a few words about registration.
Correct link to register here:
beta.aichallenge.org/submit.phpThe site in some places given the wrong link (apparently to the last championship), which scared me at the beginning and I almost gave up this venture.
After registration, you can download the original starter pack, as is, in the archive, so that your name appears as soon as possible in the ratings.
See you on the battlefield!